What is Unit Testing? A Practical Guide for Developers
Author: Test Author
Last updated: October 1, 2024
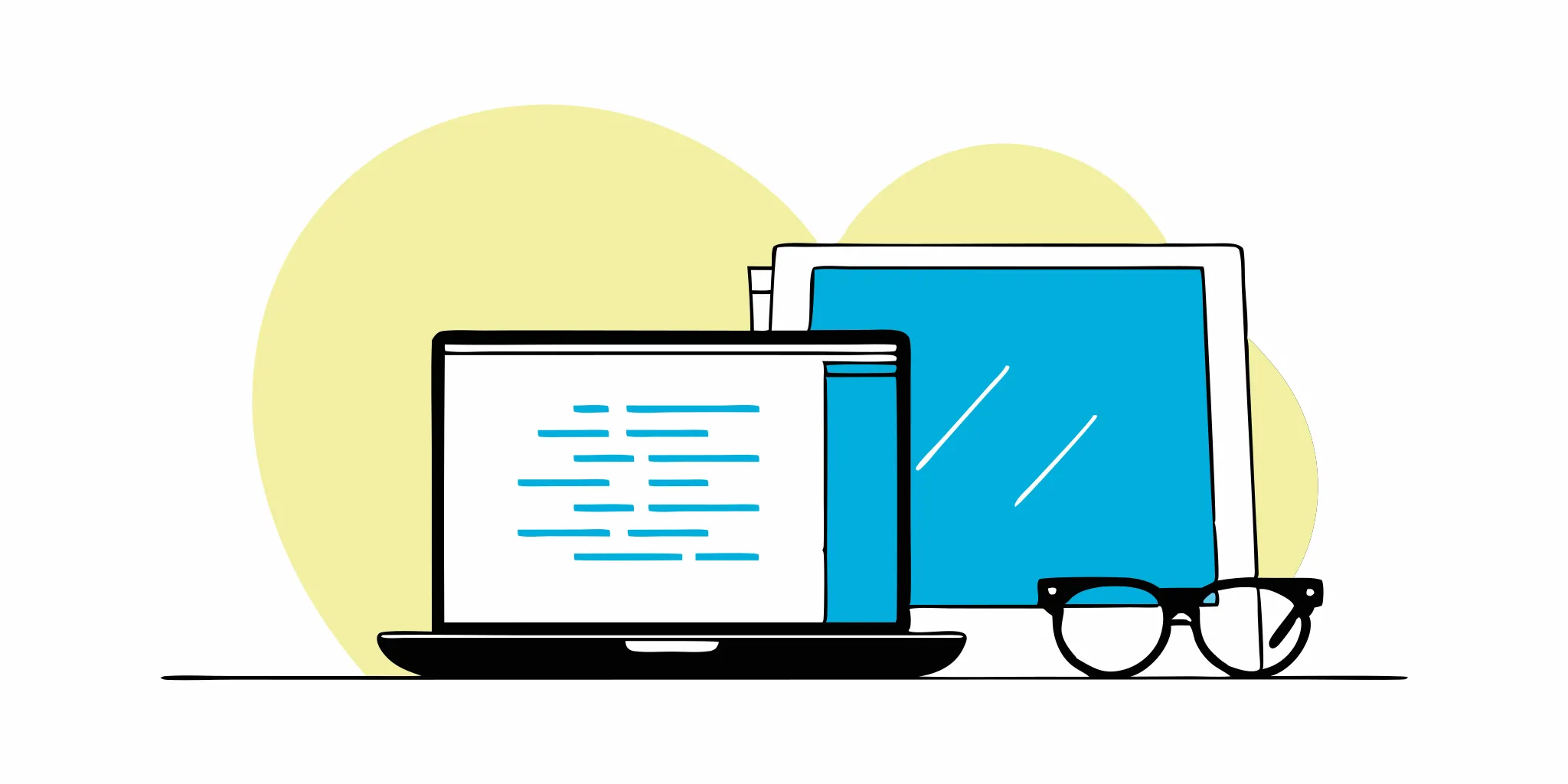
Table of Contents
Ever feel like you're building a house of cards with your code, just waiting for it to collapse? That's where unit testing comes in. If you're a software developer, tester, or QA professional, understanding what is unit test is crucial. It's like having a safety net for your code, catching errors before they become major headaches. In this guide, we'll break down the essentials of unit testing, from the basics to advanced techniques, and show you how it can transform your development process. We'll explore why it's so important, how to write effective tests, and which tools can make your life easier. Ready to build a more robust and reliable codebase? Let's get started.
Key Takeaways
- Unit testing builds better software: Testing individual components in isolation improves code quality, reduces debugging time, and simplifies future maintenance.
- A strategic approach to unit testing is key: Write focused tests with clear names and automate them within your CI/CD pipeline for faster development. Test-driven development (TDD) helps design testable, maintainable code.
- Unit testing creates a culture of quality: It improves team collaboration, reduces bug-fixing costs, and promotes a proactive approach to software quality, resulting in more reliable applications.
What is Unit Testing?
Unit testing is a software testing method where individual units or components of a software application are tested in isolation. Think of it like testing individual LEGO bricks before assembling a complex structure. Each brick needs to be sturdy and fit perfectly for the final creation to be stable. Similarly, each unit of code must work flawlessly on its own for the entire software system to perform as expected. This process helps identify and fix bugs early in the development, saving time and resources.
Definition and Purpose
Unit testing focuses on verifying the smallest testable parts of an application, such as individual functions, methods, or classes, independently. The primary goal is to validate that each unit performs its intended function correctly. By isolating these units, developers can pinpoint errors, making debugging much easier. This approach also prevents cascading failures where a bug in one unit affects other parts of the system. This method ensures that each piece of the software puzzle works correctly before it's integrated with others.
Key Components
Unit tests are typically written by the same developers who write the code. They define what the code should do under various conditions and then check if it behaves as expected. A well-written unit test includes assertions that compare the actual output of the code with the expected output. These tests should be isolated and independent, meaning they shouldn't rely on external factors like databases or networks. This isolation ensures that the test results are consistent and reliable. A comprehensive suite of unit tests significantly improves the quality and reliability of software, giving developers confidence in their application's individual building blocks.
Understand the Unit Testing Process
This section breaks down the unit testing process into two key phases: writing effective test cases and executing those tests. Understanding these steps is crucial for a successful unit testing strategy.
Write Effective Test Cases
Think of unit tests as mini-specifications for your code. They're typically written by the same programmers who develop the code, clarifying what each piece should do. This often involves a "test-function-expected value" approach: provide a specific input to a function, predict the output, and then compare that prediction to the actual result.
Each test should focus on a single aspect of the code's behavior. This isolation makes it much easier to pinpoint the source of a problem if a test fails. Instead of sifting through a complex test that covers multiple scenarios, you can quickly identify the specific function or behavior that isn't working correctly. These tests also serve as living documentation, illustrating how different parts of your code function.
Execute Tests
After writing your test cases, the next step is running them. Automating this process is key, especially in a fast-paced development environment. Tools can automatically execute your tests every time you modify your code, providing immediate feedback and catching regressions early.
Test doubles (like mock objects) are valuable tools during test execution. They simulate the behavior of dependent components, allowing you to isolate the unit under test and focus on its specific functionality. This practice simplifies testing and makes it easier to identify the root cause of any issues. Automated tests, combined with the use of test doubles, save significant time and resources compared to manual testing. This efficiency is particularly beneficial in a DevOps environment, where continuous integration and delivery are paramount.
Why Unit Testing Matters
Unit testing is more than just a checkbox in the software development lifecycle. It's a fundamental practice that significantly impacts the quality, reliability, and maintainability of your code. Let's explore why unit testing should be an integral part of your development workflow.
Find Bugs Early and Improve Code Quality
Think of unit tests as your first line of defense against bugs. By verifying the behavior of individual components in isolation, you can identify and fix defects early in the development process, before they become larger, more complex issues. Catching these issues early also leads to cleaner, more modular code. Writing unit tests often encourages better design practices and can even serve as a form of documentation, clarifying how different parts of your code should work. This improved code quality makes your project easier to understand, maintain, and extend.
Save Time and Resources
While writing unit tests might seem like extra work upfront, it saves you significant time and resources down the line. Unit testing reduces development and maintenance time by catching defects early and minimizing the need for extensive manual testing. Finding and fixing a bug in the early stages is much cheaper than addressing it after deployment. This cost-effectiveness is a major advantage of incorporating unit testing into your process. By investing in unit tests, you're investing in the long-term health and success of your project.
Best Practices for Unit Testing
Solid unit tests are the foundation of a reliable codebase. Here are a few best practices to make your unit testing efforts more effective:
Isolate and Keep Tests Independent
Think of unit tests like individual experiments. Each test should examine a small, isolated part of your code—a single function or method—independently of others. This isolation ensures that if a test fails, you can pinpoint the source of the problem quickly. It also prevents cascading failures where one failing test causes others to fail, obscuring the real issue. This practice of isolating tests makes debugging much more straightforward.
Use Clear Naming Conventions
Descriptive test names are crucial for maintaining a readable and understandable test suite. A well-named test clearly communicates its purpose. For example, instead of test1()
, use a name like test_calculate_total_with_valid_input()
. This practice makes your tests easier to understand and helps when tests fail. A clear name immediately tells you what functionality isn't working as expected. For more context on unit testing frameworks and best practices.
Apply Test-Driven Development (TDD)
Test-driven development (TDD) flips the traditional development script. Instead of writing code first and tests later, you write the unit test before the code it's designed to test. This approach forces you to think deeply about the desired behavior of your code and define clear expectations upfront. TDD can lead to more modular and maintainable code because it encourages you to design with testability in mind. For a good overview of TDD and its benefits. MuukTest seamlessly integrates with TDD workflows, allowing you to automate your testing process from the start. Learn more about how MuukTest can support your TDD implementation.
Unit Testing Tools and Frameworks
Unit testing involves several tools and frameworks that help streamline the process. Choosing the right ones depends on factors like your project's programming language, team preferences, and integration needs. Let's explore some popular options and how to select the best fit for your project.
Explore Popular Frameworks
Several unit testing frameworks cater to different programming languages and project requirements. Popular choices include JUnit and TestNG for Java, Jest and Jasmine for JavaScript, and pytest for Python. These frameworks offer features like test runners, assertion libraries, and reporting tools to make writing and running unit tests more efficient. Lightweight frameworks like Mocha, Jasmine, and QUnit are especially well-suited for rapid application development, allowing quick implementation and execution of tests in fast-paced environments. Understanding the strengths of each framework helps you choose the one that best suits your workflow. A robust framework provides insights into the testing codebase, enabling developers to make code changes quickly and easily.
Choose the Right Tool
Selecting the right unit testing tool is crucial for a smooth development process. Consider a tool compatible with your project's programming language and existing frameworks. Seamless integration with your development workflow is key to avoid disruptions and ensure efficient testing. Look for essential features like ease of use, support for various languages, integration capabilities, and robust reporting. These features contribute to the effectiveness of your unit testing efforts. A strong community backing is another important factor. Active communities offer quick fixes and updates, ensuring long-term maintenance and support for your chosen framework. By carefully evaluating these aspects, you can choose a unit testing tool that best suits your project's needs and contributes to higher quality code.
Overcome Unit Testing Challenges
Unit testing, like any process, has its challenges. But understanding these hurdles is the first step to overcoming them. Let's break down some common roadblocks and how to address them.
Manage Time Constraints
It's true; writing unit tests takes time. This can feel especially tricky when you're working with tight deadlines. It might seem easier to skip testing and focus on churning out code. However, think of unit testing as preventative medicine. By catching bugs early in the development cycle, you'll significantly reduce the time spent on debugging later. Integrating unit testing into your workflow can actually save you time and headaches down the road. Think of it as an investment that pays off in the long run. Services like MuukTest can help streamline this process and achieve comprehensive test coverage quickly, freeing up your team to focus on development. See how we can help you save time on our QuickStart page.
Handle Legacy Code
Integrating unit tests into existing legacy code can be a bit like untangling a giant ball of yarn. It can be complex and time-consuming, especially if the code wasn't written with testability in mind. Often, this involves refactoring the code—restructuring it to make it more testable. While this might require an initial investment of resources, it ultimately improves the maintainability and quality of your codebase. If you're facing this challenge, consider prioritizing the most critical parts of your legacy code for testing. You can also explore tools and techniques that assist with refactoring and make the process more manageable. MuukTest specializes in working with complex systems and can help you implement a robust testing strategy, even with legacy code. Explore our test automation services to learn more.
Fix Flaky Tests
Flaky tests—those that pass sometimes and fail others without any code changes—can be a real thorn in the side of any development team. They erode confidence in the testing process and can make it difficult to pinpoint actual bugs. Often, the root cause of flaky tests isn't a technical issue but rather lies in the testing environment or test dependencies. To address flaky tests, start by isolating the problematic tests and carefully examining their dependencies. Ensure your tests are independent and don't rely on specific execution order or external factors. Building a culture that values testing and encourages developers to take ownership of their tests is also crucial. MuukTest prioritizes test reliability and offers solutions to minimize flaky tests, ensuring your team has confidence in the results. Learn how we help our customers achieve reliable testing by visiting our Customers page.
Unit Testing vs. Other Testing Methods
Compare with Integration and Functional Testing
Unit testing is essential for verifying the smallest parts of your code work correctly. But other testing methods also play a critical role in software development. Let's look at how unit testing compares to integration and functional testing.
Think of your application as a car. Unit testing examines individual parts like the engine, brakes, and transmission in isolation. It ensures each part functions correctly on its own, which is crucial for catching bugs early in development.
Integration testing, on the other hand, checks how these parts work together. It verifies that the engine interacts correctly with the transmission, and the brakes respond as expected when you press the pedal. Integration testing ensures that the individual units, which work perfectly in isolation, also function seamlessly when combined. This helps identify issues that might arise from the interaction of different components, something unit testing alone can't address.
Finally, functional testing assesses the overall functionality of the car. Does it drive smoothly? Does the air conditioning work? Functional testing evaluates the system as a whole from the user's perspective. It ensures all the parts work together to deliver the expected experience.
In essence, each testing method serves a distinct purpose. Unit testing focuses on individual components, integration testing on their interactions, and functional testing on the overall user experience. By combining these methods, you create a comprehensive testing strategy that leads to more robust and reliable software.
Implement Unit Testing in Your Projects
Get Started Step-by-Step
Unit testing might seem intimidating at first, but it's easier than you think. Start by breaking down your code into smaller, testable units—think functions or methods. Before you write any code, consider test-driven development (TDD). This practice involves writing your unit tests before you write the actual code. It's a bit like creating a blueprint. TDD helps you clarify what your code needs to do and ensures it meets those requirements from the start. Next, pick a testing framework. Don't reinvent the wheel; use tools like pytest or unittest in Python. These frameworks provide helpers and structure to make writing and running tests much smoother. Finally, automate everything. Set up your tests to run automatically as part of your development process, either locally or on a continuous integration server. This catches errors early and often.
Integrate with Continuous Integration
Integrating unit testing with your continuous integration/continuous delivery (CI/CD) pipeline is crucial for catching regressions early. A solid suite of unit tests empowers developers to refactor code with confidence. Every time code is committed or merged, the tests automatically run, ensuring any issues are flagged before they reach production. This automation speeds up software delivery and improves software quality, helping you stay competitive. When choosing a CI/CD tool, ensure it's compatible with your project's language and frameworks and integrates seamlessly with your existing development workflow. Services like MuukTest can help you achieve complete test coverage efficiently, significantly enhancing test effectiveness. Automating your testing and deployment processes through a robust CI/CD pipeline is a game-changer for any development team.
The Impact of Unit Testing on Teams
Unit testing isn't just about catching bugs; it significantly impacts how teams work together and approach software development. It fosters better communication, improves code quality, and creates a more collaborative environment.
Foster Collaboration and Code Understanding
Think of unit tests as a practical form of documentation. They clearly demonstrate how different parts of the code should function, making it easier for other developers to understand the code's purpose and intended behavior. This shared understanding reduces confusion and makes collaboration smoother. When a new developer joins the team, or when someone revisits older code, unit tests provide valuable context and insights. This shared understanding is especially valuable in agile environments where teams work collaboratively and code changes frequently. Unit tests serve as living documentation, illustrating the expected behavior of individual code components.
Build a Quality-Focused Culture
Regular unit testing helps create a culture that prioritizes quality from the start. By catching bugs early in the development cycle teams can address issues before they become larger, more complex problems. This proactive approach improves the final product and reduces the time and resources spent on debugging later. Writing unit tests often encourages developers to write cleaner, more modular code. This practice improves code quality and fosters a culture of quality within the development team. When everyone is involved in ensuring code quality from the unit level up, it creates a sense of shared responsibility and a collective commitment to delivering a reliable and well-functioning product.
Frequently Asked Questions
If unit testing seems time-consuming, how can I fit it into a tight project schedule?
While writing unit tests requires an initial time investment, it ultimately saves time and resources. By catching bugs early, you avoid more extensive and costly debugging later. Think of it as preventative maintenance—a little effort upfront prevents major headaches down the road. Also, consider prioritizing testing for critical parts of your application first and gradually expanding your test suite. Tools like MuukTest can help automate and streamline the process, making it easier to integrate testing into even the busiest schedules.
How do I approach unit testing when dealing with a large, existing codebase (legacy code)?
Integrating unit tests into legacy code can be challenging. Start by identifying the most critical or frequently modified parts of the codebase. Focus your initial testing efforts there. Refactoring—restructuring existing code to make it more testable—is often necessary. While this takes time, it significantly improves the long-term maintainability and quality of your code. Consider seeking expert help or exploring tools that assist with refactoring and legacy code testing.
What are some common mistakes to avoid when writing unit tests?
One common mistake is writing tests that are too complex or cover too much functionality. Each test should focus on a single, isolated aspect of the code. Another pitfall is neglecting to test edge cases and boundary conditions. Make sure your tests cover not only the typical scenarios but also the unusual ones that might expose hidden bugs. Finally, avoid writing tests that are tightly coupled to the implementation details of your code. This makes your tests brittle and prone to breaking when the implementation changes.
What's the difference between unit testing, integration testing, and functional testing?
Unit testing verifies the behavior of individual components in isolation. Integration testing checks how these components interact with each other. Functional testing assesses the overall system behavior from the user's perspective. Think of it like building a car: unit tests check the engine, brakes, and transmission separately; integration tests ensure these parts work together; and functional tests confirm the car drives as expected.
How can I choose the right unit testing framework for my project?
Consider your project's programming language, the complexity of your application, and your team's familiarity with different frameworks. Research popular options and evaluate their features, ease of use, and community support. Some frameworks are better suited for specific languages or types of projects. Don't be afraid to experiment and choose the one that best fits your workflow and needs.
At vero eos et accusamus et iusto odio dignissimos ducimus qui blanditiis praesentium voluptatum deleniti atque corrupti quos dolores et quas molestias excepturi sint occaecati cupiditate non provident
Related Posts:
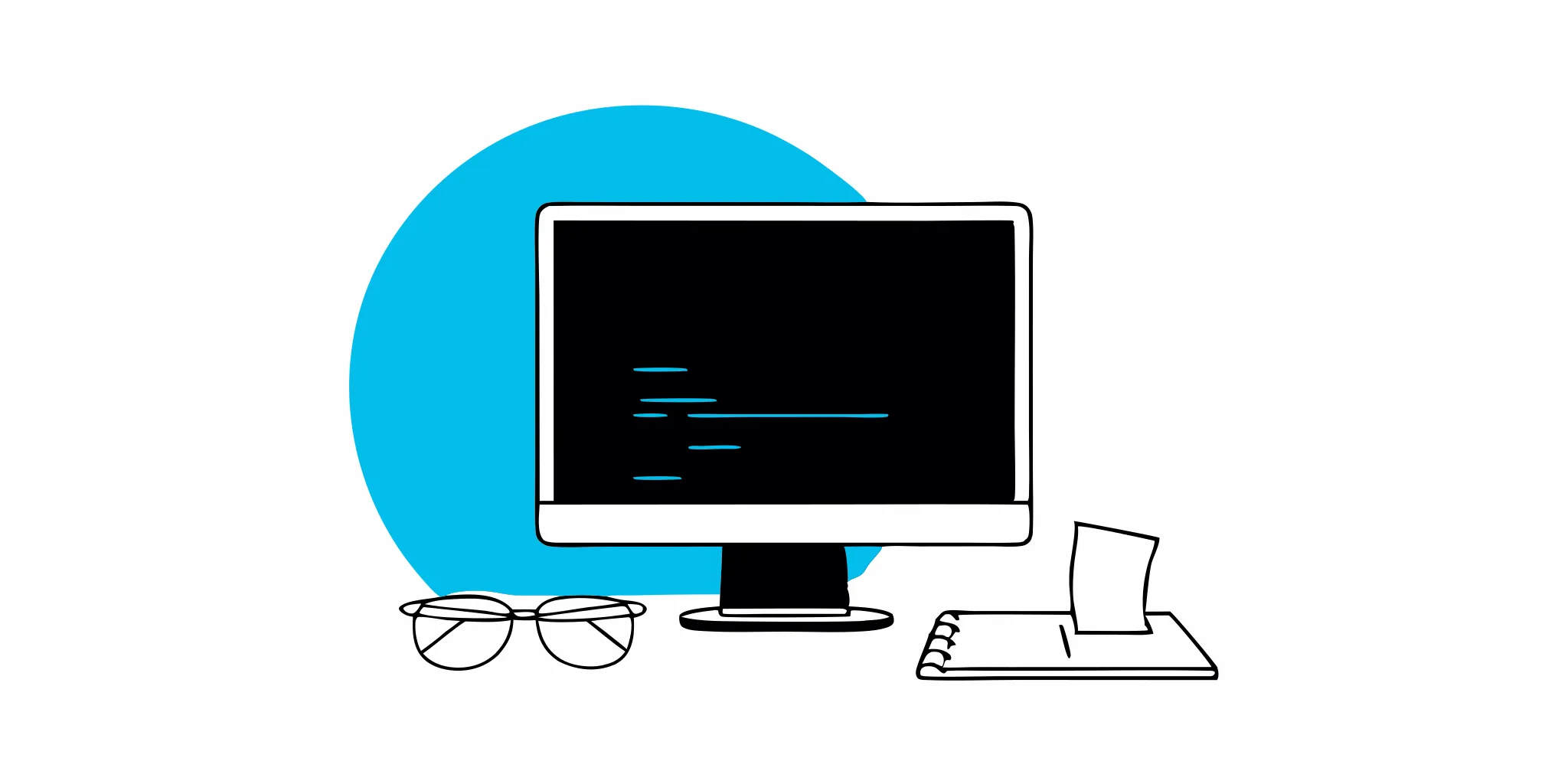
Software Module Testing: A Practical Guide
Building software is a lot like constructing a complex LEGO masterpiece. You wouldn't want to discover halfway through that some of your foundational bricks are faulty, would you? That's where...
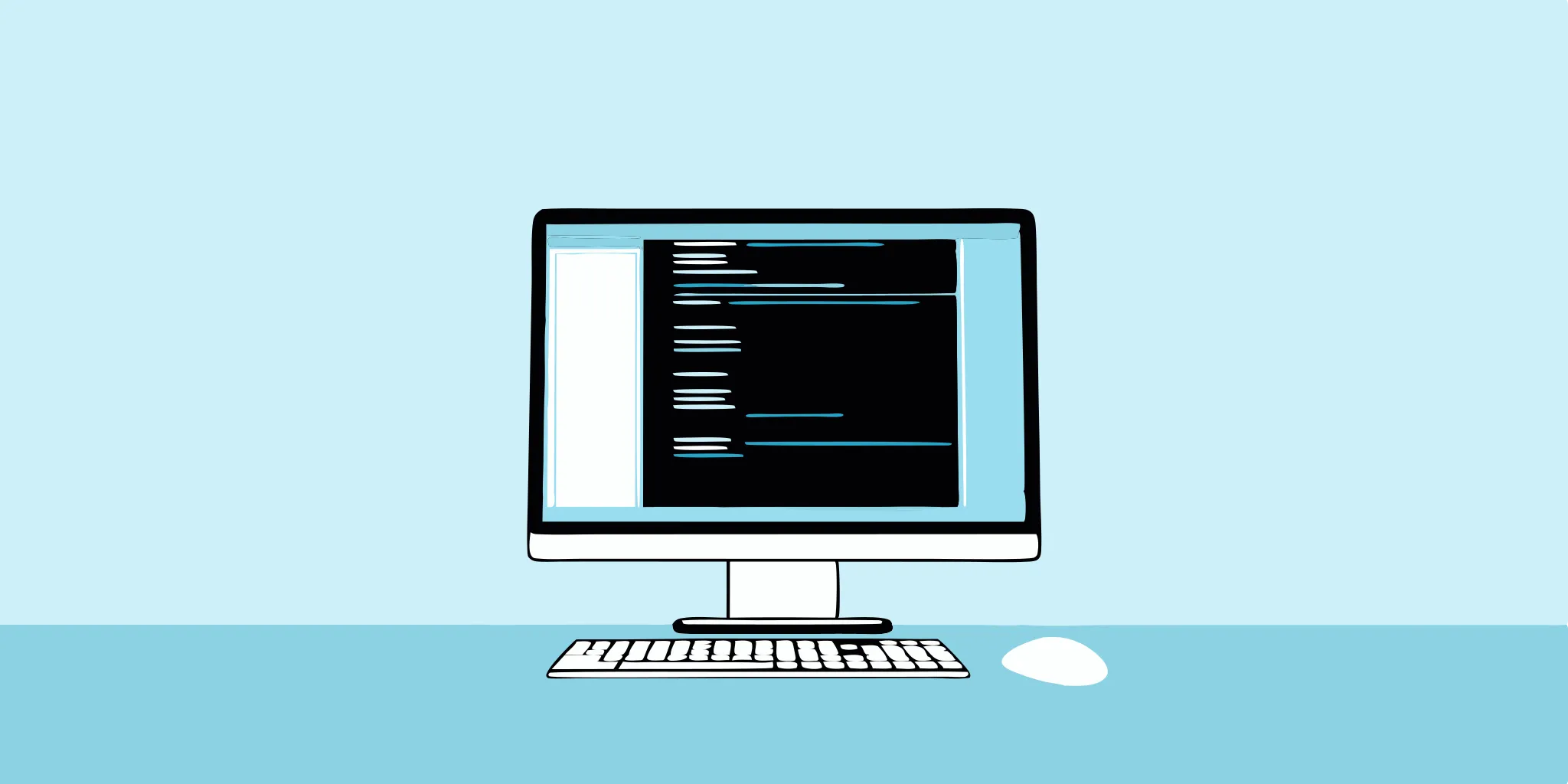
Module Testing in Software Testing: A Practical Guide
Writing software without module testing is like building a bridge without checking the strength of individual beams. Module testing in software testing is the quality assurance process that ensures...
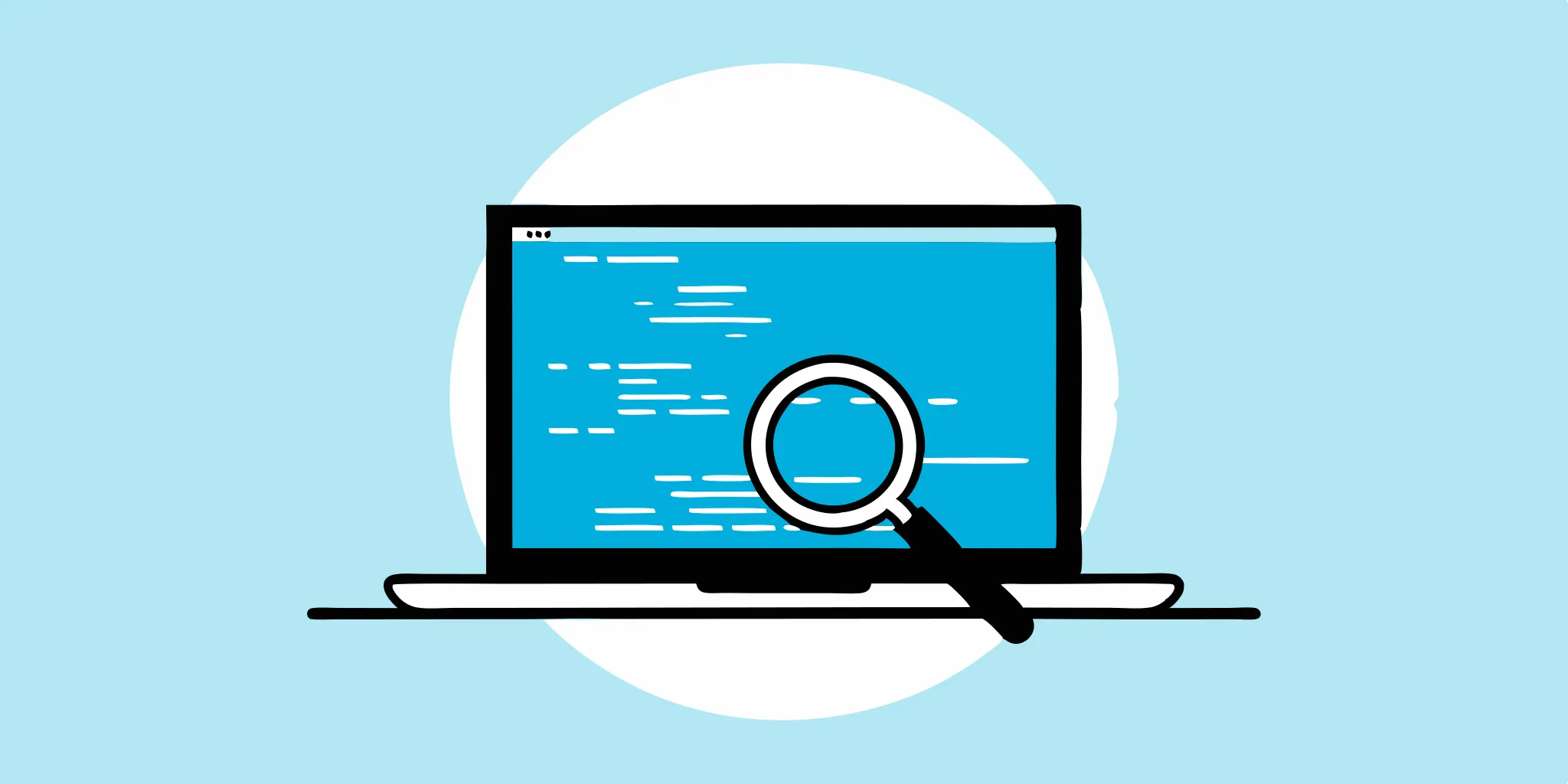
What is Unit Testing in Software Testing? A Practical Guide
Writing clean, reliable code is every developer's goal. But how do you ensure your code does what it's supposed to do, every single time? One powerful technique is unit testing. This method involves...