What is Unit Testing? A Practical Guide for Developers
Author: The MuukTest Team
Published: March 19, 2025
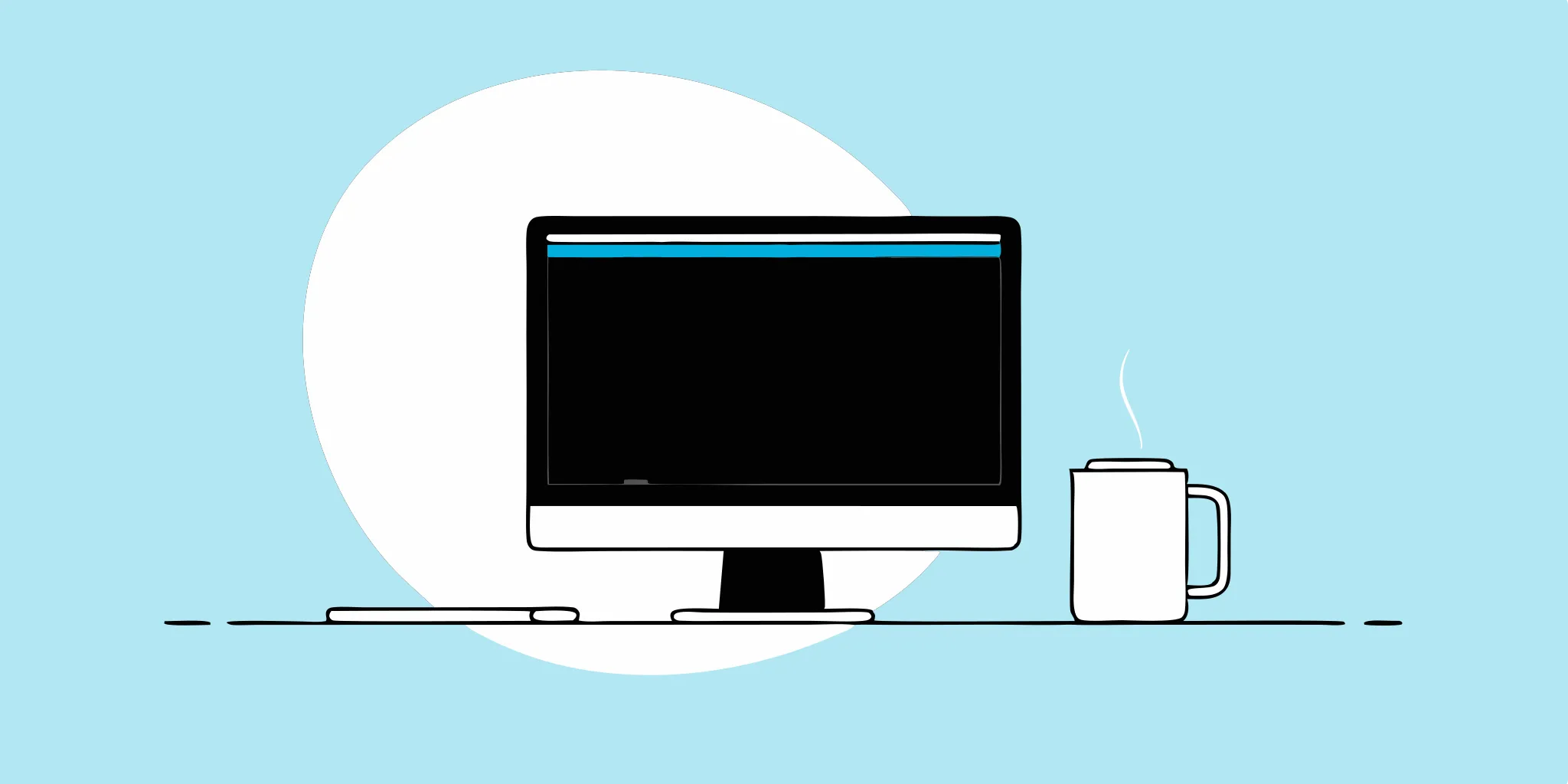
Table of Contents
Let's be honest, debugging is nobody's favorite activity. It's time-consuming, frustrating, and can often feel like searching for a needle in a haystack. What if you could prevent many of those bugs from ever appearing in the first place? That's the power of unit testing. If you're wondering, "What is unit testing?", this guide is for you. We'll break down the concept, explain its benefits, and show you how to implement it effectively in your workflow. We'll cover everything from writing clear test cases to automating your tests and integrating them with your CI/CD pipeline. Plus, we'll share how MuukTest can help you achieve comprehensive test coverage within 90 days, freeing up your time to focus on building great software, not chasing bugs.
Key Takeaways
- Unit testing is essential for high-quality software: Testing individual components helps you find bugs early, leading to more robust and maintainable code. This proactive approach saves time and headaches down the line.
- Strategic unit testing maximizes impact: Write focused, independent tests, automate your testing efforts, and don't neglect edge cases. Combine unit testing with other methods like integration and functional testing for a comprehensive testing strategy.
- Unit testing is an investment, not a cost: While it requires an initial time commitment, unit testing ultimately saves you time and resources by preventing larger issues later. Use tools like MuukTest to streamline your testing and achieve complete test coverage efficiently.
What is Unit Testing?
Definition and Purpose
Unit testing is a software testing method where you isolate and test individual units or components of your software. Think of it like testing the individual Lego bricks of a large castle—you're making sure each piece works perfectly on its own before assembling the whole structure. This helps catch problems early in the development cycle, when they're much easier and less expensive to fix. This early bug detection speeds up your overall testing strategy and prevents wasted time on later, more complex tests. At MuukTest, we understand the value of early testing and incorporate it into our process to deliver comprehensive test coverage within 90 days. Learn more about our test automation services to see how we can help you achieve similar results.
Key Components
Effective unit tests share a few key characteristics. They're small and focused, providing granular information about your code's behavior and running much faster than larger, more complex tests. Each test case should ideally check only one specific aspect of a unit's functionality. This focused approach makes it easier to pinpoint the source of any issues. Finally, unit tests operate in isolation. You're testing each piece of code independently, without considering its interactions with other system parts. This isolation ensures you're truly validating the unit's core logic, not getting sidetracked by external factors. This isolated approach sets the stage for more comprehensive testing later on, like integration testing, where you examine how different units work together. Ready to get started? Check out our QuickStart guide to implement unit testing in your workflow today.
Why Unit Testing Matters
Unit testing offers several compelling advantages for software development. It's a cornerstone of robust and maintainable code. Let's explore some key reasons why you should incorporate unit testing into your workflow.
Find Bugs Early
Think of unit testing as your first line of defense against bugs. By testing individual components in isolation, you can identify and fix issues before they become larger, more complex problems. This early detection saves you time and headaches and contributes to a higher-quality end product. As highlighted by DZone, finding bugs early is a primary benefit of unit testing, preventing them from impacting other parts of your codebase. This early intervention keeps your project on track and minimizes the risk of unexpected issues later on.
Improve Code Quality
Unit testing isn't just about finding bugs; it's about improving the overall quality of your code. When you write unit tests, you're forced to think about your code's design and how different components interact. This process naturally leads to more modular, well-structured code that is easier to understand and maintain. Unit testing performed by developers before integration allows for early issue detection and resolution, ultimately enhancing code quality. This focus on quality from the start makes your codebase more robust and easier to work with.
Simplify Maintenance and Refactoring
Codebases are constantly evolving. Unit tests provide a safety net for making changes and refactoring your code with confidence. Knowing you have tests in place to catch regressions allows you to confidently improve your code over time. Unit testing makes refactoring safer, enabling developers to make changes and improvements without fear of introducing new bugs. This makes your codebase more flexible and adaptable to future needs, allowing you to respond to changing requirements more effectively.
How Unit Testing Works
Unit testing boils down to checking small parts of your code in isolation. Think of it like testing individual Lego bricks before using them to build an elaborate castle. This approach helps you catch problems early on, before they become larger issues. Let's break down the process:
Write Test Cases
This stage involves creating small, focused tests for specific functions or modules within your code. Each test case should target a particular behavior or functionality, ensuring it performs as expected. For example, if you have a function that calculates the area of a rectangle, your test cases might include checking the area for various dimensions, including edge cases like zero or negative values. This granular approach helps pinpoint the exact location of any defects. Unit testing focuses on verifying the smallest parts of your code.
Execute Tests
Once you've written your test cases, the next step is running them. This typically involves using a unit testing framework, which we'll discuss later. The framework executes your tests and reports the results. The beauty of unit tests is their speed and precision. Because they're small and isolated, they run quickly, providing immediate feedback. Smaller tests offer more detailed insights into how your code behaves. This rapid feedback loop helps you identify and address issues quickly.
Test-Driven Development (TDD)
Test-driven development (TDD) flips the traditional development script. Instead of writing code first and then creating tests, TDD advocates for writing the tests first. This might seem counterintuitive, but it offers powerful advantages. By defining the expected behavior upfront through tests, you clarify your requirements and ensure your code meets them. TDD also simplifies refactoring and makes it easier to maintain your code over time, as AWS explains. This approach can lead to more robust and reliable software.
Best Practices for Effective Unit Testing
Effective unit testing requires more than simply writing tests—it's about writing good tests. Here are some best practices to ensure your unit tests are valuable assets in your development process:
Write Clear Tests
Your unit test code should be clean, readable, and easy to understand. Think of your tests as documentation for your code. A well-written test clearly shows what aspect of the code it's checking and how it should behave. Focus on testing one specific behavior or unit of code per test. If a test asserts on multiple objects, it might be trying to do too much, potentially obscuring issues. Keep your tests concise and focused.
Maintain Test Independence
Each test should stand alone and not affect any other tests. Changes in one test shouldn't cause others to fail. This independence makes it easier to pinpoint the source of problems when tests fail. One way to achieve this is by using mock data. Mocking external dependencies, like databases or network connections, isolates your unit tests and makes them more reliable. This way, you're testing your code's logic, not the stability of your network or the state of your database.
Automate Testing
For larger projects, manual testing quickly becomes unsustainable. Automating your unit tests is essential for efficiency. Consider adopting strategies like Test-Driven Development (TDD), where you write tests before you write the code they're testing. This practice helps clarify requirements and ensures your code meets those requirements from the start. Another approach is Behavior-Driven Development (BDD), which focuses on the desired behavior of the code. Both TDD and BDD can be combined with mocking and stubbing techniques to simulate parts of your system, allowing you to isolate and test specific units effectively.
Cover Edge Cases
While testing the "happy path" is important, don't forget about edge cases. These are the unusual or extreme inputs and scenarios that can often reveal hidden bugs. Think about boundary conditions, invalid inputs, and unexpected situations. Thorough edge case testing helps you build more robust and reliable software. Remember, unit testing is just one piece of the puzzle. While it's great for verifying individual components, you'll also need integration testing to ensure these parts work together correctly.
Popular Unit Testing Frameworks and Tools
Picking the right tools is key for efficient unit testing. Here’s a quick look at some popular unit testing frameworks, broken down by programming language:
JUnit (Java)
JUnit is a widely used testing framework for Java, and it’s been the inspiration for many others. It’s designed specifically for writing and running tests in Java, and provides a simple, structured approach. You'll find a robust set of features, including annotations for test setup and execution, assertions for checking expected outcomes, and test runners for automating the testing process. If your team works with Java, JUnit is a solid option.
Pytest (Python)
For Python developers, Pytest is a popular choice. It’s known for making it easy to write both simple and complex test cases. Pytest supports fixtures, which help streamline test setup and teardown. It also offers a wide range of plugins to extend its functionality. This flexibility makes Pytest a good fit for projects of all sizes.
NUnit (.NET)
NUnit is the go-to unit testing framework for .NET languages. It provides a comprehensive set of tools for writing and running tests, and is designed to be easy to use, even for complex projects. Similar to JUnit, NUnit uses attributes to identify test methods and provides various assertion methods for validating expected results. If you're working in the .NET ecosystem, NUnit is worth exploring.
Jest (JavaScript)
If you're working with JavaScript, especially in a React environment, Jest is a popular and user-friendly testing framework. Developed by Facebook, Jest is designed to be simple and straightforward, while still offering powerful features like mocking, snapshot testing, and code coverage reporting. Its tight integration with React makes it a natural choice for testing React components and applications. You can learn more about Jest and other JavaScript testing tools on the MuukTest website. We can help you get complete test coverage, no matter your chosen framework.
Unit Testing vs. Other Testing Types
While unit testing is foundational for a robust testing strategy, it's not the only piece of the puzzle. Understanding how unit testing fits within the broader testing landscape is crucial for building truly reliable software. Let's look at how it compares to integration and functional testing, and how all three work together.
Integration Testing
Integration testing focuses on how different units or modules of your software interact. Think of it like testing the connections between different parts of a machine. While unit tests ensure each part works in isolation, integration tests verify that those parts function correctly when assembled. For example, an integration test might check the interaction between your user authentication system and your database. This type of testing catches issues that unit tests might miss, such as communication problems between modules or inconsistencies in data handling.
Functional Testing
Functional testing takes a higher-level view, examining the software's behavior from the user's perspective. It checks whether the system as a whole delivers the expected functionality based on specific inputs. Functional tests don't care about the internal workings of the software; they only focus on the outputs. For instance, a functional test might simulate a user logging in, adding items to a shopping cart, and completing a purchase. This type of testing ensures that the entire system works together to meet the user's needs.
Unit Tests in Your Overall Strategy
Unit testing, integration testing, and functional testing each play a vital role in a comprehensive testing strategy. Unit tests form the base, ensuring individual components work correctly. Integration tests build upon this by verifying the interactions between those components. Finally, functional tests validate the overall system behavior from the user's standpoint. This layered approach helps identify bugs early in the development process, leading to higher quality software and reduced development costs. For a deeper dive into unit testing, including its benefits for bug detection, code quality, refactoring, and continuous integration, check out this detailed guide. By combining these testing methods, you create a safety net that catches issues at different levels, resulting in a more robust and reliable product.
Overcome Unit Testing Challenges
Unit testing, while beneficial, presents some common hurdles. Let's explore these challenges and how to address them effectively.
Time and Resources
It's true that writing unit tests requires an initial time investment. However, consider this investment a down payment on future efficiency. Testing during development allows developers to catch and fix issues early. This proactive approach prevents small problems from snowballing into larger, more complex issues later, ultimately saving time and resources. Think of it like this: spending a little extra time upfront on unit tests is like getting regular maintenance on your car—it prevents major breakdowns down the road. MuukTest’s automated testing services can help streamline this process, making the most of your team's time.
Complex Dependencies
Testing complex code with intricate dependencies can be tricky. The challenge lies in isolating individual units for testing. One effective strategy is to use mock objects or stubs. These stand-ins mimic the behavior of real dependencies, allowing you to test a unit in isolation without getting bogged down by external factors. This approach simplifies testing and makes it easier to pinpoint the source of any issues. For handling dependencies in Java, JUnit offers helpful mocking capabilities. If you're working with Python, explore Pytest for its robust mocking features.
Maintaining Test Suites
As your codebase grows, so does your suite of unit tests. Keeping these tests organized and up-to-date can feel like a chore. However, well-maintained tests are crucial for long-term project health. Prioritize writing clear, concise tests that are easy to understand and modify. Automating your testing process can also significantly reduce the burden of maintenance. Tools like MuukTest integrate seamlessly with CI/CD workflows, ensuring that your tests run automatically whenever you make changes to your code. This automation not only saves time but also helps prevent regressions and keeps your codebase stable.
Implement Unit Testing in Your Workflow
Adding unit testing to your development process doesn't have to be a huge undertaking. Start small, build good habits, and gradually expand your test suite. Here’s how to weave unit testing seamlessly into your workflow:
Set Up a Testing Environment
Creating a dedicated testing environment keeps your main codebase clean and helps you manage different testing phases. This isolated environment mimics your production setup, allowing you to catch integration issues early on. Ideally, unit tests operate independently from external systems like databases or networks, focusing solely on the behavior of individual code units This isolation makes tests faster and more reliable. Choose a unit testing framework that aligns with your tech stack—this will provide structure and tools for writing and running your tests. MuukTest offers seamless integration with CI/CD workflows, simplifying the setup and management of your testing environments.
Integrate with CI/CD
Once you have a solid set of unit tests, connect them to your CI/CD pipeline. This automates the testing process, ensuring that every code change is automatically verified. This continuous testing approach helps maintain code quality throughout the development lifecycle. Set up your CI/CD system to trigger your unit tests whenever new code is pushed. This provides immediate feedback and prevents bugs from sneaking into production. Learn more about how MuukTest streamlines this integration on our test automation services page.
Measure and Improve Test Coverage
Tracking your test coverage—the percentage of your code covered by tests—is crucial. While 100% coverage is often impractical, aim for a high percentage in critical areas of your application. Tools within your testing framework can help you measure coverage and identify gaps. Regularly review your test coverage reports to understand which parts of your code need more attention. Unit testing offers several advantages, including early bug detection and easier integration. Prioritize writing tests for complex logic, frequently modified code, and areas prone to errors. This targeted approach maximizes the impact of your testing efforts. MuukTest helps clients achieve comprehensive test coverage within 90 days. Explore our customer success stories to see how. Ready to get started? Check out our quickstart guide for practical tips and resources.
Debunking Unit Testing Misconceptions
Let's clear up some common misconceptions about unit testing. These myths can discourage teams from adopting unit testing, so let's break them down:
"Unit Testing Guarantees Bug-Free Code"
It would be amazing if unit testing was a magic bullet for perfect, bug-free code, but that's not realistic. Think of unit tests as a strong safety net, not an impenetrable force field. They catch many issues early on, but they can't foresee every possible scenario or user interaction. A detailed guide on unit testing points out that while valuable, it's just one piece of a larger testing strategy. You still need other testing methods, like integration and functional testing, for comprehensive coverage.
"Unit Tests Are Only for New Code"
While it's easier to incorporate unit tests into new code from the start, that doesn't mean your existing codebase is a lost cause. You can absolutely apply unit tests to legacy code. It might take a bit more effort, and it can be time-consuming, as explained in this guide to unit testing, but the benefits are worth it. Think of it as giving your older code a health check and improving its maintainability.
"Unit Testing Is Too Time-Consuming"
Yes, writing unit tests takes time. But consider this: how much time do you currently spend debugging and fixing issues later in the development cycle? Often, catching bugs early with unit tests saves you significant time and resources.Testing requires an upfront investment, but they also emphasize the long-term benefits. Think of unit tests as an investment, not a cost. They might seem like extra work now, but they pay off by preventing bigger headaches later. Tools like MuukTest can streamline the process and achieve comprehensive test coverage efficiently.
Frequently Asked Questions
What’s the simplest way to explain unit testing?
Imagine testing individual Lego bricks before building a castle. You check each brick to ensure it's perfect before putting them together. That's unit testing – making sure each small part of your software works correctly on its own.
Why should I bother with unit testing if I’m already doing integration and functional testing?
Think of it like building a house. Unit tests check the bricks, integration tests check how the bricks fit together to make walls, and functional tests check if the whole house stands up. Each test type catches different problems, so you need all three for a truly solid structure.
How do I choose the right unit testing framework?
The best framework depends on your programming language. JUnit is popular for Java, Pytest for Python, NUnit for .NET, and Jest for JavaScript. Consider your team's familiarity and the specific needs of your project.
Our codebase is huge and old. Is it too late to start unit testing?
It's never too late! While it might take some effort, adding unit tests to existing code (even older code) improves its quality and makes future changes easier. Start with the most critical or frequently modified parts.
I’m worried about the time commitment. Is unit testing really worth it?
While unit testing requires an initial time investment, it often saves you time in the long run by catching bugs early. Think of it as preventative maintenance – a little effort upfront prevents major headaches later. Automating your tests can also help streamline the process.
Related Posts:
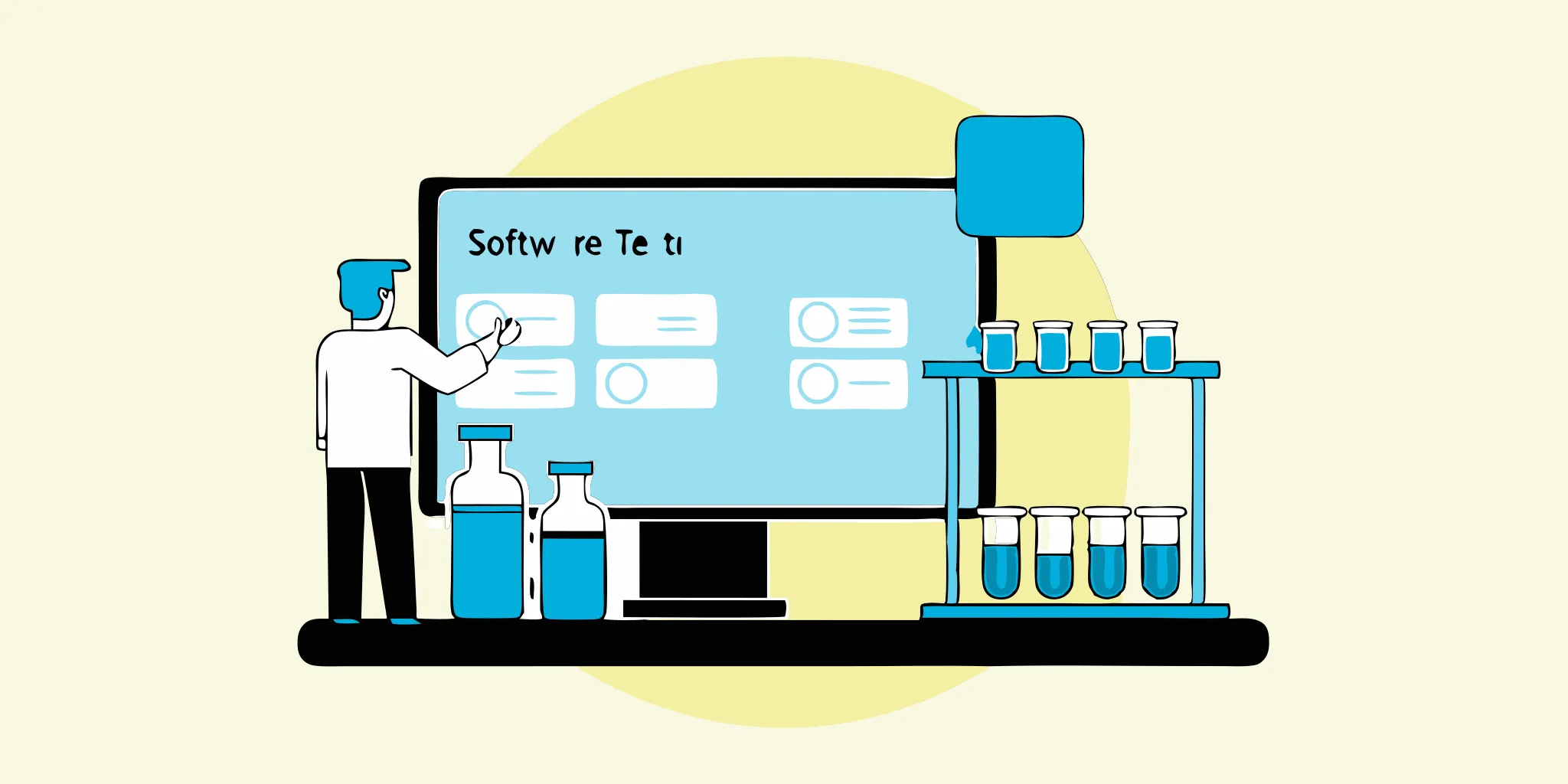
Smoke Test Your Software: A Practical Guide
Learn about smoke test software, its role in development, and how it helps catch critical bugs early. Discover best practices for effective testing.
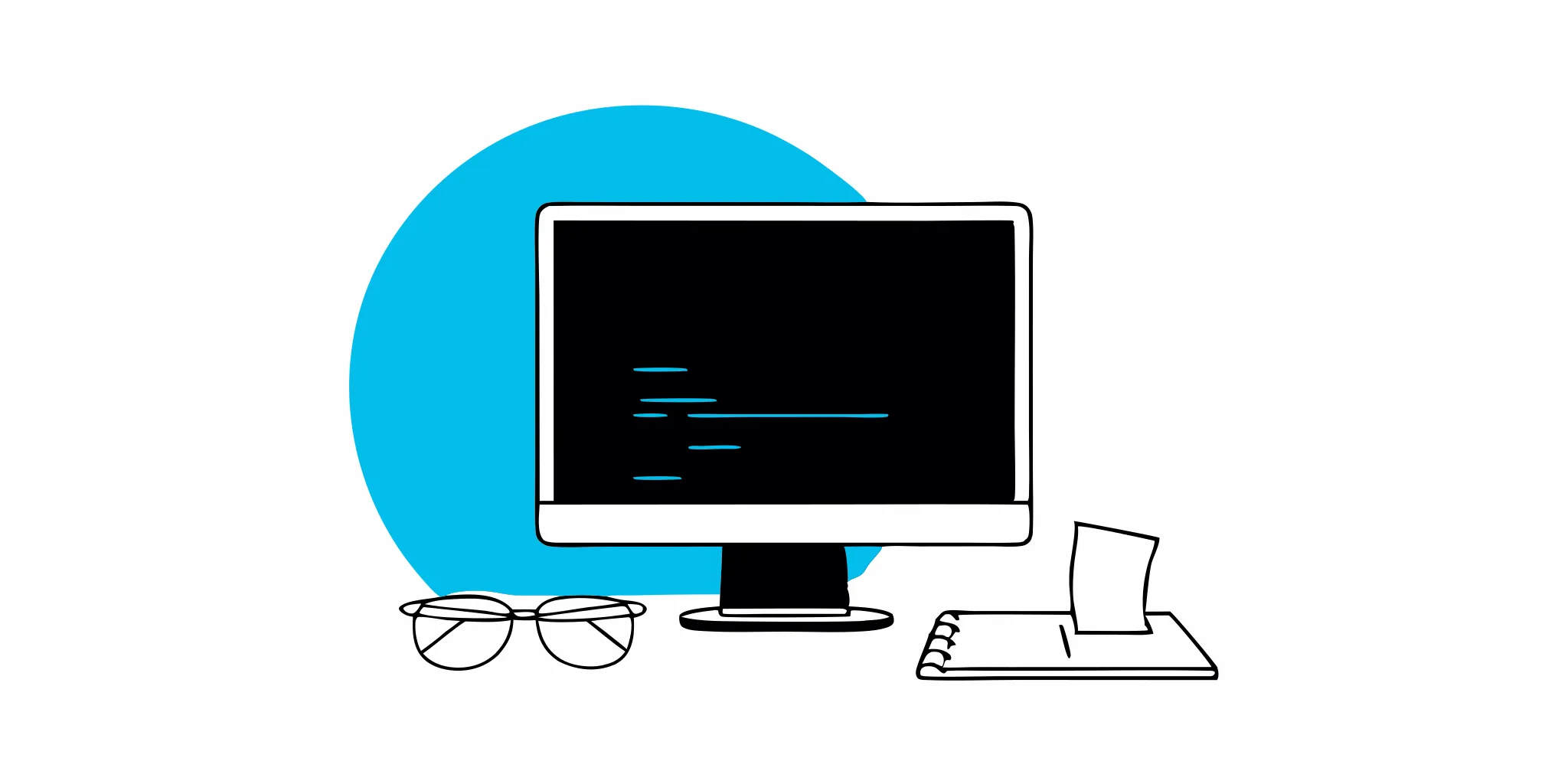
Software Module Testing: A Practical Guide
Building software is a lot like constructing a complex LEGO masterpiece. You wouldn't want to discover halfway through that some of your foundational bricks are faulty, would you? That's where...
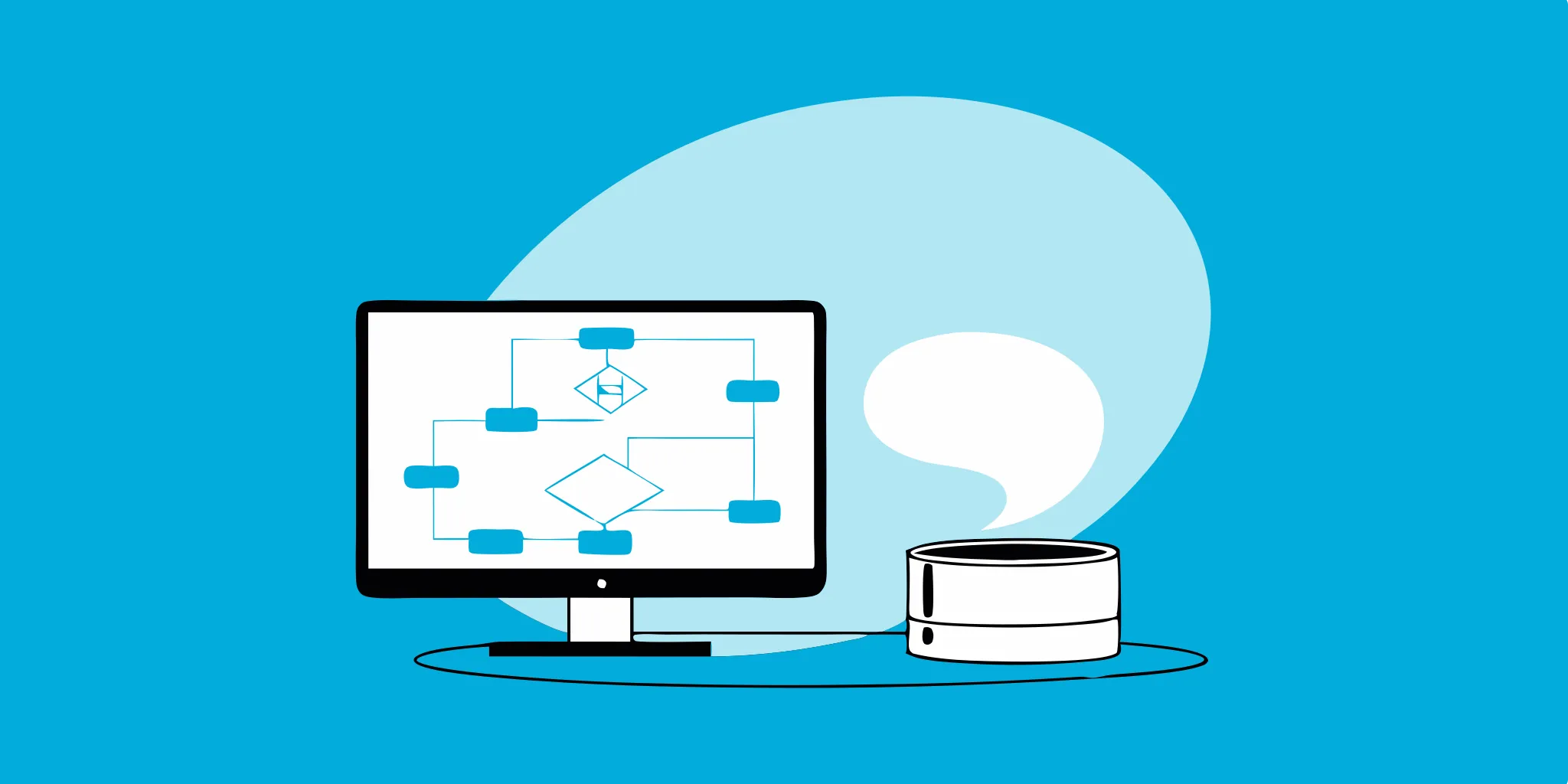
Smoke Test Automation Benefits: Streamlining Your Software Delivery
Learn about smoke test automation benefits and how it ensures reliable software releases by catching critical issues early in the development process.