Python Unit Testing: A Practical Guide
Author: The MuukTest Team
Last updated: October 1, 2024
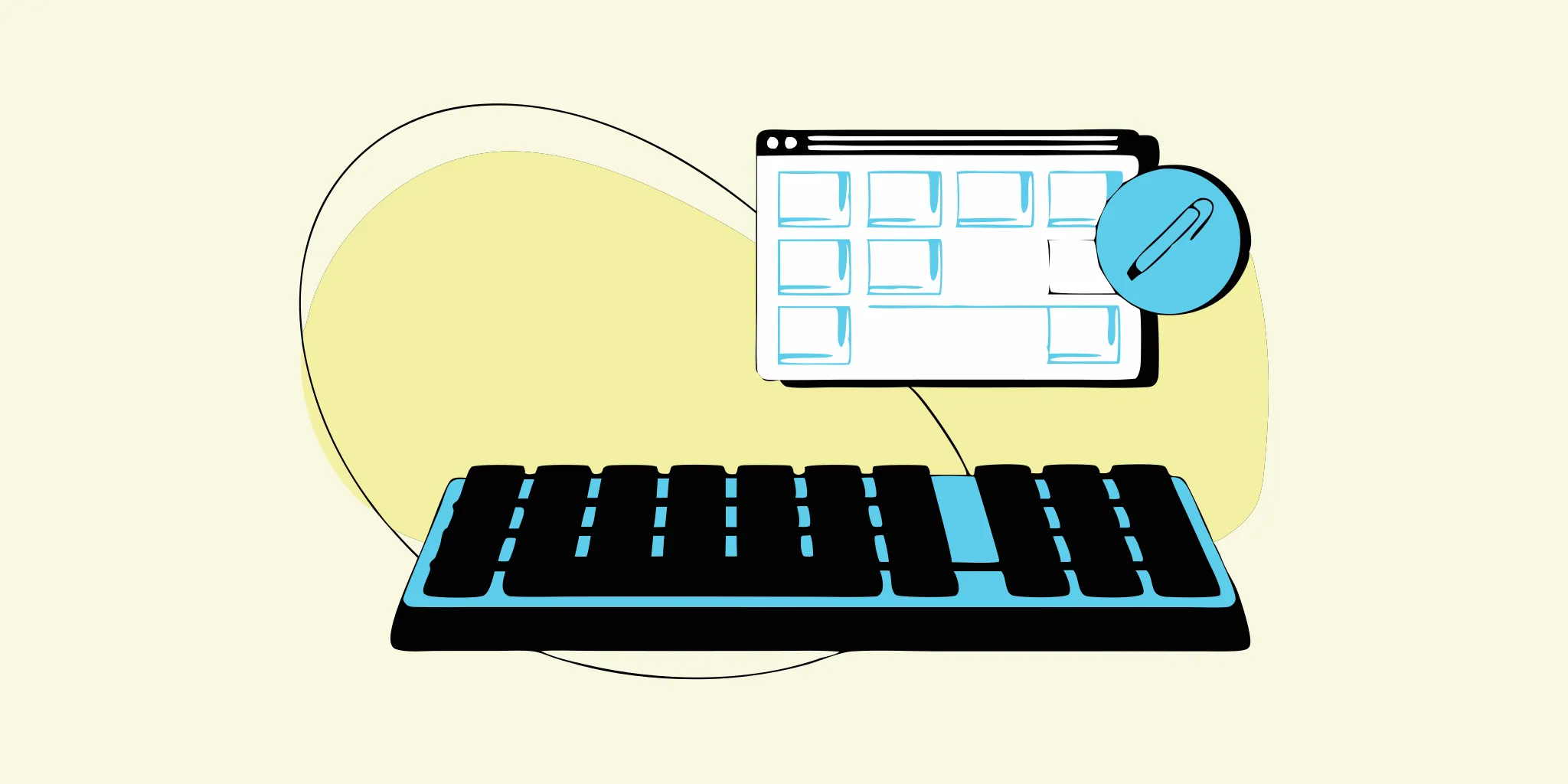
Table of Contents
Bugs. Every developer's nemesis. They lurk in the shadows of your code, waiting to pounce and wreak havoc on your application. But fear not, for there is a powerful weapon in the fight against these digital gremlins: the Python unit test. Unit testing is a fundamental practice in software development, allowing you to test individual components of your code in isolation, ensuring they function correctly before they're integrated into the larger system. This guide will provide a comprehensive overview of Python unit testing, from the basics of the unittest
framework to advanced techniques like mocking and test-driven development. We'll explore best practices for writing clear, concise, and effective Python unit tests, empowering you to build robust and maintainable software.
Key Takeaways
- Solid unit tests are essential for reliable Python code: Testing individual parts of your code in isolation helps catch problems early, making debugging easier and improving code quality. Frameworks like
unittest
orpytest
provide structure and organization for your tests. - Structure and focus are key to effective tests: Each test should check one specific thing. Use assertions to confirm expected results, and group related tests into suites. Use fixtures to set up and clean up your test environment and mocking to isolate your code from external dependencies.
- Make testing a habit: Automate your tests so they run regularly, ideally with every code change. Try writing tests before you write the code itself (TDD) – it can lead to better code design. Explore other testing tools and libraries as your needs evolve.
What is Python Unit Testing?
What is Unit Testing & Why Does It Matter?
Unit testing is a software testing method where individual components of your software are tested in isolation. Think of it like checking the individual parts of a car engine before assembling the entire thing. This approach lets developers verify each piece works as expected before integrating it with other components. Unit testing is a cornerstone of robust software development. It ensures code quality, facilitates easier maintenance, and gives you confidence when making code changes. A well-constructed test suite acts as a safety net, catching bugs early in the development cycle and preventing them from becoming larger, more complex problems. Conversely, a poorly designed suite of tests can lead to a fragile codebase, hidden bugs, and wasted time spent debugging.
Unit Testing's Role in Python Development
Unit testing plays a pivotal role in developing robust and reliable Python software. It's essential for ensuring your code behaves as intended and continues to function correctly as your project grows. By choosing the right framework and following best practices, you can write effective unit tests that make your Python projects more reliable and maintainable. This proactive approach to bug detection improves the quality of your code and streamlines the development process, allowing you to ship features faster and with greater confidence. Catching bugs early makes your code more reliable and reduces the overall cost and effort associated with fixing issues later.
Get Started with Python's unittest Framework
This section provides a practical introduction to Python's unittest
framework. We'll cover its core components and how to set up your testing environment. Whether you're new to testing or switching from another framework, this guide will give you a solid foundation.
Key unittest Components
The Python unittest
module is a built-in framework for unit testing, similar to those in other programming languages. It provides tools to automate tests, streamline setup and teardown processes, organize tests, and keep your test logic separate from reporting. Understanding its key components is crucial for writing effective tests.
The core concepts in unittest
revolve around four main elements: test fixtures, test cases, test suites, and test runners. A test fixture sets the stage for your tests, handling any necessary setup (like creating temporary files or databases) and cleanup. Think of it as preparing the environment for your tests.
A test case is the smallest unit of testing, focusing on a specific aspect of your code. You define test cases by creating classes that inherit from unittest.TestCase
. Inside these classes, you'll write individual test methods. These methods, which begin with "test_", contain assertions to verify the behavior of your code.
Test suites are collections of test cases (or even other test suites). They allow you to group related tests, making it easier to run them together. Finally, the test runner executes your tests and presents the results, whether through a command-line interface or a GUI.
Set Up Your Testing Environment
Unit testing is essential for building reliable and maintainable Python applications. It helps catch bugs early, simplifies debugging, and gives you confidence when making code changes. Choosing the right framework and following best practices are key to writing effective unit tests.
One recommended practice is dependency injection, a design pattern that makes your code more testable by decoupling its components. Another important principle is maintaining test independence. Each test should focus on a single unit of code in isolation and shouldn't rely on other tests. This ensures your tests are reliable and easy to understand. By following these principles, you can create a robust testing environment that improves the overall quality of your Python projects.
Anatomy of a Python Unit Test
This section explores the core components of a Python unit test, breaking down the structure and showing how these elements work together.
Test Cases: The Building Blocks
Think of unit tests as quality checks for small parts of your code—typically individual functions or methods. These tests confirm that each piece works correctly in isolation, which is fundamental to building robust software. A single unit of testing is called a test case. You create these test cases using Python's built-in unittest
framework by subclassing unittest.TestCase
. It's important to design each test case to focus on a single unit of code, keeping it independent from other tests. This isolation ensures that you can pinpoint the source of any issues if a test fails. This approach is crucial for creating reliable and maintainable Python applications.
Assertions: Verify Expected Outcomes
Assertions are at the heart of every test case. They verify that your code produces the expected output. The simple assert
statement in Python checks if a condition is true. If the condition is false, it raises an error, signaling a problem in your code. The unittest
framework provides a richer set of assertion methods like assertEqual
, assertTrue
, and assertFalse
. These methods offer more specific checks, making your tests more descriptive and easier to understand. Using assertions effectively is key to confirming that your code behaves as expected. This practice helps catch and address issues early in the development process, contributing to higher quality code.
Write and Organize Python Unit Tests
This section gets into the practical side of writing and structuring Python unit tests, setting you up for robust and automated testing.
Write Your First Test: A Step-by-Step Guide
Unit testing is essential for software development. It helps you confirm that individual parts of your code work as expected. Python's unittest
framework provides a structured way to create and run these tests. To write your first test, start by importing the unittest
module. Then, define a test case class that inherits from unittest.TestCase
. Within this class, create methods that begin with "test"—these are your individual test cases. For example, if you're testing a function that adds two numbers, your test case would call the function with specific inputs and use assertions to verify the output matches what you expect.
Structure Tests with Test Suites
As your project scales, organizing tests into test suites becomes essential. A test suite is a collection of test cases you can run together. This keeps things organized and makes running tests more efficient. When structuring your tests, make sure they are atomic and independent. Each test should focus on a single unit of code and not rely on the state of other tests. This isolation makes it easier to identify issues and keeps your tests reliable, even as your codebase changes. Well-structured tests are like building blocks—each one performs a specific function and combines with others to create a larger, stable structure.
Discover and Automate Tests
Automating tests is crucial for maintaining code quality. Continuous integration tools can automatically run your test suites whenever code changes. This early bug detection saves you time and effort. A well-structured test suite not only helps identify bugs early but also streamlines the testing process through automation. Integrating automated testing into your workflow acts like a safety net, catching regressions and ensuring your code stays stable and reliable. Tools like Jenkins and Travis CI can integrate with your testing framework, making automated testing a seamless part of development.
Advanced unittest Features & Techniques
Once you’ve grasped the basics of unittest
, exploring advanced features can significantly improve your testing approach. These techniques help create more robust, maintainable, and efficient tests.
Fixtures: Set Up Test Environments
Think of fixtures as the stage crew for your tests. They handle the setup and teardown of preconditions, ensuring a consistent testing environment. This is crucial for reliable tests, preventing dependencies between tests and making debugging easier. Instead of manually setting up resources (like database connections or mock objects) within each test, fixtures let you define reusable setup and teardown logic. This practice aligns with Dependency Injection, a best practice for writing testable code. By using fixtures, you ensure each test operates in isolation, preventing unexpected side effects and promoting predictable outcomes.
Mocking: Isolate Units for Testing
Mocking lets you isolate the unit of code you're testing by replacing external dependencies with controlled substitutes. Imagine testing a function that interacts with a database. Instead of actually connecting to the database (which can be slow and complex), you can use a mock object to simulate the database interaction. This approach speeds up your tests and allows you to test different scenarios, including error handling, without relying on a real database. This isolation ensures your tests focus solely on the functionality of the unit under test. By controlling the behavior of these mock objects, you can simulate various conditions and verify how your code responds.
Handle Edge Cases and Exceptions
Testing the "happy path" is essential, but robust tests also consider edge cases and exceptions. Edge cases are unusual or extreme inputs that can sometimes cause unexpected behavior. For example, if you're testing a function that calculates discounts, consider inputs like zero, negative values, or extremely large numbers. Your tests should also verify how your code handles exceptions. Does it gracefully catch errors? Does it provide informative error messages? By proactively testing these scenarios, you prevent runtime errors and build more reliable software. Remember, comprehensive testing includes verifying how your code handles unexpected inputs and situations.
Best Practices for Effective Python Unit Tests
Well-written unit tests are essential for maintaining code quality and reducing bugs. Here are some best practices to make your Python unit tests truly shine:
Write Clear, Focused Tests
Keep your tests laser-focused on specific behaviors. Each test should verify one aspect of your code's functionality. Avoid including implementation details in your tests; focus on what the code should do, not how it does it. This approach makes your tests more resilient to code changes and easier for others to understand. Think of it like a good user story: clear, concise, and focused on the outcome.
Maintain Test Independence
Each test should be a self-contained unit, independent of other tests. Avoid dependencies between tests, as this can lead to cascading failures and make it difficult to pinpoint the root cause of problems. If one test modifies shared state, it could impact the results of subsequent tests, creating confusion. Keep your tests atomic and isolated to ensure reliable and predictable results.
Ensure Comprehensive Test Coverage
Strive for comprehensive test coverage across your codebase. While achieving 100% coverage isn't always feasible or necessary, aim to test all critical paths and edge cases. A well-tested codebase provides confidence in its reliability and makes future modifications less risky. Unit testing is an investment in the long-term health of your project.
Beyond unittest: Explore Alternative Testing Tools
While Python's unittest
provides a solid foundation, exploring other tools can significantly improve your testing workflow. This section introduces pytest
and explores additional libraries that enhance your testing capabilities.
Pytest: A Powerful Testing Framework
As your projects grow, you might find that unittest
requires more verbose code and setup. Many developers prefer pytest
, especially for larger projects. Its concise syntax and rich features streamline testing. Where unittest
relies on specific class structures and methods like setUp
and tearDown
, pytest
uses plain functions and standard Python assert statements. This leads to cleaner, more readable tests. This simplicity doesn't sacrifice power; pytest
offers robust features for test discovery and fixture management, along with detailed reporting. Its extensibility through plugins makes it adaptable to various testing needs, from simple unit tests to complex integration tests. Discussions on platforms like Reddit often highlight these advantages, showcasing pytest
's popularity among Python developers. While unittest
, rooted in the Python standard library, provides a reliable starting point, pytest
offers a more modern and efficient approach for comprehensive testing. Articles like this one from TMS Outsource offer further insights into the strengths of each framework.
Enhance Tests with Additional Libraries
Testing often involves more than just unit tests. Python's rich ecosystem of libraries caters to diverse testing needs. For example, if you're working with web applications, Selenium provides tools for automating browser interactions, allowing you to simulate user behavior and test your application's front-end. For API testing, the Requests library simplifies making HTTP requests and verifying responses. These specialized libraries, alongside core testing frameworks like pytest
or unittest
, create a comprehensive toolkit. This integrated approach, discussed by resources like Testomat, streamlines your testing and ensures thorough coverage. Whether you're working on a small project or a large-scale application, leveraging these libraries with the right testing framework helps build robust and reliable software. Integrating these tools into your workflow addresses specific testing requirements and creates a more efficient and effective testing strategy.
Integrate Unit Tests into Your Development Workflow
Continuous Integration with Python Unit Tests
In software development, unit testing is crucial. A poorly designed test suite can create a fragile codebase, leading to hidden bugs and wasted debugging time. Well-constructed unit tests verify the functionality of individual code components in isolation. This not only improves code quality but also simplifies maintenance and increases confidence when making changes. Choosing the right testing framework and following best practices are essential for writing effective unit tests that make your Python projects more reliable and maintainable. Python libraries integrate easily with popular CI/CD tools, automating the testing process. This ensures tests run automatically with every code change, providing immediate feedback and preventing regressions.
Embrace Test-Driven Development (TDD)
Test-driven development (TDD) integrates unit testing directly into the development process. With TDD, you write tests before writing the code. This encourages you to think through the desired behavior upfront, leading to more modular and testable designs. A key principle of TDD is keeping tests atomic and independent. Each test should focus on a single unit of code in isolation. This practice simplifies debugging and makes tests more resilient to code changes. Avoid including implementation details in your tests. Techniques like dependency injection improve testability by decoupling components. By embracing TDD and following these best practices, you can create a robust and maintainable codebase with comprehensive test coverage. This proactive approach to testing catches bugs early, promotes better code design, and reduces long-term development costs.
Overcome Common Challenges in Python Unit Testing
Even with the best intentions, Python unit testing can present some hurdles. Let's break down common challenges and how to tackle them:
Deal with External Dependencies
One of the trickiest aspects of unit testing is managing external dependencies like databases, APIs, or file systems. You want your unit tests to focus solely on your code's logic, not the behavior of external systems. The solution? Techniques like mocking and stubbing. Mocking lets you simulate the behavior of these external dependencies, providing controlled responses without actually interacting with them. For example, if your code interacts with a database, you can mock the database connection to return predefined data for your tests. This isolates your tests and makes them faster and more reliable. The unittest.mock
library provides powerful tools for mocking in Python.
Improve Test Performance
Slow-running tests can drag down your development process. If your tests take too long, you're less likely to run them frequently, which defeats their purpose. One way to improve test performance is through dependency injection. By injecting dependencies into your code, you can easily swap them out for mock objects during testing, speeding things up considerably. Another tip is to focus on testing the core logic of your code and avoid unnecessary setup or teardown operations within your tests.
Address Common Misconceptions
There are a few misconceptions around unit testing that can hold developers back. Some believe unit tests are only for large projects, but even small projects benefit from the increased stability they offer. Others think unit testing takes too much time. While there's an initial time investment, unit tests save you time in the long run by catching bugs early and preventing regressions. Think of unit tests as an investment in code quality and maintainability. They help you build more reliable software and make it easier to refactor and modify your code with confidence. Embracing unit testing is a key step towards becoming a more effective and efficient Python developer.
Tools and Resources for Python Unit Testing
This section offers helpful resources for Python unit testing, including recommended libraries, frameworks, and useful learning materials.
Recommended Testing Libraries and Frameworks
Getting started with Python unit testing means choosing the right tools. Here are a few popular options:
- unittest: This framework, included in Python's standard library, provides a solid base for building test suites.
unittest
supports test organization and reuse, making it adaptable for projects of any size. It's an excellent starting point for developers new to testing. - PyUnit: Also known as
unittest
, PyUnit gives you the tools to create robust test suites that thoroughly examine your Python code. This classic framework remains a powerful option for ensuring code quality. - Other Libraries: Python has a rich ecosystem of testing libraries. For different testing needs, explore libraries like Selenium for web testing, Requests for API testing, or Locust for performance testing. These specialized tools can strengthen your overall testing strategy.
Useful Resources for Further Learning
Beyond the basics, many resources are available to deepen your understanding of Python unit testing:
- Importance and Best Practices: Solid unit testing practices are crucial for reliable applications. A well-constructed test suite prevents hidden bugs and saves debugging time. For practical examples and tips, see this helpful information on writing effective tests in Python.
- Choosing the Right Framework: Effective unit testing relies on choosing the right framework and following best practices. This guide highlights the importance of unit testing and offers guidance on implementing it effectively in Python. Understanding these principles helps create more reliable and maintainable projects.
Frequently Asked Questions
Why is unit testing important for my Python code?
Unit testing is like having a safety net for your code. It helps you catch bugs early in the development process, before they become bigger, more expensive problems. By testing individual pieces of your code, you can ensure each part works correctly on its own, making it much easier to build reliable and maintainable software. Plus, having a good set of unit tests makes it much less scary to change your code later, since you can easily check if your changes broke anything.
How do I choose between unittest
and pytest
?
If you're just starting out with testing, unittest
is a great choice. It's built into Python, so you don't need to install anything extra, and it provides a solid foundation for writing tests. pytest
, on the other hand, is known for its more concise syntax and powerful features, which can be especially helpful for larger projects. It also has a huge community and lots of plugins, making it very versatile. Ultimately, the best choice depends on your project's needs and your personal preferences.
What are some common mistakes to avoid when writing unit tests?
One common mistake is writing tests that are too complex. Each test should focus on a single, specific aspect of your code's behavior. Another pitfall is writing tests that depend on each other. Tests should be independent so that if one fails, it doesn't cause a chain reaction of failures. Finally, make sure your tests are actually testing something meaningful. A test that doesn't assert anything isn't very helpful!
How can I test parts of my code that interact with external systems, like databases or APIs?
Testing code that interacts with external systems can be tricky, but techniques like mocking can help. Mocking lets you simulate the behavior of these external systems without actually needing to connect to them. This makes your tests faster, more reliable, and easier to control. You can define exactly how the mock objects should behave, allowing you to test different scenarios and edge cases without relying on the real external system.
How do I integrate unit testing into my development workflow?
The best way to integrate unit testing is to make it a regular part of your coding process. Write tests as you write your code, or even before you write the code (this is called test-driven development). Also, consider using continuous integration tools to automatically run your tests whenever you make changes to your code. This helps catch regressions early and keeps your codebase healthy.
Related Posts:
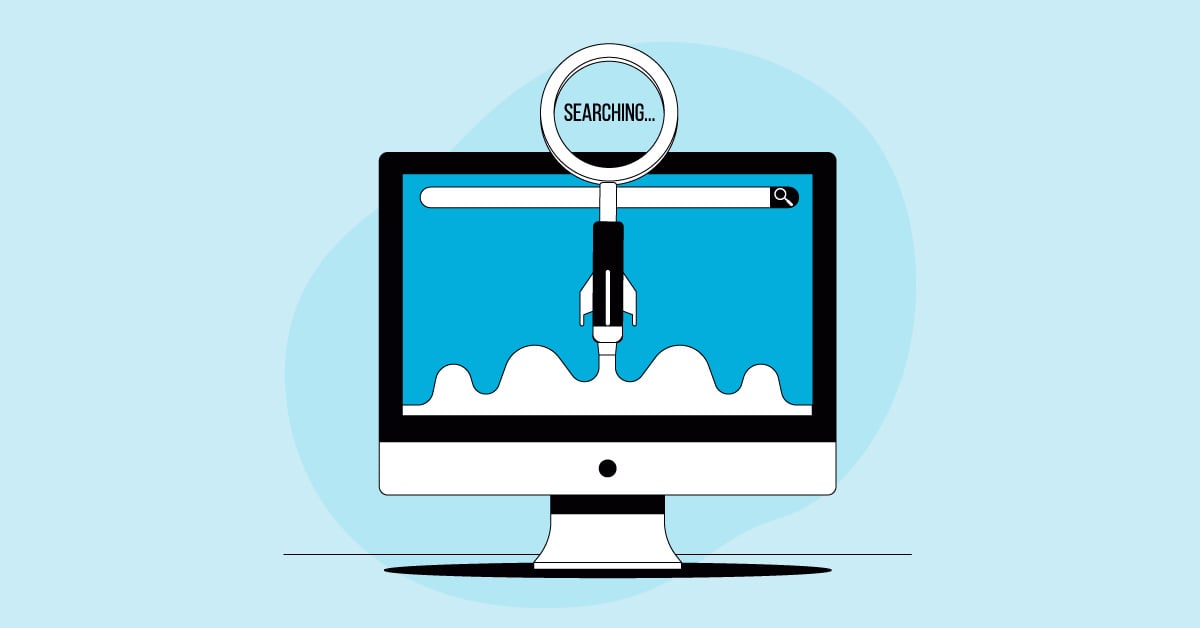
Unit Testing With Python: A Comprehensive Guide
In software development, few practices are as valuable for quality and reliability as unit testing. Unit testing involves assessing individual parts of code, or “units,” to confirm that each...
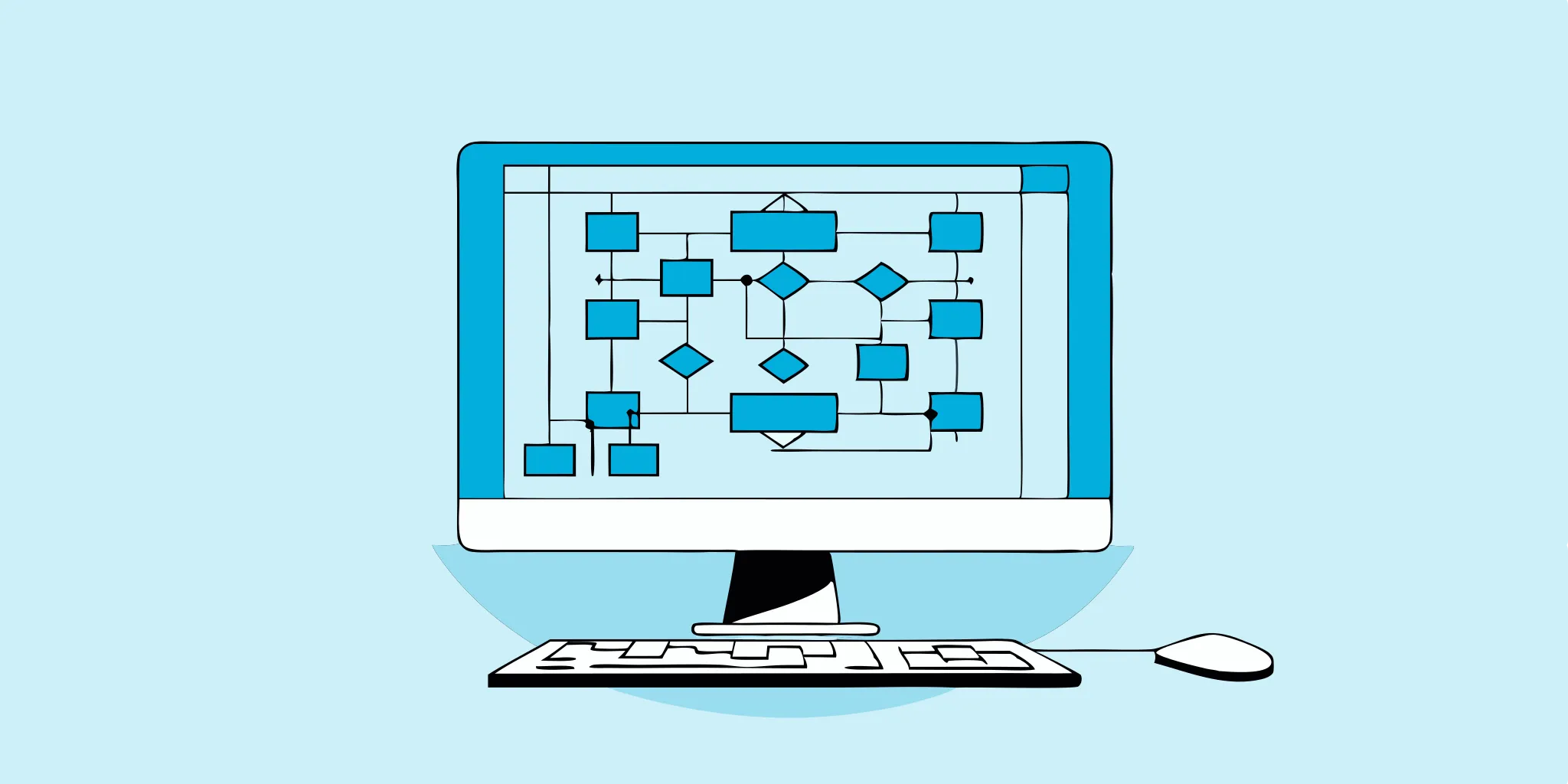
Software Unit Testing: A Complete Guide
In the world of software development, quality is paramount. Delivering a flawless product requires rigorous testing at every stage, and it all starts with software unit testing. This foundational...
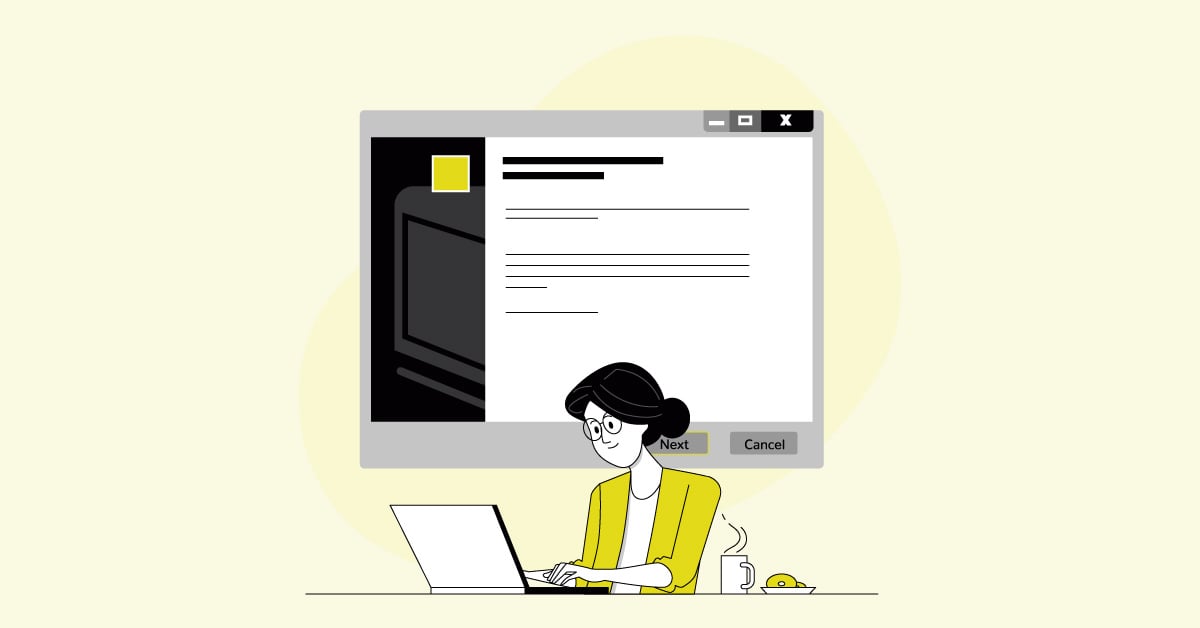
Unit Testing: A Foundation for Software Quality
Developers work hard to write clean, functional code, but even the most carefully crafted lines can carry hidden flaws. Testing these lines in isolation is a proven way to catch errors early, making...