Unit Testing Automation: A Practical Guide
Author: The MuukTest Team
Published: December 17, 2024
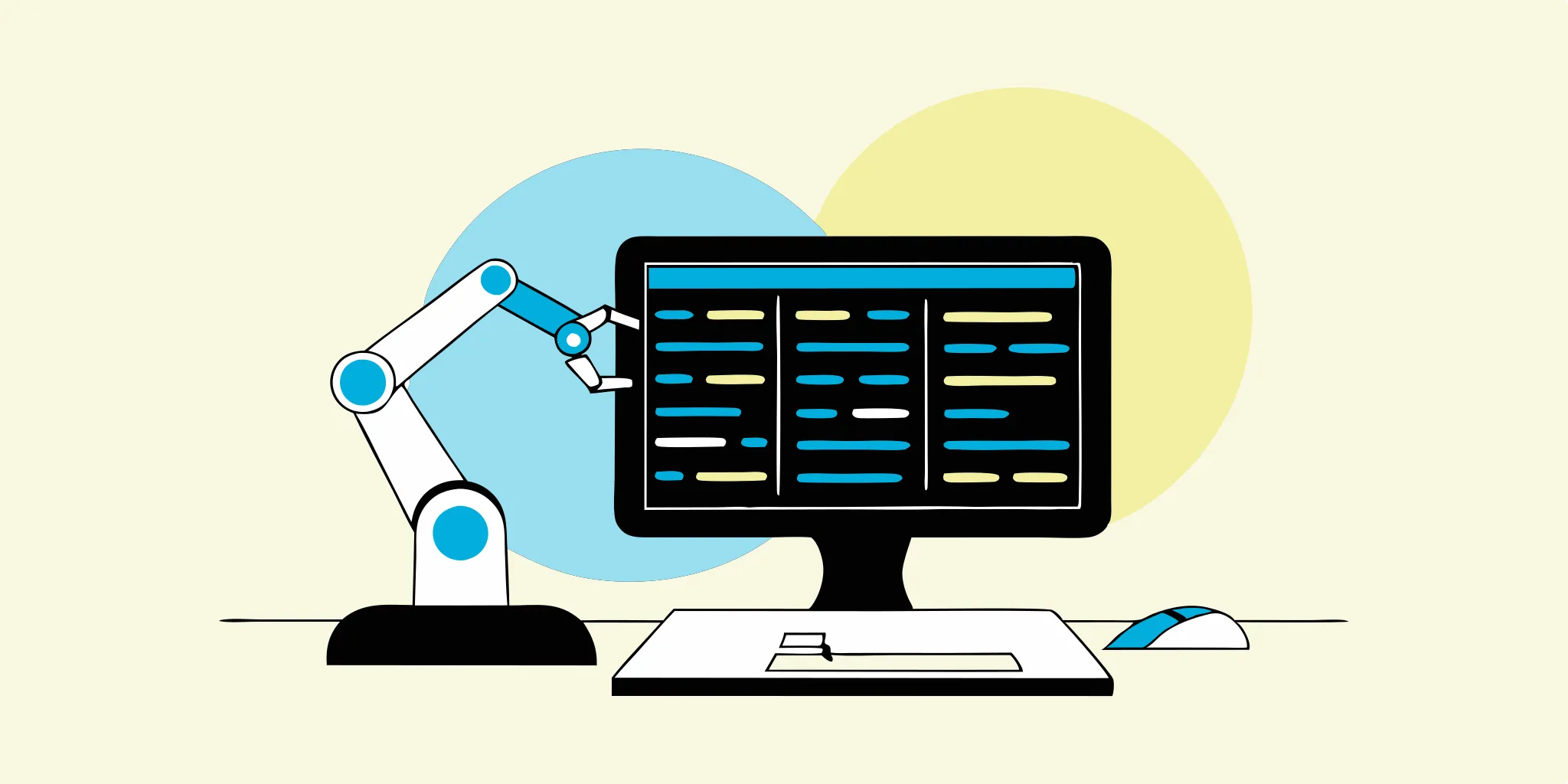
Table of Contents
Software bugs: a developer's nightmare. Delayed projects, frustrated users, and budget blowouts. But what if you could catch these bugs before they wreak havoc? Unit testing automation is your secret weapon. It's like having a dedicated QA team for every single piece of your code. This guide covers everything you need to know about unit testing automation: the core benefits, practical implementation steps, and best practices for writing effective automated unit tests. We'll also explore the top automated unit testing tools to streamline your workflow. Let's build more reliable software, together.
Key Takeaways
- Prioritize unit testing for robust software: Regularly testing individual code components helps catch errors early, improving software quality and reducing development costs. Keep your tests focused and independent, and remember to update them as your codebase changes.
- Select the right tools and integrate them into your workflow: Choose testing tools that fit your programming language and project. Frameworks like JUnit, NUnit, and pytest provide structure and helpful features. Integrate these tools into your CI/CD pipeline and code review process for a streamlined workflow.
- Address common testing challenges strategically: Use mocking to isolate units under test and simplify complex dependencies. Automate test data generation for consistency and efficiency. Focus on testing critical paths to balance thoroughness with execution time.
What is Unit Testing Automation?
Unit testing automation uses software separate from the software you're building to verify that individual units or components of code work correctly. Think of it as double-checking the building blocks of your software before assembling the whole thing. This helps catch bugs early in the development process, making them much easier and cheaper to fix. Automating these tests and integrating them into a continuous integration/continuous delivery (CI/CD) pipeline allows for consistent and frequent testing as you make code changes.
As Qodo puts it, automated unit testing uses software tools to automatically test small parts of your code, like individual functions (Qodo, n.d.). This proactive approach to quality assurance is essential for building reliable and maintainable software. By ensuring each component works correctly in isolation, you create a solid foundation for the entire system.
Beyond finding bugs, unit tests also serve as living documentation for your code. They clearly demonstrate how individual pieces of code should be used and their expected behavior. Cprime highlights this, stating that unit tests, written by developers to test individual parts of the code, help prevent errors during changes (Cprime, n.d.). This dual purpose of verification and documentation makes unit testing automation a critical practice for any development team aiming to deliver high-quality software. Services like those offered by MuukTest can help teams achieve comprehensive test coverage quickly and efficiently, ensuring software quality and faster release cycles.
Unlocking Automated Unit Testing
Unit Test Automation Explained
Automated unit testing uses software tools to automatically test individual units of code. Think of a "unit" as a small, isolated part of your program, like a single function or method. Instead of manually checking each function, automated tests do it for you, catching mistakes early in the development process. It's like having a robot meticulously examine every tiny gear in a clock to ensure it functions correctly before assembling the whole thing. This approach is especially valuable in fast-paced software development where finding and fixing bugs quickly is essential.
What is a Unit Test?
A unit test is like a tiny, focused inspection of a single part of your code—a function, method, or class. Imagine testing one gear in a complex clock mechanism to ensure it works perfectly before assembling the entire clock. That's what a unit test does for your software. It isolates a small piece of code and verifies it behaves exactly as expected in different scenarios. These tests are typically automated, meaning a separate program runs them and checks the results, saving you time and effort.
Why Automate Unit Tests?
Automating unit tests offers several key advantages. First, it dramatically speeds up the testing process. Imagine manually testing hundreds or even thousands of individual functions—it would be incredibly time-consuming! Automated tests can run in seconds, providing rapid feedback on your code changes. This speed is crucial for continuous integration and continuous delivery (CI/CD), where code is constantly being integrated and deployed. This rapid feedback loop allows developers to catch and address issues quickly, keeping the project on track.
Early bug detection is another major benefit. Unit tests help you catch errors early in the development cycle, when they're much easier and cheaper to fix. Think of it as preventative maintenance for your code. By catching bugs early, you avoid the headaches of debugging complex, integrated systems later on. This proactive approach to quality assurance is essential for building reliable and maintainable software, as highlighted by Qodo. Services like those offered by MuukTest can help streamline this process, ensuring comprehensive test coverage and faster release cycles. This means quicker time to market and a more efficient use of development resources.
Finally, automated unit tests act as living documentation for your code. They clearly show how individual components should function and what outputs to expect given specific inputs. This documentation is invaluable for other developers working on the project, especially as the codebase grows and evolves. As Cprime points out, this helps prevent errors during code changes and ensures everyone understands how different parts of the system work together. This clarity and consistency contribute to a more maintainable and collaborative development process, reducing onboarding time for new team members and facilitating smoother collaboration.
Manual Unit Testing: A Primer
Sometimes, manual testing makes the most sense. Consider situations where you need real user interaction, automating tests is too expensive, or you only run unit tests infrequently. As Study.com explains, manual unit testing is simply unit testing performed by a person, not a program. It's the classic method of having a developer or tester work through the code, checking inputs and outputs to ensure everything functions correctly.
Manual testing is especially valuable when evaluating user experience aspects that are difficult to automate. For example, consider testing the visual layout of a user interface or the flow of a drag-and-drop interaction. In these user-focused scenarios, Cprime highlights how manual testing offers nuanced insights that automated tests often miss. A human tester can quickly identify inconsistencies or awkward user flows that a script might overlook.
While automation is frequently the goal, manual testing still holds value. Study.com points out that if automation costs exceed the benefits, or if you're only running unit tests occasionally, manual testing might be more practical. It lets you focus resources effectively and avoid unnecessary overhead.
Manual Unit Testing: A Deep Dive
Manual unit testing involves personally scrutinizing every single unit of your code, running test cases, and verifying results by hand. While this hands-on approach might seem thorough, it quickly becomes time-consuming and error-prone, especially as your codebase grows. Imagine manually inspecting thousands of lines of code—it's a recipe for fatigue and missed bugs. Plus, manual testing is difficult to repeat consistently, making it hard to ensure that fixes don't introduce new problems elsewhere.
Think of it this way: you could spend hours meticulously checking every ingredient in a complex recipe, or you could use a kitchen scale for precise measurements every time. Manual testing is like the former—slow, tedious, and prone to human error. Automated testing, like the kitchen scale, provides accuracy and efficiency, letting you focus on the creative aspects of cooking (or coding!). As experts point out, relying solely on manual testing isn't sufficient for the rapid pace of modern software development. Automated testing leads to better software quality, faster releases, and less stress for your development team. The faster feedback loops provided by automated testing significantly reduce time spent fixing bugs and allow for quicker releases.
While manual unit testing has a place in some situations, like exploratory testing or usability testing, it shouldn't be your primary method for verifying code correctness. Unit testing is fundamental to building a solid foundation for your software, and automating these tests is key to efficiency and reliability. It's an integral part of the development process, not simply an add-on. For comprehensive test coverage quickly and efficiently, consider exploring AI-powered test automation services like those offered by MuukTest. We can help you achieve complete test coverage within 90 days, significantly enhancing your testing efficiency.
Key Components of Automated Unit Tests
Automated unit tests involve a few key parts working together. First, you write test cases—small pieces of code designed to check specific aspects of your unit's behavior. They provide quick answers about whether your code changes work. A testing framework provides the structure for running these tests and reporting the results. Many unit tests use "mocks" to stand in for real dependencies, like databases or external APIs. This isolation ensures you're only testing the unit itself, not its interactions with other parts of the system, making it easier to pinpoint issues. Developers write these small code tests to prevent regressions and build confidence in their code changes, contributing to a more robust and reliable software product.
Writing Effective Unit Tests
Well-written unit tests are the cornerstone of effective automated testing. They adhere to the FIRST principles—Fast, Independent, Repeatable, Self-Validating, and Thorough. While all of these are important, let's focus on Independence, Parallelizability (related to Independence), and being Order-agnostic. These characteristics ensure your tests are robust, reliable, and efficient.
Independent: Each test should stand on its own, unaffected by the outcome of other tests. This means no shared state or dependencies between tests. Imagine testing individual LEGO bricks. One brick's color shouldn't influence whether another brick snaps correctly. Similarly, one test's success or failure shouldn't impact another. This independence, as highlighted in discussions like this Reddit thread on automated testing, is crucial for effective automation, allowing tests to run in any order or even concurrently.
Parallelizable: Closely tied to independence, parallelizability means tests can run simultaneously. This significantly speeds up the testing process, especially beneficial for large projects. Think of it like checking out multiple groceries at once instead of one by one. If your tests are independent, they can be parallelized, drastically reducing overall testing time and providing faster feedback. Experts emphasize the importance of parallelization in modern software development for quicker release cycles.
Order-agnostic: The order in which tests run should not influence their results. A test should always yield the same outcome, regardless of its position in the sequence. This characteristic, also linked to independence, simplifies debugging. If a test fails only when run after a specific other test, it points to a hidden dependency. Maintaining order-agnostic tests ensures a reliable and consistent testing suite, contributing to a more robust end product, as discussed in resources like this article on unit testing and test automation.
The FIRST Principles of Unit Testing
Well-written unit tests are the cornerstone of effective automated testing. They adhere to the FIRST principles—Fast, Independent, Repeatable, Self-Validating, and Thorough. Each of these principles plays a crucial role in ensuring your tests are robust, reliable, and efficient.
1. Fast: Automated tests should execute quickly, providing immediate feedback. Think milliseconds, not minutes. This speed encourages frequent testing and integration, essential in agile development. Fast tests keep developers in a flow state and prevent context switching, boosting productivity. Agile methodologies benefit significantly from rapid feedback loops.
2. Independent: Each test should stand alone, unaffected by others. This means no shared state or dependencies. As highlighted in discussions on automated testing, this independence is crucial, allowing tests to run in any order or concurrently. One test's success or failure shouldn’t impact another, much like testing individual LEGO bricks—one brick's color shouldn’t influence whether another snaps correctly.
3. Repeatable: Tests should yield the same results every time, regardless of the environment. This repeatability maintains confidence in the results and ensures code changes are accurately reflected. A test that passes sometimes and fails other times without code changes is a flaky test, eroding trust in the entire suite. Consistent results are key for regression testing and ensuring software stability.
4. Self-Validating: A unit test should have a clear pass/fail outcome without human interpretation. This allows developers to quickly assess if their code works or needs further investigation. The test itself should contain the logic to determine if the code under test behaves as expected. Clear results streamline the code review process.
5. Thorough: While tests should be focused (testing one specific thing), they should also cover a wide range of scenarios, including edge cases and boundary conditions. This thoroughness helps catch potential bugs. Think about all the possible inputs and how the code should handle them, both valid and invalid. Test coverage is a key metric for evaluating thoroughness.
By adhering to these FIRST principles, development teams create a more effective and efficient testing process, leading to higher quality software. Services like those offered by MuukTest can help implement these principles and achieve comprehensive test coverage efficiently.
Mock Objects: Streamlining Unit Tests
Think of mock objects as stunt doubles for the real dependencies in your code. Let's say you're testing a function that interacts with a database. Instead of actually connecting to the database (which can be slow and complex), you use a mock object that mimics the database's behavior. This mock object provides pre-programmed responses to your function's requests, allowing you to isolate the function's logic and test it independently. This technique is especially useful when dealing with external services, APIs, or other components that might not be readily available during testing. By using mocks, you create a controlled environment where you can focus solely on the unit under test, simplifying the testing process and making it more efficient. For a deeper dive into these concepts, resources on unit testing and test automation offer valuable insights.
Using mock objects offers several advantages. It simplifies complex dependencies, making your tests easier to write and understand. It also speeds up your tests by avoiding time-consuming interactions with real dependencies. Plus, it makes your tests more reliable by eliminating external factors that could influence the results. For example, if your tests rely on a live database, network issues or database outages could cause your tests to fail even if your code is correct. Mocks eliminate these external dependencies, ensuring that your tests are consistent and repeatable. This focus on isolated testing is a core principle of unit test automation, allowing developers to pinpoint issues quickly and efficiently. Services like MuukTest specialize in providing comprehensive and efficient test automation services, helping developers achieve complete test coverage and ensure software quality.
Why Automate Your Unit Tests?
Automated unit testing offers several advantages that contribute to more efficient and higher-quality software development. Let's explore some key benefits:
Benefits of Automated Unit Testing
Automated unit testing offers several advantages that contribute to more efficient and higher-quality software development. Let's explore some key benefits:
Improved Teamwork and Collaboration
Automated tests provide a common framework for all team members, making it easier to collaborate and share knowledge. When everyone understands the expected behavior of individual code units, it streamlines communication and reduces misunderstandings. This shared understanding fosters better teamwork and a more cohesive development process. Plus, with automated tests in place, new team members can quickly understand the codebase and contribute effectively. For more insights on the impact of automated testing on team dynamics, explore resources like this Qodo glossary entry.
Reduced Risk and Increased Confidence
By testing each part individually, you reduce the risk of bugs in the overall system, leading to greater confidence in your code changes. Unit tests act as a safety net, catching errors early before they become larger problems. This early detection minimizes the risk of introducing regressions and allows developers to make changes with more confidence, knowing their code is thoroughly vetted. Qodo offers further explanation of these benefits.
Enhanced Efficiency and Time Savings
Automating the testing process saves time and resources compared to manual testing, allowing developers to focus on more complex tasks. Automated tests handle repetitive checks, freeing up developers for more creative and strategic work. This increased efficiency translates to faster development cycles and quicker time to market. Learn more about the efficiencies gained through unit test automation.
Faster Feedback Loops
Automated testing provides faster feedback loops, significantly reducing the time spent fixing bugs and allowing for quicker releases. Instead of waiting for manual testing to uncover issues, automated tests provide immediate feedback during coding. This rapid feedback allows you to address problems while they're still fresh in your mind, making debugging much more efficient. This, in turn, leads to faster iteration and quicker release cycles. Cprime discusses the importance of fast feedback loops in software development.
Higher Quality Software
Catching bugs early makes fixing them much easier and cheaper, ultimately leading to higher quality software. The cost of fixing a bug increases exponentially the later it's discovered. Automated unit tests, by catching bugs early in the development process, minimize the cost and effort of fixing them. This proactive approach results in higher quality software with fewer defects and improved reliability. This focus on quality is a key driver for businesses investing in test automation services like those offered by MuukTest. For a deeper understanding of how early bug detection contributes to higher quality software, explore resources like this Qodo glossary entry.
Write Better Code with Automated Unit Tests
Think of automated unit tests as tiny robots inspecting individual parts of your code, like functions or methods, ensuring they perform exactly as expected. This process, often integrated directly into your coding workflow, catches errors early on. Finding and fixing these issues early is significantly easier (and cheaper) than addressing them later in the development cycle. This proactive approach leads to more robust and dependable software. By verifying each component works correctly in isolation, you build a solid foundation for the entire application. This early error detection minimizes the risk of bugs propagating through the system and impacting other parts of the codebase.
Improve Code Quality and Modularity
Automated unit testing encourages better code design. When you write unit tests, you consider your code from a user's perspective. This naturally leads to more modular and well-defined components. By focusing on testing individual units in isolation, you create code that is easier to understand, maintain, and reuse. Think of it like building with LEGOs: each brick (unit) has a specific function, and when combined correctly, they create complex structures. Similarly, well-defined units of code, validated by unit tests, contribute to a more robust and maintainable software architecture. This modularity simplifies future development, as changes to one unit are less likely to affect others. This improved code structure, facilitated by unit testing, is key to long-term software quality, as highlighted in resources like this explanation of unit test automation. For teams looking to enhance their testing strategies, services like MuukTest offer comprehensive automated testing solutions.
Find Bugs Early and Save Money
Finding and fixing problems early is significantly cheaper than addressing them later. Automated unit testing acts like a dedicated quality assurance team, carefully examining each part of your code. As Qodo explains, this proactive approach catches errors early in the development process, reducing the cost of fixes. Imagine building a house—it's much easier (and cheaper) to fix a cracked foundation before the entire structure is built. Similarly, identifying and resolving bugs during unit testing prevents them from becoming larger issues later. This early bug detection translates directly into cost savings by reducing debugging time and rework. Industry experts emphasize this benefit, highlighting how automated unit testing contributes to more efficient and higher-quality software development. By catching bugs early, you improve software quality and protect your budget. To learn more about cost-effective automated testing, explore MuukTest's pricing page for details on their AI-powered test automation services.
Modular Code, Fewer Bugs: The Power of Unit Tests
Automated unit tests are like tiny robots inspecting individual parts of your code—functions or methods—ensuring they perform exactly as expected. This process, often integrated directly into your coding workflow, catches errors early on. Finding and fixing these issues early is significantly easier (and cheaper) than addressing them later in the development cycle, as highlighted in resources on unit testing and test automation. This proactive approach leads to more robust and dependable software. By verifying each component works correctly in isolation, you build a solid foundation for the entire application. This not only improves overall code quality but also encourages a more modular design. When you know each unit functions correctly on its own, you can confidently combine these units like building blocks, creating more complex and feature-rich applications. This modularity makes your code easier to understand, maintain, and extend over time.
Catch Bugs Early, Save Time and Money
Automated unit testing offers several advantages that contribute to more efficient and higher-quality software development, as explained in this piece on unit test automation. A key benefit is finding and fixing bugs early in the development process when they are easier and cheaper to address. Think of it like finding a small crack in a bridge during construction versus discovering it after the bridge is open to traffic. The earlier you catch the problem, the less disruptive and expensive the fix. Similarly, early defect detection through unit testing minimizes the risk of bugs spreading through the system and impacting other parts of the codebase. This early intervention reduces development costs and leads to a faster time to market, allowing you to deliver value to your users more quickly. For businesses looking to streamline this process, MuukTest offers AI-powered test automation services designed for efficient and cost-effective comprehensive test coverage.
Develop Faster with Automated Unit Tests
Automated tests provide rapid feedback on the impact of code changes. This quick feedback loop allows for more efficient development and faster iteration. You can confidently make changes, knowing that automated tests will immediately flag any unintended consequences. This accelerates the development process and allows you to deliver working software more quickly. This rapid feedback cycle also fosters a more agile development environment, enabling teams to respond quickly to changing requirements and deliver value faster.
Cut Costs with Automated Unit Testing
Early bug detection is a cornerstone of efficient software development. Automated unit tests help identify bugs early in the development process, when they are less complex and less expensive to fix. Addressing bugs early contributes to better software quality and reduces overall development costs. Finding a bug in a small, isolated unit is much simpler than tracking it down within a complex, integrated system. This early detection minimizes the time and resources spent on debugging later, which can become significantly more costly and time-consuming as the project progresses. Early bug detection also reduces the likelihood of critical issues arising in production, saving you from potential headaches and reputational damage.
Cost-Effective Testing: Unit Tests vs. the Rest
While all testing is crucial, unit testing offers a particularly cost-effective approach compared to other methods like integration, system, or UI testing. Think of it this way: catching a typo in a single sentence is much easier than finding it after you've printed a whole book. Similarly, identifying and fixing a bug in a small, isolated unit of code is significantly less expensive than discovering it later during integration or system testing. As this article points out, finding and fixing these issues early is significantly easier (and cheaper) than addressing them later in the development cycle. When bugs are found later in the development cycle, they often involve more complex interactions and require more effort to track down and resolve. This proactive approach leads to more robust and dependable software.
Imagine building a house. Unit testing is like inspecting each brick and board before construction. Integration testing is like checking if the walls fit together properly once they're built. System testing is like making sure the plumbing and electricity work after the house is complete. And UI testing is like confirming all the doors and windows open and close smoothly after the house is furnished. While each step is important, finding a faulty brick early on is much less disruptive (and cheaper) than having to tear down a wall or rewire the entire house later. Early bug detection is a cornerstone of efficient software development and reduces overall costs. For comprehensive and efficient test automation services, consider exploring MuukTest, which can help you achieve complete test coverage and ensure software quality.
Reduce Technical Debt with Unit Testing
Technical debt is like those nagging household chores you keep putting off. Ignoring them seems fine now, but eventually, you'll have a much bigger mess to clean up. In software development, those "chores" are the shortcuts and quick fixes that accumulate over time. Automated unit testing helps you stay on top of things. By catching bugs early, when they're small and manageable, you prevent them from snowballing into major problems that require significant time and resources to fix. Think of unit tests as your code's regular check-ups, ensuring everything runs smoothly and preventing minor issues from becoming chronic conditions. This proactive approach improves the health of your codebase and reduces the accumulation of technical debt, allowing your team to move faster and more efficiently. Cprime highlights how addressing these issues early is significantly easier (and cheaper) than dealing with them later in the development cycle, leading to more robust and dependable software.
Unit tests also give developers the confidence to refactor code—reorganizing and improving its structure—without fear of introducing new bugs. It's like rearranging your closet; you want to make it tidier without accidentally losing a favorite sweater. Unit tests act as a safety net, ensuring that while improving the code's organization, you're not breaking its functionality. This ability to refactor with confidence is crucial for maintaining a healthy, manageable codebase and minimizing technical debt. Qodo explains how these small tests prevent regressions and build confidence in code changes, contributing to a more robust and reliable software product. This confidence empowers continuous improvement and adaptation to changing requirements without the risk of destabilizing the entire system.
Simplify Code Maintenance with Automated Unit Tests
Automated unit tests provide a safety net when refactoring or maintaining existing code. They give you the confidence to make changes without fear of introducing new bugs. Automated tests run repeatedly, ensuring that updates don't break existing functionality. This makes maintaining and updating your codebase much less risky and time-consuming. You can refactor with confidence, knowing that your tests will alert you to any unintended side effects. This safety net encourages continuous improvement and allows developers to adapt the codebase to evolving needs without compromising stability. Well-maintained unit tests contribute to a more sustainable and maintainable codebase in the long run.
How Automated Unit Testing Works
Automated unit testing systematically checks individual parts of your software, ensuring each component functions correctly in isolation. This process typically involves three key steps: writing test cases, executing those tests, and interpreting the results.
The Importance of Test Isolation
Isolation is key to effective unit testing. Think of it like testing a single ingredient in a recipe before combining everything. You want to understand how that ingredient behaves on its own, not how it interacts with others. In unit testing, we achieve this isolation using mocks to stand in for real dependencies, like databases or external APIs. These mocks mimic the behavior of real components, but let us control their responses and isolate the unit under test. This ensures we're only testing the unit itself, not its interactions with other parts of the system, making it easier to pinpoint issues. This precise focus simplifies debugging and helps you quickly identify the root cause of problems.
Testing Methods and Functions Effectively
Automated unit testing focuses on the smallest testable parts of your code: individual methods and functions. These are the building blocks of your application, and ensuring they work correctly in isolation is crucial. Each test case focuses on a specific method or function, verifying it produces the expected output given a particular input. By verifying each component works correctly in isolation, you build a solid foundation for the entire application. This early error detection minimizes the risk of bugs spreading through the system and impacting other code. This methodical approach helps you build confidence in your code's reliability, one function at a time. For larger projects, consider services like MuukTest's test automation to streamline this process and ensure comprehensive test coverage.
Writing Powerful Test Cases for Automation
Think of test cases as specific scenarios you create to evaluate a small piece of your code, like a single function or method. Each test case defines inputs, executes the code with those inputs, and checks if the actual output matches what you expect. For example, if you have a function that calculates the area of a rectangle, your test cases might include various combinations of length and width, along with the expected area for each. Clear, well-defined test cases are crucial for effective unit testing. This stage often involves using a unit testing framework which provides tools and structure for writing and organizing your tests.
Run Tests Efficiently
Once you've written your test cases, an automated testing tool takes over. This tool runs your tests, feeding the specified inputs to your code and comparing the actual outputs against your expected results. This process is typically very fast, allowing you to quickly assess the impact of code changes. The execution phase often happens automatically as part of a continuous integration/continuous delivery (CI/CD) pipeline, providing immediate feedback on the health of your code.
Integrating Unit Tests into a CI/CD Pipeline
Integrating your unit tests into a continuous integration/continuous delivery (CI/CD) pipeline sets up automated quality checks throughout your software development process. Every code change triggers your unit tests, providing immediate feedback on potential bugs. This early warning system catches issues before they become larger problems, much like spotting a misplaced LEGO brick early in the building process. Automating these tests within a CI/CD pipeline ensures consistent and frequent testing with every code change.
This integration offers significant advantages. Faster feedback loops reduce debugging time and enable quicker releases. Catching bugs early simplifies fixes and lowers costs. This rapid feedback also promotes a more agile environment, allowing teams to adapt to changing requirements and deliver value quickly. Integrating testing tools into your CI/CD pipeline and code review process streamlines your workflow. Services like MuukTest can help integrate automated testing into your workflows, ensuring comprehensive test coverage and faster releases.
Interpreting Test Results and Next Steps
After the tests run, the testing tool generates a report summarizing the outcome of each test case. A successful test indicates the code behaved as expected, while a failed test highlights a potential problem. These reports help you pinpoint bugs early in the development cycle, making them easier and less expensive to fix. Interpreting the results effectively allows you to quickly identify and address issues, leading to more reliable and maintainable software. MuukTest provides comprehensive test coverage reports, giving you a clear picture of your software's quality.
Choosing the Right Automated Unit Testing Tools
Choosing the right tools and frameworks is crucial for effective automated unit testing. Your selection will depend on factors like your programming language, project requirements, and team expertise. Let's explore some popular options:
Language-Specific Testing Frameworks
Many excellent unit testing frameworks are designed for specific programming languages. These frameworks offer tailored features and integrate seamlessly with your development environment. For instance, if you're working with Java, JUnit is a robust and widely adopted framework. It provides a rich set of annotations and assertions to structure and execute your tests. Similarly, NUnit is a popular choice for C# and VB.NET developers, offering a comparable feature set within the .NET ecosystem. For Python developers, PyTest stands out for its simplicity and extensibility. Its concise syntax and powerful plugin system make it a favorite for projects of all sizes.
JUnit (Java)
JUnit is a robust and widely adopted framework for Java. It provides a rich set of annotations for defining test methods and setting up test data, and assertions for verifying expected outcomes within your tests. This makes structuring and running tests straightforward. JUnit's widespread adoption means ample community support and resources are readily available.
TestNG (Java)
TestNG, another popular Java testing framework, offers advanced features like parallel test execution and data-driven testing. Running tests in parallel, much like checking out groceries at multiple counters, significantly speeds up the process. Data-driven testing allows you to use different sets of data with the same test, similar to trying a recipe with various types of flour. These features make TestNG suitable for complex testing scenarios.
PyUnit (Python)
PyUnit, also known as unittest
, is Python's built-in testing framework. It's readily available with your Python installation, providing a solid foundation for writing and running tests. PyUnit's simplicity and accessibility make it a great starting point, especially for those new to Python testing.
PyTest (Python)
PyTest stands out for its simple syntax and extensibility. Writing tests with PyTest feels natural, and its powerful plugin system allows for easy customization and added features. This flexibility makes PyTest a favorite for Python projects of all sizes.
PerlUnit (Perl)
PerlUnit is a testing framework specifically designed for Perl. It provides a structured approach to writing and executing unit tests, allowing developers to create test cases that verify the functionality of individual components. This ensures each unit behaves as expected. This framework is particularly beneficial for maintaining code quality in Perl applications because it facilitates early bug detection and promotes a more modular code structure, aligning with the principles of unit test automation.
As with other unit testing frameworks, PerlUnit emphasizes test isolation. By using mock objects to simulate dependencies, developers can focus on testing the logic of their code without interference from external factors. This isolation is crucial for identifying issues quickly and efficiently, making it easier to maintain and refactor code over time. This practice also contributes to reducing technical debt, a key benefit we discussed earlier.
Incorporating PerlUnit into your development workflow can significantly improve software quality. Automated unit tests catch bugs early and serve as documentation, illustrating how different components interact. This dual purpose enhances team collaboration and contributes to a more robust development process. For more information on PerlUnit, explore resources like this Perl.com article.
Test::Unit (Ruby)
Test::Unit is a popular Ruby testing framework. It offers a straightforward approach to writing effective unit tests, making it easy for developers to get started with testing in Ruby. Test::Unit provides the essential features you need for solid test coverage.
Jest (JavaScript)
Jest is a delightful JavaScript testing framework, particularly well-suited for React projects. Its focus on simplicity makes writing tests a more pleasant experience. Jest's snapshot testing feature captures your component's output and compares it against future renders, preventing unexpected visual changes in your UI.
NUnit (C#)
NUnit is a popular choice for C# and VB.NET developers. It offers a robust set of features comparable to JUnit, providing a familiar testing environment for those working within the .NET ecosystem. NUnit ensures thorough testing for your C# code.
xUnit.net (C#)
xUnit.net is a modern testing framework for .NET known for its extensibility and flexibility. It encourages testing best practices and offers a clean, contemporary approach, making it a popular choice for C# developers building modern applications.
PHPUnit (PHP)
PHPUnit is a widely used testing framework for PHP. It provides a rich set of features, giving developers detailed control over their tests. Whether for small websites or large web applications, PHPUnit offers the tools necessary for comprehensive testing and quality assurance in PHP projects.
Jasmine (JavaScript)
Jasmine is a behavior-driven development (BDD) framework for JavaScript. BDD focuses on describing the desired behavior of your code clearly. Jasmine's clean syntax and focus on behavior make writing understandable and effective tests easier. Its seamless integration with other tools makes it a versatile choice for various JavaScript projects.
xUnit.net for C# and VB.NET
xUnit.net is a popular testing framework for C# and VB.NET developers. It provides a rich set of features that help structure and execute tests effectively. Its design encourages best practices in test writing and offers a flexible approach to testing, making it a preferred choice among .NET developers.
PHPUnit for PHP
PHPUnit is a widely used testing framework for PHP developers. PHPUnit offers a comprehensive set of tools for writing and running unit tests, making it easier to ensure code quality. With its focus on simplicity and ease of use, PHPUnit allows developers to create tests quickly and integrate them into their development workflow seamlessly.
Jasmine for JavaScript Testing
Jasmine is a popular testing framework for JavaScript that provides a behavior-driven development (BDD) approach to testing. It allows developers to write clear and expressive tests, making it easier to understand the expected behavior of their code. Jasmine's rich feature set and ease of integration with other tools make it a go-to choice for JavaScript testing.
Benefits of Using a Testing Framework
Choosing the right tools and frameworks is crucial for effective automated unit testing. Your selection will depend on factors like your programming language, project requirements, and team expertise. Frameworks like JUnit, NUnit, and pytest provide structure and helpful features that streamline the testing process, ensuring that tests are easy to write, maintain, and execute. For more information on choosing the right framework for your needs, explore MuukTest's test automation services. This Cprime article also offers a deeper dive into unit testing and test automation.
Comparing Framework Advantages
Each unit testing framework offers unique strengths. JUnit, a stalwart for Java, boasts extensive documentation and vast community support, making it easy to find solutions and examples. This robust framework provides a rich set of annotations and assertions, giving your tests structure and clarity. If you're working with C# or VB.NET, NUnit is a popular choice, offering a comparable feature set within the .NET ecosystem. A key advantage of NUnit is its support for data-driven testing, allowing you to run the same test with various inputs, maximizing efficiency and coverage. For Python enthusiasts, PyTest shines with its simplicity and extensibility. Its concise syntax makes writing tests a breeze, and the powerful plugin system caters to projects of all sizes. Pytest also excels at creating shorter, more readable test cases and offers fixtures for efficient setup and teardown processes. Another strong contender in the .NET world is xUnit.net. This framework encourages best practices in test writing and offers a flexible approach to testing, making it a favorite among .NET developers. For PHP projects, PHPUnit provides a comprehensive suite of tools for writing and running unit tests, simplifying quality assurance. Finally, for JavaScript developers, Jasmine offers a behavior-driven development (BDD) approach. This allows for clear, expressive tests that focus on the expected behavior of your code, making them easier to understand and maintain. For a deeper dive into the nuances of unit testing and test automation, Cprime offers valuable insights.
Selecting the Right Tool for Your Needs
Key Features of Unit Testing Frameworks
Choosing the right tools and frameworks is crucial for effective automated unit testing. Your selection will depend on factors like your programming language, project requirements, and team expertise. Think of it like choosing the right kitchen gadget—a blender is great for smoothies, but not so much for chopping vegetables. Similarly, different testing frameworks excel in different areas. MuukTest's test automation services can provide guidance on selecting the best tools for your specific needs.
Look for frameworks that provide a clear and consistent structure for writing your tests. This structure makes tests easier to read, understand, and maintain. Features like annotations (in Java’s JUnit, for example) or attributes (like those in NUnit for C#) can help organize and describe your tests. Robust assertion libraries are also essential. These libraries provide pre-built functions for checking various conditions, like equality, inequality, or whether an exception was thrown. This simplifies the process of verifying your code’s behavior. A good framework should also offer clear and concise reporting, making it easy to identify which tests passed and which failed.
Many excellent unit testing frameworks are designed for specific programming languages. These frameworks offer tailored features and integrate seamlessly with your development environment. For instance, if you're working with Java, JUnit is a robust and widely adopted framework. It provides a rich set of annotations and assertions to structure and execute your tests. This article on unit testing and test automation offers a deeper look into the benefits of using frameworks like JUnit. Similarly, NUnit is a popular choice for C# and VB.NET developers, offering a comparable feature set within the .NET ecosystem. For Python developers, PyTest stands out for its simplicity and extensibility, making it a favorite for projects of all sizes.
Cloud-Based Testing Platforms
Cloud-based testing platforms offer a powerful way to streamline and enhance your automated unit testing. These platforms give you access to various testing environments—different operating systems, browsers, and devices—without managing physical infrastructure. This flexibility is invaluable for ensuring your software works seamlessly across different user setups.
A key advantage of cloud-based testing is accelerating the testing process. Platforms like pCloudy let you run tests on many devices concurrently, drastically reducing the overall testing time. This speed boost is essential for rapid release cycles and delivering value quickly. Services like MuukTest enhance this efficiency with AI-powered test automation for comprehensive test coverage.
Integrating cloud-based testing tools into your CI/CD pipeline allows consistent and frequent testing as you change code. This continuous testing catches bugs early and prevents them from spreading. This integration ensures thorough vetting of every code change, contributing to higher software quality and faster releases. Qodo notes that automated unit testing, like a dedicated quality assurance team, can lead to significant cost savings.
Cprime points out that cloud-based platforms facilitate better team collaboration. By providing real-time updates and shared access to testing environments, these platforms enhance communication and transparency within development teams. This shared visibility keeps everyone aligned and allows for quick responses to testing issues.
Cross-Platform Testing with Selenium
While language-specific frameworks are essential for unit testing, you might also need tools for broader testing needs. Selenium is a powerful tool for automating web browser interactions. This allows you to test your web applications across different browsers and operating systems, ensuring consistent functionality for all users. Selenium's cross-platform capabilities are invaluable for verifying the compatibility of your web application, ensuring a seamless user experience regardless of the browser or operating system.
Selenium: Integration and Functional Testing
Selenium, primarily known for end-to-end testing, also provides significant value for integration and functional testing. In these scenarios, Selenium helps verify the interactions between different application components or tests the functionality of complete features. For example, you could use Selenium to test the data flow between your user interface and backend API. Alternatively, it's useful for testing a specific user workflow, like completing an online purchase or submitting a form, ensuring all integrated parts work together seamlessly.
Selenium's strength lies in simulating real user interactions within a browser. This makes it especially useful for testing UI elements and JavaScript functionality, often difficult to test with other methods. Automating these interactions helps catch browser compatibility issues, JavaScript errors, and unexpected UI behavior, ensuring a consistent user experience across different browsers and platforms. For a deeper dive into cross-platform testing and its benefits, check out this article on Selenium and unit testing frameworks.
Streamline Your Workflow with IDE Extensions
Most modern Integrated Development Environments (IDEs) offer extensions or built-in features that streamline automated testing. These tools can significantly enhance your workflow by allowing you to run tests directly within your coding environment. This tight integration reduces context switching and makes it easier to incorporate testing into your daily development routine. Many IDE extensions also provide helpful features like test result visualization and code coverage analysis, giving you valuable insights into your test suite's effectiveness.
Automated Unit Testing vs. Other Testing Methods
The Testing Pyramid (and Trophy)
Visualize your testing strategy like a pyramid (or, if you're feeling ambitious, a trophy!). Unit tests form the solid base, followed by a smaller layer of integration tests, and a still smaller top layer of end-to-end tests. This structure helps you prioritize and balance your testing efforts, ensuring comprehensive coverage without overspending on slower, more complex tests. Think of it like building a house: you wouldn't install the roof before ensuring the foundation is solid. Similarly, a strong base of unit tests ensures your individual components work correctly before testing how they interact. For a deeper dive into testing strategies and the reasoning behind this layered approach, check out Martin Fowler's insightful article on the practical test pyramid.
The Honeycomb Approach for Microservices
Think of a honeycomb—a structure of individual cells working together. That’s analogous to a microservices architecture. Like a honeycomb, a microservices architecture consists of independent services (the cells) that function autonomously yet contribute to the overall system. This structure offers several advantages, especially for testing.
In a microservices architecture, each service is self-contained, like individual honeycomb cells. This allows teams to develop, deploy, and scale services independently. This independence is key, enabling teams to work on different system parts without conflict. It also simplifies testing.
The “Testing Honeycomb” approach mirrors this structure. Just as a honeycomb supports independent microservices, this testing strategy emphasizes testing each service thoroughly in isolation. This involves various testing types, including unit tests, integration tests, and end-to-end tests. This approach ensures each microservice operates correctly both individually and within the larger system. This comprehensive testing builds confidence in the functionality of each service and the entire system. Because each service is tested independently, it’s easier to pinpoint and fix problems quickly.
The honeycomb structure facilitates better communication and integration between services. Well-defined interfaces and APIs connect microservices, much like the walls of a honeycomb connect individual cells. This allows for flexible and scalable systems, where changes in one service don’t require changes in others. This loose coupling is a key advantage of microservices, making the system more resilient and adaptable. For more on this approach, read more here.
API Testing vs. Unit Testing
API tests check how different parts of your software interact, essentially verifying the "glue" that holds your application together. They focus on the communication between components, ensuring data flows correctly and the system behaves as expected when different parts work together. Unit tests, conversely, zoom in on individual components in isolation. They're like testing individual LEGO bricks before assembling a larger structure. Finding the right balance between API and unit testing is key to a robust testing strategy. While unit tests provide a solid foundation, API tests ensure those units collaborate effectively. This Reddit discussion offers helpful perspectives on the differences between API and unit tests. For projects looking to achieve a robust balance between different testing types, consider exploring MuukTest's test automation services, designed for comprehensive test coverage.
Automated Testing vs. Unit Testing
Automating unit tests means they run automatically, typically during your build process or as part of a continuous integration/continuous delivery (CI/CD) pipeline. This automation provides rapid feedback and ensures consistency. However, not all unit tests are automated; some teams perform manual unit testing, especially for exploratory testing or when automation is impractical. It's important to remember that automated testing is a broad umbrella encompassing various test types, including unit, integration, regression, and automated UI testing. This Reddit thread clarifies the relationship, highlighting that unit testing is one piece of the larger automated testing puzzle. Services like MuukTest can help streamline your automated testing strategy, ensuring comprehensive coverage and efficient execution.
Comparing Different Testing Approaches
Understanding how automated unit testing fits within the broader testing landscape is crucial for leveraging its full potential. While other testing methods play important roles, unit testing offers unique advantages at a specific stage of the software development lifecycle.
Understanding the Test Pyramid
The Test Pyramid is a visual metaphor that helps developers create a balanced and efficient testing strategy. It’s a simple way to visualize the ideal distribution of different types of software tests. Picture a pyramid with three levels. Each level represents a different type of test, with the quantity of tests decreasing as you ascend the pyramid.
At the base of the pyramid are unit tests. These tests examine individual components of your code in isolation, like a single function or method. Because they are small and isolated, unit tests are usually quick to write and execute. They are the foundation of your testing strategy, catching errors early and preventing them from becoming larger issues. As Martin Fowler explains, the Test Pyramid guides developers to group software tests by their scope, with unit tests forming the largest group.
The middle layer of the pyramid contains integration tests. These tests verify how different units of code work together. For example, an integration test might check the interaction between your application and a database. Integration tests are more complex than unit tests and generally take longer to run. CircleCI notes that the testing pyramid encourages developers to prioritize tests that are fast, reliable, and easy to maintain, such as unit tests.
At the top of the pyramid are end-to-end (E2E) tests. These tests evaluate the entire application from start to finish, simulating real user scenarios. E2E tests are the most complex and time-consuming, but they are vital for ensuring the overall functionality of your application. Perfecto by Perforce highlights the testing pyramid as a key element of a successful software testing strategy for app releases.
The Test Pyramid emphasizes a balanced testing approach. By focusing on fast and reliable unit tests at the base, you can catch most errors early and efficiently. The tests become more complex and time-consuming as you move up the pyramid, so you'll want fewer of them. This approach, as Software Tester describes, allows for fast, effective testing that builds confidence in the quality of your software. A well-structured testing strategy, guided by the Test Pyramid, is essential for delivering high-quality software efficiently. For a deeper understanding of how to implement a robust testing strategy, explore MuukTest's test automation services.
Unit Testing vs. Integration Testing
Unit testing zeroes in on the smallest parts of your code, isolating individual functions or methods to verify they perform as expected. Think of it like testing individual LEGO bricks before assembling a complex structure. This differs from integration testing, which examines how these individual units interact with each other. Integration testing is like ensuring all the LEGO bricks fit together seamlessly once you start building the model. Both are essential, but they serve distinct purposes. Unit testing catches issues early within individual components, while integration testing identifies problems that arise from the interplay between those components.
Unit Testing vs. Functional Testing
Functional testing takes a higher-level view, evaluating the system against its specified requirements. It's less concerned with the internal workings of individual units and more focused on whether the overall system delivers the expected functionality from the user's perspective. Going back to our LEGO analogy, functional testing is like checking if the finished LEGO model looks and functions as intended, regardless of how the individual bricks are connected. Unit testing ensures the individual bricks are sound, while functional testing ensures the entire model works as a whole.
End-to-End Testing Explained
End-to-end testing checks the entire software product from start to finish. It simulates real user scenarios, like a customer completing an order on an e-commerce site. This comprehensive approach ensures all parts of your system work together correctly, providing valuable insights into the overall user experience and identifying potential issues that might not surface during individual unit tests. Think of it as a final dress rehearsal before opening night. Learn more about end-to-end testing.
Acceptance Testing: Validating User Needs
Acceptance testing determines if the system meets pre-defined acceptance criteria and is ready for release. End-users or stakeholders often conduct this testing, evaluating the software's functionality and usability against their specific requirements. It's like a focus group providing feedback before a product launch. This user-centric approach ensures the software is not only functional but also user-friendly and suitable for its intended purpose.
Performance and Stress/Load Testing
Performance testing assesses how your system performs under different conditions. Load testing, a subset of performance testing, checks system behavior under expected and peak loads, like increased website traffic during a sale. Stress testing pushes the system beyond its limits to evaluate its response to extreme conditions, such as a sudden spike in database queries. These tests identify bottlenecks and ensure your system can handle real-world demands. MuukTest can help optimize your software's performance.
Regression Testing: Ensuring Code Stability
After code changes, regression testing ensures existing functionality remains intact. It's like double-checking your work after editing a document. Essential after updates or bug fixes, it verifies that previous features still work correctly. Automated regression tests provide a safety net for making changes without introducing new issues, maintaining software stability, and reducing the risk of unexpected problems after deployment. Get started with automated regression testing today.
Smoke/Synthetic Testing: Quick Checks for Critical Functionality
Smoke testing is a preliminary check of an application's critical functions—a quick health check before a thorough examination. It ensures core features work before more detailed testing. Synthetic testing simulates user interactions to validate performance and availability, identifying potential issues early and ensuring readiness for rigorous testing.
Data-Driven Testing
Data-driven testing (DDT) is a software testing method where you use the same test multiple times, but with different data each time. Think of it like trying a new recipe—you might use different types of flour or sweeteners to see how it affects the final product. In DDT, the test logic (the recipe) stays the same, but the input data (the ingredients) changes. This approach separates the test data from the test logic, making your tests more flexible and easier to maintain. You can store your test data in a table or spreadsheet, and your test script will pull the data from this source for each run. This allows you to test a wide range of scenarios without writing a separate test for each one. This method is particularly useful when you need to test the same functionality with a variety of inputs, such as boundary conditions, invalid data, or different data types. DataSunrise discusses how data-driven testing enhances software quality by allowing for broader test coverage with less code.
Keyword-Driven Testing
Keyword-driven testing uses keywords to represent actions within your test scripts. Imagine having a set of instructions where each step is a simple command like "Login," "Add to Cart," or "Checkout." These commands are your keywords, and they abstract the underlying code complexity. This approach makes it possible to create tests without needing to understand the code itself, opening up testing to non-technical team members. Keyword-driven testing promotes reusability. You can create a library of keywords and reuse them across multiple tests. This not only saves time but also ensures consistency in your testing approach. If you need to change a specific action, you only need to update the corresponding keyword, and the change will automatically apply to all tests using that keyword. This makes maintenance much easier and reduces the risk of errors. This reusability of test scripts increases efficiency.
Hybrid Testing
Hybrid testing combines the best of both data-driven and keyword-driven testing. It's like having a recipe (keyword-driven) that you can try with different ingredients (data-driven). This approach offers maximum flexibility and reusability. You can use keywords to define the actions in your tests and then use data-driven techniques to run those tests with various inputs. This combination allows for comprehensive test coverage while minimizing redundancy. You can create reusable test scripts that are easily adaptable to different data sets. This makes hybrid testing a powerful approach for complex testing scenarios where you need both flexibility and efficiency. Learn more about the benefits of hybrid testing.
Cross-Browser Testing
In today's multi-device world, ensuring your web application works seamlessly across different browsers is crucial. Cross-browser testing does exactly that. It verifies that your application functions correctly across various browsers like Chrome, Firefox, Safari, and Edge, and on different operating systems like Windows, macOS, and iOS. This type of testing helps identify compatibility issues that might arise due to variations in browser rendering engines or JavaScript implementations. Automated cross-browser testing tools can simulate user interactions across various browsers, providing valuable insights into how your application performs in different environments. These tools can automate tasks like clicking buttons, filling out forms, and navigating between pages, allowing you to test a wide range of user scenarios efficiently. This helps you catch browser-specific bugs early and ensure a consistent user experience for everyone.
Automated UI Testing: A Practical Guide
Automated UI testing focuses on your application's user interface, ensuring it behaves as expected across different devices, browsers, and screen sizes. Tools like Selenium automate these tests, simulating user interactions like clicking buttons and navigating pages. This ensures a consistent user experience across various platforms and helps catch visual bugs and usability issues before they impact your users. Learn how MuukTest can enhance your UI testing.
Unit Testing's Place in Your Testing Strategy
Unit testing, typically performed by developers, acts as the first line of defense against bugs. By creating small, focused tests for individual code units, developers can prevent regressions and build confidence in their code changes. It's not a separate activity but an integral part of the development process, much like regularly checking your work as you write. Automated unit testing is especially valuable because it provides rapid feedback, allowing developers to catch and fix errors quickly. This early detection significantly improves the overall quality of the software and reduces the time and cost associated with fixing bugs later in the development cycle. It sets the foundation for a more robust and reliable software product.
QA's Evolving Role in Test Automation
Automated testing significantly impacts a QA professional's role, shifting it from primarily manual testing toward a more strategic and technical approach. It's not about being replaced by robots, but about working with them. Think of automation as augmenting QA's capabilities, freeing them to focus on higher-level tasks and contribute more strategically to software quality.
While automation handles repetitive tasks like regression testing, QA professionals can dedicate more time to exploratory testing. This involves creatively exploring the software to uncover unexpected issues and edge cases that automated tests might miss. It's about thinking outside the scripted scenarios and understanding how the software behaves in real-world situations. As Cprime points out, this shift allows QA to find unusual issues and contribute a deeper understanding of user experience.
Managing and maintaining automated testing systems also becomes a key responsibility. This includes developing and refining test scripts, integrating tests into the CI/CD pipeline, and analyzing test results. QA professionals become experts in using automation tools and frameworks, ensuring the tests remain effective as the software evolves. This technical expertise is crucial for maximizing the benefits of automation and ensuring comprehensive test coverage. Services like those offered by MuukTest can help streamline this process, providing expert QA support and integration with CI/CD workflows.
Collaboration with developers also becomes more crucial. Automated tests provide rapid feedback on the impact of code changes. This quick feedback loop fosters a more collaborative environment where developers and QA work closely together to identify and resolve issues early. This shared responsibility for quality leads to more efficient development and faster iteration, allowing teams to deliver working software more quickly. This also provides a safety net, giving developers more confidence to make changes and refactor code without fear of introducing new bugs, as noted by Qodo.
When to Automate Your Tests
While unit tests are fundamental, automated testing offers significant advantages beyond verifying individual components. Understanding when to use automation for other testing types, like integration, system, and UI tests, can dramatically improve your overall testing strategy. Here are some key scenarios where automation shines:
- Repetitive Tasks: Automating repetitive tests, such as regression tests, frees your team from tedious, repeated tasks. This not only saves time and resources but also reduces the risk of human error, which is more likely in monotonous tasks. Think of it like using a robot vacuum—it handles the mundane floor cleaning, allowing you to focus on other things.
- Non-Functional Testing: Non-functional testing, including performance and load testing, is often complex and resource-intensive. Automation excels here. Tools can simulate thousands of users interacting with your application, providing valuable insights into its performance under stress. This helps ensure your application meets performance benchmarks under various conditions, as highlighted in this article comparing unit testing and automation testing.
- Cross-Platform Testing: If your application needs to work seamlessly across different browsers, operating systems, or devices, automated cross-platform testing is crucial. Automated tools like Selenium help verify compatibility and ensure a consistent user experience, regardless of the platform. This is especially important for web applications, where browser compatibility can be a significant challenge. For more on cross-platform testing with Selenium, check out this article on unit testing frameworks.
- Testing Main Workflows: Automating tests for your application's core workflows—the critical paths users take to achieve key tasks—provides continuous assurance that these essential functions remain operational as your codebase evolves. This approach prevents regressions in critical areas and maintains a stable user experience. This article further explains the benefits of automating these main workflows.
- Parallel Testing: Automation enables parallel testing, running multiple tests simultaneously across different environments. This significantly speeds up the testing process, especially beneficial for large, complex applications. Parallel testing drastically reduces the overall testing time, allowing for faster feedback and quicker releases. Learn more about parallel testing here.
Best Practices for Automated Unit Testing
Solid unit tests are the foundation of a robust application. Here are some best practices to make your automated unit testing strategy more effective:
Writing Crystal-Clear Test Cases
Keep your tests concise and laser-focused. Each test should verify a single, specific aspect of your code. This clarity makes it easier to understand the purpose of each test and pinpoint the source of any failures, simplifying debugging and maintenance.
Maintaining Independent Tests
Design your unit tests to run independently of each other. The outcome of one test should never influence another. This independence ensures that tests can run in parallel and in any order, crucial for efficient automated testing. This practice also isolates issues when a test fails. This discussion offers further insights into the relationship between automated and unit testing.
Keep Your Test Suites Up-to-Date
Your test suite is a living document. Just as your code evolves, so should your tests. Regularly update them to reflect changes in your codebase. This ensures your tests remain relevant and effective in catching regressions, keeping your safety net strong.
Test-Driven Development (TDD): An Introduction
Consider integrating Test-Driven Development (TDD) into your workflow. In TDD, you write tests before you write the code. This approach encourages cleaner code design and more comprehensive testing from the outset. It's like having a blueprint before building.
Effective Mocking in Unit Tests
When testing individual units of code, you might encounter dependencies on other parts of your system. Mocking lets you isolate the unit under test by simulating these dependencies. Using mock objects helps you focus on the specific behavior of the component you're testing, without the complexities of its interactions with other system parts.
Test Frequently for Best Results
The power of automated unit testing isn’t a one-time fix—it’s an ongoing process. The more test cases you create and the more often they run, the more effective they are. A robust suite of frequently executed unit tests creates a safety net, catching bugs quickly and maintaining a healthy codebase. Qodo emphasizes this point, stating that more test cases and higher frequency lead to greater benefits.
Integrating automated tests into a CI/CD pipeline maximizes test frequency. Every code change triggers automatic test execution, providing immediate feedback. This rapid feedback loop is crucial for catching regressions early and maintaining a fast development pace. Cprime highlights how this immediate feedback enables more efficient development and faster iteration, giving developers the confidence to make changes knowing potential issues will be flagged promptly.
Overcoming Unit Testing Challenges
Automated unit testing, while beneficial, presents some common hurdles. Understanding these challenges and their solutions is key to implementing a successful testing strategy.
Managing Complex Test Dependencies
Dependencies between units can make testing complex. Isolating a unit for testing becomes difficult when it relies heavily on other components. One effective solution is to use mock objects. Mocking frameworks create simulated versions of dependencies, allowing you to control their behavior and focus your tests on the isolated unit. This simplifies testing and makes it easier to pinpoint issues. Another approach involves dependency injection, which provides a way to manage and provide dependencies to a unit during testing, promoting flexibility and maintainability.
Handling Test Data Effectively
Creating and managing test data can be time-consuming. Manually generating data is not only tedious but can also lead to inconsistencies. Automating test data generation with specialized tools ensures consistency and reliability. Consider using factories or builders to create test data objects, making your tests more readable and easier to maintain. For complex data scenarios, consider data-driven testing, where test data is separated from the test logic, allowing you to run the same tests with different data sets.
Balancing Test Coverage and Execution Time
Achieving comprehensive test coverage is essential, but it can lead to long execution times. Finding the right balance is crucial. Prioritize testing critical paths and functionalities first. Focus on areas of the code that are most prone to errors or have undergone recent changes. Tools like MuukTest can help you achieve comprehensive testing efficiently. Setting realistic expectations, as suggested by Testrigor, is also important. Don't aim for 100% coverage immediately; gradually increase coverage as your project evolves.
Overcoming Time Constraints and Learning Curves
Implementing automated unit testing requires an initial time investment. Developers need to learn new tools and frameworks, write test cases, and integrate testing into the workflow. This can be challenging, especially in projects with tight deadlines. Start with small, manageable steps. Focus on training your team on the chosen tools and best practices. Begin by automating tests for critical components and gradually expand coverage. Using a service like MuukTest can help streamline the process. Remember that while there's an upfront cost, automated testing ultimately saves time and resources by catching bugs early and reducing debugging efforts. Addressing the challenges of automation testing strategically is key to long-term success.
Integrating Automated Unit Testing into Your Workflow
Integrating automated unit testing seamlessly into your development workflow is key to realizing its full potential. It's not about writing tests in isolation; it's about weaving them into how you build and maintain software. This involves continuous integration, thoughtful code reviews, and a commitment to measuring and improving test coverage.
Continuous Integration and Automated Testing
Automated testing thrives in a continuous integration (CI) environment. CI systems automatically build and test your code every time you commit changes, providing rapid feedback and catching integration issues early. While we often assume automated testing implies full automation, the reality is more nuanced. Even with unit tests, some CI pipelines might require manual steps. Think of it as a spectrum: you can have fully automated unit tests triggered by each code push, or a semi-automated setup where a developer manually initiates the tests within the CI pipeline. The key is to find the right balance for your team and project. Services like MuukTest's test automation can help automate your builds and tests, freeing you to focus on coding.
CI/CD Pipeline Automation: Best Practices
Automated testing thrives in a continuous integration (CI) environment. CI systems automatically build and test your code with each commit, providing rapid feedback and catching integration issues early. While we often think of automated testing as fully automated, the reality is more nuanced. Even with unit tests, some CI pipelines might incorporate manual steps. It's more of a spectrum: you might have fully automated unit tests triggered by every code push, or a semi-automated setup where a developer manually initiates the tests within the CI pipeline. Finding the right balance for your team and project is key. This rapid feedback loop allows for more efficient development and faster iteration.
Setting Up Automated Unit Testing in CI/CD
Automated testing thrives in a continuous integration (CI) environment. CI systems automatically build and test your code every time you commit changes, providing rapid feedback and catching integration issues early. This rapid feedback loop significantly reduces debugging time and enables faster releases. Integrating automated unit testing into your CI/CD pipeline seems like it should be automatic, right? Not quite.
While the benefits are clear, setting up this automation requires specific configuration. It's not inherently automatic out-of-the-box. As pointed out in this Reddit discussion, configuration is key. While we often think of automated testing as fully automated, the reality is more nuanced. Even with unit tests, some CI pipelines might require manual steps. MuukTest's guide on test automation services acknowledges this, explaining that finding the right balance of automation within your CI/CD pipeline depends on your specific project needs.
It's more of a spectrum: you might have fully automated unit tests triggered by every code push, or a semi-automated setup where a developer manually initiates the tests within the CI pipeline. The key is to choose the level of automation that best suits your team and workflow. So, what does configuring automated unit testing in your CI/CD pipeline actually involve? It depends on your specific CI/CD platform (like Jenkins, GitLab CI/CD, or CircleCI) and your chosen testing framework.
Generally, you'll need to define specific steps within your pipeline configuration. These steps might include installing necessary dependencies, building your project, running your unit tests, and reporting the results. For example, in a YAML-based CI/CD configuration, you might have a dedicated test
stage that specifies the commands to execute your unit tests. This stage would typically run after the build
stage, ensuring that tests are run against the latest compiled code. The specifics of these commands will depend on your testing framework. For instance, if you're using JUnit, you'll likely use a command like mvn test
to execute your tests.
Code Review and Unit Testing: Better Together
Code reviews and unit testing are powerful allies. Unit testing, performed by developers, involves small, focused tests that verify individual units of code. These tests act as a safety net, preventing regressions and building confidence in code changes. When integrated with code reviews, unit tests provide concrete evidence that the code functions as expected. Reviewers can then focus on the overall design, logic, and maintainability of the code, rather than verifying basic functionality. This collaborative approach, where developers write tests and reviewers assess both the code and the tests, leads to higher quality and more robust software. Check out MuukTest's customer success stories to see how this works in practice.
Measure and Improve Your Test Coverage
Simply having unit tests isn't enough; you need to understand how effectively they cover your codebase. Measuring test coverage—the percentage of your code executed during testing—provides valuable insights into potential gaps and vulnerabilities. While 100% coverage is often impractical, aiming for high coverage in critical areas of your application is a good practice. Regularly reviewing and analyzing your test coverage helps you identify areas needing additional tests, ensuring your tests evolve alongside your code. Understanding the challenges of automation testing, such as managing test data and balancing coverage with execution time, is crucial for an effective testing strategy. For a quick start, explore MuukTest's QuickStart guide and pricing options.
MuukTest: Streamlining Your Test Automation
Building and maintaining a robust suite of automated unit tests can be challenging. It requires dedicated resources, expertise in testing frameworks, and ongoing maintenance. This can feel like a whole separate project in itself! That's where MuukTest comes in. We specialize in helping businesses like yours achieve comprehensive test coverage quickly and efficiently.
Comprehensive Test Coverage with MuukTest
We understand the importance of thorough testing. Our AI-powered platform analyzes your codebase to identify critical paths and potential vulnerabilities, ensuring your tests cover the most important parts of your application. This focus on comprehensive test coverage helps you catch more bugs early, reducing the risk of unexpected issues in production. MuukTest is designed to provide complete test coverage within 90 days, giving you confidence in your software's quality and stability.
Accelerated Testing with AI-Powered Automation
Time is of the essence in software development. MuukTest's AI-powered automation accelerates the testing process, providing rapid feedback on code changes. Our platform automatically generates and executes tests, freeing your team from tedious manual tasks and allowing them to focus on building new features. This accelerated testing process, combined with our focus on early bug detection, helps you deliver high-quality software faster.
Just as automated unit testing acts as a dedicated quality assurance team examining each part of your code, MuukTest's AI-powered automation enhances this process. Catching errors early reduces the cost of fixes, as explained by Qodo. This allows your team to iterate more quickly and efficiently.
Seamless Integration with Your CI/CD Workflow
Integrating automated testing into your existing CI/CD pipeline is crucial for continuous quality assurance. MuukTest seamlessly integrates with popular CI/CD platforms, allowing you to automate your entire testing workflow. This integration ensures that tests run automatically with every code change, providing immediate feedback and preventing regressions. This allows your team to maintain a consistent and reliable codebase.
As Cprime notes, while the benefits of CI/CD integration are clear, setting up this automation can be complex. MuukTest simplifies this process, providing a streamlined integration experience that minimizes disruption to your existing workflow.
Getting Started with MuukTest
Ready to streamline your test automation and improve software quality? Getting started with MuukTest is easy. Our QuickStart guide provides a step-by-step walkthrough of the onboarding process. We also offer a range of pricing plans tailored to different business needs. Explore our test automation services and see how we can help you achieve complete test coverage efficiently and cost-effectively.
Getting the Most Out of Unit Testing
The more you test, the more you find. This fundamental principle of software testing highlights the increasing value of thorough testing. The value of unit testing increases with the number of test cases, how often you run them, and your level of automation. A single test might catch a major flaw, but a comprehensive suite of automated tests, run frequently, will uncover subtle issues that might otherwise be missed. This proactive approach to finding bugs early, when they're easier and cheaper to fix, leads to more robust and dependable software. By verifying each component works correctly in isolation, you build a solid foundation for your application. This minimizes the risk of bugs spreading through the system and causing larger problems. MuukTest understands this and focuses on building comprehensive automated unit testing solutions.
Unit Testing in DevOps
Automated unit testing is a crucial component of a modern DevOps environment. DevOps emphasizes rapid, continuous delivery, and automated testing provides the necessary safety net. Automated tests are particularly valuable in a continuous integration (CI) environment, where code changes are frequently integrated and automatically tested. This rapid feedback loop catches integration issues early, ensuring that every change contributes to a stable and functional product. Developers write focused unit tests to verify individual pieces of code, creating a safety net against regressions and building confidence in every change. This safety net is invaluable in a fast-paced DevOps workflow, allowing for speed without sacrificing quality. MuukTest specializes in integrating automated unit testing seamlessly into CI/CD workflows, empowering teams to deliver high-quality software quickly.
Getting Started with Automated Unit Testing
So, you're ready to dive into automated unit testing? Great! This section provides a practical roadmap to get you started. We'll cover choosing the right tools, setting up your environment, and finding valuable learning resources.
Streamlining Test Automation with MuukTest
MuukTest offers AI-powered test automation services designed to provide comprehensive test coverage efficiently and cost-effectively. We understand the challenges of building and maintaining a robust test suite. From managing complex dependencies to balancing coverage with execution time, testing can feel like a constant uphill battle. That's why we've developed a streamlined approach that leverages AI to accelerate the process and maximize your return on investment.
We can help you achieve complete test coverage within 90 days, significantly improving your testing efficiency. This rapid turnaround time allows you to release software faster and with greater confidence. Our services encompass a range of testing needs, including unit, integration, and end-to-end testing. We work closely with your team to understand your specific requirements and tailor our solutions to fit your needs.
Whether you're looking to improve code quality, reduce technical debt, or accelerate your release cycles, MuukTest can help. We provide expert QA support, seamless integration with your existing CI/CD workflows, and scalable solutions that grow with your business. By partnering with MuukTest, you can free up your development team to focus on building great software, while we ensure its quality and reliability. Learn more about our services and how we can help you streamline your test automation process.
Unit Testing Automation with MuukTest
Unit testing automation with MuukTest can significantly enhance your software development process. By leveraging AI-powered automated unit testing, MuukTest helps developers catch bugs early, ensuring each component of your code functions correctly in isolation. This proactive approach leads to more robust and dependable software. It also integrates seamlessly into your continuous integration (CI) environment, providing rapid feedback and catching integration issues early. MuukTest focuses on comprehensive test coverage, empowering teams to uncover subtle issues that might otherwise be missed. This ultimately improves software quality and reduces development costs. Learn more about how MuukTest helps you achieve complete test coverage within 90 days, significantly enhancing your testing efficiency. For a deeper understanding of how automated unit testing contributes to more efficient and higher-quality software development, explore resources like this Cprime article on unit testing and test automation.
Comprehensive Testing with MuukTest
Building robust software requires a comprehensive testing strategy, and automated unit testing is essential. But setting up and maintaining a thorough testing system can be complex and eat up valuable time. MuukTest specializes in helping businesses achieve comprehensive test coverage efficiently and cost-effectively.
Achieve Complete Test Coverage with MuukTest
MuukTest uses AI-powered test automation to create and execute tests, covering a wide range of scenarios and edge cases. Our approach goes beyond simply checking individual units of code; we integrate with your existing CI/CD workflows, providing continuous feedback and ensuring your tests run seamlessly as part of your development process. This helps you catch bugs early, accelerate development, and reduce costs. We aim to achieve complete test coverage within 90 days, giving you confidence in your software's quality and reliability. Learn more about how MuukTest works.
Why Choose MuukTest?
Partnering with MuukTest offers several key advantages. Our expert QA team brings years of experience, ensuring your tests are designed and executed effectively. We tailor our scalable solutions to your specific needs, whether you're a small startup or a large enterprise. By automating the tedious aspects of testing, we free up your developers to focus on building great software. Our transparent pricing makes it easy to understand the value we provide. See how MuukTest has helped other customers achieve their testing goals.
Getting Started with MuukTest
Ready to experience the benefits of comprehensive test automation? Getting started with MuukTest is simple. Our QuickStart guide provides a step-by-step process to integrate MuukTest into your existing workflow. We also offer personalized onboarding and support to ensure a smooth transition. Contact us today to learn more about how MuukTest can help you achieve complete test coverage and build better software.
Select the Right Automated Unit Testing Tools
Selecting the right tools is the first step. Your choice often depends on your programming language and project requirements. Thankfully, there are plenty of robust and well-supported frameworks available. If you're working with Java, JUnit is a popular choice. For .NET developers, NUnit is a go-to framework. Python developers often prefer the simplicity and extensibility of pytest. These frameworks provide the structure for writing tests and offer features like assertions, test runners, and reporting. They also integrate well with CI/CD pipelines, which we'll discuss later. For cross-platform testing, consider tools like Selenium, which is particularly useful for web applications. Choosing the right toolset can significantly impact your testing efficiency.
Setting Up Your Testing Environment
With your tools selected, the next step is setting up your testing environment. This involves configuring your development environment to work seamlessly with your chosen testing framework. Make sure you've correctly managed any project dependencies and integrated the framework with your build system. A well-configured environment ensures that your tests run consistently and reliably. Consider using containerization tools like Docker to create isolated and reproducible testing environments. This helps eliminate inconsistencies that can arise from differences in development environments across your team. Proper environment setup is key to smooth and effective testing.
Find Helpful Learning Resources
Learning automated unit testing is an ongoing process. There are tons of resources available to help you along the way. Online tutorials, comprehensive documentation, and active community forums are excellent starting points. Websites like Stack Overflow and GitHub offer a wealth of information and practical examples. For more structured learning, explore online courses dedicated to unit testing practices and specific tools. Remember, becoming proficient in automated unit testing takes time and practice, so be patient with yourself and keep learning. Continuous learning is essential for staying up-to-date with the latest testing techniques and best practices.
Frequently Asked Questions
Why should I bother with automated unit testing? It seems like extra work.
I get it – adding testing to your workflow might feel like adding more to your plate. However, think of automated unit testing as a proactive way to prevent future headaches. By catching bugs early, you actually save time and effort in the long run. Fixing a small bug in an isolated unit is much easier than debugging a complex, integrated system later on. Plus, having a solid suite of tests gives you the confidence to refactor and improve your code without fear of breaking things.
How much automated unit testing is enough? Do I need to test every single line of code?
While 100% test coverage sounds ideal, it's not always practical or necessary. Focus on testing the critical parts of your application – the core functionality, areas prone to errors, and parts that have undergone recent changes. Start with the most important areas and gradually increase coverage as your project evolves. Finding the right balance between coverage and execution time is key.
What's the difference between unit testing and integration testing?
Unit testing focuses on individual components in isolation, like testing individual gears in a clock. Integration testing, on the other hand, checks how these components work together, ensuring the gears mesh correctly when assembled. Both are important, but they serve different purposes. Unit tests catch errors early within individual units, while integration tests identify problems that arise from the interaction between units.
Which unit testing framework should I use? There are so many options!
The best framework for you depends on your programming language and project needs. If you're working with Java, JUnit is a solid choice. NUnit is popular for .NET developers, while pytest is a favorite among Python users. There are also cross-platform tools like Selenium for web application testing. Do some research and see which framework best fits your specific requirements.
How do I integrate automated unit testing into my existing workflow?
Start small and focus on gradual integration. Begin by adding tests for new code and gradually incorporate tests for existing critical components. Integrate your tests into your continuous integration/continuous delivery (CI/CD) pipeline so they run automatically with each code change. Make testing a regular part of your code review process. Over time, automated testing will become a seamless part of your development cycle.
Related Articles
- A Practical Guide to Automated Unit Testing
- Why Unit Testing is a Foundation for Software Quality
- 6 Unit Testing Best Practices for an Effective QA Strategy
- Your Complete Guide to Automated UI Testing
- Automated Testing Tools: Your Ultimate Guide
Key Takeaways
- Early bug detection is key: Finding and fixing problems early in the development cycle is significantly cheaper than addressing them later. Automated unit testing acts as your first line of defense, meticulously examining each part of your code and preventing small issues from snowballing into major problems.
- Unit tests as living documentation: Unit tests serve as clear documentation of how each component should function. This documentation helps prevent errors during code changes and improves overall maintainability.
- Proactive quality assurance: Automated unit testing, using software tools to automatically test small parts of your code, like individual functions, is a proactive approach to quality assurance. This approach is essential for building reliable and maintainable software.
- Rapid feedback loops: Automated tests provide rapid feedback on the impact of code changes. This rapid feedback fosters a more efficient development process and faster iteration, allowing you to confidently make changes, knowing that automated tests will immediately flag any unintended consequences.
Additional Resources
- Choosing the Right Tools: Selecting appropriate tools and frameworks is crucial for effective automated unit testing. Your choices depend on factors like your programming language, project requirements, and team expertise. Consider factors like community support, ease of use, and integration with your existing development environment.
- Comprehensive Test Coverage: For comprehensive test coverage quickly and efficiently, consider exploring AI-powered test automation services like those offered by MuukTest. We can help you achieve complete test coverage within 90 days, significantly enhancing your testing efficiency.
- Understanding Unit Testing Automation: Automated unit testing verifies that individual units or components of code work correctly. This method is a proactive approach to quality assurance and is essential for building reliable and maintainable software.
- Benefits of Automated Unit Testing: Automated unit testing offers several advantages that contribute to more efficient and higher-quality software development. Explore the benefits further to understand how automated unit testing can improve your development process.
Related Posts:
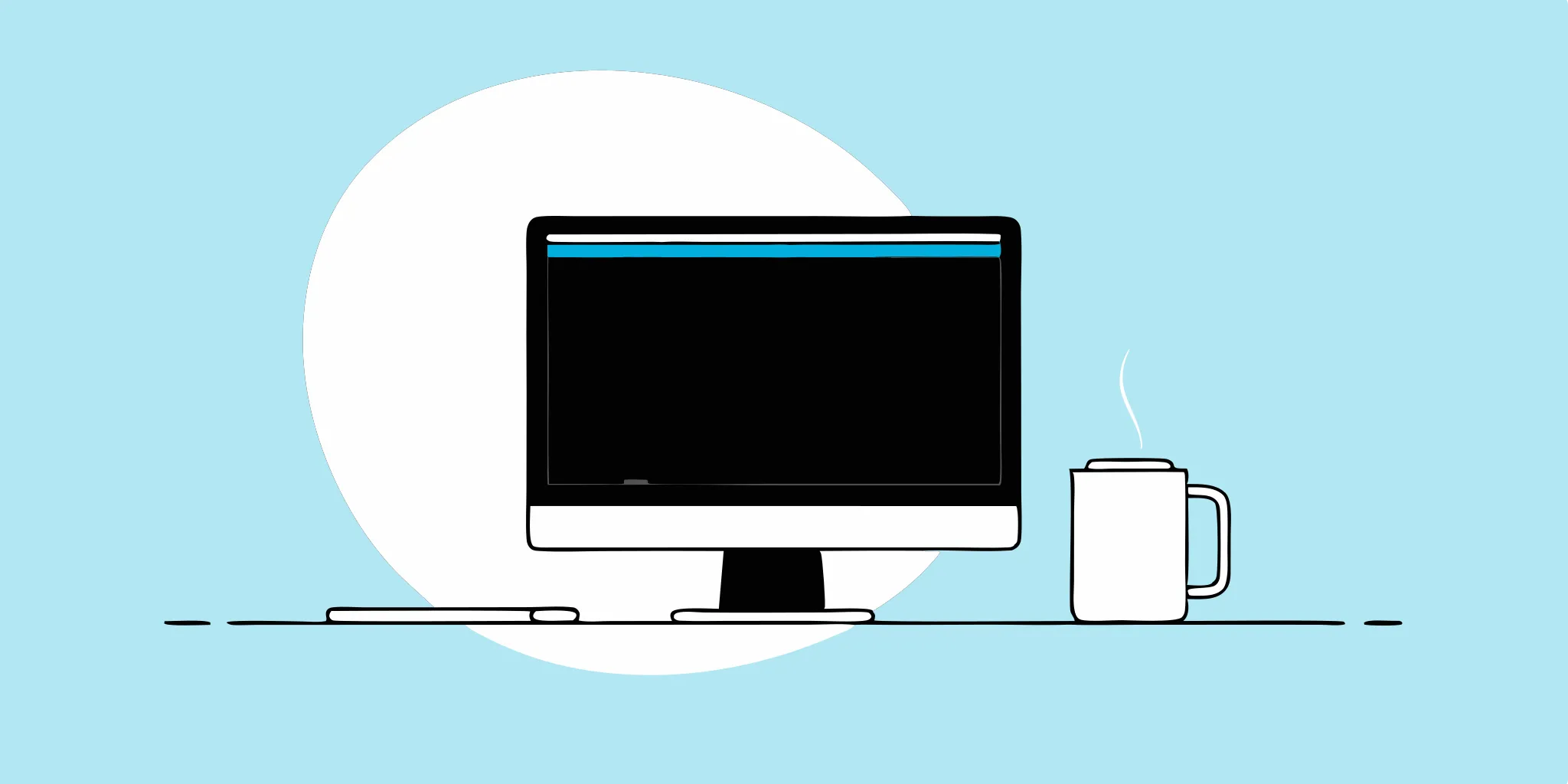
What is Unit Testing? A Practical Guide for Developers
Let's be honest, debugging is nobody's favorite activity. It's time-consuming, frustrating, and can often feel like searching for a needle in a haystack. What if you could prevent many of those bugs...
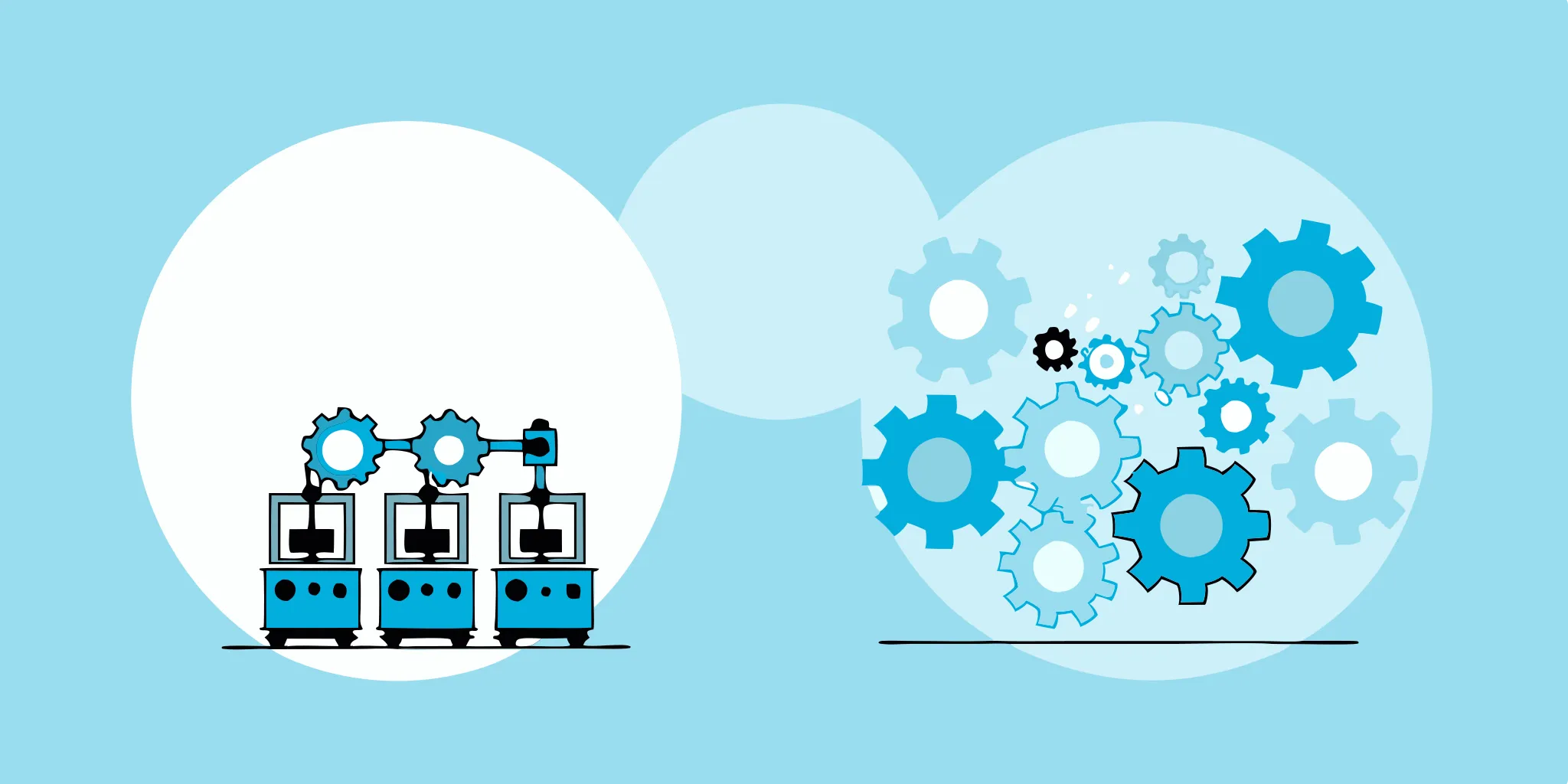
Verify Code Functions Correctly: Unit & Integration Testing
Learn how to verify that the units or pieces of code function correctly when integrated. Explore practical tips for effective unit and integration testing.
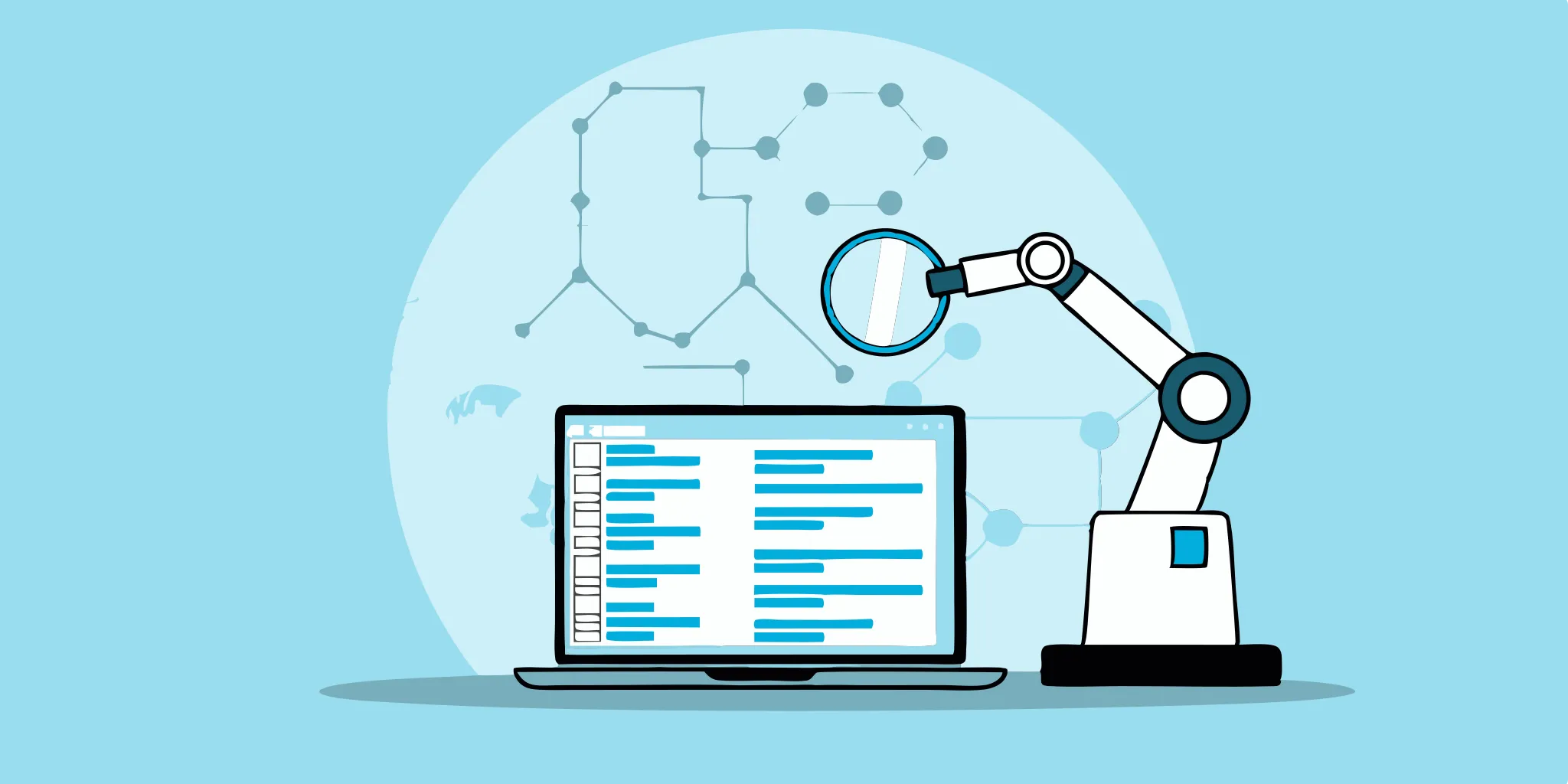
Automated Testing in Quality Assurance: A Practical Guide
Master automated testing in quality assurance with this comprehensive guide, covering benefits, strategies, and tools to enhance your software development process.