Selenium Best Practices for Efficient & Reliable Tests
Author: The MuukTest Team
Last updated: October 1, 2024
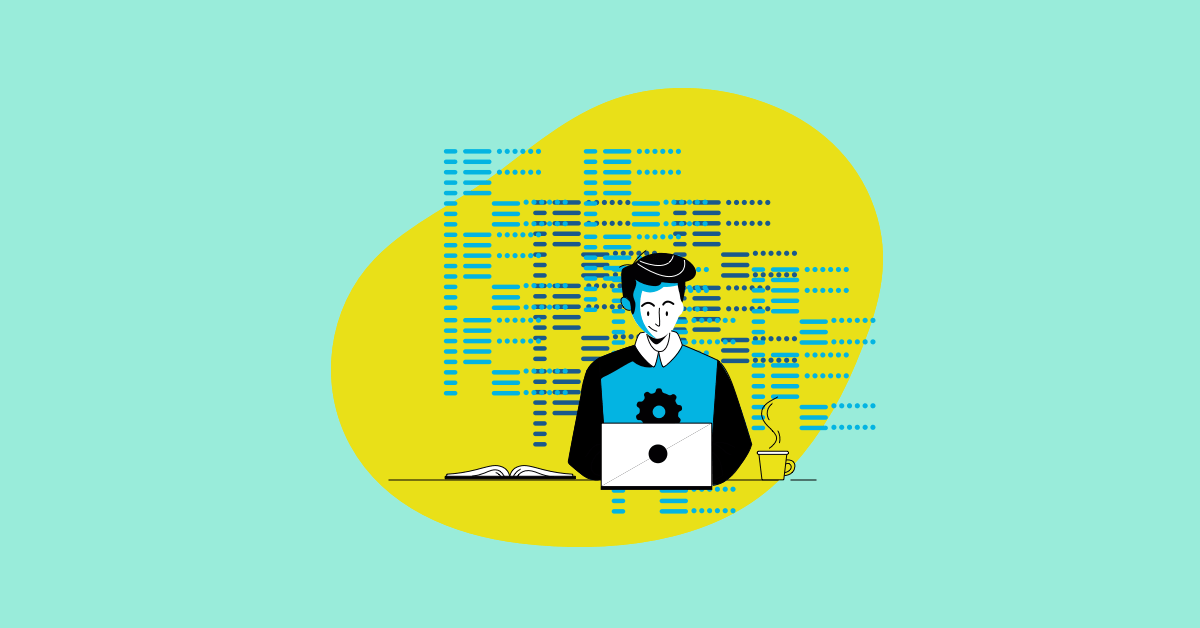
Table of Contents
Want flawless web apps? Selenium test automation makes it happen. This guide delivers practical Selenium best practices to thoroughly test across browsers and platforms, ensuring a smooth user experience. Whether you're just starting with Selenium functional testing or an expert exploring Selenium test automation services, we've got you covered.
Selenium has built its reputation as a trusted tool for automating web application testing. It enables teams to automate repetitive tasks, catch bugs early, and improve software quality. By automating browser interactions, Selenium saves time and reduces the risk of human error.
Key Takeaways
- Selenium simplifies web app testing: Its cross-browser support and flexible integration options make it easy to automate repetitive tasks, improving software quality and freeing up your team.
- Well-structured Selenium tests are key for success: Using smart locator strategies and the Page Object Model keeps tests maintainable, while techniques like mocking external services ensure reliability.
- Expand your Selenium expertise: Advanced methods like parallel testing and integration with other tools optimize efficiency. Remember to adapt best practices to your project's unique needs.
What is Selenium?
Selenium is an open-source framework that automates web application testing. It interacts with browsers through browser-specific drivers that execute the instructions provided in test scripts.
Functional testing is a core use case for Selenium. It allows teams to verify that all features of a web or mobile application function correctly by simulating user interactions in various environments. These scripts, written in programming languages like Java, Python, or C#, control the browser to mimic real user behaviors. Automating these testing scenarios reduces manual effort and improves the speed and consistency of test execution.
Selenium’s primary components include:
- Selenium WebDriver: WebDriver allows engineers to control web browsers programmatically. It interacts directly with a browser’s engine, making it capable of performing tasks like clicking buttons, filling out forms, or navigating through a website. WebDriver also supports multiple browsers like Chrome, Firefox, and Edge, making cross-browser testing simple and efficient.
- Selenium IDE: As a more user-friendly tool, Selenium IDE is a browser extension that enables testers to record and playback test scripts. This component is useful for quickly creating tests without needing to write code. It is a great starting point for teams looking to explore test automation without diving deep into programming.
- Selenium Grid: When teams need to scale their testing efforts, Selenium Grid allows for parallel execution of tests across multiple machines and environments. This means teams can run tests on different browsers and operating systems simultaneously, speeding up test cycles and improving overall test coverage.
What is Selenium Used For?
Selenium is primarily used for automating web application testing, allowing teams to verify that all features of a web or mobile application function correctly. By simulating user interactions in various environments, Selenium helps catch bugs early and improve software quality. As an open-source framework, it interacts with browsers through browser-specific drivers that execute instructions provided in test scripts, making it a versatile tool for developers and testers.
One core use case for Selenium is functional testing. This involves writing scripts in programming languages like Java, Python, or C# to control the browser and mimic real user behaviors. Automating these tests not only reduces manual effort but also enhances the speed and consistency of test execution. For a deeper understanding, check out our guide to functional testing.
Selenium WebDriver, a primary Selenium component, allows engineers to control web browsers programmatically. WebDriver interacts directly with a browser’s engine, enabling actions like clicking buttons, filling out forms, and website navigation. It supports multiple browsers, including Chrome, Firefox, and Edge, simplifying cross-browser testing. Need to streamline your testing? MuukTest offers expert QA and CI/CD workflow integration to optimize your process.
Selenium Grid enables parallel test execution across multiple machines and environments. This allows simultaneous testing on different browsers and operating systems, significantly speeding up test cycles and improving overall test coverage. Learn more about maximizing test coverage in our blog post on test coverage techniques.
What Can You Do with Selenium?
Selenium is primarily used for automating web browsers, allowing you to control actions like clicking links, filling out forms, and navigating between pages. This makes it incredibly useful for functional testing, where you verify that your web application behaves as expected. Think of it as a robot user meticulously checking every corner of your website, ensuring everything works smoothly. This automation saves time and effort, catching bugs early in the development cycle.
Beyond functional testing, Selenium handles tasks like performance testing, assessing how your website handles traffic spikes, and compatibility testing, ensuring your website renders correctly across different browsers and devices. This broad applicability makes Selenium a versatile tool for any web developer.
Why is Selenium So Popular?
Selenium's popularity stems from several key factors. First, it's open-source and free to use and modify. This accessibility has fostered a large and active community, providing ample resources and support. The open-source nature also allows for continuous improvement and adaptation to the ever-evolving web landscape.
Second, Selenium supports various programming languages like Java, Python, and C#, giving developers flexibility. It integrates seamlessly into existing workflows and toolchains. Its cross-browser compatibility lets you test on Chrome, Firefox, Safari, and Edge, ensuring a consistent user experience. This broad compatibility is a major advantage.
Finally, Selenium integrates with other testing frameworks and tools like TestNG and JUnit, enhancing its power and flexibility. This allows for more comprehensive testing strategies and streamlines testing. For teams aiming for comprehensive test coverage quickly and efficiently, consider services like those offered by MuukTest.
Why Choose Selenium for Test Automation?
Selenium test automation offers several advantages for teams seeking flexibility and efficiency:
- Cross-browser compatibility: Selenium enables teams to create test scripts that run across all major browsers, eliminating repetitive manual testing across platforms.
- Open-source and free: Selenium is free, with a vast open-source community that continuously enhances its capabilities.
- Supports multiple programming languages: With support for languages like Java, Python, C#, and JavaScript, Selenium adapts to existing workflows, helping teams avoid steep learning curves.
- Strong community and ecosystem: Selenium’s popularity means teams can access extensive libraries, plugins, and support from a global community of testers and developers.
- Scalability and flexibility: Selenium Grid allows for parallel testing across browsers and environments, increasing test efficiency and supporting agile workflows as projects grow.
- Parallel execution for faster test execution: Selenium test script execution can be optimized by running tests in parallel, particularly through the use of Selenium Grid. By executing multiple tests simultaneously across different browsers and platforms, teams can significantly reduce the time required for test cycles and improve efficiency.
- Maintaining quality across environments: Maintaining a consistent user experience across different browsers and environments is crucial for modern applications. Implementing a well-structured test suite and grouping scripts logically (e.g., functional tests versus UI tests) helps catch potential issues affecting user experience early in the testing process.
Benefits of Using Selenium
Open-Source and Adaptable
One of Selenium’s biggest advantages is that it's open-source and free. This removes the cost barrier for individuals and small teams and fosters a large, active community that continually improves it. This means access to a wealth of resources like documentation, tutorials, and support forums, all thanks to the collaborative nature of the Selenium project. For a helpful guide on functional testing with Selenium, check out the MuukTest blog.
The Power of Community Support
This active community creates a robust support ecosystem. You can find solutions to almost any Selenium challenge through online forums, communities like Stack Overflow, and dedicated Selenium groups. This collaborative environment ensures you're never alone when facing a roadblock, and the shared knowledge accelerates problem-solving and learning. The community-driven development and updates mean you're always working with a tool that evolves to meet the latest testing demands.
Language and Framework Flexibility
Selenium supports many popular programming languages, including Java, Python, C#, JavaScript, and Ruby. This flexibility lets teams integrate Selenium into their existing workflows and preferred coding environments. It eliminates learning a new language just for testing, reducing the learning curve and making it easier for developers and testers to adopt Selenium. This adaptability is a significant advantage, especially for teams already invested in a particular tech stack.
Cross-Browser and Platform Compatibility: Test Everywhere
Testing across different browsers and operating systems is crucial for ensuring your web application works consistently for all users. Selenium excels here, supporting all major browsers like Chrome, Firefox, Safari, and Edge, and various operating systems. This cross-browser and platform compatibility streamlines testing. Write tests once and run them across multiple environments, saving significant time and effort compared to manual testing on each platform.
Seamless Integrations with Your Favorite Tools
Selenium integrates well with other tools commonly used in software development and testing. Selenium Grid enables parallel testing, dramatically reducing test execution time. It also integrates with testing frameworks like TestNG and JUnit, reporting tools like Allure and ExtentReports, and CI/CD tools like Jenkins and GitLab CI, allowing seamless test automation. This broad integration capability makes Selenium a versatile tool that fits into diverse development ecosystems. Learn more about how MuukTest leverages these integrations on our Customers page.
Selenium and AI-Powered Test Automation (MuukTest)
Selenium is a powerful tool, but combining it with AI-powered test automation elevates its capabilities. Selenium provides the engine for automated interactions, while AI adds the intelligence to make those interactions smarter and more efficient. This addresses challenges like test maintenance and slow execution times common in traditional Selenium testing.
AI enhances Selenium in several key ways. AI-powered tools can intelligently generate test scripts, reducing manual test creation time and effort. These tools analyze application code and user behavior to automatically create test cases covering a wider range of scenarios. AI algorithms also improve test stability by using robust locators less susceptible to UI changes, meaning fewer broken tests and less maintenance.
Finally, AI optimizes test execution by prioritizing critical tests and running them in parallel, significantly reducing overall testing time. This is a game-changer for teams aiming for comprehensive test coverage quickly. MuukTest offers AI-powered test automation services that leverage Selenium’s strengths. Our platform integrates seamlessly with Selenium, providing intelligent test generation, enhanced test stability, and optimized test execution. We help teams achieve complete test coverage within 90 days, significantly improving test efficiency and coverage.
Learn more about how MuukTest can improve your Selenium testing through our customer success stories or explore our pricing plans. Ready to start? Visit our QuickStart guide for smarter, faster, and more efficient Selenium testing.
Selenium's Limitations: What to Watch Out For
Is Selenium Hard to Learn?
While Selenium offers many benefits, it has a learning curve, especially for those new to programming or test automation. Building effective Selenium tests requires understanding programming concepts and the Selenium framework. Resources like GeeksforGeeks offer insights into the time commitment for learning Selenium, which can range from a few weeks for basic understanding to several months for mastery. Consider exploring MuukTest's QuickStart program for accelerated onboarding.
Do You Need Coding Skills for Selenium?
Selenium is code-based, so you need programming skills to write and maintain tests. While Selenium IDE offers record-and-playback for simpler tests, more complex scenarios require coding expertise. This can be a barrier for teams without dedicated test automation engineers or those with limited programming experience. However, investing in learning Selenium coding pays off with more robust and flexible test automation.
Selenium Test Maintenance: Tips and Tricks
As web applications evolve, so must your Selenium tests. Maintaining many Selenium tests can be challenging, requiring regular updates and refactoring to keep them aligned with application changes. BrowserStack’s best practices emphasize regular test maintenance for continued effectiveness. This ongoing maintenance can require significant time and effort, especially for complex applications with frequent updates. For a more manageable approach, consider MuukTest's managed services.
Beyond the Web: Selenium's Scope
Selenium is primarily designed for testing web applications. It interacts with web browsers but doesn't directly support testing desktop or mobile applications. For mobile app testing, explore tools like Appium, which leverages the Selenium WebDriver protocol but is designed for mobile automation. Understanding this limitation is crucial when choosing the right automation tool. LambdaTest provides further insights into Selenium's focus on web testing.
Your First Selenium Test: A Step-by-Step Guide
Setting up Selenium is straightforward, even for teams new to test automation:
Download and install Selenium WebDriver: First, download the WebDriver for the browser the team will test (e.g., ChromeDriver for Chrome). WebDrivers enable communication between the test scripts and the browser.
Choose a programming language: Selenium supports many languages, so teams should choose one they are familiar with — Java, Python, or C# are popular choices. Python’s simplicity and libraries make it great for beginners, while Java is ideal for larger projects.
Write the first test script: Start with a simple script to automate basic actions, such as opening a browser, navigating to a website, and verifying that certain elements load.
from selenium import webdriver
driver = webdriver.Chrome() # Launch the Chrome browser
driver.get("https://www.example.com") # Navigate to the target website
# Perform actions on the webpage
element = driver.find_element_by_name("q") # Find the search box
element.send_keys("Selenium test automation") # Input text
element.submit() # Submit the form
driver.quit() # Close the browser
Execute the Selenium test script: Run the script using an integrated development environment (IDE) or command line. Selenium will automate browser actions so teams can verify a web application functions correctly.
As testing needs evolve, teams can scale their efforts by integrating Selenium with other tools and executing parallel tests.
Setting Up Your Selenium Environment
Getting started with Selenium is easier than you might think. First, set up your testing environment with these key steps:
- Download and Install a WebDriver: Selenium uses WebDrivers to control browsers. Download the correct WebDriver for your browser. For example, if you're testing on Chrome, you'll need ChromeDriver. The official Selenium documentation lists WebDrivers for different browsers.
- Choose a Programming Language: Selenium supports various languages like Java, Python, C#, and JavaScript. Pick the one that best suits your team's existing skills and project needs. Python is often a good choice for beginners due to its readability and extensive libraries. For larger, more complex projects, Java might be a better fit. This functional testing guide offers more insights into choosing the right language.
- Install Necessary Libraries: After choosing your language, install the Selenium libraries. This usually involves a package manager like pip for Python or Maven for Java. The Selenium documentation provides clear instructions for each language.
A Simple Selenium Test Example
Now that your environment is ready, let's look at a simple Selenium test example using Python. This script opens Chrome, goes to a website, performs a search, and closes the browser:
from selenium import webdriver
driver = webdriver.Chrome() # Initialize ChromeDriver
driver.get("https://www.example.com") # Navigate to the target website
element = driver.find_element("name", "q") # Locate the search bar (using the 'name' attribute)
element.send_keys("Selenium test automation") # Enter search text
element.submit() # Submit the search
driver.quit() # Close the browser
This script demonstrates a basic Selenium test. It initializes ChromeDriver, navigates to a website, locates the search bar using its name attribute, enters text, submits the search, and closes the browser. This example provides a foundation for more complex tests. For more detailed examples and best practices, see this guide on functional testing. As your testing needs evolve, explore advanced techniques like parallel testing and integrating Selenium with your CI/CD pipeline. For a comprehensive and efficient solution, MuukTest’s AI-powered test automation services can help you achieve complete test coverage within 90 days.
Selenium Best Practices: Cleaner & More Efficient Tests
Test data management plays a necessary role in creating reliable tests. Keeping test data consistent and separate from the test scripts allows for more reusable and adaptable tests, reducing the likelihood of errors due to changing environments.
It’s also important to maintain a consistent test environment that replicates the production setup. This practice helps avoid discrepancies during testing. Tools like Jenkins or CircleCI can automate the creation of test environments, making the process more efficient.
Organizing test scripts logically by functionality or type, such as functional tests versus UI tests, improves both scalability and maintenance. This makes it easier to manage large test suites.
Effective error handling and debugging are vital for maintaining the integrity and reliability of automated tests. When test failures occur, capturing detailed logs provides crucial insights into the test’s execution flow and the specific point of failure. These logs should include information such as time stamps, error messages, and the specific actions being performed when the error occurred.
Lastly, integrating Selenium into continuous integration (CI) pipelines automates the testing process with each code commit. This method reduces risk and provides fast feedback to developers, which supports more efficient and reliable software releases.
Generating and Managing Application State
Think of your application state as a snapshot of its current condition—the data in its memory, the values of its variables, everything that determines its behavior at a specific moment. In testing, you want to start each test with a known, predictable state. This ensures consistent and reliable results. Imagine testing a shopping cart feature; you wouldn't want leftover items from a previous test messing with your current one.
Techniques like database seeding, API calls to set up initial data, or directly manipulating objects within your application can help establish the right starting point. For instance, before testing checkout, use an API call to create a test user and add items to their cart. This ensures your test focuses solely on the checkout functionality. This controlled environment makes tests more robust and easier to debug. The Selenium documentation offers more on encouraged behaviors.
Mocking External Services and Dependencies
Web applications often rely on external services—databases, APIs, third-party payment gateways. While crucial in a live environment, these integrations can create testing headaches. Slow responses, network issues, or changes in the external service can make your tests flaky. Mocking simulates these services, giving you control over their behavior. Instead of hitting a real database, your test interacts with a mock database that returns predefined data, making tests faster and more isolated.
Imagine testing a feature sending data to an external API. Instead of relying on the actual API, create a mock API that returns a canned response. This speeds up your test and allows testing various scenarios, including errors, without affecting the real API. This isolation is key for robust tests. Learn more about mocking in the Selenium documentation.
Avoiding Shared State Between Tests
Tests should be independent. One test shouldn't influence another. Shared state is a recipe for unpredictable failures. Imagine one test modifies a database record that another test relies on. If the tests run in a different order, the second test might fail, even if its code is fine. This interdependence makes pinpointing problems harder.
Treat each test as a self-contained unit. Set up the necessary state before each test and clean up afterward. This ensures tests don't interfere with each other. Techniques like database transactions (rollback after each test) or creating fresh test data maintain this isolation. This independence allows for parallel execution, speeding up your test suite, as highlighted in LambdaTest's best practices. Autonomous tests create a more stable testing environment.
Precise Element Targeting with Smart Locators
Locator Strategies: ID, Name, CSS, XPath
Writing reliable Selenium tests depends on choosing the right locator strategy. Locators are like addresses, telling Selenium how to find elements on a web page. Prioritize IDs, then names, followed by CSS selectors. If those aren’t an option, use XPaths, but try to avoid absolute XPaths. A solid locator strategy keeps your tests stable even with UI changes.
Why You Should Avoid Absolute XPaths
Absolute XPaths are brittle. Because they rely on the full path from the root of the HTML document, even small page structure tweaks can break your tests. Opt for relative XPaths or stick to IDs, names, or CSS selectors. This will make your tests more robust and easier to maintain.
Effective Locator Management for Long-Term Maintainability
Think of locators as the addresses Selenium uses to pinpoint elements on your web page. Choosing the right locator strategy is crucial for building robust, long-lasting tests. A well-defined strategy ensures your tests remain stable even when the UI undergoes changes, saving you time and frustration down the line.
Prioritize using IDs whenever possible. They're typically unique and the most reliable way to target elements. If IDs aren't available, names are a good second choice. Then consider CSS selectors, which offer a balance of flexibility and performance. Experts often recommend CSS selectors for their efficiency and readability.
While XPaths are useful when other options fail, avoid absolute XPaths. These paths are highly dependent on the page's structure, and even a small HTML change can break your tests. Relative XPaths, which target elements based on their relationship to others, offer more stability. However, sticking to IDs, names, or CSS selectors generally leads to more robust and maintainable tests, simplifying updates and reducing the risk of breakage from minor UI adjustments. For more on building maintainable tests, see our earlier discussion on precise element targeting.
Choosing the Right Locator for Your Test
Picking the right locator involves understanding your web page structure and how likely elements are to change. If an element has a unique ID, use it. Otherwise, a name attribute can work. CSS selectors offer flexibility and efficiency, while relative XPaths are a good fallback. Choosing wisely upfront saves debugging time later.
Building Robust and Reliable Selenium Tests
Organize Your Tests with the Page Object Model (POM)
Maintaining a large test suite can be tough. The Page Object Model (POM) helps organize your tests by creating a class for each page in your application. This promotes code reusability and simplifies updates. For maintainable tests, POM is essential.
Readable Tests with a Domain Specific Language (DSL)
Want your test code to be more understandable? A Domain Specific Language (DSL) lets you create custom code that makes tests more readable, even for non-technical folks. This can improve collaboration and maintenance.
Managing Your Application's State Effectively
Before each test, ensure your application is set up correctly. This might involve logging in, preparing a database, or configuring settings. A consistent setup ensures reliable results and prevents unexpected behavior from leftover data.
Test Isolation with Mock Dependencies
External dependencies (databases, APIs) can make tests unpredictable. Mocking these services isolates your tests and controls their behavior, making them faster and more reliable. It also helps you test edge cases and errors that are hard to reproduce otherwise.
Creating Useful Test Reports
Clear test results are key for quick feedback. Use good reporting tools and practices. Detailed reports with logs, screenshots, and error messages help you quickly identify and fix issues.
Writing Truly Independent Tests
Tests should be independent and self-contained. Avoid shared state, as it can cause unexpected interactions and flaky results. Each test should start fresh, ensuring you can run them in any order without impacting reliability.
Fresh Browsers for Reliable Test Results
A fresh browser instance for each test ensures a clean environment and prevents interference. This improves reliability, especially with complex interactions or browser-specific quirks.
Consistent Browser Settings: Key to Test Reliability
Maintaining consistent browser settings is crucial for reliable Selenium tests. Think of it like baking a cake—if you change oven temperatures mid-bake, your results will be unpredictable. Similarly, fluctuating browser settings can lead to inconsistent test outcomes. For example, setting the zoom level to 100% and maximizing the browser window ensures the visual layout of your application remains consistent. This seemingly small detail can prevent discrepancies, especially when dealing with elements positioned or styled differently based on the browser window size. Industry experts at BrowserStack highlight this as a key best practice in Selenium automation.
Replicating your production setup within your test environment is equally important. This minimizes discrepancies during testing and ensures your tests accurately reflect real-world user interactions. At MuukTest, we emphasize this best practice to help our clients ensure a consistent user experience across different browsers and environments—a critical aspect for modern web applications. Prioritizing cross-browser testing across different browser and operating system combinations, based on user data and analytics, further reinforces the importance of consistent browser settings for valid test results.
Faster Feedback with Concise Tests
Shorter, focused tests provide faster feedback and simplify debugging. Aim for tests that target specific functions, rather than covering too much at once.
Clear and Descriptive Test Names: Best Practices
Use descriptive test names that clearly explain what each test verifies. This makes it easier for everyone to understand their purpose and improves report readability.
Improve Test Readability with a Fluent API
A Fluent API dramatically improves the readability of your Selenium tests. It's like writing in plain English. This approach chains commands, creating a clear flow that expresses the test's purpose. For example, instead of separate commands for finding an element, clicking it, and typing text, a Fluent API streamlines these actions. This chained approach boosts readability and simplifies the process of following the steps within your test, especially as complexity increases. This style also simplifies maintenance and updates because the logic flows naturally. This approach is encouraged within the Selenium documentation for more maintainable tests.
Consistent Results Through Effective State Management
Reliable tests require a predictable environment. Before each test, ensure your application starts in the correct state. This might involve logging in, preparing a test environment, or configuring settings. A consistent starting point ensures reliable results, preventing unexpected behavior from leftover data. This practice helps prevent flaky tests and simplifies debugging. Controlling your application's initial state is crucial for robust test automation. For complex scenarios, consider tools that automate environment setup and teardown. Maintaining consistent test conditions is a best practice highlighted by many testing resources.
Prioritizing Implicit and Explicit Waits over Thread.sleep()
Timing is everything in web testing. Web pages load at different speeds, influenced by factors like network conditions and server load. Hardcoding wait times with Thread.sleep()
in your Selenium tests creates brittle tests. If the page loads faster than the fixed wait, you’re wasting precious time. Worse, if the page loads slower, your test will fail. Ditch Thread.sleep()
and embrace Selenium's implicit and explicit waits. Experts champion this approach for building robust and reliable tests.
An implicit wait instructs WebDriver to poll the DOM for a specified duration when searching for an element. If the element isn't immediately present, WebDriver patiently checks for its appearance before throwing an exception. This handles minor loading delays gracefully. For more specific scenarios, use explicit waits. Explicit waits allow you to define precise conditions that WebDriver should wait for. For instance, you might wait for an element to become clickable or for specific text to appear. This targeted waiting strategy provides granular control over how your tests handle dynamic web page content.
Leveraging Parallel Testing for Faster Test Execution
Want a speed boost for your test suite? Parallel testing is the answer. Instead of running tests sequentially, execute them concurrently across multiple browsers and devices. This dramatically reduces the overall test execution time. This best practice is essential for efficient test automation. Selenium Grid simplifies parallel testing by distributing tests across various machines and environments. This maximizes resource utilization and accelerates feedback cycles. At MuukTest, we harness the power of parallel testing to optimize test execution speed and deliver comprehensive test results quickly.
Proactive Test Design for Scalability and Maintainability
Before writing any test code, invest time in planning. Consider what you need to test, how you'll structure your tests, and your test environment and data management strategy. Careful planning prevents future maintenance headaches. A well-structured test suite is easier to scale and maintain as your project grows. The Page Object Model (POM) is a valuable pattern for organizing your tests. POM creates a class for each page in your application, promoting code reusability and simplifying updates. Organize your test scripts logically, grouping them by functionality or test type. This logical grouping enhances both scalability and maintainability, making it easier to manage large test suites and adapt to evolving project needs.
Practical Tips for Selenium Success
Headless Browsers: Speed Up Your Tests
When visual feedback isn't needed, use headless browsers. They run without a GUI, speeding up test execution, which is especially useful for continuous integration.
Simplify Debugging with Screenshots
Screenshots are invaluable for debugging. Configure your tests to automatically capture them on failure, providing visual context to pinpoint the cause.
Real Device Testing for Accurate Results
Emulators and simulators are helpful tools, but they don't fully replicate the real-world quirks of different devices and browsers. Testing on real devices and browsers gives you the most accurate results, catching issues that might slip through the cracks otherwise. Cloud-based testing platforms like BrowserStack can provide access to a wide range of devices if you need them.
Prioritize Your Selenium Tests
Concentrate your testing efforts where they matter most—the critical parts of your application. Prioritizing high-risk areas and core functionalities ensures that the most important features are thoroughly vetted, maximizing the impact of your testing strategy.
Reusable Test Components: Write Once, Use Often
Repetitive code is a maintenance nightmare. Create reusable code components for common actions and functionalities. This practice streamlines your codebase and makes updates much easier. A single change in one component applies everywhere it's used, boosting efficiency and consistency.
Avoid Hardcoding Test Data
Hardcoding data directly into your test scripts makes them rigid and difficult to maintain. Store your test data externally, whether in separate files, databases, or configuration files. This separation allows you to easily modify test data without touching the test scripts themselves, increasing flexibility.
Assert vs. Verify: Making the Right Choice
Use assertions for critical failures that should stop the test immediately. Use verifications for less critical issues that you want to track but don't need to halt the entire test run. Understanding this difference gives you finer control over how your tests handle errors.
Effective Data-Driven Testing with Selenium
Data-driven testing lets you run the same test with various data sets, significantly expanding your test coverage without writing extra code. This is especially helpful for testing different input scenarios and boundary conditions, ensuring your application handles a wide range of user inputs.
Maintain a Clear Project Structure
A well-organized project is easier to understand and work with. Maintain a consistent directory structure for your test files and resources. This makes it simple for everyone on the team to find what they need and collaborate effectively.
Should You Use a BDD Framework?
Behavior-Driven Development (BDD) frameworks like Cucumber help bridge the gap between technical and non-technical team members. BDD frameworks use plain language to describe test scenarios, making them accessible to everyone involved in the project.
Maintaining Tests for Evolving Web Apps
Web applications are constantly changing. New features get added, designs get tweaked, and code gets updated. This means your Selenium tests need regular care and attention to stay effective. Think of it like keeping your car running smoothly—regular maintenance prevents bigger problems down the road. Here’s how to keep your Selenium tests in top shape:
First, regularly update your tests. As your web application evolves, so should your test scripts. Make sure to review and adjust them alongside development changes, ensuring they accurately reflect the current functionality. Out-of-date tests can lead to false positives or miss critical bugs, defeating the purpose of automated testing. Regular test updates are crucial for reliable results.
Maintaining a consistent user experience is paramount. Users expect your application to work seamlessly across different browsers and devices. A well-structured Selenium test suite helps catch inconsistencies early. Ensure your tests cover various environments and reflect any UI changes. As mentioned earlier, using the Page Object Model (POM) significantly helps maintain a consistent user experience as your application grows.
Robust error handling and debugging are essential. When tests fail (and they will!), detailed logs and error messages can save you hours of frustration. Implement clear logging within your Selenium tests to quickly identify the root cause of issues. Thorough error handling and debugging maintain the integrity and reliability of your automated tests.
Finally, integrate your Selenium tests into your continuous integration (CI) pipelines. This automates testing with every code change, providing rapid feedback to developers and catching integration issues early. CI integration reduces risk and promotes a more efficient development workflow. Services like MuukTest can streamline this integration, ensuring comprehensive test coverage and faster release cycles.
Understanding the Nuances of Different Wait Types
Timing is everything in Selenium tests. Web pages load at different speeds, and if your test tries to interact with an element before it's ready, it will fail. That's where waits come in. Avoid using Thread.sleep()
, which pauses execution for a fixed time. It's inefficient and makes tests unreliable. Instead, use Selenium's built-in wait mechanisms:
Implicit Waits: Setting a Global Timeout
An implicit wait tells WebDriver to poll the DOM for a certain amount of time when trying to find an element. If the element isn't immediately available, WebDriver will keep checking for it within the specified timeframe. This is a global setting, applying to all element searches in your test. It's a good way to handle minor delays.
Explicit Waits: Waiting for Specific Conditions
Explicit waits are more targeted. You define a specific condition, such as an element becoming visible, clickable, or having specific text. WebDriver waits until that condition is met, up to a maximum time you specify. This approach is more flexible and efficient than implicit waits, especially for elements that might take longer to load. Experts at both BrowserStack and LambdaTest recommend using implicit or explicit waits over fixed sleeps.
Benefits of External Test Data Storage
Keeping your test data separate from your test code is crucial. Hardcoding data directly into your scripts makes them inflexible and difficult to update. Imagine changing values scattered throughout hundreds of tests! Externalizing your test data offers several advantages:
Easy Maintenance and Updates
Storing test data in separate files (like CSV, Excel, or JSON) simplifies management and modification. You can update test data without altering your test scripts, streamlining maintenance and reducing errors. BrowserStack highlights this as a best practice.
Improved Test Reusability
With external data, you can reuse the same test script with different data sets. This is incredibly useful for data-driven testing, where you test the same functionality with various inputs. LambdaTest emphasizes avoiding hardcoded data.
Better Organization and Collaboration
Separating test data improves the organization of your test suite. It clarifies what data each test uses, making collaboration smoother and reducing confusion. This also simplifies version control and tracking changes.
Using Parameterized Tests for WebDriver Flexibility
Don't restrict your tests to a single browser. Parameterized tests let you run the same test logic with different WebDriver instances, enabling cross-browser testing with minimal code duplication. This ensures your web application works correctly across various browsers.
Write Once, Test Everywhere
With parameterized tests, you write your test logic once and provide different browser configurations as parameters. This lets you easily test on Chrome, Firefox, Safari, Edge, or any other browser supported by Selenium WebDriver. LambdaTest recommends this for flexibility.
Simplified Cross-Browser Testing
Parameterized tests streamline cross-browser testing. You don't need separate test scripts for each browser, saving time and effort while maintaining a comprehensive test suite. BrowserStack also supports using multiple WebDriver implementations.
Selenium 4: Considerations for Upgrading
Selenium 4 introduced significant improvements and new features. While upgrading might require some adjustments, the benefits are often worth it. Here's what to consider:
New Features and Enhancements
Selenium 4 offers enhanced support for the W3C WebDriver protocol, improved Chrome DevTools interactions, and relative locators, simplifying test creation and improving stability. LambdaTest discusses these advantages.
Compatibility and Deprecations
Before upgrading, review the Selenium 4 documentation for deprecated methods or changes that might affect your existing tests. BrowserStack suggests considering an upgrade to leverage these advancements.
Common Selenium Pitfalls to Avoid
Challenges in Selenium Functional Testing
Functional testing with Selenium can present challenges due to application complexity, dependencies on external systems, and browser inconsistencies. Being aware of these challenges is the first step. Plan your testing strategy accordingly, using tools and techniques like mocking external services and cross-browser testing platforms to address these issues.
Automating File Downloads
Selenium isn't designed for automating file downloads; it can be unreliable. Use HTTP request libraries instead for direct and more robust file downloads.
Automating CAPTCHAs
Avoid automating CAPTCHAs. They're designed to prevent automation, and trying to bypass them is often complex and against website rules. If CAPTCHA handling is unavoidable in your testing, explore alternatives like disabling CAPTCHAs in your test environment or using third-party CAPTCHA-solving services (proceed with caution).
Automating 2FA
Automating 2FA is tricky and generally best avoided. If you must test with 2FA, consider dedicated test accounts with 2FA disabled or alternative authentication methods within your test environment.
Link Spidering/Crawling with Selenium: Is it a Good Idea?
Selenium isn't the right tool for extensive link spidering or crawling. Use dedicated web crawling tools for more efficient and robust website traversal and data extraction.
Automating Logins on Major Platforms
Be careful when automating logins on major platforms like Google, Facebook, or Twitter. They often have security measures to detect and block automated logins. Respect their terms of service and consider alternatives like dedicated test accounts or mocking the login process.
Managing Test Dependencies Effectively
Keep your tests independent. Avoid scenarios where one test relies on another. Independent tests can run in any order and are more reliable, preventing cascading failures.
Performance Testing with Selenium
Selenium can handle basic performance measurements, but it's not a dedicated performance testing tool. For in-depth performance testing, use specialized tools like JMeter or Gatling, designed for load testing and performance analysis.
Avoid Blocking Sleep Calls
Don't use blocking sleep calls like Thread.sleep()
or time.sleep()
. They slow down your tests and make them brittle. Use Selenium's implicit and explicit waits instead to handle dynamic page elements and asynchronous operations for more efficient and reliable tests.
Set Browser Zoom to 100%
Set your browser zoom level to 100% during testing. Different zoom levels can affect how web pages render, leading to inconsistent results. A consistent 100% zoom ensures consistent behavior across different environments.
Maximize Your Browser Window
Maximize your browser window during tests to ensure all elements are visible and accessible. This is especially important for screenshots and verifying page layouts.
Choosing the Right Web Locators
Choose your web locators strategically. Prioritize IDs, then names, followed by CSS selectors. Use XPaths only when necessary, and avoid absolute XPaths. This makes your tests more robust and resistant to UI changes.
Create a Browser Compatibility Matrix
A browser compatibility matrix helps you define which browsers and operating systems you need to test on. This allows you to prioritize your cross-browser testing and ensures comprehensive coverage.
Prioritizing Browser Compatibility Testing
Cross-browser compatibility testing is crucial. Users expect a consistent experience no matter which browser they use. A well-structured Selenium test suite helps catch inconsistencies early. But how do you prioritize which browsers to test? A browser compatibility matrix is a great place to start. This matrix helps define the most important browsers and operating systems for your audience, ensuring you're testing where it matters most. Consider your user demographics—are they primarily on Chrome, Firefox, Safari, or Edge? Do they mostly use desktop or mobile? This data informs your testing strategy.
Prioritization is key, especially with limited resources. Focus on the browsers your target audience uses most. Testing on real devices offers the most accurate results, but emulators and simulators can be a cost-effective starting point. Like functional testing, browser compatibility testing should concentrate on critical user flows. Ensure core features work seamlessly across prioritized browsers before testing less critical aspects. This risk-based approach thoroughly vets the most important features. For a more streamlined approach to comprehensive testing, explore MuukTest's test automation services.
Selenium, with its cross-browser support, simplifies this process. Write tests once and run them across multiple browsers, eliminating repetitive manual testing. This efficiency frees up your team to focus on other important tasks. A consistent user experience is crucial for user satisfaction and retention. Prioritizing browser compatibility testing ensures your web application delivers a smooth experience, regardless of browser choice.
Effective Logging and Reporting
Use logging to capture detailed information about your test runs. Use appropriate log levels and generate comprehensive reports with logs, screenshots, and error messages. This information is crucial for debugging and tracking test results.
Design Autonomous Test Cases
Create autonomous test cases that can run independently. This enables parallel execution, speeding up testing and improving efficiency. Independent tests are also more reliable, as a failure in one won't impact others.
Cross-Browser Testing with Multiple WebDriver Implementations
Cross-browser testing is essential for a consistent user experience. Using multiple WebDriver implementations within Selenium is key. Think of WebDrivers as translators between your tests and the browsers. Each browser has a specific WebDriver: ChromeDriver for Chrome, geckodriver for Firefox, and so on. By incorporating these different WebDrivers, you can run the same tests on various browsers, ensuring your application functions and looks as expected, regardless of the user's browser choice.
Using multiple WebDrivers provides flexibility. Don't rely on just one. Instead, parameterize your tests to handle different browsers. This lets you easily switch between browsers without rewriting code. For example, test on Chrome, Firefox, and Edge by simply changing a parameter.
Selenium lets you create test scripts that run across major browsers, eliminating manual testing. Combining this with Selenium Grid allows parallel execution across multiple machines and environments. This significantly speeds up testing and improves coverage, providing faster feedback and a consistent user experience.
Mastering Waits in Selenium: Avoid Timing Issues
Handle Dynamic Content with Explicit Waits
Use explicit waits to handle dynamic web pages where elements load at different times. Explicit waits tell Selenium to wait for a specific condition, preventing failures due to timing issues. Avoid implicit waits, as they can cause unnecessary delays.
Faster Tests with Parallel Testing
Accelerate Your Tests with Parallel Execution
Parallel testing significantly reduces overall testing time by running multiple tests concurrently across different environments. This allows for faster feedback and quicker iterations.
Scale Your Tests with Cloud-Based Grids
Cloud-based Selenium grids offer access to a wide range of browsers and operating systems, enabling large-scale testing without managing your own infrastructure. This is a powerful way to ensure cross-browser compatibility.
Leverage Multiple Driver Implementations
Don't rely on just one WebDriver. Design your tests to work with different WebDriver implementations. This approach ensures your tests can adapt to various environments and browser updates, making your testing strategy more robust. Using multiple drivers, like ChromeDriver for Chrome and GeckoDriver for Firefox, lets you cover more user scenarios and catch browser-specific issues. This adaptability is key for maintaining reliable tests as browsers change. For more helpful advice, LambdaTest offers insights into Selenium best practices.
Maintaining Your Selenium Tests
Regularly update your tests to keep them working correctly. Test maintenance is crucial. It ensures that your automation suite remains effective and relevant as your application evolves. This practice helps identify and fix issues quickly, keeping your testing framework aligned with the latest application changes. Just like any code, Selenium tests require ongoing care. Refactoring tests, updating locators, and addressing deprecated methods all contribute to a healthy test suite. BrowserStack provides a helpful guide on Selenium automation best practices, including the importance of regular maintenance.
A Comprehensive Approach to Selenium Testing
Selenium Guidelines: Best Practices for Success
The best approach to Selenium testing depends on your project. Treat best practices as guidelines, not rules. Be flexible and adapt. The Selenium documentation emphasizes this adaptability.
Learning Selenium: Your Path to Automation
So, you’re ready to dive into Selenium and automate all the things? Great! It's a powerful tool, and learning it can significantly improve your testing efficiency. But like any new skill, it takes time and dedicated effort.
How Long Does it Take to Learn Selenium?
If you're starting with no programming experience, expect to spend about two to four months learning the basics. This assumes you're dedicating a few hours each day to studying and practicing. It might seem like a significant time investment, but grasping the fundamentals of programming alongside Selenium is worthwhile. If you already have programming experience, especially with Java or a similar language, the learning process will be quicker. You could be up and running in one to two months. These are general estimates, as suggested by resources like GeeksforGeeks. Your own timeline will depend on your learning style, the time you invest, and your prior experience.
Don't feel intimidated by these timelines. Start with small steps, concentrate on core concepts, and gradually expand your skills. Remember, even seasoned Selenium users are always learning and refining their techniques. The field of test automation is constantly evolving.
Learning Resources and Communities for Selenium
Ready to dive deeper into Selenium? Tons of resources exist, from structured courses to active communities, making leveling up your skills easier than ever. Whether you’re a visual learner, prefer hands-on tutorials, or thrive in collaborative environments, you’ll find something that fits.
For a comprehensive overview, check out Prometteur Solutions’ list of the top 13 resources for learning Selenium. They cover everything from beginner tutorials to advanced framework creation. If you’re looking for a structured learning path, recommendations on Reddit offer a great transition from manual to automated testing, starting with the basics and progressing to more complex topics like Selenium Grid and Cucumber BDD. Joe Colantonio from Test Guild also curated a helpful list of books and video courses perfect for Selenium newbies.
The beauty of open-source tools like Selenium lies in the vibrant community. As mentioned earlier, the Selenium community offers incredible support. You’ll find solutions to almost any Selenium challenge through online forums, communities like Stack Overflow, and dedicated Selenium groups. DZone also offers a helpful compilation of 13 resources for learning Selenium automation, including courses and cheat sheets. And for a quick overview of top learning resources, Test Bytes offers a concise list.
Hands-on Practice: The Key to Selenium Mastery
Reading tutorials and watching videos is helpful for getting started, but true mastery comes from hands-on practice. As GeeksforGeeks points out, practical experience is essential for solidifying your understanding of Selenium. The more you experiment, the more comfortable you'll become with writing and executing tests. Work on personal projects or contribute to open-source projects to gain valuable real-world experience. There are numerous online resources available, including tutorials, documentation, and community support forums. Don't hesitate to use these resources when you need help or guidance. The Selenium community is known for being supportive and helpful.
If you're looking for a fast and efficient way to implement comprehensive testing, consider MuukTest's AI-powered test automation services. We can help you achieve complete test coverage within 90 days, allowing your team to focus on other priorities. See how we've helped other businesses by checking out our customer success stories. Ready to learn more? Visit our QuickStart page.
Streamline Selenium Test Automation with MuukTest
Building and maintaining a comprehensive suite of Selenium tests can be complex. MuukTest specializes in AI-powered test automation, designed to enhance your existing Selenium workflows and achieve complete test coverage efficiently.
Comprehensive Test Coverage with MuukTest
At MuukTest, we understand the power and flexibility of Selenium. Its cross-browser support and language flexibility are key advantages for development teams. We build upon these strengths, integrating our AI-powered automation to create and maintain a robust test suite. This ensures a consistent user experience across different browsers and environments. Our approach allows us to achieve comprehensive test coverage within 90 days, a significant improvement compared to traditional methods. Learn more about our test automation services.
MuukTest: Benefits for Selenium Test Automation
MuukTest streamlines several key aspects of Selenium test automation. We leverage best practices like the Page Object Model (POM) for maintainable tests, ensuring your test suite remains organized as your application grows. Our platform supports parallel testing, dramatically reducing testing time and providing faster feedback. We also prioritize clear, concise reporting, enabling quick identification and resolution of issues. Seamless integration with your CI/CD pipeline ensures automated testing with every code commit. Explore our customer success stories, or check out our pricing plans. Ready to get started? Visit our quickstart guide.
Advanced Selenium Techniques to Explore
Once familiar with Selenium basics, these advanced techniques can help teams improve test efficiency:
- Page Object Model (POM): Use POM to separate web element code from test scripts. This reduces redundancy and simplifies test maintenance.
- Waits and sleeps: Use implicit or explicit waits to handle dynamic content. Avoid hardcoded waits, which slow down execution.
- Handling dynamic elements: Use flexible locators like XPath to manage elements with frequently changing attributes.
- Parallel test execution: Selenium Grid supports parallel testing across browsers, reducing test execution time and improving CI/CD workflows.
- Integration with other tools: Integrate Selenium with other testing frameworks like TestNG or JUnit for better test management, reporting, and execution.
Troubleshooting Common Selenium Issues
While Selenium is versatile, it does come with a few challenges that teams must address.
Test flakiness is one common issue, often caused by timing mismatches or unreliable locators. These can be resolved by using waits (implicit or explicit) and more stable locators like XPath or CSS Selectors, which improve reliability.
Frequent UI changes can lead to test maintenance challenges, where scripts break due to updates in the application. Using the Page Object Model (POM) design pattern helps separate the logic from locators, simplifying maintenance and reducing script fragility.
For complex web applications, especially those with heavy JavaScript usage, Selenium can struggle to interact with certain elements. In such cases, using JavaScript executors or integrating Selenium with specialized tools helps manage these complexities more effectively.
Selenium isn’t designed for performance testing, which limits its ability to evaluate application performance under load. Tools like JMeter or Lighthouse can complement Selenium’s functional tests, providing insights into performance alongside functionality.
Start Automating with Selenium Today
Selenium test automation is a powerful tool for engineering teams aiming to improve software quality and efficiency. Its cross-browser compatibility, open-source nature, and strong community support make it ideal for teams seeking scalability.
Advanced techniques and best practices ensure maintainable, efficient testing processes. By integrating Selenium into their testing strategy, teams can create smoother workflows and better software, empowering them to focus on innovation and delivering reliable applications.
Related Articles
- A Comprehensive Guide to Selenium Test Automation
- Implementing Selenium Automation Testing Framework
- Your Complete Guide to Automated UI Testing
- 13 Key Differences Between Playwright vs. Selenium Frameworks
- How to Navigate Element Click Intercepted Selenium
Frequently Asked Questions
Is Selenium difficult to learn?
Selenium's user-friendliness depends on your coding background. The Selenium IDE requires minimal coding, making it easy to get started with recording and playing back tests. For WebDriver, some programming knowledge is helpful, but many resources and a supportive community are available to help you learn. Python is often recommended for beginners due to its readability.
How does Selenium handle testing on different browsers?
Selenium uses browser-specific drivers (like ChromeDriver for Chrome, geckodriver for Firefox) to interact with each browser. This allows you to write test scripts that work across various browsers, ensuring cross-browser compatibility for your web application. Selenium Grid further enhances this by enabling parallel testing across multiple browsers and operating systems simultaneously.
What's the difference between Selenium IDE and Selenium WebDriver?
Selenium IDE is a browser extension ideal for quick, record-and-playback tests without extensive coding. It's great for simple tests and getting started with Selenium. WebDriver, on the other hand, provides a more robust and flexible framework for complex test scenarios. It requires programming but offers greater control over browser interactions and integrates well with other testing tools.
How can I make my Selenium tests more maintainable?
As your test suite grows, maintaining it can become challenging. The Page Object Model (POM) is a design pattern that helps organize your tests by representing each web page as a class. This promotes code reusability and makes updates easier. Following a clear locator strategy (prioritizing IDs, names, then CSS selectors) also contributes to more robust and maintainable tests.
What if my web application changes frequently? How do I prevent my tests from breaking?
Frequent UI changes can indeed break your Selenium tests. Using robust locator strategies (like relative XPaths or CSS selectors that focus on stable attributes) helps minimize this. The Page Object Model (POM) also isolates the web element locators, making updates easier when the UI changes. Additionally, incorporating explicit waits in your tests allows them to handle dynamic content and timing variations, making them more resilient to changes.
Related Posts:
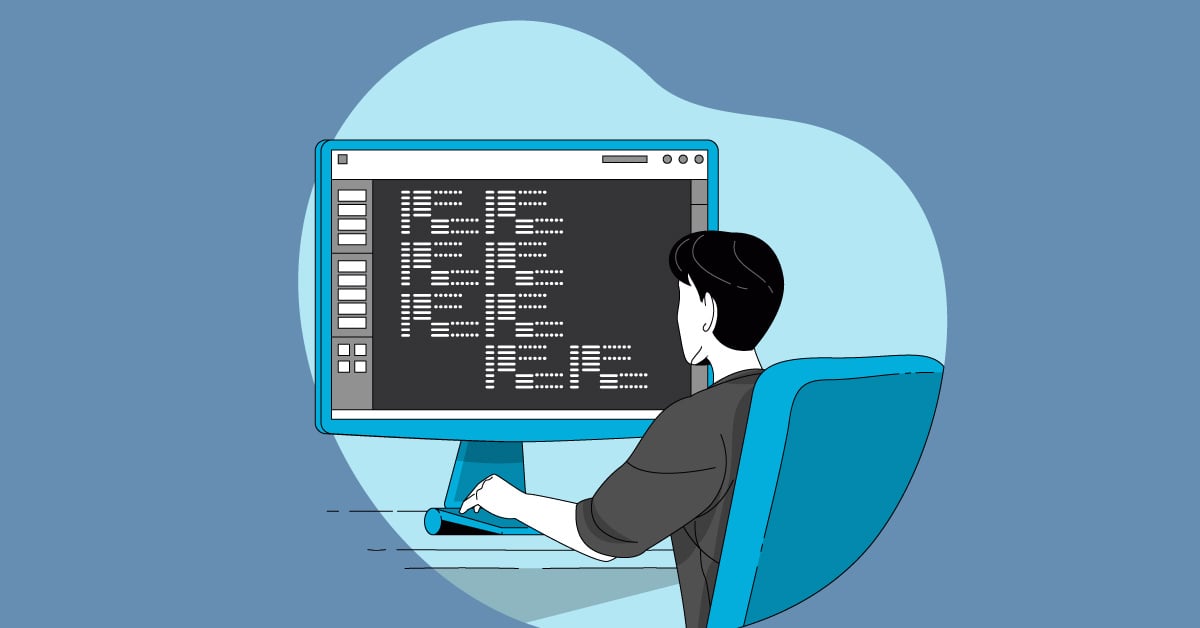
Ensure Seamless User Experiences With UI Automation
Creating a seamless user experience requires more than just a visually appealing design. It demands a reliable interface that performs flawlessly across a range of devices and platforms.
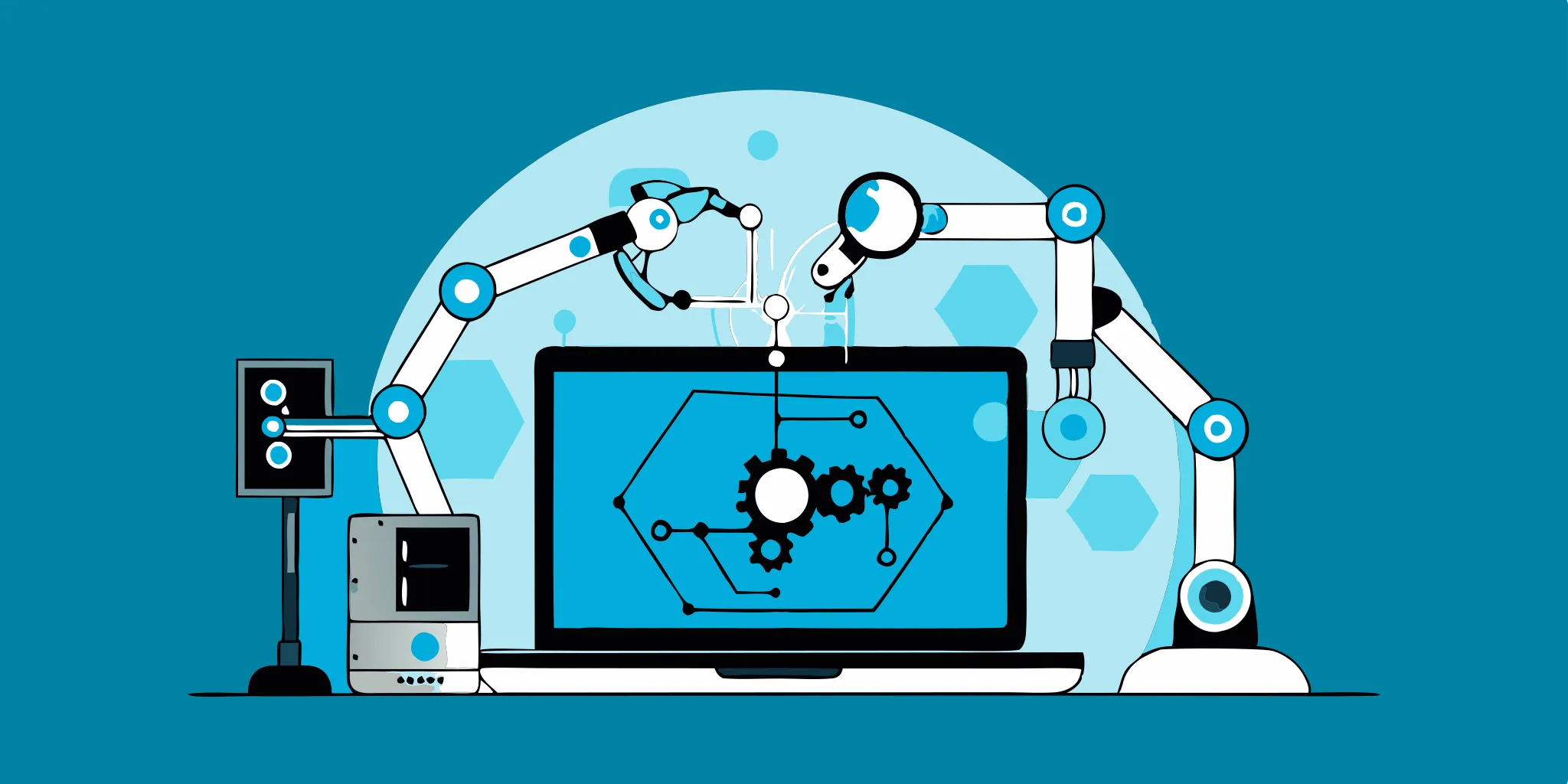
Automated UI Testing: Your Complete Guide
Tired of the endless grind of manual UI testing? As software becomes increasingly complex, ensuring a flawless user interface is more critical than ever. But manually clicking through every scenario...
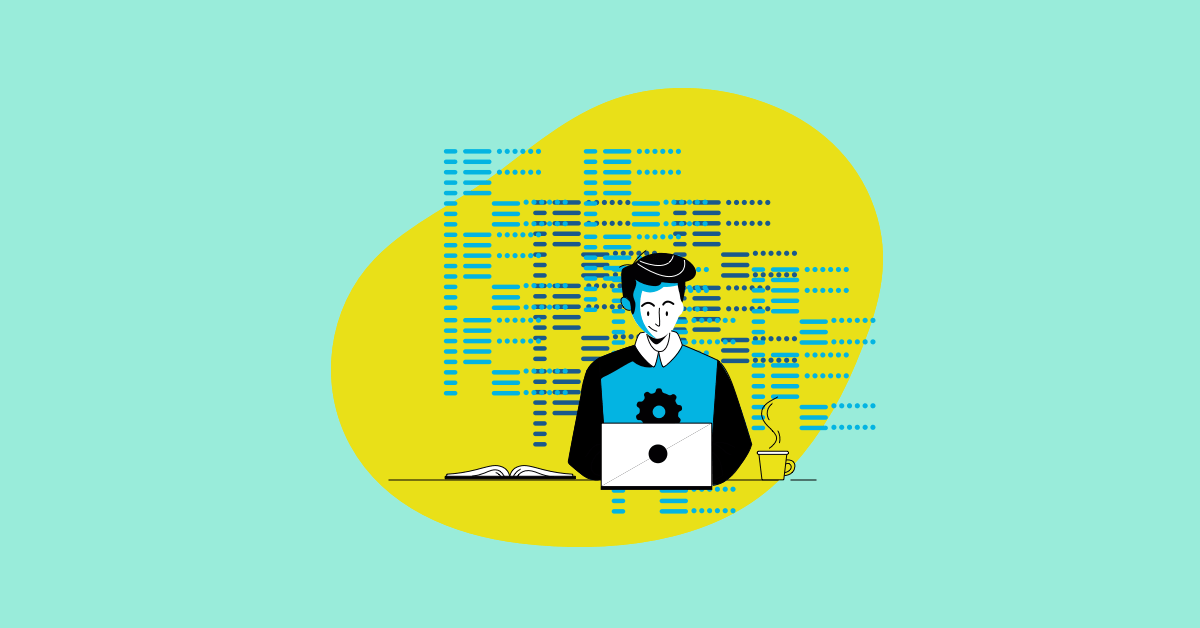
Why You Should Try Selenium Automation Testing Framework
When it comes to running tests, managing test data, and using those results to improve software quality, automation surely makes things simpler if you compare it to its counterpart, manual testing....