What is Unit Testing in Software Testing? A Practical Guide
Author: The MuukTest Team
Published: March 20, 2025
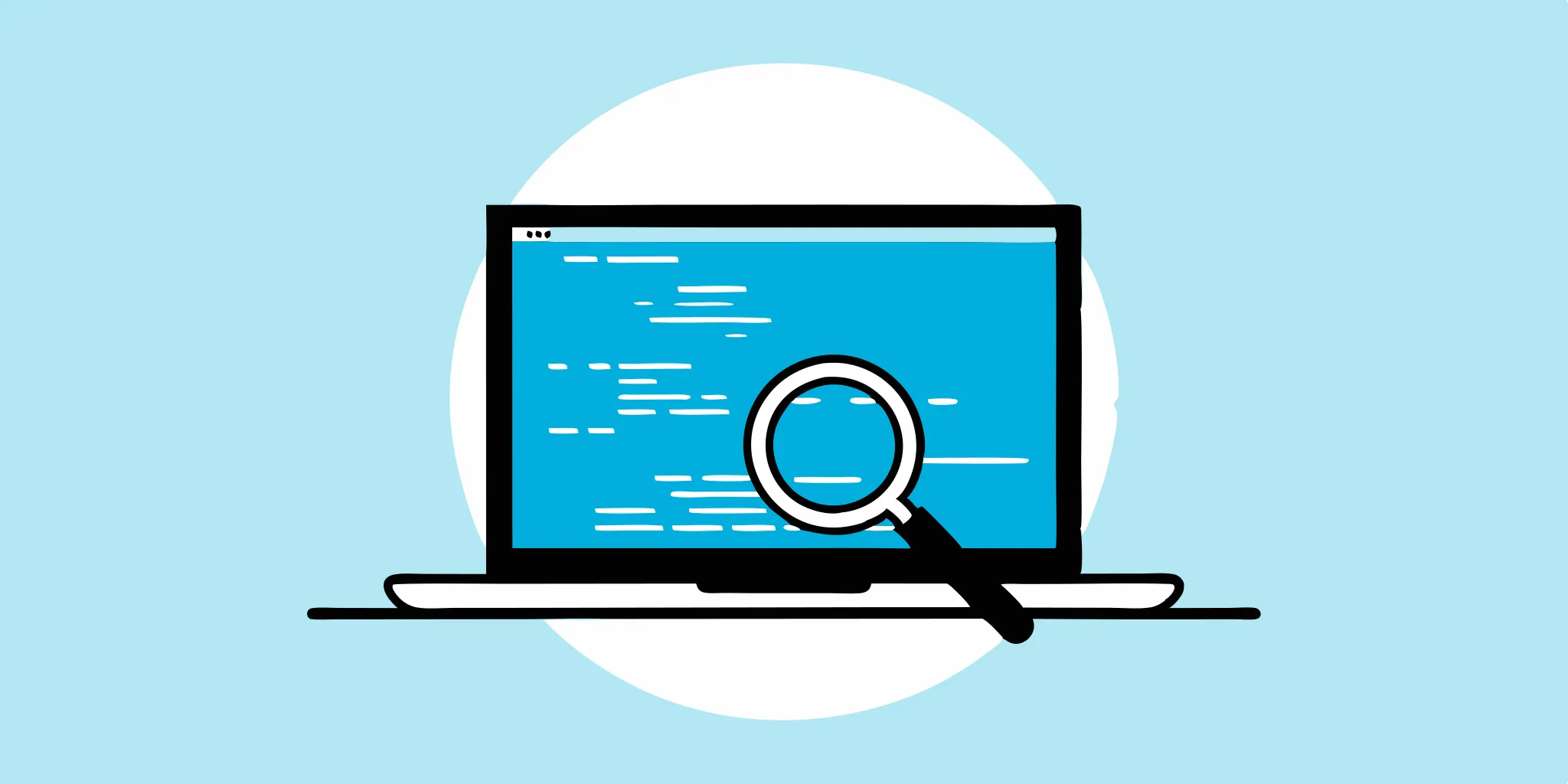
Table of Contents
Writing clean, reliable code is every developer's goal. But how do you ensure your code does what it's supposed to do, every single time? One powerful technique is unit testing. This method involves testing individual units of your code in isolation, ensuring each component functions correctly before it's integrated into the larger system. This guide will answer the question, what is unit testing in software testing, and why it's so important. We'll explore the benefits of unit testing, from catching bugs early to improving code design and simplifying maintenance. We'll also delve into the practical aspects, including common tools, frameworks, and best practices for writing effective unit tests. Whether you're a seasoned pro or new to the game, this guide will equip you with the knowledge to write better, more reliable code.
Key Takeaways
- Unit testing improves code quality and reduces costs: By catching bugs early, unit testing minimizes the expense and effort of fixing them later. It also encourages better code design, resulting in more maintainable and reusable components.
- Effective unit tests are isolated, repeatable, and well-named: Focus on testing individual units of code in isolation from external dependencies. Ensure your tests can be run consistently and use descriptive names to clarify their purpose.
- Make unit testing a habit: Integrate unit testing into your development workflow, whether through Continuous Integration or Test-Driven Development. This consistent practice builds a strong foundation for reliable and maintainable software.
What is Unit Testing?
Unit testing is a software testing method where individual units or components of code are tested in isolation to confirm they work as expected. Think of it like testing individual Lego bricks before assembling a complex structure. Each brick needs to be sound before you can rely on the whole creation. Similarly, each function, and method helps catch defects early in the development cycle, making them much easier and cheaper to fix.
Imagine trying to find a faulty Lego brick in a completed castle—it's a lot harder than checking each brick beforehand. The same principle applies to software. Finding and fixing bugs in a large, integrated system is far more complex and time-consuming than addressing them at the unit level. Unit testing allows developers to isolate potential problems and correct them before they become larger issues. Effective unit testing involves designing specific test cases that exercise different aspects of a unit's functionality. These tests verify that the unit produces the correct output for a given input and handles unusual scenarios gracefully.
By systematically testing each unit, developers can build confidence in the reliability and stability of their codebase. This granular approach improves the overall quality of the software and simplifies future maintenance and updates. When a change is made, unit tests can quickly identify any unintended consequences, preventing regressions and ensuring that the code continues to function as intended.
Why Unit Testing Matters
Unit testing offers several compelling advantages for software development. It improves code quality, reduces costs, and makes maintenance easier. Let's explore some key benefits:
Catch Bugs Early
Think of your code as a complex machine with many moving parts. Unit testing is like checking each part individually before assembling the whole thing. This helps you identify defects early in the development process, when they are much easier and less expensive to fix. Finding bugs early is a core benefit of unit testing, allowing you to address issues before they escalate. Imagine building a house – it's much simpler to correct a foundation problem before you've built the entire structure. The same principle applies to code. Early bug detection through unit testing saves you time, money, and headaches down the line.
Improve Code Quality
Unit testing not only helps find bugs but also encourages better code design. When you write unit tests, you're forced to think about how your code will be used and how different parts interact. This leads to more modular, reusable, and maintainable code. Plus, well-written unit tests serve as excellent documentation, making it easier for other developers (or even your future self) to understand and work with the codebase. This focus on individual components often reveals design flaws that might otherwise go unnoticed.
Simplify Maintenance
Regular unit testing makes maintaining and updating your code significantly simpler. When you make changes, you can run your unit tests to quickly verify that everything still works as expected. This safety net gives you the confidence to refactor code, add new features, and address technical debt without fear of introducing regressions. Automated unit tests are a reliable way to ensure your code functions correctly, even after modifications. This reduces the risk of unexpected issues cropping up later, saving you time and effort in the long run. Discover the benefits of unit testing.
How Unit Testing Works
Unit testing verifies small, isolated parts of your code—like individual functions or modules—to ensure they work correctly in isolation. Think of it as testing individual Lego bricks before assembling a complex structure. This approach helps identify and fix problems early in the development cycle, saving you time and resources. At MuukTest, we specialize in helping you build robust and reliable software through comprehensive unit testing.
The Unit Testing Process
The unit testing process typically involves three main steps:
- Setup: Prepare the unit of code you want to test. This might involve creating objects, initializing variables, or establishing database connections.
- Action: Provide input to the unit and execute the function or method you're testing. This step simulates how the unit would be used in real-world scenarios.
- Assertion: Verify the output of the unit against your expected results. This step confirms whether the unit behaves as intended. For a quick start, check out our QuickStart guide.
Common Tools and Frameworks
Several tools and frameworks can streamline your unit testing efforts. Some popular options include:
- JUnit: A widely used framework for Java development. JUnit provides annotations and assertions for writing and running tests.
- NUnit: Similar to JUnit, NUnit is a unit testing framework for .NET languages like C#.
- TestNG: Another popular Java framework. TestNG offers advanced features like parallel test execution and data-driven testing.
- Jasmine: A behavior-driven development (BDD) framework for JavaScript. Jasmine helps you write tests that describe how your code should behave.
- Mocha: A flexible JavaScript testing framework that runs on Node.js and in the browser. Mocha supports various assertion libraries and reporters.
- Mockito: A mocking framework for Java. Mockito allows you to create mock objects for testing dependencies and isolating units of code. Explore our customer success stories to see how MuukTest has helped businesses achieve comprehensive test coverage. Review our pricing plans for more information.
Key Components of Unit Testing
Unit testing relies on a few key components working together. Understanding these elements helps you write effective tests that thoroughly evaluate your code.
Test Cases and Suites
Think of a test case as a single, specific scenario you want to check. It verifies a small piece of functionality in your code, like whether a function returns the expected output given a certain input. You'll create many test cases, each testing different aspects of your code's behavior. These individual tests are then grouped into test suites. Test suites help organize your tests, making them easier to run and manage, especially as your project grows. Imagine them as containers holding related test cases, like all the tests related to a specific class or module. This organization makes it simpler to target specific areas of your code during testing. Smaller tests offer more granular feedback and run faster, sometimes thousands per second.
Assertions and Mocking
Assertions are the heart of your test cases. They're like checkpoints that verify whether the actual outcome of your code matches what you expect. For example, if your function is supposed to add two numbers, an assertion would check that the result is indeed the sum of those numbers. If the assertion fails, it means something isn't working as expected. Mocking comes into play when your code interacts with external dependencies, like databases or APIs. Directly interacting with these dependencies during unit testing can be slow and complex. Mocking allows you to simulate these dependencies, giving you more control over the testing environment. Mocking tools isolate your unit of work by removing these outside dependencies, so you can focus on testing the internal logic of your code in isolation.
Best Practices for Effective Unit Testing
Effective unit testing requires a thoughtful approach. Here are some best practices to ensure your unit tests are valuable and maintainable:
Write Isolated and Repeatable Tests
Your unit tests should be self-contained and independent. They shouldn't rely on databases, networks, or other external systems. This isolation ensures that tests can be run quickly and consistently in any environment, without external factors influencing the results. Think of each test as a small, controlled experiment—set up the necessary conditions, run the test, and verify the outcome, all within the confines of the test itself. This practice makes debugging much easier, as you can pinpoint the source of errors more quickly.
Use Descriptive Test Names
Clarity is key when writing unit tests. Each test should verify one specific aspect of your code. Use descriptive names that clearly explain the test's purpose. This practice not only improves readability but also makes it easier to identify failing tests and understand what functionality is broken. A well-named test acts as documentation, making it easier for you and other developers to understand the intent and scope of the test.
Maintain Test Cases
Unit tests serve as living documentation, illustrating how different parts of your code are intended to work. However, writing and maintaining these tests requires ongoing effort. As your codebase evolves, so too should your tests. Regularly review and update your test cases to ensure they accurately reflect the current state of your code and continue to provide value. Treat your tests with the same care as your production code, refactoring and improving them as needed. This ongoing maintenance ensures your tests remain a reliable safety net for your code. This ongoing maintenance ensures your tests remain a reliable safety net, catching regressions and preventing unexpected behavior.
Common Unit Testing Challenges
While unit testing offers significant advantages, it also presents some hurdles that development teams often face. Understanding these challenges is the first step toward addressing them effectively and reaping the full benefits of unit testing.
Time Constraints and Complex Dependencies
One of the most common challenges in unit testing is the time investment required. Writing comprehensive unit tests takes time and effort, which can be a significant constraint for projects with tight deadlines. Developers often feel pressure to deliver features quickly, which can lead to skimping on testing. This pressure is amplified when dealing with complex codebases with intricate dependencies. Testing individual units within such systems can become complicated and time-consuming, as isolating units for testing requires careful planning and execution. The overhead of writing and maintaining tests for highly complex code can be substantial. For instance, consider a scenario where a single unit interacts with multiple external services or databases. Creating isolated test environments for such units can be a considerable undertaking.
Balance Coverage and Efficiency
Another challenge lies in finding the right balance between test coverage and efficiency. While aiming for 100% test coverage might seem ideal, it's not always practical or efficient. Striking a balance involves prioritizing the most critical parts of your codebase and ensuring those areas have thorough tests. This requires careful consideration of the potential risks and impact of failures in different parts of the system. A team might decide to focus on testing core business logic more extensively than utility functions, for example. Moreover, fostering a culture of testing within the team, perhaps with a dedicated "champion" to promote best practices, can significantly improve the overall effectiveness of unit testing efforts. This cultural shift can help teams view testing as an integral part of the development process, rather than an afterthought.
Unit Testing vs. Other Testing Methods
While unit testing is essential, it's not the only testing method you need for robust software development. Understanding how unit testing fits within the broader testing landscape is crucial for building high-quality software. Let's look at how it compares to other common methods.
Integration and System Testing
Unit testing focuses on individual components in isolation. Think of it like testing the engine of a car before it's installed. It ensures each part works correctly on its own. Integration testing, on the other hand, checks how these individual units work together. This is like making sure the engine, transmission, and wheels all interact smoothly. It often follows unit testing and helps identify issues that might arise from the interaction of different components. System testing takes a broader view, evaluating the entire system as a whole. This is like taking the car for a test drive, ensuring all the parts work together to deliver the intended experience.
Acceptance Testing
Acceptance testing is the final stage before deployment. It verifies the software meets the specified business requirements and is ready for users. Think of it as a final inspection before the car leaves the factory. While unit testing ensures individual components function correctly, acceptance testing confirms the entire system delivers the intended value to the end-user. Acceptance testing typically follows integration testing and focuses on validating the end-to-end business flow. By ensuring the code works as expected, unit tests indirectly support acceptance testing, laying the groundwork for a functional and reliable system.
Integrate Unit Testing into Your Workflow
Integrating unit testing seamlessly into your development workflow is key to realizing its full benefits. Two popular methods that champion this integration are Continuous Integration and Test-Driven Development. Let's explore how these practices can elevate your testing game.
Continuous Integration
Continuous Integration (CI) is a development practice where developers frequently integrate code changes into a shared repository, often multiple times a day. Each integration triggers an automated build process, including running unit tests, to catch integration errors quickly. Think of CI as a safety net, catching issues before they become major headaches. This automation ensures that tests run consistently, providing rapid feedback on the impact of code changes. This consistent execution is crucial for maintaining code quality and preventing regressions as the project grows.
Test-Driven Development (TDD)
Test-Driven Development (TDD) flips the traditional development script. Instead of writing code first and then creating tests, TDD advocates writing tests before the code. This approach forces you to think critically about the desired behavior of the code and define clear expectations upfront. It's like creating a blueprint before building a house. You start by writing a failing test that defines a specific feature. Then, you write just enough code to pass that test. Finally, you refactor the code to improve its design, all while ensuring the tests still pass. This "red-green-refactor" cycle ensures thoroughly tested code that meets requirements. TDD might feel unusual at first, but many find it leads to more robust and maintainable code. It also encourages simpler, more modular design, making your codebase easier to understand and change. For a quicker understanding of how to get started with TDD and integrate it into your workflow, check out MuukTest's QuickStart guide.
The Future of Unit Testing
Unit testing is constantly evolving, and two key trends are shaping its future: AI-powered solutions and automated test generation. These advancements promise to make unit testing even more efficient, effective, and accessible.
AI-Powered Testing Solutions
AI is transforming software testing by automating complex tasks and improving overall efficiency. AI-powered tools can analyze code, predict potential bugs, and even generate test cases automatically. This not only speeds up the testing process but also helps improve test coverage and accuracy. Services like MuukTest are at the forefront of this transformation, using AI to achieve comprehensive test coverage within 90 days. AI continually refines its capabilities with each test cycle, using machine learning to enhance effectiveness. Automation tools capitalize on these advancements by streamlining the identification and testing of critical components. AI is also revolutionizing API testing by automating complex tasks and enhancing overall testing efficiency, making it essential in modern software development. These AI-driven approaches greatly improve test coverage, efficiency, and accuracy.
Automated Test Generation
Automated test generation is another exciting development in the future of unit testing. Tools are emerging that can automatically generate test cases based on code analysis and predefined rules. This reduces the manual effort required for test creation and allows developers to focus on other critical tasks. By harnessing machine learning algorithms, these tools enhance test coverage, reduce human intervention, and accelerate the testing process. This shift towards automation allows teams to achieve more comprehensive testing with fewer resources. For companies looking to quickly scale their testing efforts, automated test generation offers a powerful solution. If you're interested in exploring how automated testing can benefit your team, check out MuukTest's QuickStart guide.
Get Started with Unit Testing
Ready to dive into unit testing? It's easier than you think. Here’s a practical guide to get you started:
-
Grasp the Fundamentals: Unit testing boils down to checking small parts of your code—like individual functions or modules—to ensure they work correctly in isolation. Think of it like testing individual Lego bricks before assembling a complex structure. This approach helps identify defects early in the development process, making them simpler and less expensive to fix.
-
Pick the Right Tools: A unit testing framework provides a structured way to write and run your tests. Popular frameworks, like pytest and unittest in Python, automate the testing process and offer helpful features like reporting and test discovery. Keep your tests focused—ideally, each test should make only one assertion to pinpoint issues precisely. Start testing early in your project to prevent bugs from accumulating.
-
Write Tests First (or After, But Test!): Many developers advocate for writing tests before writing the code itself. This test-driven development (TDD) approach helps clarify requirements and makes refactoring smoother. If TDD doesn't fit your workflow, you can still write tests after coding to verify functionality. Make testing a consistent part of your development cycle.
-
Follow a Simple Process: A typical unit test involves three straightforward steps: setup (prepare the component for testing), action (provide input to the component), and assertion (check if the output matches your expectations). This clear structure ensures your tests are organized and easy to understan.
By following these steps, you can seamlessly integrate unit testing into your development process, resulting in more robust and maintainable code. Explore MuukTest's AI-powered test automation services for a comprehensive solution to streamline your testing and achieve complete test coverage efficiently. Get started with our QuickStart guide today.
Frequently Asked Questions
How much time should I dedicate to unit testing?
While it might seem like an added task, unit testing ultimately saves you time. Aim to integrate it into your regular development process. The initial investment in writing tests pays off by catching bugs early, reducing debugging time later. Think of it as preventative maintenance—a little effort upfront saves major headaches down the road.
What's the difference between unit testing, integration testing, and system testing?
Unit testing focuses on individual components in isolation, like testing a single Lego brick. Integration testing checks how these individual units work together, ensuring the bricks connect properly. System testing evaluates the entire assembled structure, making sure the final Lego creation is stable and functions as intended.
Is 100% test coverage always necessary?
While comprehensive testing is important, 100% coverage isn't always practical or the most efficient use of your time. Prioritize testing critical parts of your codebase, focusing on areas with the highest risk or complexity. A balanced approach ensures you're thoroughly testing the most important parts of your system without getting bogged down in less crucial areas.
What if my code interacts with external systems like databases or APIs?
When your code relies on external dependencies, use mocking. Mocking simulates these dependencies, allowing you to test your code in isolation without needing access to the actual external systems. This makes your tests faster, more reliable, and easier to manage.
How can I get started with unit testing quickly?
Choose a unit testing framework relevant to your programming language (like JUnit for Java or pytest for Python). Start by writing small, focused tests for individual functions or modules. Follow the setup, action, assertion pattern: prepare the component, provide input, and check the output against your expectations. There are plenty of online resources and tutorials available to guide you through the process.
Related Posts:
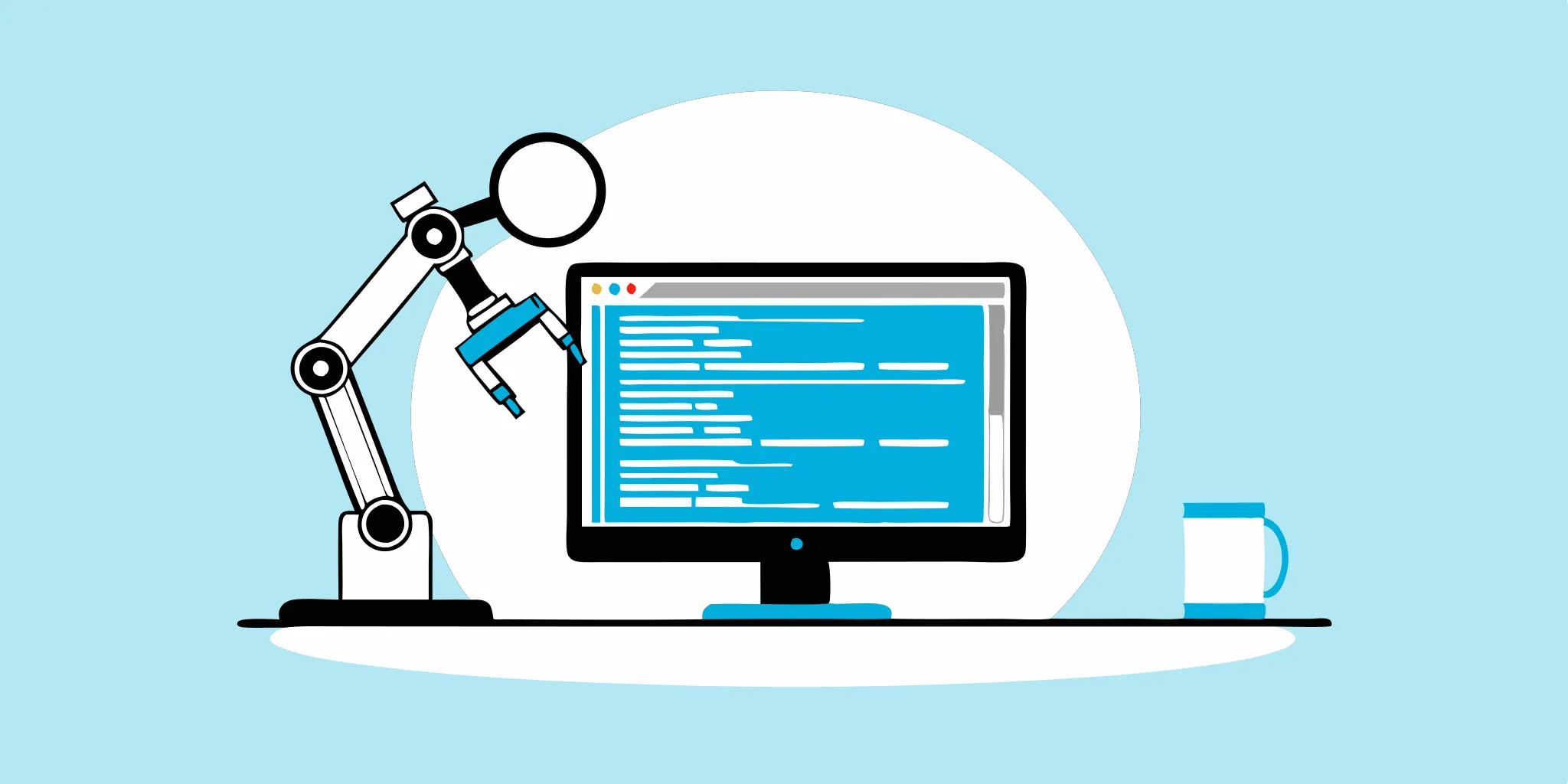
Software Testing Help: Your Guide to a Successful Career
Get comprehensive software testing help with this ultimate guide, covering essential methodologies, tools, and tips to enhance your testing skills.
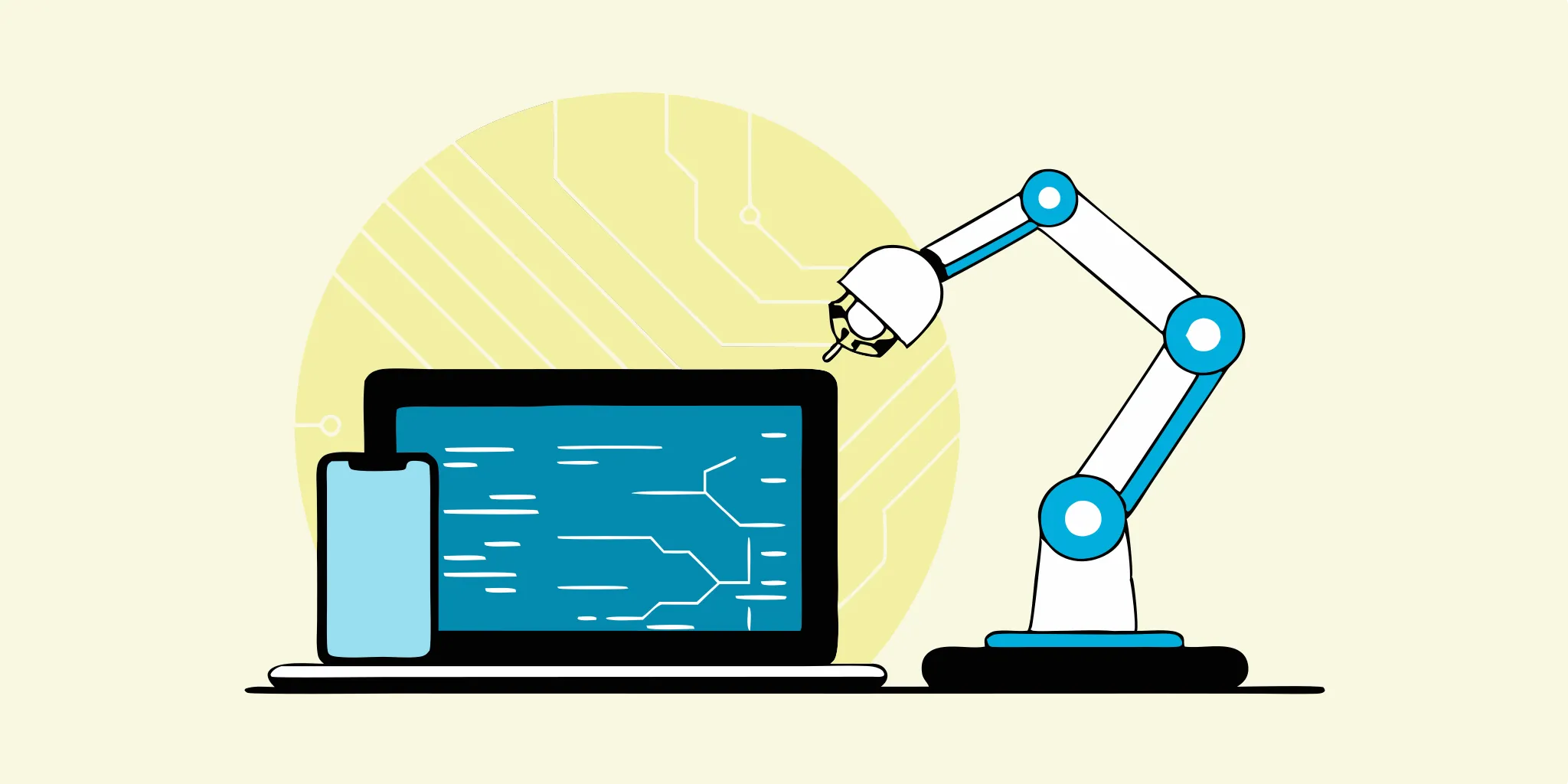
Test Automation Testing: Your Complete Guide
Repetitive tasks can drain your team's energy and time, especially in software development. Test automation offers a powerful solution by automating repetitive testing processes, freeing up your team...
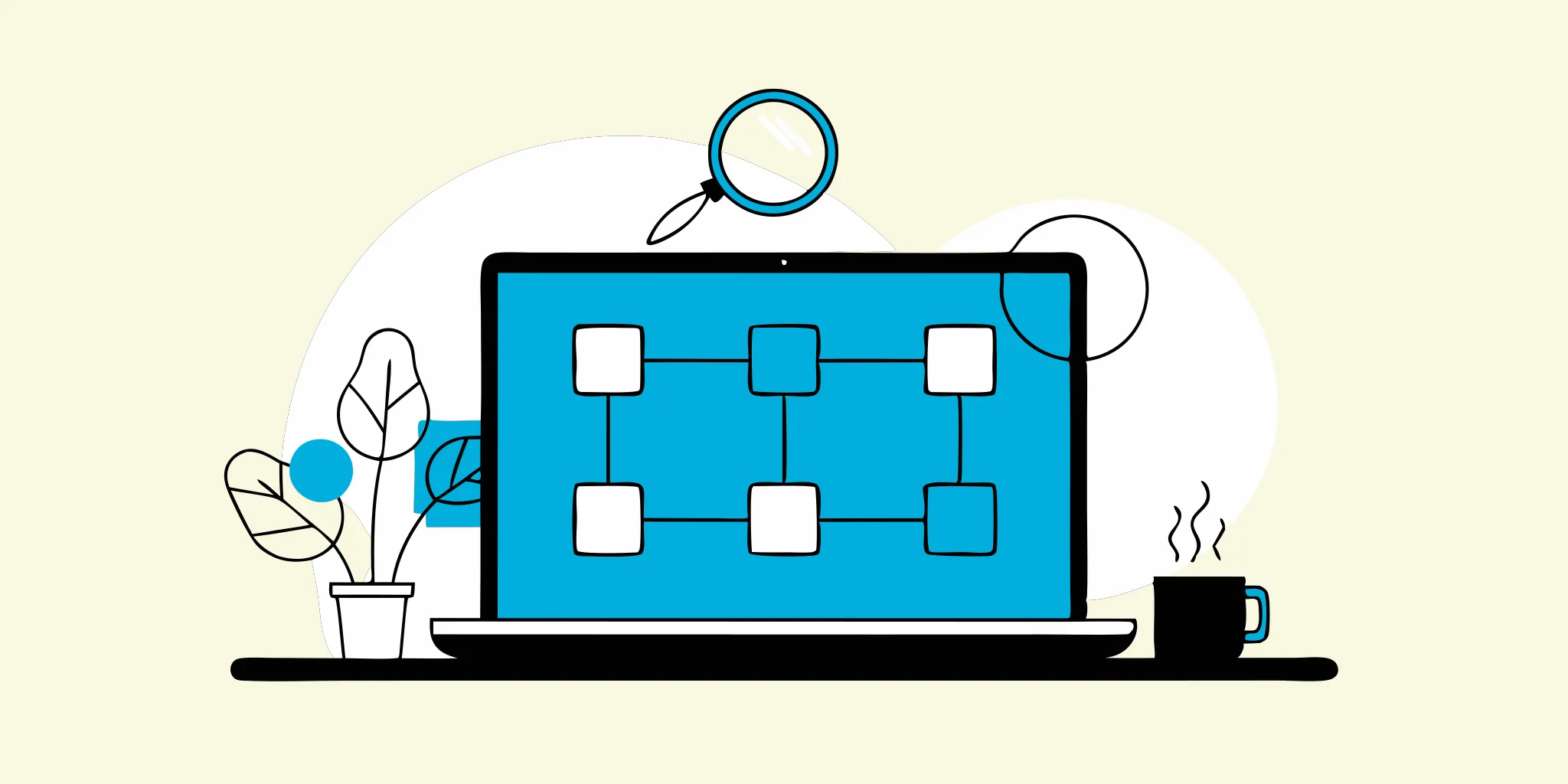
End-to-End Testing in Software Testing: A Practical Guide
Releasing software without thorough testing is like sending a ship out to sea without checking for leaks. You might make it to your destination, but the journey could be fraught with problems....