JavaScript Automation: The Definitive Guide
Author: The MuukTest Team
Published: May 16, 2025
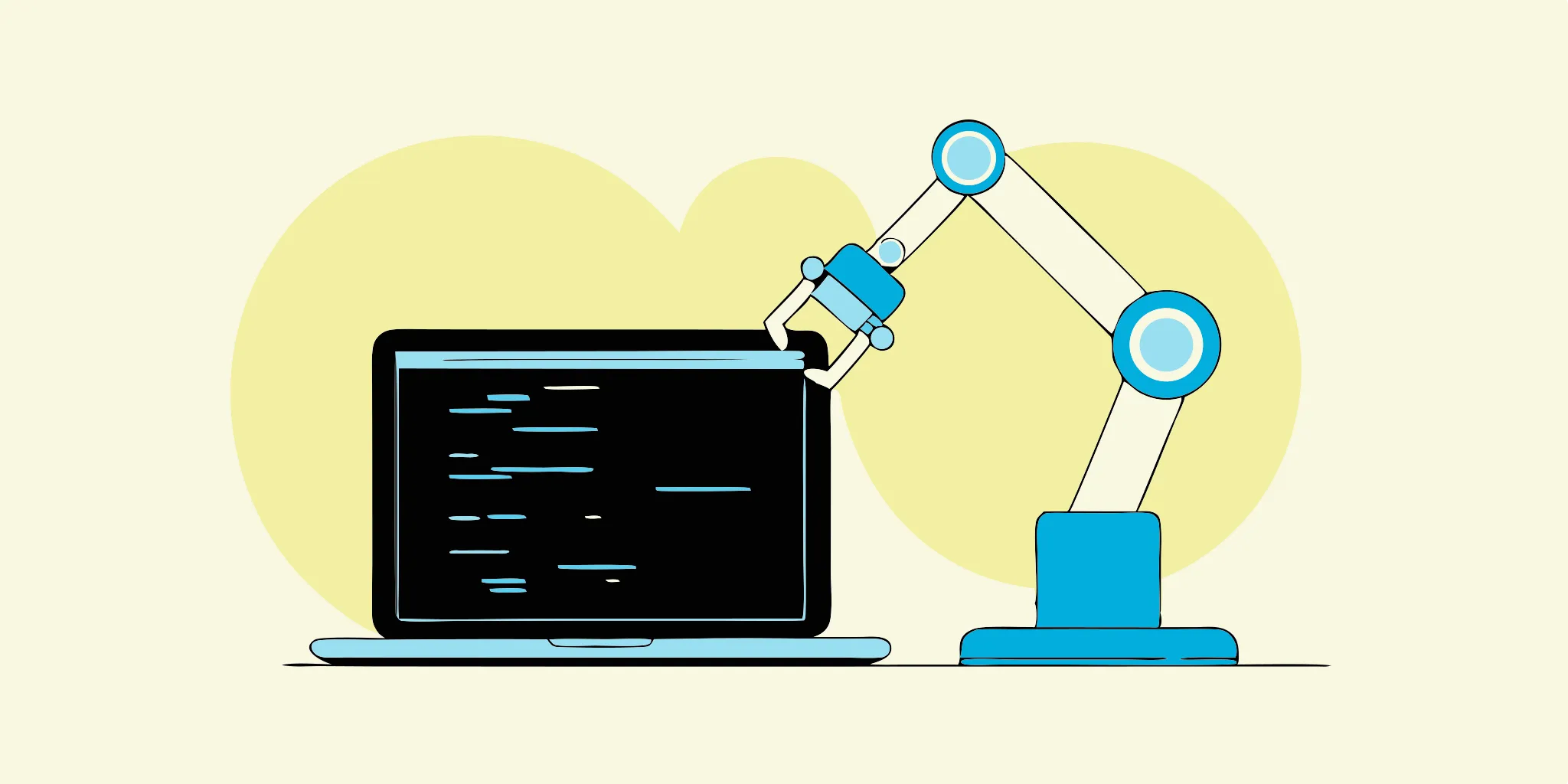
Table of Contents
Is manual testing slowing you down? If you're already working with JavaScript, using it for automation testing is a natural next step. This post explores how JavaScript automation can streamline your workflow and improve the quality of your web applications. We'll cover the key benefits, best practices, and even some advanced techniques for writing efficient and reliable tests. Whether you're a seasoned pro or new to JavaScript automation testing, this guide offers practical advice to transform your testing process.
Key Takeaways
- JavaScript offers significant advantages for web app testing: Its prevalence in web development, along with a rich ecosystem of testing frameworks, simplifies testing and improves team efficiency. Using JavaScript for both development and testing streamlines workflows.
- Building a robust JavaScript test suite requires a strategic approach: Select the right framework for your needs, write clear and concise tests, handle asynchronous operations effectively, and use page object models for better organization. Address common testing challenges like flaky tests and dynamic elements to ensure reliable results.
- Maintaining and optimizing your JavaScript tests is crucial for long-term success: Integrate tests into your CI/CD pipeline, analyze test results for actionable insights, and stay current with evolving JavaScript testing trends. Regularly review and refine your tests to ensure they remain efficient and effective.
Why Use JavaScript for Test Automation?
JavaScript is a versatile programming language used extensively in web development. It empowers developers to build interactive and dynamic web apps. Its widespread use makes it a natural fit for test automation, especially for web apps. Many developers already have JavaScript skills, simplifying the learning curve for automated testing. This is particularly helpful when testing applications built with popular JavaScript frameworks like React, Angular, or Vue.js. Using the same language for development and testing streamlines workflows and improves communication within teams.
Essential JavaScript Testing Features
Several key features make JavaScript ideal for test automation. It supports a rich ecosystem of testing frameworks and libraries, such as Mocha, Jasmine, and Jest. These tools offer structure and best practices for writing and organizing tests, increasing efficiency. JavaScript's asynchronous nature is another significant advantage. Asynchronous operations are common in web applications, and JavaScript handles them effectively. This capability is crucial for testing applications that rely on asynchronous processes, ensuring accurate and reliable test results.
JavaScript in Web Development and Testing
JavaScript plays a vital role in modern web development. It allows developers to create rich user interfaces and dynamic content, improving user experience. Its importance carries over to testing, where it enables automating user interactions and validating application behavior. Combining Selenium with JavaScript creates a powerful approach to web testing, providing comprehensive coverage for applications, especially those built with JavaScript frameworks. This synergy between development and testing using JavaScript leads to faster development cycles and higher quality software.
Benefits of JavaScript for Test Automation
JavaScript has emerged as a leading choice for test automation, especially for web applications. Its widespread use makes it a natural fit for testing, offering several key advantages:
1. Seamless Integration with Web Technologies: JavaScript’s prevalence in web development simplifies testing and improves team efficiency. Many developers are already familiar with JavaScript, which eases the transition to automated testing. This is particularly beneficial when testing applications built with popular JavaScript frameworks like React, Angular, or Vue.js. Using the same language for both development and testing streamlines workflows and enhances team communication. Plus, a rich ecosystem of JavaScript testing frameworks further simplifies the process. This can be especially helpful for teams looking to implement a solution like MuukTest's AI-powered test automation services quickly and efficiently.
2. Rich Ecosystem of Testing Frameworks: JavaScript boasts a robust ecosystem of testing frameworks and libraries, such as Mocha, Jasmine, and Jest. These tools provide structure and best practices for writing and organizing tests, significantly increasing efficiency. They offer a range of features, from simple unit testing to complex end-to-end testing, allowing developers to select the right tools for their specific needs and build a comprehensive test suite. This flexibility is a major advantage for teams seeking customized testing solutions. For instance, MuukTest leverages this rich ecosystem to provide tailored solutions that meet specific client requirements.
3. Effective Handling of Asynchronous Operations: JavaScript’s asynchronous nature is a significant advantage for test automation. Asynchronous operations are common in web applications, and JavaScript handles them effectively. This capability is crucial for testing applications that rely on asynchronous processes, ensuring accurate and reliable test results. Testing frameworks like Mocha provide features specifically designed for managing asynchronous tests, making it easier to write tests that accurately reflect real-world application behavior. This is a key aspect of achieving comprehensive test coverage, a core focus of MuukTest.
4. Comprehensive Coverage with Selenium: Combining JavaScript with Selenium creates a powerful approach to web testing. Selenium is a widely used open-source framework for automating web browsers, and its integration with JavaScript provides comprehensive coverage for applications, particularly those built with JavaScript frameworks. This synergy between development and testing using JavaScript leads to faster development cycles and higher quality software. For robust, cross-browser testing, Selenium WebDriver, controlled by JavaScript, is an excellent choice. This aligns with MuukTest's commitment to delivering complete test coverage within 90 days, as highlighted on our pricing page.
Get Started with JavaScript Test Automation
This section provides a practical starting point for implementing automated tests with JavaScript. We'll cover setting up your environment and choosing a suitable framework.
Preparing Your JavaScript Testing Environment
Before writing tests, ensure you have the correct tools. First, install Node.js and npm. Node.js is essential for running JavaScript code outside a web browser, while npm (Node Package Manager) helps manage project dependencies. After installation, verify everything works by checking the versions in your terminal with node -v
and npm -v
. Next, create a new project directory and initialize a Node.js project inside it using npm init -y
. This creates a package.json
file to manage your project's settings and dependencies. Finally, choose a suitable IDE. VS Code is a popular choice for JavaScript development, offering robust features and helpful extensions. You can then install testing packages. For Selenium-based testing, use npm install selenium-webdriver
. This package provides the bindings to interact with web browsers.
Choosing the Right JavaScript Testing Framework
JavaScript, commonly used for building web apps, is a natural fit for testing those same applications. This simplifies testing since your application and tests use the same language. For UI testing, consider Selenium WebDriver with JavaScript. Selenium provides a way to control web browsers programmatically, allowing you to simulate user interactions and verify application behavior. For more complex test suites, a testing framework like Mocha or Jest is helpful. These frameworks provide structure, helpful functions for writing tests (like assertions), and tools for running tests and reporting results, making it easier to organize and maintain your growing test suite. If you're working with modern web apps built with frameworks like React, Angular, or Vue.js, look into Cypress or Puppeteer, which offer features specifically designed for testing these types of applications.
Manual vs. Automated Testing
Choosing between manual and automated testing depends on your project’s specific needs. Manual testing, where a human tester interacts with the software, is great for usability and exploratory testing. Think of it as having a real user try out your application, uncovering unexpected bugs and providing feedback on the user experience. This approach is adaptable to quick requirement changes, which is essential in agile development environments. However, manual testing can be time-consuming, prone to human error, and doesn’t scale well for large projects. Imagine having to manually retest every feature after each code change—it quickly becomes impractical.
Automated testing, on the other hand, uses scripts to execute tests, making it fast, efficient, and repeatable. This significantly improves test coverage and integrates well with CI/CD pipelines, a cornerstone of modern development workflows. Automated tests excel at regression testing, ensuring that new code doesn’t break existing functionality. The downside? Automated testing requires specialized skills and an initial setup investment. Plus, it can’t replace human judgment in usability or exploratory testing.
The sweet spot often lies in a balanced combination of manual and automated testing. Leverage manual testing for user experience feedback and exploratory testing, while automating repetitive tasks like regression testing. This approach ensures comprehensive test coverage and efficient use of resources.
Task Runners for Automating Build Processes
Beyond testing itself, consider automating your entire build process. Task runners like Grunt, Gulp, and npm scripts are invaluable tools for this. They automate repetitive tasks such as code linting, minification, and adding CSS prefixes. These tasks, while essential, can be tedious and time-consuming to perform manually. Task runners streamline the build process, freeing you to focus on writing code rather than managing these tasks.
For example, Gulp, a popular JavaScript task runner, simplifies complex build processes. You can define tasks to compile your Sass files into CSS, minify your JavaScript code, and optimize images, all with a single command. Combining task runners with browser automation systems like Selenium creates a comprehensive testing workflow that significantly enhances productivity. Imagine running your entire test suite automatically after each code change—that’s the power of task runners and automated testing combined. Services like MuukTest can further streamline this process by providing AI-powered test automation that integrates seamlessly into your existing workflows. Check out our test automation services to learn more.
Top JavaScript Test Automation Frameworks
Understanding the available testing frameworks is essential for effective JavaScript test automation. Let's explore some popular options and their strengths.
Selenium WebDriver with JavaScript for Automation
Selenium is a widely used open-source tool for automating web browsers. It supports multiple programming languages, including JavaScript, making it versatile for testing web applications. With Selenium WebDriver, you can write tests that directly interact with web elements, mimicking user actions and validating functionality across different browsers. This ensures comprehensive test coverage and helps identify potential issues early.
Key Steps and Code Examples for Selenium and JavaScript
To effectively use Selenium with JavaScript for test automation, follow these essential steps:
- Install Prerequisites: Start by installing Node.js and npm. Node.js lets you run JavaScript outside a web browser. Verify your installation by checking the versions in your terminal with
node -v
andnpm -v
. - Initialize Your Project: Create a project directory and initialize a Node.js project inside using
npm init -y
. This generates apackage.json
file to manage your project’s settings and dependencies. - Install Selenium WebDriver: Use
npm install selenium-webdriver
to add the necessary Selenium tools. This package provides the bindings to interact with web browsers. Also, download the appropriate browser driver for Chrome, Firefox, or whichever browser you plan to use for testing. - Write Your First Test: Create a new file (e.g.,
firstTest.js
) and start with a simple test. This example automates a Google search:
This code opens Chrome, goes to Google, enters "Selenium" into the search bar, and presses Enter. Remember to add assertions to validate the test results. For example, you could assert that the page title contains "Selenium". Chai is a popular assertion library you can use with Selenium and JavaScript.
- Explore More Complex Scenarios: As you become more comfortable, expand your tests to interact with different web elements like buttons, dropdowns, and forms. Practice handling asynchronous operations with
async
andawait
. The Page Object Model (POM) can help organize and maintain your tests, especially as your test suite grows. POM keeps your test code clean and efficient.
By following these steps and expanding on these code examples, you can create a robust test suite with Selenium and JavaScript, improving the quality of your web applications. Services like MuukTest offer a faster, more comprehensive approach to test automation if you're looking for a managed solution.
Cypress for Modern Web App Testing
Cypress is a modern JavaScript testing framework built for today's web applications. It offers a fast, reliable testing experience, especially for end-to-end testing. Cypress simplifies setup and provides rich features for interacting with and testing web elements. While it excels in modern web app testing, Cypress has limited support for mobile applications and primarily works with Chrome-family browsers, WebKit, and Firefox.
Cypress.io Key Features and Statistics
Cypress.io shines with features designed for modern web app testing. Tests run directly in the browser, giving developers real-time feedback and speeding up debugging. This tight browser integration makes Cypress especially well-suited for testing complex user interactions and dynamic web applications. Setting up Cypress is generally straightforward, and its API offers robust commands for interacting with web elements, making it accessible even for developers new to automated testing. It also integrates easily with popular Continuous Integration (CI) providers, enabling automated testing within your development pipeline.
Beyond the core framework, Cypress offers a cloud-based service with advanced features. This includes test parallelization for faster feedback loops, visual debugging of CI failures, test suite analytics, and integrations with tools like Slack and Jira. The popularity and community support behind Cypress are impressive, with over 5.3 million weekly downloads, 46,000+ GitHub stars, and 1.3 million+ dependent repositories, indicating a vibrant and active community. This strong community contributes to readily available resources and support for those using Cypress.
Puppeteer for Headless Browser Automation
Puppeteer, a Node.js library, provides a high-level API for controlling headless Chrome or Chromium. This makes it powerful for automating various browser tasks, including generating screenshots, crawling web pages, and running tests. Puppeteer's ease of use and stability make it a popular choice for automating browser interactions and performing tasks like performance and UI testing.
Jest and Mocha for JavaScript Testing
For unit and integration testing, Jest and Mocha are excellent options. Jest, known for its simplicity, is particularly well-suited for React projects. It offers a comprehensive API and integrates seamlessly with various tools. Mocha, a flexible framework, lets developers choose their preferred assertion libraries and works well for both Node.js and browser testing. Both frameworks help build robust and reliable JavaScript applications through thorough component and interaction testing.
In-Depth Framework Comparison (WebdriverIO, Cypress, TestCafe, Playwright, Puppeteer)
Choosing the right framework depends on your specific needs and project requirements. Here’s a closer look at popular choices to help you make an informed decision. For a service that streamlines this process and guarantees comprehensive test coverage, consider exploring MuukTest's AI-powered test automation services.
WebdriverIO is a powerful and versatile choice. It supports both browser and native mobile app testing, offering a broad reach for various application types. Its extensibility is a major plus, allowing customization for complex testing scenarios. Plus, it integrates well with popular testing libraries like Mocha, Jasmine, and Cucumber. If you need a solution that can handle a wide range of testing needs, WebdriverIO is worth considering. For a more tailored and efficient approach, see how MuukTest helps leading companies achieve comprehensive testing.
Cypress is known for its ease of setup and developer-friendly experience, making it a good option for front-end developers getting started with testing. It shines in front-end testing and can handle some back-end testing as well. However, be aware of its limitations in mobile app and broader browser support. If your focus is primarily front-end testing with standard requirements, Cypress might be a good fit. If you anticipate needing more extensive coverage in the future, consider a solution like MuukTest, designed for scalability.
TestCafe is another strong contender, particularly for its ease of deployment and support for both JavaScript and TypeScript. It supports most major desktop browsers but, like Cypress, has limited mobile app support. TestCafe excels at client-side testing for desktop and mobile web apps, but it's not suitable for unit or integration testing. Keep this in mind when evaluating your testing needs. If you're looking for a faster way to get started with comprehensive testing, explore MuukTest's quickstart options.
Playwright is a relatively new but promising open-source framework. Its multi-language support (JavaScript, .NET C#, Java, Python) is a significant advantage, broadening its appeal to diverse development teams. Playwright supports most modern browsers (except Internet Explorer) and handles both desktop and mobile testing. However, being newer, it has fewer integrations compared to more mature frameworks like WebdriverIO. If cross-browser and cross-platform support is a priority, and you're comfortable with a framework that's still evolving, Playwright is worth exploring. For a solution with robust existing integrations and a proven track record, consider MuukTest.
Finally, Puppeteer offers a stable and mature platform, particularly for developers focused on Chrome and Chromium. It's easy to deploy and JavaScript-first, making it a natural fit for JavaScript developers. While primarily focused on desktop app testing, some Firefox support is in development. If your testing needs are centered around Chrome and Chromium, Puppeteer is a reliable choice. For a more comprehensive solution that covers a wider range of browsers and testing needs, consider other options like WebdriverIO or Playwright. Or, explore how MuukTest can provide complete test coverage within 90 days, simplifying your testing process and accelerating your development lifecycle.
Writing Effective JavaScript Automated Tests
Once you’ve chosen a framework, you can start writing tests. But before you dive in, let’s review some essential techniques for writing effective JavaScript tests. These best practices will not only improve the quality of your tests but also make them easier to maintain and scale as your project grows.
JavaScript Testing Best Practices
Writing clear, concise, and well-structured tests is crucial for long-term maintainability. Think of your tests as living documentation of your application's behavior. If they're difficult to understand, they lose their value. Start by defining clear objectives for your tests. What exactly are you trying to verify? Avoid overly complex tests—keep them focused on a single unit of functionality. This targeted approach makes it easier to pinpoint issues when tests fail. Adhering to best practices for test case design ensures your tests are reliable and effective, minimizing false positives or negatives. A test should have a clear purpose and defined objectives to avoid ambiguity and ensure effective validation.
Handling Asynchronous Operations in JavaScript Tests
Asynchronous operations are common in JavaScript, especially when dealing with web applications. Testing these operations requires special handling. Promises, callbacks, and async/await are all valuable tools in your testing arsenal. Make sure your testing framework supports these asynchronous patterns. Most modern frameworks provide mechanisms to handle promises and asynchronous functions, allowing you to write tests that accurately reflect the behavior of your application. Remember to test at different layers, including unit, integration, and end-to-end tests, to ensure comprehensive coverage of your asynchronous code. Consider using a framework that provides robust support for asynchronous testing to simplify the process and ensure accurate results.
Implementing Page Object Models in JavaScript
For UI testing, the Page Object Model (POM) is a powerful pattern. A POM represents a web page as a JavaScript class. This class contains properties that represent elements on the page and methods that represent actions you can perform on the page. Using a POM organizes your test code and makes it easier to maintain. By separating test logic from element locators, you create a more robust and adaptable test suite. If the structure of your web page changes, you only need to update the POM, not every test that interacts with that page. Learn how to implement the PageObject pattern to create a more maintainable and scalable test suite.
Advanced JavaScript Test Automation Strategies
As you gain more experience with JavaScript test automation, exploring advanced techniques can significantly improve the quality and efficiency of your tests. These techniques help create more robust, maintainable, and reliable test suites.
Mocking and Stubbing for Isolated JavaScript Tests
Mocking and stubbing are crucial for isolating the unit of code you're testing. Think of it like creating a controlled environment where you can dictate the behavior of external dependencies. By substituting real dependencies with mock objects, you gain precise control over their responses, allowing you to focus solely on the unit's behavior. This isolation prevents external factors from influencing your test results and simplifies debugging. For example, if your function depends on an API call, you can mock the API response to simulate different scenarios without actually making the request. This not only speeds up your tests but also makes them more predictable.
Data-Driven Testing with JavaScript
Data-driven testing lets you run the same test multiple times with different input values. This is incredibly useful for verifying how your application handles various data sets and edge cases. Imagine testing a login form—you could use data-driven testing to try different username and password combinations, including valid and invalid credentials. This approach ensures comprehensive coverage and helps uncover unexpected behavior related to specific data inputs. Many testing frameworks provide built-in support for data-driven testing, making it easy to implement.
Managing Dynamic Elements with JavaScript
Dynamic elements, common in modern web applications, can be tricky to test due to their ever-changing nature. Elements that appear, disappear, or change attributes based on user interactions or asynchronous operations require special handling in your tests. Employing techniques like explicit waits ensures your tests interact with elements only when they are available in the DOM. Using CSS selectors and XPath provides powerful ways to target specific elements, even if their properties change dynamically. Understanding how to manage these elements is key to building reliable and robust tests for dynamic web applications.
Troubleshooting JavaScript Automation Challenges
Even with the best tools, test automation has its hurdles. Let's break down some common roadblocks in JavaScript automation and how to address them.
Selecting Efficient Locators in JavaScript
A robust locator strategy is fundamental to reliable tests. Think of locators as the addresses you use to find elements on a web page. If the address is incorrect or changes frequently, you won't find what you're looking for. Prioritize CSS selectors whenever possible. They're generally faster and more resilient to DOM changes than XPath. A well-chosen CSS selector keeps your tests stable, even with minor UI updates.
Handling Flaky JavaScript Tests
Flaky tests—those that pass sometimes and fail others without code changes—are a major source of frustration. They erode trust in your test suite and can stall your development process. Often, flaky tests stem from timing issues, especially with asynchronous operations. Implement proper waiting strategies to ensure elements are loaded and ready before interacting with them. Investing in robust testing frameworks and training your team also minimizes flakiness. This upfront investment in quality tools and skilled team members pays off by reducing debugging time and increasing the reliability of your test results. Addressing the challenges in automation testing head-on improves the overall efficiency of your testing process.
Cross-Browser Testing with JavaScript
Your web application needs to function seamlessly across different browsers and devices. Cross-browser testing ensures a consistent user experience regardless of how someone accesses your site. While testing on your local machine is a good starting point, running tests on a real device cloud like BrowserStack offers more accurate results. Cloud-based testing provides access to a wider range of browsers and operating systems, giving you greater confidence in your application's compatibility.
Browser Automation Systems and Services
Browser automation systems and services are essential for efficient and comprehensive testing of web applications. These tools let you automate interactions with web browsers, simulating user actions and validating application behavior across different browsers and operating systems. Let's explore some key players in this space.
Selenium is a widely used open-source tool for automating web browsers. It supports multiple programming languages, including JavaScript, making it versatile for testing web applications. With Selenium WebDriver, you can write tests that directly interact with web elements, mimicking user actions and validating functionality across different browsers. This direct interaction ensures comprehensive test coverage and helps identify potential issues early. Selenium's flexibility and broad language support make it a popular choice for many testing scenarios.
Commercial services like Sauce Labs, BrowserStack, and TestingBot offer convenient remote testing environments. These services provide access to a wide range of browser and operating system combinations, eliminating the need for complex local setups. Their APIs allow programmatic access for advanced automation, integrating seamlessly with your existing workflows. This cloud-based approach simplifies cross-browser testing and ensures your application works correctly for users on different platforms. These services can be particularly valuable when testing on less common browsers or operating systems.
For more modern JavaScript-based testing, frameworks like Cypress and Puppeteer offer specialized solutions. Cypress provides a fast and reliable testing experience, especially for end-to-end testing of modern web applications. It simplifies setup and offers rich features for interacting with and testing web elements. Puppeteer, a Node.js library, provides a high-level API for controlling headless Chrome or Chromium. This makes it powerful for automating various browser tasks, including generating screenshots, crawling web pages, and running tests. Puppeteer’s ease of use and stability make it a popular choice for automating browser interactions.
Optimizing JavaScript Test Automation
Once you’ve set up your JavaScript test automation, optimize it for performance, scalability, and maintainability. This ensures your tests run efficiently and provide valuable insights.
Improving JavaScript Test Performance and Scaling
Performance is key in test automation. Slow tests can bottleneck your development cycle. Focus on writing efficient test code, minimizing unnecessary actions, and leveraging browser caching. Consider implementing parallelization to run tests concurrently, significantly reducing overall execution time. If you're working with a large test suite, explore cloud-based testing platforms for on-demand scaling of your testing infrastructure. This approach helps you handle peak loads and ensures consistent performance. While there can be a high initial investment in automation tools, the right strategies and tools mitigate these costs and deliver higher quality applications.
Integrating JavaScript Tests into CI/CD
Integrating your JavaScript tests into your CI/CD pipeline is crucial for continuous feedback and faster releases. This automation allows developers to focus on new features and code quality. Set up automated triggers to run your tests after every code commit or merge. This helps catch regressions early and prevents bugs from making their way into production. Choose a CI/CD platform that seamlessly integrates with your chosen JavaScript testing framework. This simplifies the setup process and ensures a smooth workflow. While automation testing accelerates execution, it also presents challenges that can impact efficiency if not properly integrated.
Integrating with MuukTest for Comprehensive Test Coverage
Thorough test coverage is often a challenge for development teams. Balancing speed, cost, and quality can be tricky. MuukTest offers a solution by blending AI-powered test automation with expert QA services. This helps teams quickly find and fix weaknesses in their current testing. MuukTest works with clients to achieve complete test coverage within 90 days, making sure all key features are carefully tested. This reduces the chance of bugs in production and lets developers focus on building new features. Whether you have a complex web application or a simple mobile app, MuukTest’s solutions adapt to your needs. See how other companies have used MuukTest and explore pricing options or the quickstart guide.
Reporting and Analyzing JavaScript Test Results
Clear reporting and analysis of test results are essential for understanding the health of your application. Employ automated test result analysis tools that provide clear dashboards and insights. These tools can help you identify trends, pinpoint failing tests, and track the overall progress of your testing efforts. Integrate with reporting platforms like Grafana or Kibana to generate comprehensive reports and visualizations. Make sure your reports are easily accessible to the entire development team, facilitating collaboration and faster issue resolution. When developers are quickly notified of issues, they can address them promptly. Regularly review and refine your tests to ensure they accurately reflect expected system behavior. This collaborative approach improves the effectiveness of your JavaScript test automation. Automated testing has revolutionized software quality assurance, enabling faster releases and continuous integration.
Writing Maintainable JavaScript Test Code
Writing clean, maintainable JavaScript test code is crucial for long-term success in test automation. As your project grows, well-structured tests are easier to understand, update, and debug, saving you time and frustration. This section covers some key best practices to help you write robust and maintainable JavaScript test code.
Organizing JavaScript Test Code
Keep your test files concise and focused. Each test file should ideally test a specific feature or component. Break down large test suites into smaller, manageable modules. This improves code readability and makes it easier to isolate and fix issues. Avoid writing overly complex tests. If a test becomes too long or convoluted, consider refactoring it into smaller, more focused tests. This modular approach makes your test suite more efficient and easier to maintain. Think of it like organizing your closet—everything is easier to find when it has its place. This also helps prevent common test automation mistakes that lead to inefficient and difficult-to-maintain tests.
Using Assertions Effectively in JavaScript
Assertions are the backbone of your tests. They verify that your application behaves as expected. Use clear and descriptive assertions to make it obvious what each test is checking. Frameworks like Jest and Chai offer a variety of assertion styles. Choose the style that best enhances the readability of your tests. Effective assertions, combined with robust locators, ensure that your tests are reliable and maintainable. A good JavaScript tutorial can offer more guidance on using assertions and locators effectively. Remember, the goal is to make your tests easy to understand and update, so choose your assertions wisely.
Staying Current with JavaScript Testing Trends
The JavaScript ecosystem is constantly evolving. New tools, frameworks, and best practices emerge regularly. Staying informed about these changes can significantly impact the effectiveness of your test automation. Subscribe to relevant blogs, follow industry experts, and participate in online communities to keep your skills sharp. Explore new testing frameworks and tools as they become available. Consider adopting new techniques like visual regression testing or incorporating AI-powered testing tools. Continuous learning is essential for writing efficient and maintainable JavaScript tests and delivering high-quality applications. Understanding the challenges and solutions in JavaScript testing frameworks is a good starting point. By staying current, you can ensure your tests remain robust and relevant, and your automation efforts continue to deliver value.
JavaScript vs. Other Test Automation Languages
Choosing the right language for test automation depends on several factors, including your team's existing skills, the type of application, and the complexity of your testing needs. While other languages like Java, Python, and Ruby have their strengths, JavaScript offers unique advantages, especially for web applications.
Advantages of Using JavaScript for Automation
JavaScript excels in web testing because it's the foundation of many web apps. This allows developers to write tests in the same language as their application code, creating a more efficient workflow and tighter integration. Using JavaScript simplifies things for your team, who can often handle both development and testing without switching languages. This streamlines communication and reduces context switching.
JavaScript also has a rich ecosystem of testing frameworks. Tools like Cypress, Jest, and Mocha provide robust features for writing and running tests, from simple unit tests to complex end-to-end scenarios. These frameworks are easy to set up, offer rich APIs, and have strong community support, making JavaScript practical for teams of all sizes. Because it runs directly in the browser, JavaScript enables real-time feedback during testing, speeding up development. This tight browser integration makes it easier to quickly identify and fix issues. You can explore getting started with Selenium and JavaScript through readily available resources.
Language Comparison (Python, Java, JavaScript)
Choosing the right language for test automation depends on several factors, including your team's existing skills, the type of application, and the complexity of your testing needs. While other languages like Java and Python have their strengths, JavaScript offers unique advantages, especially for web applications.
JavaScript excels in web testing because it's the foundation of many web apps. This allows developers to write tests in the same language as their application code, creating a more efficient workflow and tighter integration. Using JavaScript simplifies things for your team, who can often handle both development and testing without switching languages. This streamlines communication and reduces context switching. Plus, a wealth of JavaScript testing frameworks makes getting started a breeze.
JavaScript also boasts a rich ecosystem of testing frameworks. Tools like Cypress, Jest, and Mocha provide robust features for writing and running tests, from simple unit tests to complex end-to-end scenarios. These frameworks are easy to set up, offer rich APIs, and have strong community support, making JavaScript practical for teams of all sizes. Because it runs directly in the browser, JavaScript offers real-time feedback during testing, which speeds up development. This tight browser integration makes it easier to quickly identify and fix issues. Selenium and JavaScript work beautifully together for comprehensive web testing.
While many engineers prefer Python for UI test automation because of its readability and extensive libraries, it might require additional setup for front-end testing. Java, with its established frameworks like JUnit and TestNG, offers robust features for managing Java tests, but can be more verbose than JavaScript. Ultimately, the best language for your team depends on your specific project needs and the skillset of your team. If your focus is primarily web applications and your team is already proficient in JavaScript, it's a strong contender for streamlining your testing process.
When to Consider Alternatives to JavaScript
While JavaScript is powerful for web app testing, other languages might be a better fit in certain situations. For complex testing scenarios, especially those with intricate backend systems or specialized performance requirements, languages like Java or Python might offer more flexibility and specialized libraries. These languages often have mature ecosystems for handling complex tasks.
Another factor is the initial investment in a JavaScript-based testing infrastructure. While many JavaScript tools are open-source, costs are still associated with training, tooling, and integration. If your team already has expertise in another language like Java or Python, using those skills might be more cost-effective.
Finally, for extremely performance-intensive tests, JavaScript might not always be optimal. Languages like Java, known for its performance, could be more suitable. Assess your performance needs and choose a language that handles the scale and complexity of your tests. Guides addressing challenges in automated testing can inform your decisions.
Frequently Asked Questions
Why should I use JavaScript for test automation?
JavaScript is a good choice for test automation, especially for web apps, because it's commonly used for building them. This simplifies testing since your application and tests use the same language, streamlining workflows and improving team communication. Plus, JavaScript has a rich ecosystem of testing frameworks and libraries like Mocha, Jasmine, and Jest, which offer structure and best practices for more efficient testing.
What are some popular JavaScript test automation frameworks?
Selenium WebDriver is a widely used option for automating web browsers, allowing you to simulate user interactions. Cypress is a modern framework specifically designed for testing modern web applications, offering a fast and reliable testing experience. Puppeteer is excellent for controlling headless Chrome or Chromium, useful for tasks like generating screenshots and running tests. Jest and Mocha are popular for unit and integration testing, providing structure and helpful functions for building robust applications.
How do I handle asynchronous operations in my JavaScript tests?
Asynchronous operations are common in web applications, so handling them correctly in your tests is important. Modern JavaScript testing frameworks usually have built-in support for promises and asynchronous functions. Use async/await, promises, and callbacks to structure your tests and ensure they accurately reflect how your application behaves. Testing at different layers (unit, integration, and end-to-end) ensures comprehensive coverage of your asynchronous code.
What are some best practices for writing maintainable JavaScript test code?
Write clear, concise tests focused on a single unit of functionality. Organize your tests into smaller, manageable modules, and use a descriptive naming convention. Use assertions effectively to verify expected behavior. Keep your test code DRY (Don't Repeat Yourself) by using helper functions and page object models. Stay updated with the latest JavaScript testing trends and best practices.
How does JavaScript compare to other test automation languages?
JavaScript shines in web testing due to its tight integration with web browsers and the abundance of JavaScript-based web applications. It simplifies testing by allowing developers to use the same language for both development and testing. However, other languages like Java or Python might be more suitable for complex backend systems or performance-intensive tests. Consider your team's existing skills and the specific needs of your project when choosing a language.
Related Articles
Related Posts:
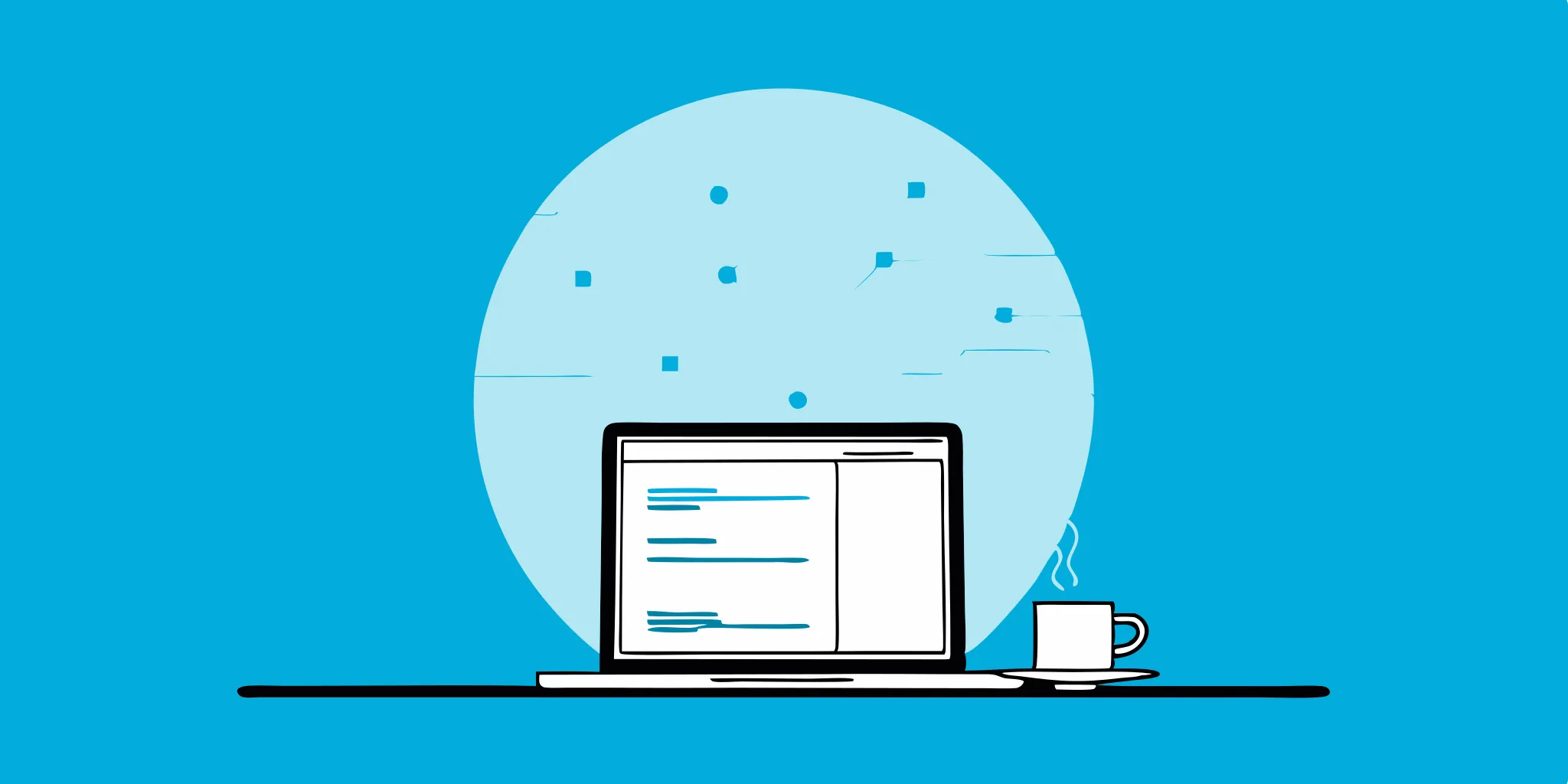
Practical Guide to Java Automation Testing
Master Java automation testing with this practical guide, covering essential tools, frameworks, and strategies to enhance your testing efficiency and reliability.
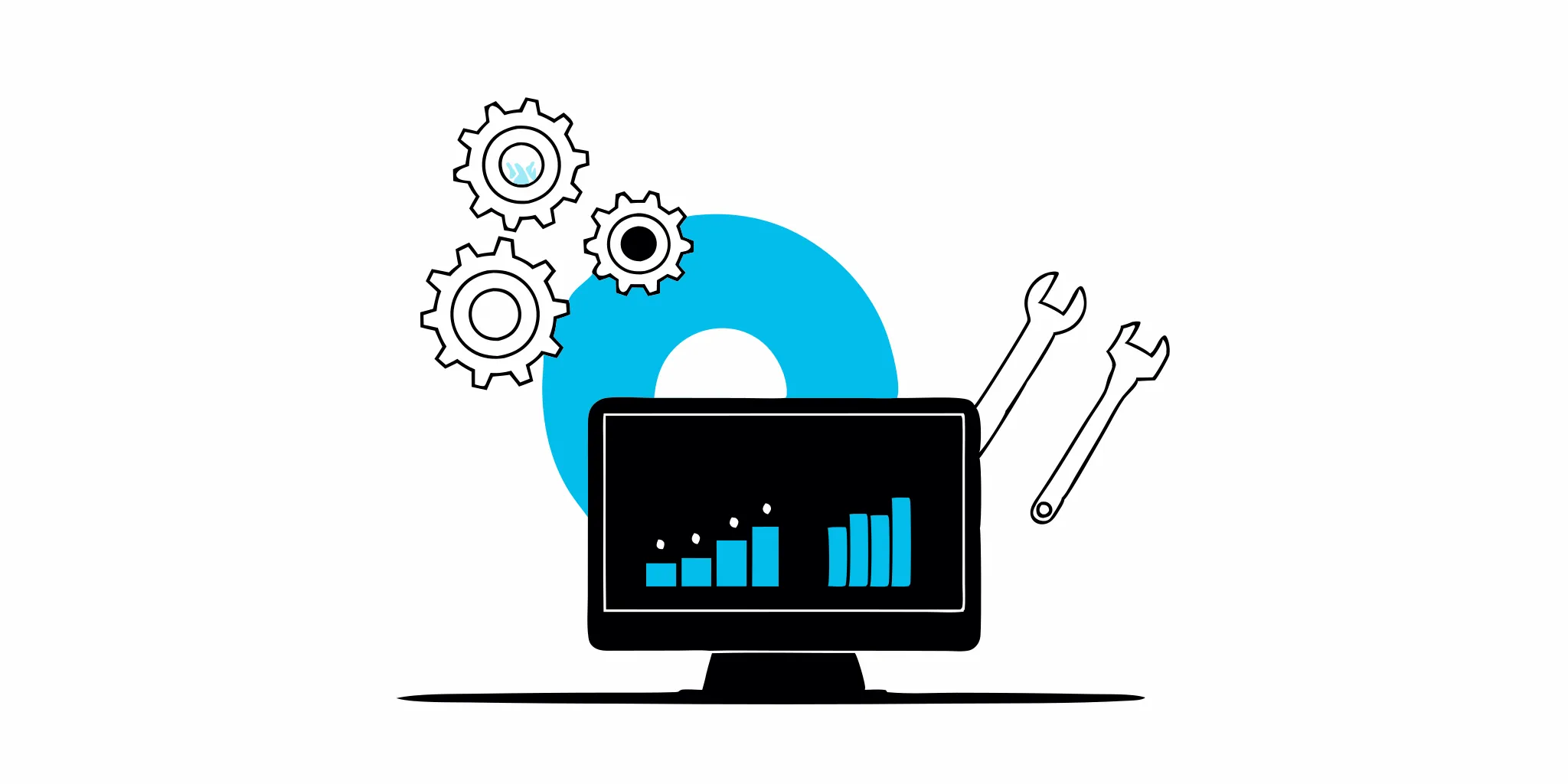
Best Software Testing Automation Tools for 2024
Find the best software testing automation tools to streamline your testing process. Explore top options and choose the right fit for your team's needs.
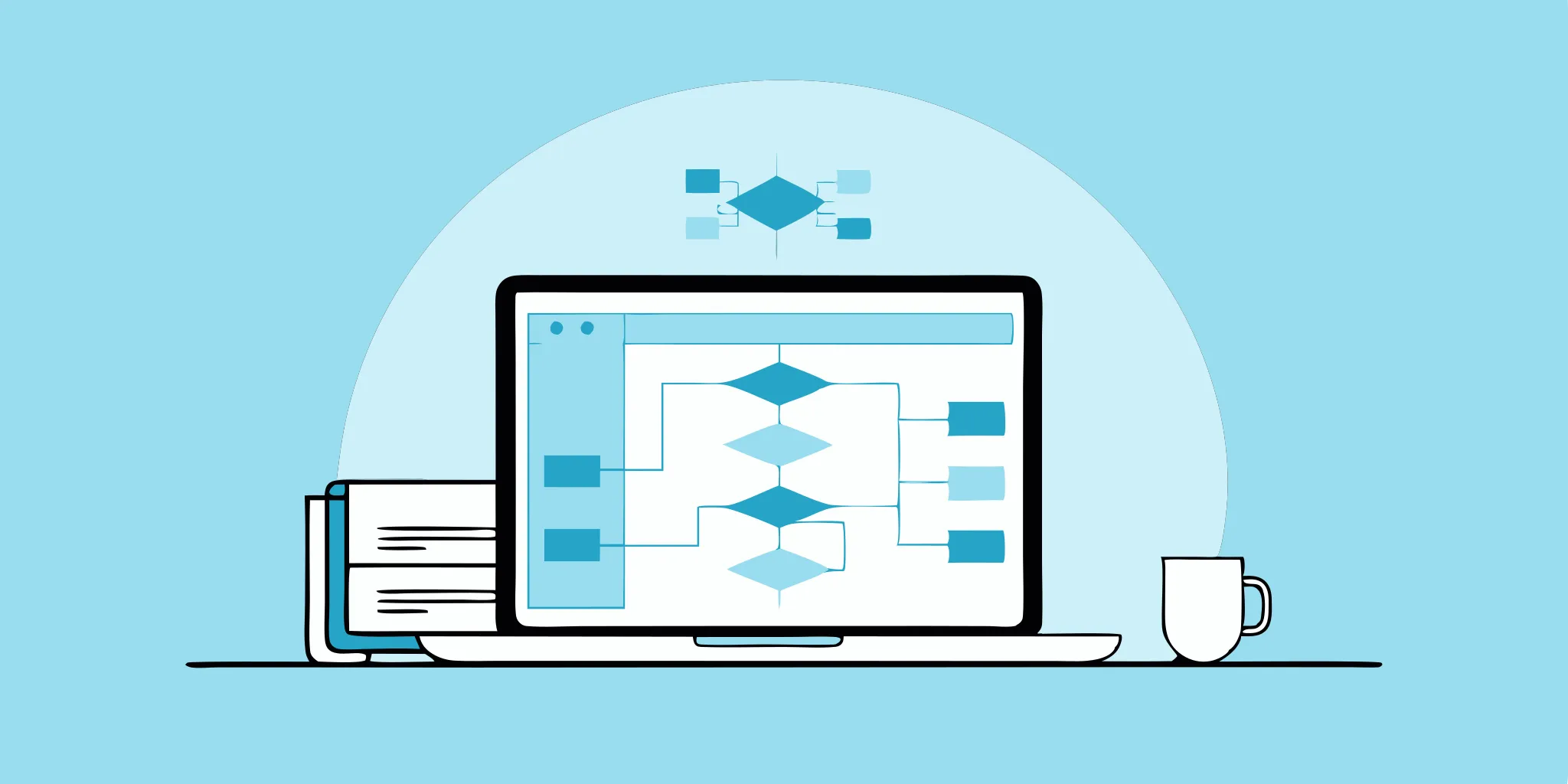
How to Choose a Testing Automation Company: A Practical Guide
In today's competitive market, releasing buggy software means serious consequences for businesses. Test automation is key in ensuring high-quality products and apps while also keeping up with the...