Practical Guide to Java Automation Testing
Author: The MuukTest Team
Published: April 17, 2025
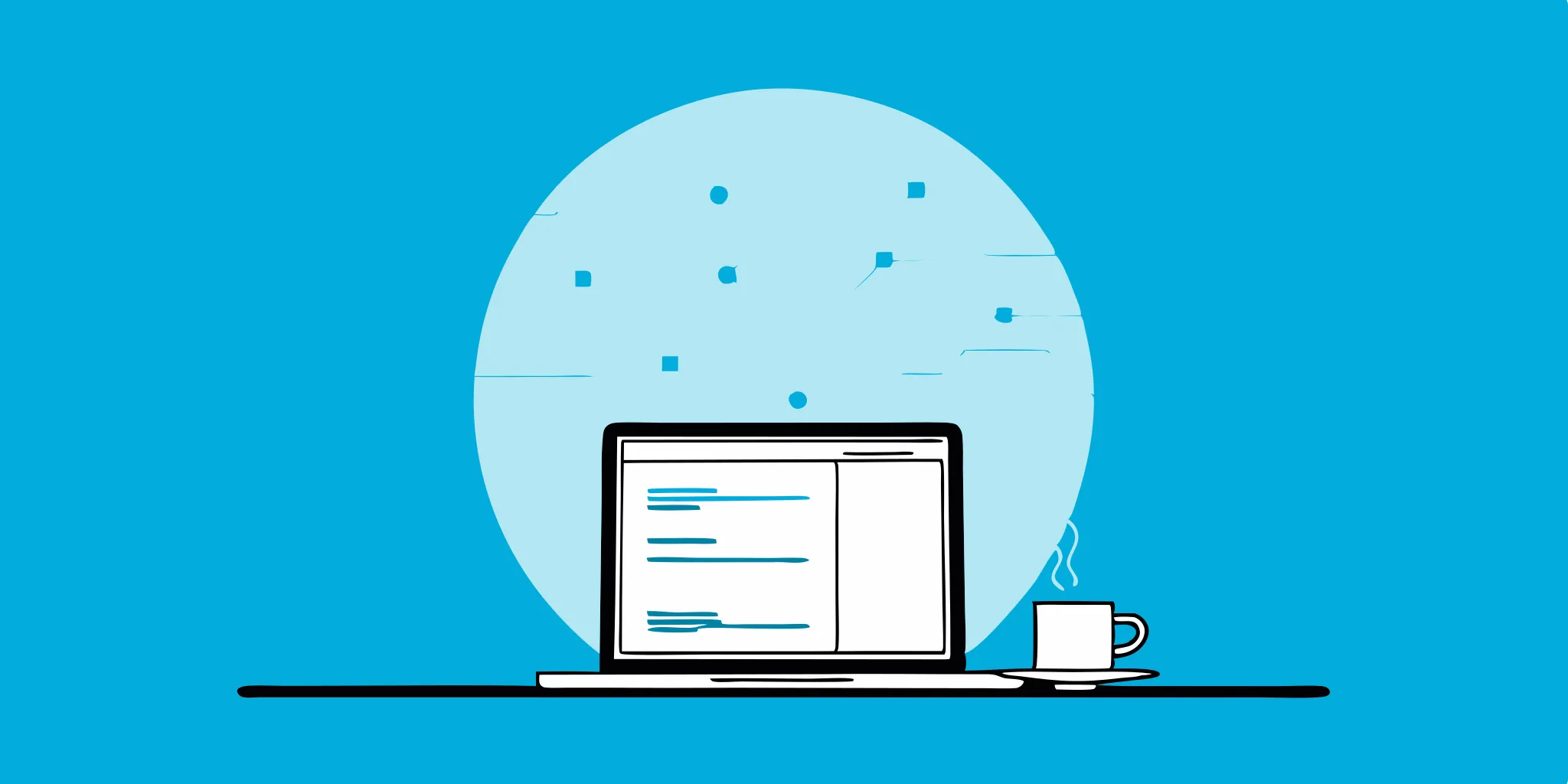
Table of Contents
Tired of endless manual testing? It's time-consuming and mistakes happen. Java automation testing offers a better way. It automates those repetitive tasks, giving you back valuable time and boosting accuracy. In this article, we'll explore Java automation testing from the basics to advanced techniques. We'll cover why Java is so popular for automation, introduce essential tools like Selenium and JUnit, and share practical tips for writing tests that are both effective and easy to maintain. Ready to transform your testing workflow? Let's get started.
Key Takeaways
- Java's strengths streamline test automation: Its versatility, extensive libraries, and platform independence simplify building reliable and efficient tests, making it a valuable skill for any QA professional.
- Structured testing is key for maintainability: Using design patterns like POM, understanding OOP principles, and mastering data structures and exception handling are crucial for creating scalable and manageable Java tests. CI/CD integration further enhances testing efficiency.
- Continuous learning is essential in testing: Exploring advanced Java techniques like multi-threading and detailed reporting, and staying updated on current trends, ensures your Java tests remain robust and performant.
Introduction to Java Automation Testing
Java plays a significant role in the world of automation testing. Its robust features and extensive ecosystem make it a popular choice for building and running automated tests. This section explores why Java is so well-suited for this purpose and provides a starting point for setting up your Java automation testing environment.
Why Choose Java for Automation Testing?
Java offers several advantages that make it a compelling choice for automation testing. Its platform independence, strong community support, and rich set of libraries contribute to its popularity among testers.
Benefits of Java's Strong Community and Ecosystem
Java boasts a large and active community, which translates to readily available resources, support forums, and a wealth of shared knowledge. This vibrant ecosystem ensures you can find solutions to common challenges and stay up-to-date with the latest trends in Java automation testing. Plus, Java's object-oriented nature promotes code reusability and maintainability, making it easier to create and manage complex test suites. This can be especially helpful when working with frameworks like Selenium, allowing you to create efficient and scalable Selenium Java scripts.
Cross-Platform Compatibility and Stability
Java's platform independence ("write once, run anywhere") is a major advantage for automation testing. This means you can write your Java test code on one operating system (like Windows) and run it seamlessly on others (like macOS or Linux) without modification. This flexibility simplifies cross-platform testing and reduces the need for platform-specific test scripts. Java's maturity as a language also contributes to its stability, making it a reliable choice for building robust automated tests. The wide range of testing tools compatible with Java further expands its capabilities, allowing you to handle various testing needs, from unit testing to end-to-end testing. For a faster start, consider exploring services like MuukTest's QuickStart for streamlined test automation setup.
Getting Started: Setting Up Your Environment
Before you can start writing and running Java automation tests, you need to set up your development environment. This involves installing the Java Development Kit (JDK), choosing an Integrated Development Environment (IDE), and setting up Selenium WebDriver.
Installing Java Development Kit (JDK)
The Java Development Kit (JDK) provides the necessary tools and libraries to compile and run Java code. You can download the appropriate JDK version for your operating system from the official Oracle website or a trusted distribution like OpenJDK. Follow the installation instructions specific to your platform to ensure a smooth setup.
Choosing an IDE (Eclipse, IntelliJ)
An Integrated Development Environment (IDE) provides a comprehensive environment for writing, debugging, and running your Java code. Popular choices for Java development include Eclipse and IntelliJ IDEA. Both offer features like code completion, debugging tools, and project management capabilities that streamline the development process. Choose the IDE that best suits your preferences and workflow. Many IDEs offer free community editions, making them accessible to everyone. If you're looking for a comprehensive, AI-powered testing solution, consider exploring MuukTest's services.
Setting Up Selenium WebDriver
Selenium WebDriver is a popular library for automating web browser interactions. It allows you to write Java code that controls web browsers, simulates user actions, and verifies web application behavior. To use Selenium WebDriver in your Java project, you need to download the Selenium Java client driver from the official Selenium website and add it to your project's dependencies. This will enable you to write Java code that interacts with web browsers for automated testing. For a simplified approach to test automation, see how MuukTest helps its customers achieve comprehensive test coverage.
Java Automation Testing: A Practical Guide
Java automation testing combines the power of the Java programming language with automated testing principles. It automatically checks software quality and functionality, using Java code to control test execution and validate results. This offers significant advantages over manual testing, especially for complex projects or frequent updates. It saves teams time, reduces human error, and ensures consistent testing across different environments.
What is Automation Testing?
Automation testing uses scripts to run tests automatically, making it far more efficient than manual testing, especially for repetitive tasks like regression testing. Instead of manually clicking through your application, you write a program to do it for you. This is invaluable when adding new features to web applications, ensuring existing functionality remains intact. Automated tests act as a safety net, catching issues early in development. This is crucial for ensuring the quality of web applications, especially with frequent updates. For more information, check out MuukTest's guide on Automation Testing.
Why Java for Test Automation?
Java is a popular choice for test automation for several key reasons. It's versatile and platform-independent, meaning tests can run on various operating systems without modification. The large, active Java community offers ample support and resources. Java's features—object-oriented nature, robust security, and automatic memory management—make it ideal for the task. Selenium, combined with Java, provides a powerful framework for automated testing, especially for web applications. Knowing Java is a valuable skill for QA engineers, increasing job prospects and adaptability across projects.
Java's Popularity in Test Automation
Java's widespread adoption in test automation isn't accidental. It boils down to a few key advantages. First, Java is platform-independent. Write your test once, and it can run on Windows, macOS, Linux—pretty much anywhere. This "write once, run anywhere" capability simplifies testing and reduces the need for platform-specific code. Second, Java boasts a massive and active community. This means readily available support, tons of online resources, and a wealth of pre-built libraries and frameworks to speed up development. If you hit a snag, chances are someone else has already solved it and shared the solution. Finally, Java's object-oriented nature, strong security, and automatic memory management make it perfect for building robust test automation frameworks. These features contribute to cleaner code, easier maintenance, and fewer unexpected errors during test execution.
The Power of Open-Source Libraries
One of Java's biggest strengths in test automation is its rich ecosystem of open-source libraries. Selenium, a leading open-source framework, is a prime example. Selenium automates interactions with web browsers, making it perfect for testing web applications. Imagine automatically testing user logins, form submissions, or navigating through different pages—Selenium handles it all with ease. And because it's open-source, it's free to use and constantly evolving thanks to community contributions. Another powerful library is JUnit, a widely used framework for writing and running unit tests. JUnit provides a simple yet effective way to structure your tests, making them easier to understand, maintain, and integrate into your development workflow. These open-source libraries, combined with Java's capabilities, provide a powerful toolkit for building comprehensive and efficient test automation solutions. They reduce development time and empower you to create more robust and reliable tests, ultimately leading to higher quality software. For businesses looking to streamline their testing, exploring services like those offered by MuukTest can provide tailored and scalable solutions.
Essential Java for Testers
Getting started with Java test automation requires a grasp of some core concepts. These building blocks will set you up for success when writing effective and efficient test scripts.
OOP in Test Automation
Java is an object-oriented programming (OOP) language. Understanding OOP principles is crucial for writing well-structured and reusable test code. Key concepts include:
- Classes and Objects: Think of a class as a blueprint for creating objects. For example, a "Car" class could define properties like "model" and "color." Each individual car (like a red Toyota or a blue Honda) would be an object, an instance of the "Car" class. In testing, you might create a class to represent a web page element, with properties like "name" and "location." Each instance of this class would represent a specific element on the page.
- Inheritance: This allows you to create new classes (child classes) based on existing ones (parent classes), inheriting their properties and methods. This promotes code reusability. You might have a parent class for a generic web element and then create child classes for specific element types like buttons or text fields, inheriting common functionalities.
- Polymorphism: This lets you use a single interface to represent objects of different classes. For example, a "click" method could work on both a button and a link, even though they are different types of elements. This simplifies your test code.
- Encapsulation: This bundles data (properties) and the methods that operate on that data within a class, protecting it from outside interference. This improves code maintainability and reduces errors.
These OOP principles help you create modular, reusable, and maintainable test code, which is especially important as your test suite grows.
Exceptions, Data Structures, and You
Beyond OOP, understanding how Java handles exceptions and uses data structures is essential:
- Exception Handling: Exceptions are unexpected events that can disrupt the flow of your program. Java's
try-catch
blocks allow you to gracefully handle these exceptions, preventing your tests from crashing. For instance, if your test tries to interact with an element that isn't loaded yet, you can catch the exception and retry the action. Robust exception handling is key for stable tests. - Data Structures: These are ways of organizing and storing data efficiently. Common data structures include arrays, lists, and maps. Understanding how to use these structures is important for managing test data, such as storing test inputs or expected results. For example, you might use a list to store a set of usernames to test with.
Mastering exception handling and data structures will make your tests more robust and easier to manage.
Must-Know Java Testing Libraries
Java's rich ecosystem of libraries simplifies test automation. Here are a few key ones:
- JUnit and TestNG: These frameworks provide structure for writing and running your tests. They offer features like assertions (checking if expected conditions are met), test runners (executing your tests), and reporting. Well-structured tests are easier to maintain and debug.
- Selenium: This is the go-to library for automating web browser interactions. You can use Selenium to simulate user actions like clicking buttons, filling out forms, and navigating between pages.
- REST Assured: This library simplifies API testing by providing a fluent API for making HTTP requests and validating responses. This makes API testing more efficient and readable.
Familiarizing yourself with these libraries will significantly streamline your Java test automation efforts. They provide pre-built functionalities, saving you time and effort. For a jumpstart on comprehensive testing, explore MuukTest's QuickStart guide.
Top Java Testing Tools & Frameworks
Java's robust ecosystem offers a wealth of tools and frameworks explicitly designed for testing. These tools help streamline your testing process, improve code quality, and ensure comprehensive test coverage. Let's explore some popular choices:
Overview of Popular Java Testing Frameworks
Java's popularity in test automation is largely due to its robust testing frameworks. These frameworks provide the structure and tools necessary to write effective, maintainable, and scalable tests. Here’s a closer look at some of the most popular ones:
- JUnit: This widely-used framework provides a foundation for writing unit tests. It offers a simple and clear API for defining test cases, making assertions, and organizing tests into suites. JUnit integrates seamlessly with most Java IDEs and build tools, making it easy to incorporate into your development workflow. Its reporting capabilities provide valuable insights into test results, helping you quickly identify and address issues.
- TestNG: Building upon JUnit's strengths, TestNG offers additional features like annotations, parallel test execution, and advanced reporting. Annotations provide a more flexible and expressive way to define test dependencies and configurations. Parallel execution significantly reduces test execution time, especially for large test suites. TestNG's detailed reporting helps pinpoint failures and track testing progress effectively.
- Selenium: A powerful framework for automating web browsers, Selenium allows you to simulate user interactions with web applications. You can write tests to verify that your web application functions correctly across different browsers and platforms. Selenium's WebDriver API provides a consistent interface for interacting with various web browsers, simplifying cross-browser testing. For practical examples and expert insights, explore MuukTest's test automation services.
- REST Assured: This framework simplifies API testing by providing a fluent API for making HTTP requests and validating responses. REST Assured makes it easy to write clean and readable tests for RESTful APIs, ensuring that your APIs function as expected. Its intuitive syntax and built-in matchers streamline the process of verifying API responses, making API testing more efficient.
These frameworks, combined with Java's strengths, create a powerful toolkit for test automation. By leveraging these tools, you can build robust and reliable tests, ensuring the quality and stability of your Java applications. To learn more about how MuukTest can help you achieve comprehensive test coverage, explore customer success stories and see our pricing.
Web Testing with Selenium & Java
Selenium is the go-to framework for automating web browsers. It supports multiple programming languages, including Java, making it a versatile choice for developers. With Selenium, you can create scripts that interact with web elements, simulate user actions like clicks and form submissions, and verify application behavior across different browsers. This makes Selenium essential for ensuring your web application functions correctly and delivers a seamless user experience.
Selenium WebDriver Basics
Selenium WebDriver is the heart of Selenium, letting you control web browsers through code. It uses a platform- and language-neutral wire protocol, enabling external programs to direct browser behavior. This means you can write tests in Java and run them on any browser Selenium supports, such as Chrome, Firefox, or Safari. This cross-browser compatibility is key for ensuring your web application works smoothly for everyone. For a deeper understanding of how WebDriver functions, check out the official Selenium documentation.
Locating Web Elements
Interacting with web page elements like buttons and text fields requires locating them first. Selenium provides several methods for this, using locators such as ID, Name, XPath, or CSS selectors. The right locator is essential for stable tests. IDs and Names are generally preferred for their simplicity and speed, but XPath or CSS selectors are sometimes necessary for more complex situations. This guide offers more on effective locator strategies, which, in turn, create faster, more resilient tests when web page structure changes.
Interacting with Web Pages
Selenium WebDriver enables diverse interactions with located elements. You can click buttons, input text, select dropdown options, and replicate other user actions. Retrieving text from elements or verifying properties (like a checkbox's status) is also possible. These interactions are fundamental to automated tests, verifying your web application's expected behavior. For example, a test could simulate filling a login form, clicking submit, and confirming successful login.
TestNG and JUnit: Test Structuring
TestNG and JUnit are popular testing frameworks that provide structure and organization to your Java test code. They offer features like annotations for defining test methods, test suites for grouping related tests, and assertions for validating expected outcomes. JUnit is known for its simplicity and ease of use, while TestNG offers more advanced features like parallel test execution and dependency management. Both frameworks help you write clean, manageable tests and generate detailed reports for analyzing test results.
API Testing with REST Assured
Modern applications often rely on APIs (Application Programming Interfaces) to communicate and exchange data. REST Assured is a powerful Java library specifically designed for testing RESTful APIs. It simplifies sending HTTP requests, validating responses, and performing assertions on API endpoints. With its intuitive syntax and powerful features, REST Assured makes it easier to ensure your APIs function correctly and handle data efficiently.
Setting Up Your Java Test Environment
Getting started with Java automation testing means setting up your environment correctly. First, install the Java Development Kit (JDK), which provides the core tools and libraries for Java development. Next, choose an Integrated Development Environment (IDE). Popular choices include Eclipse and IntelliJ IDEA, both offering robust features for coding, debugging, and testing. Finally, you'll need Selenium WebDriver, a powerful library for automating web browsers. Use a build tool like Maven or Gradle to manage Selenium and other project dependencies. This initial setup is essential for any Java automation project.
Writing Your First Java Test
With your environment ready, write your first Java test script. A simple starting point is a script that opens a webpage using Selenium. This exercise introduces the basic functionality of Selenium and how to interact with web elements. Plenty of online resources and tutorials, like this one from BrowserStack, offer step-by-step instructions for creating and running your first test. Experiment with different actions, like clicking buttons or filling out forms, to understand how Selenium automates browser interactions.
CI/CD Pipeline Integration
Integrating your Java automation tests into your Continuous Integration/Continuous Deployment (CI/CD) pipelines is key for modern software development. Services like MuukTest offer seamless CI/CD integration, allowing quick and efficient test execution with a fully managed infrastructure. This not only speeds up testing but also ensures consistent execution with every code change, leading to faster releases and higher software quality. Automating tests within your CI/CD workflow helps catch regressions early and maintain a robust development cycle.
Integrating with Jenkins
Jenkins is a popular open-source automation server, a cornerstone for continuous integration and continuous delivery (CI/CD). It automates building, testing, and deploying software, making it a natural fit for running your Java automation tests. Integrating your tests with Jenkins ensures they run automatically with every code change, providing quick feedback and catching potential problems early. You can configure Jenkins to pull your test code from a Git repository like GitHub, compile it, and execute your tests using tools like Maven or Gradle. Check out this Jenkins test automation tutorial to get started. This automation streamlines your testing and ensures consistency.
Integrating with GitLab CI/CD
If your team uses GitLab, its built-in CI/CD features offer a convenient way to incorporate your Java automation tests. With GitLab CI/CD, you define pipelines that automatically build, test, and deploy your code. Integrating your Java tests into these pipelines ensures every code change is thoroughly vetted before deployment. This is essential for maintaining high software quality and frequent releases. Similar to Jenkins, GitLab CI/CD works with Maven or Gradle, creating a smooth, automated testing process within your development workflow. For a deeper dive into automated testing and CI/CD, explore MuukTest's Test Automation Services.
Java Test Automation Best Practices
Efficient and maintainable test automation requires a strategic approach. Let's explore some best practices to elevate your Java test automation game.
Code Organization with Design Patterns
Writing clean, organized code is crucial for any project, and test automation is no exception. As projects grow, messy code becomes a nightmare to maintain and debug. A good approach is to leverage design patterns like the Page Object Model (POM). POM encapsulates the elements and actions of a web page into a dedicated class. This improves code readability and makes your tests more reusable and easier to update. Think of it like creating blueprints for your application's pages. If the UI changes, you only need to modify the corresponding page object, rather than hunting down and fixing every test that interacts with that page.
Writing Maintainable & Scalable Tests
As your application evolves, so should your test suite. Writing maintainable and scalable tests ensures your automation efforts can adapt to changes without requiring massive rewrites. Consider a platform like MuukTest, which offers a fully managed automation testing solution. This frees you from the complexities of managing infrastructure and allows you to focus on building robust tests that provide comprehensive coverage. With MuukTest handling the backend, your team can concentrate on development and deliver high-quality software faster.
Effective Test Data Management
Test data is the lifeblood of your automated tests. How you manage it directly impacts the reliability and consistency of your results. A well-defined strategy for test data management is essential. This might involve creating dedicated test datasets, using data factories to generate synthetic data, or implementing data-driven testing approaches. The key is to ensure your tests have access to the right data at the right time, without compromising the integrity of your testing environment. As pointed out in this article on automation testing challenges, managing test data effectively is a strategic imperative for successful automation. A solid strategy improves the accuracy of your tests and simplifies debugging and analysis when issues arise.
Common Java Testing Challenges (Solved!)
Java testing, while powerful, presents some common hurdles. Let's explore a few and how you can clear them.
Managing Dependencies
Assembling the right tools for a project is tricky, but an even bigger challenge is building the most efficient automation testing framework. Teams also struggle with setting up the infrastructure, especially cloud-based solutions. A well-structured project, using a build tool like Maven or Gradle, can simplify dependency management. These tools help manage external libraries and ensure compatibility, streamlining your project setup and maintenance. Consider exploring dependency injection frameworks to further decouple your code and improve testability.
Handling Dynamic Web Elements
Dynamic web elements, those that change properties during runtime, are another testing challenge. These changes require testers to use strategies like explicit waits or dynamic locators to interact with these elements reliably. Explicit waits pause your test until a specific condition is met, preventing it from failing prematurely. Dynamic locators, on the other hand, adjust to changes in the element's properties, ensuring your tests remain stable even when the UI evolves. Consider using tools like Selenium's WebDriverWait class combined with CSS selectors or XPath expressions to target dynamic elements effectively. For more robust solutions, explore advanced techniques for handling dynamic web elements.
Debugging Java Test Scripts
Debugging Java test scripts becomes complex when working with multiple frameworks and libraries. Logging and debugging tools within your IDE are essential for efficient troubleshooting. Clear, concise logs pinpoint issues quickly, while debuggers let you step through your code, inspect variables, and understand the flow of execution. Leverage your IDE's debugging capabilities and consider logging frameworks like Log4j or SLF4j for comprehensive logging. These practices will significantly reduce the time spent identifying and resolving issues in your test scripts. You can find helpful tips and tricks for debugging Java code online.
Advanced Java for Robust Tests
As you gain more experience with Java automation testing, incorporating advanced techniques can significantly improve the efficiency and robustness of your tests. Let's explore some key strategies:
Multi-Threading for Faster Tests
Java's multithreading capabilities allow you to run multiple tests concurrently, drastically reducing the overall execution time. Think of it like checking out groceries at multiple checkout counters instead of waiting in one long line. By leveraging Java's built-in threading libraries, you can create test suites that execute in parallel, making your automation process much more efficient. This is especially valuable for large test suites or when dealing with time-consuming tests, such as those involving extensive UI interactions or complex data processing.
Comprehensive Test Reporting
Clear and detailed reporting is essential for understanding test results and quickly identifying any issues. Imagine trying to diagnose a car problem without any error messages—frustrating, right? Similarly, comprehensive reports in your testing framework provide the insights you need to fix bugs efficiently. Java offers various logging libraries, such as Log4j and SLF4J, which you can integrate into your test scripts to generate detailed logs and reports. These reports can include information about passed and failed tests, execution time, error messages, and other relevant metrics. For more information on how MuukTest implements comprehensive reporting, see our test automation services.
Parallel Test Execution
Running tests in parallel is a powerful technique for optimizing your test execution time. Instead of running tests sequentially, one after the other, parallel execution allows you to run multiple tests simultaneously, significantly speeding up the entire process. Frameworks like TestNG and JUnit provide built-in support for parallel execution. These frameworks leverage Java's concurrency features, allowing you to configure your test suites to run multiple tests at the same time. For a broader look at the challenges and solutions in automated testing, including parallel execution, take a look at this guide from BrowserStack. By implementing parallel execution, you can maximize resource utilization and complete your test cycles much faster.
Optimizing Java Tests for Performance
Getting your Java tests to run smoothly and efficiently is key for any successful project. This section covers practical tips for organizing your test suite and optimizing performance. A well-structured approach saves you time and resources down the line.
Test Suite Organization
A disorganized test suite can quickly become a nightmare to manage. Start by leveraging popular open-source automation tools like Selenium and Cypress, which offer robust frameworks for structuring your tests. Think of your test suite like a well-organized library—you want to find what you need quickly. Group similar tests, use clear naming conventions, and implement a logical hierarchy. This makes it easier to understand each test, locate specific tests for maintenance, and add new tests as your project grows. Collaboration between testers and developers also contributes to a more streamlined and efficient test suite. Sharing knowledge and best practices across teams keeps everyone aligned and contributes to a more cohesive testing strategy.
Performance Profiling and Optimization
Once you have a well-organized test suite, optimize its performance. Start by profiling your tests to identify bottlenecks. Several profiling tools can pinpoint performance issues, allowing you to focus your optimization efforts effectively. Consider your testing infrastructure, whether cloud-based or on-premise. Experimenting with different solutions helps you find the optimal setup for your needs. A poorly chosen infrastructure can lead to early test automation failures, so it's worth investing the time to get it right. Finally, keep up with emerging testing trends and best practices to ensure your Java tests remain efficient and effective. Continuous learning and adaptation are essential in software testing.
Real-World Applications and Examples
Let’s see how Java automation testing works in real-world scenarios. These examples show how versatile and powerful Java is for tackling different testing challenges.
E-commerce Website Testing
Imagine building an e-commerce website. Testing is crucial. Customers need to browse products, add items to their carts, and complete purchases smoothly. Java with Selenium is perfect for this. You can write Java code to simulate user actions, like searching for products, adding them to the cart, and going through checkout. Selenium handles the browser interactions, while your Java code controls the test flow and verifies the expected outcomes. You can check that the right product is added to the cart, the total price is calculated correctly, and the order confirmation page displays the right information. This automation ensures a smooth and reliable shopping experience. For a deeper dive into e-commerce testing with Selenium, check out this MuukTest article.
Mobile App Testing with Appium
Now, think about mobile apps. With so many smartphones and tablets, your app must work flawlessly across different devices and operating systems. Appium, an open-source tool, simplifies mobile app testing. Appium lets you write tests using the same Selenium WebDriver API. If you already know Selenium and Java for web testing, you can easily transfer those skills to mobile testing. Write Java code to interact with your app's UI elements, simulate user gestures like taps and swipes, and verify the app's behavior. This cross-platform compatibility makes Appium powerful for ensuring your mobile app delivers a consistent experience, regardless of the user's device. BrowserStack offers a helpful guide on using Selenium with Java, a great starting point for exploring Appium.
The Future of Java in Testing
Java remains a powerhouse in test automation. Its maturity, extensive libraries, and cross-platform compatibility make it a solid foundation for building reliable tests. But what's next? Let's explore emerging trends and Java's evolving role.
Emerging Java Testing Trends
Java's adaptability is key to its continued relevance in the testing landscape. A strong Java foundation is crucial for testers. This core programming knowledge empowers testers to handle complex scenarios and create reusable, maintainable test code using Java's object-oriented nature. Valuable features like automatic memory management and multithreading are for robust test automation. We're seeing growing demand for testers with expertise beyond basic Java syntax, delving into areas like design patterns and concurrent programming. This shift enables more efficient and scalable testing, especially critical for applications with frequent updates, a point. This trend reinforces the importance of continuous learning for Java testers.
Java's Role in Continuous Testing
Java is integral to the expanding world of continuous testing. Its seamless integration with popular CI/CD tools makes it a natural fit for automated testing pipelines. Services like MuukTest leverage Java's strengths to deliver comprehensive and efficient test automation. MuukTest's AI-powered platform, combined with expert QA services, enables rapid test development and execution, ensuring faster feedback and quicker releases. Automating regression testing, as discussed in this Medium article, is essential for maintaining software quality throughout development. Java's object-oriented design, which promotes reusable code, further enhances team collaboration and efficiency in continuous testing environments. As development practices evolve, Java's robust ecosystem and adaptability ensure its continued importance in the future of test automation.
Frequently Asked Questions
Why is Java so popular for test automation?
Java's platform independence lets you run tests on any operating system. Its large, active community provides tons of support and readily available libraries. Plus, Java's robust features, like built-in security and automatic memory management, make it perfect for handling the complexities of test automation.
What are the must-know Java concepts for someone new to test automation?
Definitely focus on understanding object-oriented programming (OOP) principles like classes, objects, inheritance, and polymorphism. These concepts are key for writing clean, reusable, and efficient test code. Also, get familiar with exception handling for writing robust tests and data structures for managing your test data effectively.
Which Java libraries are essential for test automation?
JUnit or TestNG for structuring your tests, Selenium for web browser automation, and REST Assured for API testing are your core tools. These libraries provide pre-built functions and structures that will save you a lot of time and effort.
What's the best way to handle dynamic web elements in my tests?
Dynamic elements can be tricky. Use explicit waits to pause your test until an element appears or a condition is met. Alternatively, use dynamic locators that adjust to changes in the element's properties. Combining these techniques ensures your tests remain stable even when the UI changes.
How can I make my Java tests run faster?
Consider multithreading to run tests concurrently, like having multiple checkout lines at a grocery store. Also, profile your tests to identify and eliminate performance bottlenecks. A well-organized test suite and a solid testing infrastructure also contribute significantly to faster test execution.
Related Articles
Related Posts:
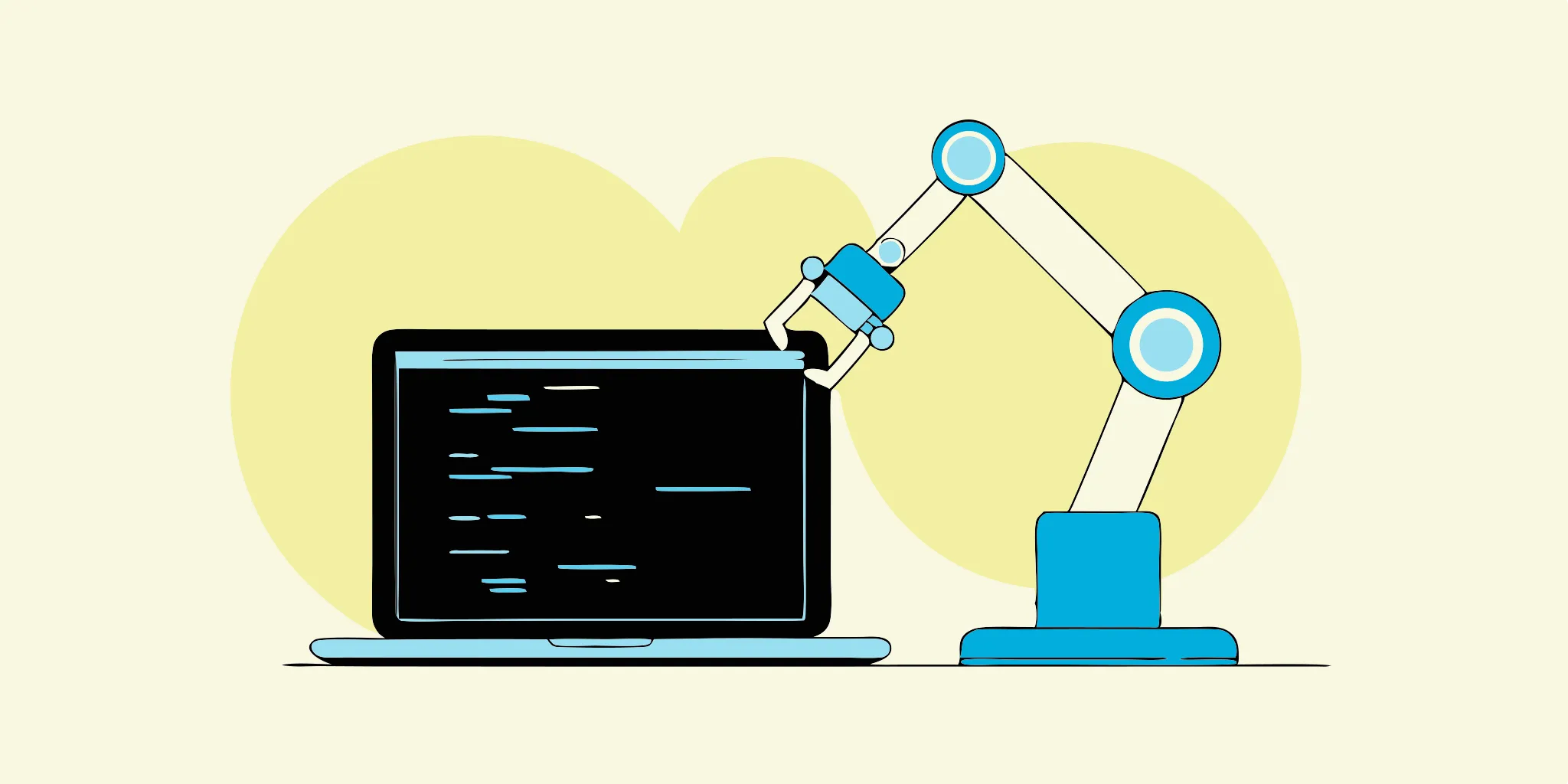
JavaScript Automation: The Definitive Guide
Master JavaScript automation testing with this practical guide. Learn key features, best practices, and techniques to enhance your testing process.
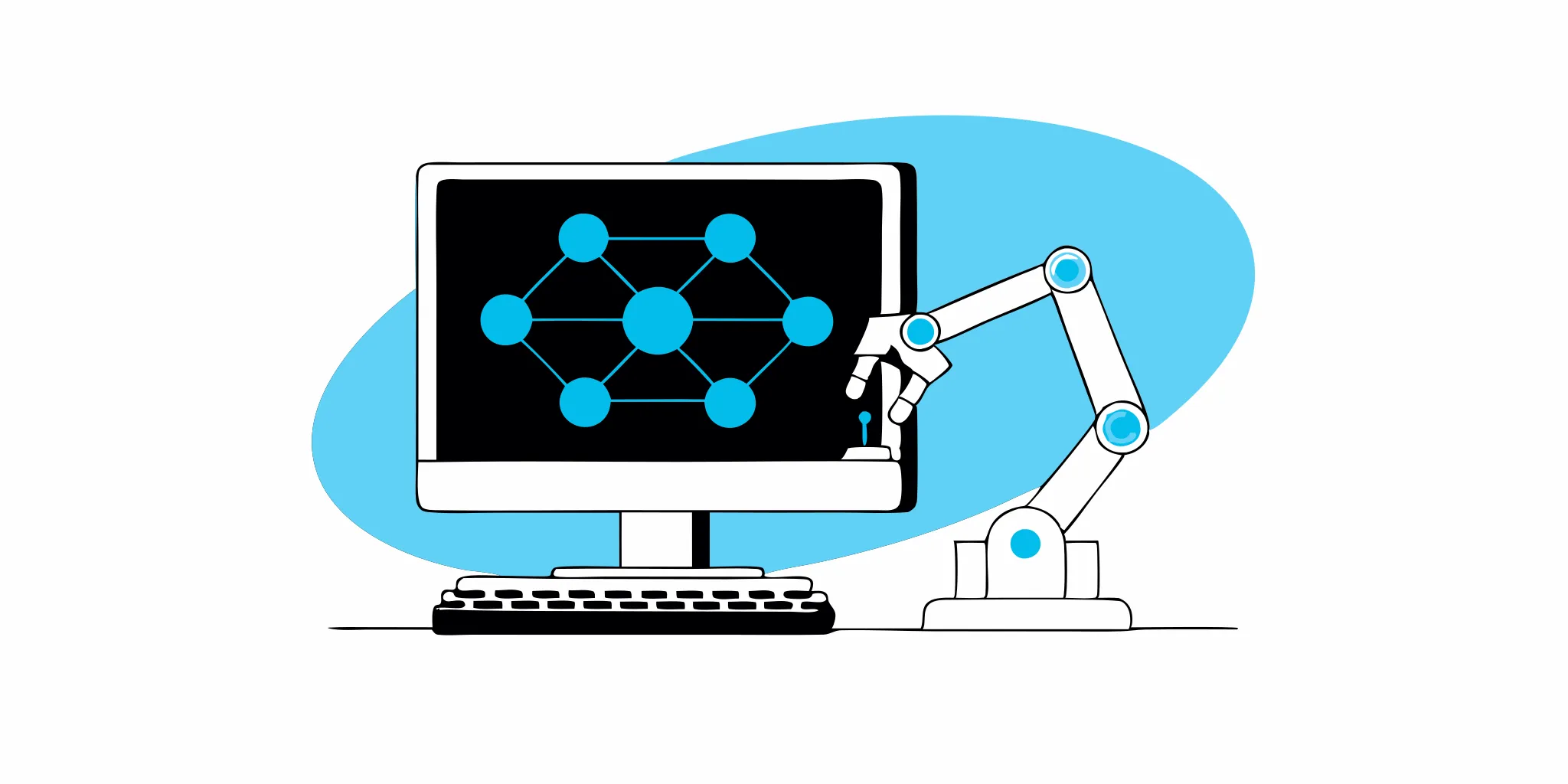
QA Automation Testing Services: Your Ultimate Guide
Tired of endless bug hunts and slow release cycles? Software testing can feel like a never-ending game of whack-a-mole. You squash one bug, and another pops up. But what if you could predict where...
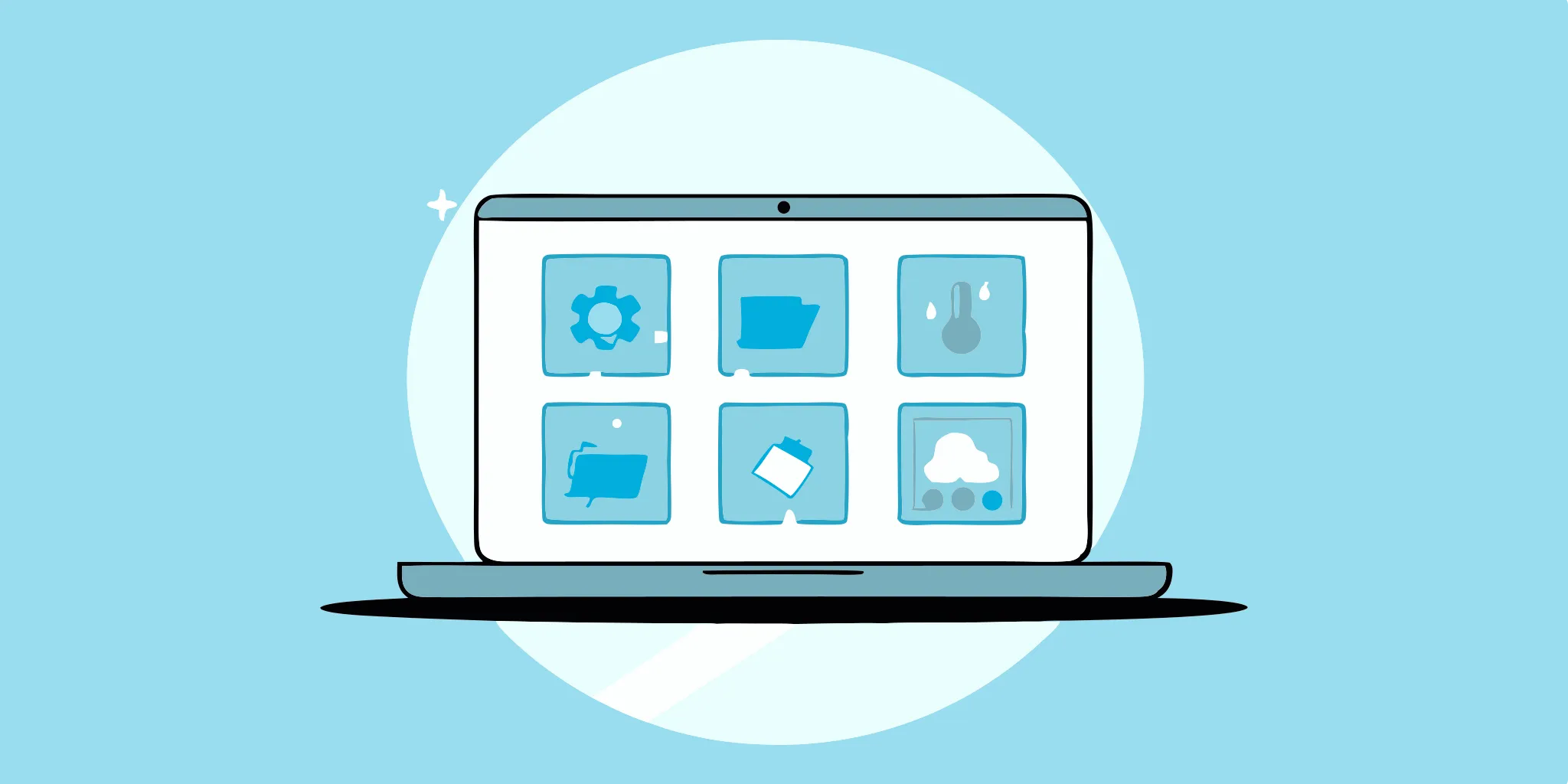
Automated Interface Testing: A Practical Guide
In the world of software development, time is of the essence. You need to deliver high-quality software quickly and efficiently. Manual interface testing can be a bottleneck, slowing down your...