Verify Code Functions Correctly: Unit & Integration Testing
Author: The MuukTest Team
Last updated: October 1, 2024
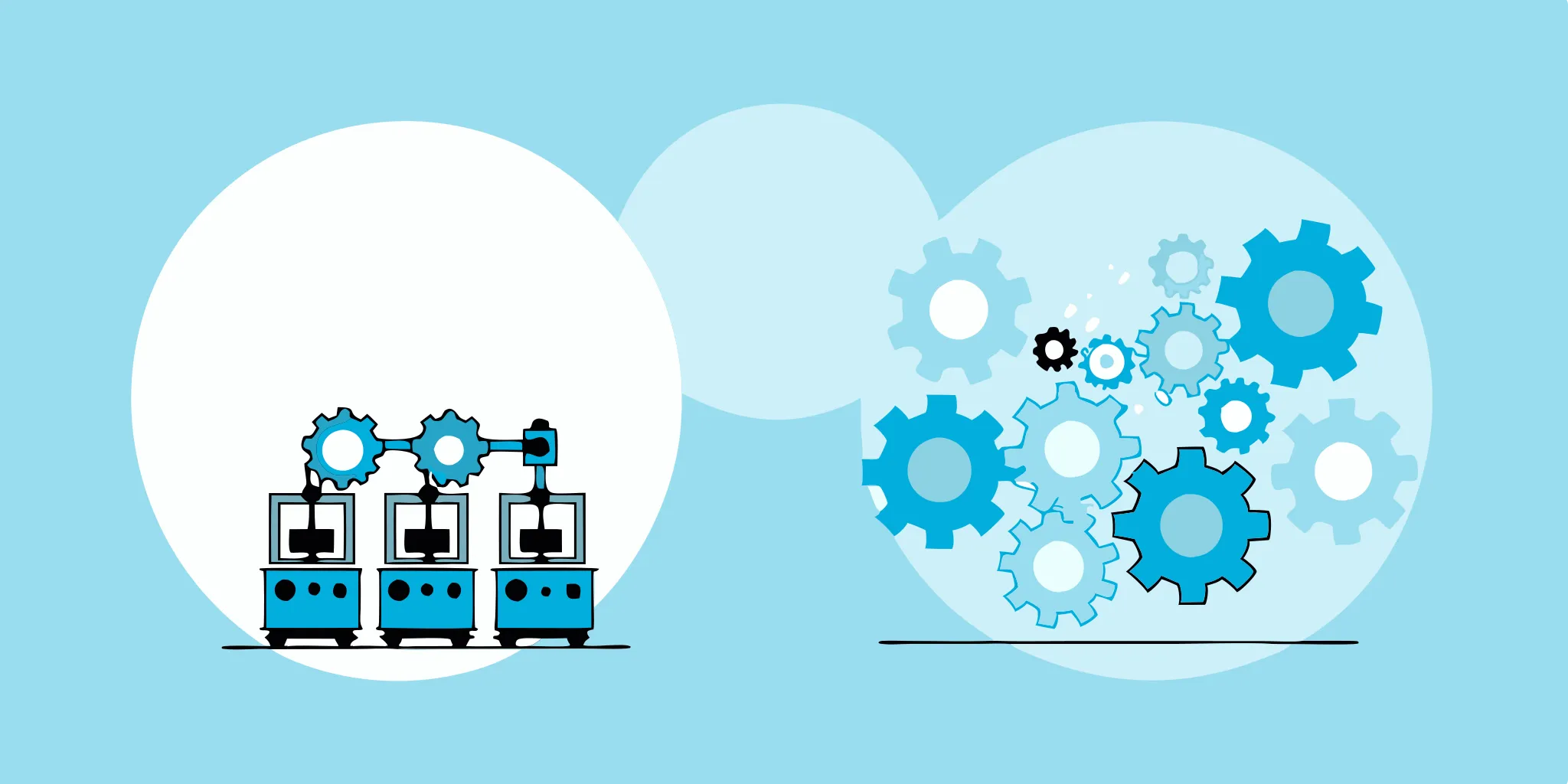
Table of Contents
Creating software is like building with LEGOs. You test each brick (unit test!), but the real magic is seeing how they connect. That's where integration testing comes in. It verifies that the units or pieces of code function correctly when integrated, ensuring your software works as a whole. This article clarifies the differences between unit and integration testing, showing you when and how to use each. We'll also cover best practices, common mistakes, and helpful tools to build a solid testing strategy.
Key Takeaways
- Unit and integration tests work best together: Unit tests check individual parts; integration tests ensure those parts function correctly as a whole. Use both for a complete testing strategy.
- Smart testing means smart tools and planning: Plan integration tests to cover real-world scenarios. Choose the right testing frameworks and tools for your project.
- Automate and prioritize your tests: Integrate tests into your CI/CD pipeline for rapid feedback. Focus on testing the most important parts of your application.
What is Unit Testing?
Unit testing is a software testing method where individual units or components of a software product are tested in isolation. Think of it like testing the individual parts of a car engine—each spark plug, piston, and valve—before assembling the entire engine. This approach ensures each piece works correctly on its own before interacting with other system parts. In software, a "unit" often refers to a single function or method within your codebase. Developers write these tests, primarily for their own use, to quickly catch and fix bugs during development. This process often involves using mock objects to simulate interactions with external dependencies, like databases or APIs, allowing developers to focus solely on the logic of the unit under test.
Understanding Unit Tests: A Quick Definition
Unit tests are characterized by their small scope and fast execution speed. They target very specific pieces of code, making it easy to pinpoint bugs. Because they test isolated units, they run quickly, providing developers with rapid feedback. This speed and precision make unit tests essential for agile development, allowing for quick iterations and adjustments as code evolves. Another key characteristic is their focus on verifying the internal logic of a unit. By using mock objects to stand in for external dependencies, unit tests ensure that the unit's behavior is correct regardless of external factors. This isolation is crucial for creating reliable and maintainable tests.
Why Use Unit Tests?
Unit testing offers several advantages. First, it helps catch bugs early in the development process when they are typically less expensive and easier to fix. Imagine finding a faulty spark plug before the entire engine is assembled—much simpler than tearing down the whole engine later! Second, unit testing improves overall code quality by encouraging developers to write modular and testable code. This makes the codebase more maintainable and easier to refactor. Finally, unit testing provides a safety net for future changes. When refactoring or adding new features, running unit tests can quickly identify any unintended side effects, giving developers confidence that their changes haven't broken existing functionality.
Challenges in Unit Testing
While unit testing offers significant advantages, it also presents some challenges. Let's explore some common hurdles and how to overcome them.
Dealing with Legacy Code
Integrating unit tests into a large, pre-existing codebase (legacy code) can be tricky. It's often tightly coupled, making it difficult to isolate individual units for testing. A good approach is to prioritize. Start by testing the most critical parts of the application. As you add new features or refactor existing code, incorporate unit tests from the start. For particularly tangled sections of legacy code, consider refactoring to improve testability. Small, incremental changes can make a big difference over time.
Handling Flaky Tests
Flaky tests—those that pass sometimes and fail others without any code changes—are a major headache. They erode trust in the testing process and can lead to developers ignoring genuine failures. The key is to identify and fix these flaky tests promptly. Often, they arise from dependencies on external factors like network conditions or system time. Ensure your tests are self-contained and don’t rely on unpredictable elements. Tools that help identify and track flaky tests can be invaluable.
Avoiding Over-Mocking
Mocking is a powerful technique for isolating units under test, but overusing it can lead to tests that don’t accurately reflect real-world behavior. If you find yourself mocking large portions of your system, it might be a sign that your units are too tightly coupled. Strive for a balance. Mock external dependencies and complex interactions, but avoid mocking so much that your tests lose their value. Focus on testing the core logic of your unit and its interactions with immediate collaborators.
Step-by-Step Guide to Unit Testing
Ready to get started with unit testing? Here’s a practical, step-by-step guide to help you implement unit testing effectively:
1. Understand the Code
Before writing any tests, thoroughly understand the code you're about to test. What is its purpose? What are the expected inputs and outputs? A clear understanding of the code’s functionality is crucial for writing effective tests. Review the documentation, specifications, and the code itself to gain a solid grasp of its behavior.
2. Choose a Testing Framework
Select a testing framework appropriate for your programming language and project. Popular frameworks like JUnit (Java), pytest (Python), and Mocha (JavaScript) provide helpful structures and utilities for writing and running tests. Research the available options and choose one that aligns with your needs and preferences. Many unit testing guides offer recommendations based on language and project type.
3. Set Up the Environment
Create a dedicated testing environment separate from your main development environment. This ensures that your tests don’t interfere with other parts of your application and provides a clean slate for each test run. This might involve setting up a separate database, configuring test-specific settings, or using virtualization tools.
4. Write Test Cases (Using the AAA Pattern)
Structure your test cases using the AAA (Arrange, Act, Assert) pattern. The Arrange step sets up the necessary preconditions for the test, such as initializing objects and setting input values. The Act step executes the code being tested. The Assert step verifies the outcome against the expected result. Each test case should focus on a single, specific aspect of the unit’s behavior.
5. Use Mocking/Stubbing
Employ mocking or stubbing techniques to isolate the unit under test from its dependencies. This involves replacing real dependencies with simulated objects that mimic their behavior. This allows you to control the interactions and focus solely on the unit’s logic. Learn more about mocking and stubbing.
6. Run Tests
Execute your tests frequently, ideally as part of an automated build process. Most testing frameworks provide command-line tools or integrations with IDEs to facilitate running tests. Regularly running tests helps catch bugs early and provides rapid feedback on code changes.
7. Analyze Results
Carefully examine the test results. Identify any failing tests and investigate the cause of the failures. Debugging tools and logging can be helpful in pinpointing the source of errors.
8. Refactor and Retest
After fixing any bugs, refactor your code as needed to improve its design and maintainability. Rerun your tests after refactoring to ensure that your changes haven’t introduced new issues. This iterative process of refactoring and retesting helps maintain code quality over time.
9. Maintain Tests
Treat your tests as an integral part of your codebase. Keep them updated as the code evolves and ensure they remain relevant and accurate. Regularly review and refactor your tests to keep them clean, concise, and effective.
Automation vs. Manual Testing in Unit Testing
While automated unit testing is generally preferred for its efficiency and repeatability, manual testing still plays a role, particularly in scenarios involving complex logic or user interface interactions. Automated testing excels in CI/CD pipelines, providing rapid feedback and ensuring consistent test execution. Manual testing, on the other hand, allows for more exploratory testing and can uncover edge cases that automated tests might miss. A balanced approach that leverages both automated and manual testing techniques often yields the best results.
What is Integration Testing?
Integration Testing Defined
Integration testing checks if different parts of a software system work together. Think of it like testing if all the instruments in a band harmonize, not just if each instrument plays the right notes individually. It focuses on the interfaces between modules—how they communicate and exchange data—to catch problems early on. This helps ensure that when individual units are combined, they function correctly as a whole. Integration testing is crucial for uncovering defects that might not show up when testing units in isolation.
Advantages of Integration Testing
Integration testing offers several key advantages. It helps teams develop faster and with more confidence by verifying that all parts of the system work together. Automating these tests allows you to catch integration issues early, even before code is merged, ensuring a smoother development process. This leads to higher quality software and reduces the risk of unexpected problems later. While integration testing offers substantial benefits in improving software quality and mitigating risks, it's also important to be aware of the planning and resources required.
Challenges in Integration Testing
While integration testing offers substantial benefits in improving software quality and mitigating risks, it also presents unique challenges. One common hurdle is managing dependencies between different modules. If one module isn't working correctly, it can cause a chain reaction, making it tricky to pinpoint the exact source of the problem. Think of it like a band where the drummer is off-beat—it throws off the entire performance, making it hard to isolate the issue. Another challenge is the potential for unstable tests. Sometimes, integration tests can pass or fail intermittently without any code changes, which can be frustrating and erode trust in the testing process. This “flakiness” can stem from various factors, including timing issues or inconsistent test environments. Finally, setting up integration tests can be more time-consuming than unit tests. It often involves configuring databases, networks, and other dependencies, which can slow down the development process. Services like MuukTest's automated testing solutions can help streamline this process and improve efficiency.
Unit vs. Integration Tests: Spotting the Differences
Now that we’ve defined unit and integration testing, let’s explore their key differences. Understanding these distinctions helps determine when to use each method for maximum impact.
Scope and Complexity Differences
Unit tests zero in on small, isolated parts of your code—individual functions, methods, or classes. Think of testing individual LEGO bricks. You’re verifying that each brick is structurally sound before combining it with others. Integration tests, on the other hand, examine how these units interact. You’re checking if the LEGO bricks connect correctly to build a larger structure. This broader scope makes integration tests inherently more complex than unit tests. They involve multiple components, increasing the potential points of failure and requiring more setup.
Test Environments: What You Need
Unit tests are typically written by developers for their own use during development. They serve as a quick way to validate code changes and catch errors early. Because unit tests focus on isolated components, they don’t require a fully configured testing environment. Integration tests, however, demonstrate the functionality of the entire system. They’re valuable for showing that the system works as a whole, especially to non-programmers. This often requires a more complete test environment, closer to the production setup.
Speed and Execution Comparison
Unit tests are generally faster to write and execute. Their narrow focus allows for quick feedback loops during development. Finding and fixing bugs during unit testing is also more efficient. Since you’re dealing with smaller code segments, identifying the source of the problem is easier. Integration tests, due to their complexity, take longer to run. Pinpointing the root cause of a failure can also be more challenging, as the issue might stem from the interaction between multiple components. This can make debugging and fixing bugs in integration tests more time-consuming.
Isolation vs. Interaction: How They Work
Unit tests excel at isolating individual code units. They’re often "white-box" tests, meaning you have full knowledge of the code's internal workings. This allows for precise testing of specific logic and behavior. Integration tests can be either "white-box" or "black-box." In black-box testing, you don't need to know the internal workings of external systems or components. You're focused on verifying the interactions and data flow between them.
Who Typically Performs Each Test (Developers vs. QA)
Understanding who typically handles each type of test clarifies roles and responsibilities within a development team. Developers usually write and execute unit tests. This makes sense, as unit tests check small, isolated pieces of code and provide quick feedback during development, allowing developers to catch and fix errors early in the process. It's like a carpenter checking each piece of wood before building a chair.
Integration testing, however, often involves the QA team, although developers may also participate. Since integration tests assess how different parts of the system work together, they require a broader understanding of the system's interactions. This aligns well with the QA team's focus on verifying overall functionality and performance. These tests demonstrate the system working as a whole, which is valuable for everyone involved, technical or not. They're more like checking if all the parts of the chair fit together properly and if the chair is stable.
While these are the typical roles, the exact division of labor can vary based on team structure and project needs. In smaller teams or those using Agile approaches, developers might handle more integration testing. The important thing is to clearly define who's responsible for which tests to ensure thorough testing and smooth teamwork.
When to Use Each Test Type
Knowing when to implement each testing type is key to an effective testing strategy. While distinct, unit and integration tests complement each other, providing comprehensive coverage when used strategically.
Unit Testing in Your Development Cycle
Think of unit tests as your first line of defense against bugs. Use them early and often in the development process. They're ideal for verifying the smallest parts of your program—individual functions, methods, or classes—in isolation. This helps you catch problems quickly, before they become larger issues. Unit testing is particularly valuable when you're making changes to existing code, ensuring you haven't inadvertently broken anything. They're also essential when testing components designed to work independently, confirming they function correctly regardless of external dependencies.
Timing Your Integration Tests
After verifying individual components with unit tests, integration testing steps in to ensure these pieces work together harmoniously. This is where you test the interactions between different modules or services, confirming data flows correctly and the system behaves as expected. Integration testing is crucial for identifying issues that might arise from the combined behavior of multiple units—problems that unit tests alone wouldn't catch. This process is especially important in complex programs with many interacting parts.
Balancing Unit and Integration Tests Effectively
Finding the right balance between unit and integration tests depends on your project's specific needs. While some advocate for a strict testing pyramid with a heavy emphasis on unit tests, a more flexible approach is often necessary. The key is to understand the distinct value each test type provides. Unit tests offer granular verification of individual components, while integration tests ensure these components collaborate effectively as a complete system. By strategically combining both, you can achieve comprehensive test coverage and build a robust, reliable application.
Key Similarities Between Unit and Integration Testing
While distinct in their approach, unit and integration testing share fundamental similarities. Recognizing these commonalities helps us understand their combined role in a robust software testing strategy. Both aim to identify bugs early in the development lifecycle, contributing to improved software quality and reduced debugging time. Think of them as two sides of the same coin, working together to ensure your software functions flawlessly.
Both unit and integration tests are valuable components of a shift-left testing strategy. This approach emphasizes testing early and often during development, catching defects before they become larger, more costly problems. By integrating these tests into a CI/CD pipeline, development teams receive rapid feedback, allowing for quick corrections and adjustments. This proactive approach minimizes the risk of unexpected issues arising later, saving time and resources.
Another shared characteristic is their ability to be automated. Automated testing streamlines the testing process, making it more efficient and less prone to human error. Various testing frameworks and tools facilitate automated unit and integration testing, enabling developers to run tests frequently and integrate them seamlessly into the development workflow. This automation is crucial for maintaining code quality and ensuring consistent results. For a more streamlined and efficient approach to test automation, consider exploring MuukTest's services, designed to help you achieve comprehensive test coverage.
Ultimately, both unit and integration testing validate that the code performs as intended, albeit at different levels of granularity. Unit tests confirm the correct functionality of individual components, while integration tests ensure these components interact correctly when combined. They are complementary, not mutually exclusive, and using both contributes to a more comprehensive and effective testing strategy. Services like MuukTest can help your team achieve complete test coverage efficiently, ensuring your software is thoroughly vetted.
Testing Best Practices: Tips and Tricks
Effective testing is the cornerstone of robust software. By adhering to established best practices, you can ensure your tests are thorough, reliable, and contribute meaningfully to the quality of your applications.
Writing Focused Unit Tests
Unit tests examine individual components of your code in isolation. Think of them as the foundation of your testing strategy. Each unit test should focus on a specific function or method, verifying that it produces the expected output given a particular input. Isolate the unit under test by mocking or simulating any external dependencies. This practice ensures you're testing the unit's logic, not the behavior of its dependencies, making it easier to pinpoint the source of any failures.
Using Mocks and Stubs Effectively
In unit testing, you'll often find components depend on external systems like databases or APIs. Directly interacting with these dependencies during unit tests adds complexity and slows down testing. Mocks and stubs offer a solution. These test doubles simulate the behavior of external dependencies, isolating the unit under test. Think of them as stunt doubles, standing in for the real actors (dependencies) to create controlled and predictable test outcomes. Using mock objects lets you test a unit's logic regardless of external factors. This isolation is key for reliable and maintainable tests, as QATouch points out. For example, if your unit interacts with a database, a mock database can return predefined data, allowing you to test different scenarios without touching the real database.
Considering Test-Driven Development (TDD)
Test-Driven Development (TDD) integrates unit testing directly into the development process. With TDD, you write tests before writing the corresponding code. This approach offers several advantages. It encourages careful consideration of the desired code behavior beforehand, leading to clearer specifications and a more modular design. TDD also provides immediate feedback. By continuously running tests as you code, you ensure each change moves you closer to the desired outcome. This iterative process helps catch bugs early, making them less expensive and easier to fix, as Testlio explains. Finally, the resulting unit tests serve as live documentation, clearly illustrating each component's function. As QATouch recommends, use unit tests as your first line of defense against bugs. Employ them frequently, especially when verifying small parts of your program—individual functions, methods, or classes—in isolation.
Planning Integration Test Scenarios
While unit tests verify individual components, integration tests assess how these components interact. Plan your integration test scenarios carefully to cover real-world use cases. Consider the different ways users might interact with your application and design tests that reflect these interactions. This approach helps uncover issues that might not be apparent when testing units in isolation, such as communication problems between modules or data inconsistencies. A comprehensive guide to modern testing practices can offer valuable insights into planning effective integration tests.
Focusing on Real Interactions
Integration testing illuminates the interplay between different parts of your software. It’s like observing how musicians in a band perform together, not just their individual solos. The focus is on the interfaces—how modules communicate and exchange data—to catch compatibility issues early. For example, if you're testing an e-commerce platform, your integration tests should mimic real user flows, like adding items to a cart, proceeding to checkout, and completing a purchase. This helps uncover issues that might not surface during unit testing, such as data inconsistencies between the shopping cart and the order processing module. By focusing on real interactions, you gain a realistic view of your system's performance under real-world conditions.
Using Realistic Data and Environments
To maximize the effectiveness of your integration tests, use realistic data and environments. Don’t just test with idealized or simplified data. Instead, use data that reflects the variety and complexity of real-world scenarios. This might include edge cases, boundary conditions, and even invalid inputs. Similarly, your testing environment should closely resemble your production environment. This includes using the same database, operating system, and network configurations. By mirroring real-world conditions, you increase the likelihood of uncovering hidden bugs that might otherwise impact your users. A well-configured testing environment, combined with realistic data, provides a more accurate assessment of your system's performance and reliability. For more information on setting up effective test environments, check out this guide to software testing environments. Consider using a service like MuukTest to help achieve comprehensive test coverage efficiently.
Maintaining Test Independence
Whether you're writing unit tests or integration tests, maintaining test independence is paramount. Each test should be self-contained and able to run independently of other tests. This practice prevents cascading failures, where one failing test causes subsequent, unrelated tests to fail. Independent tests simplify identifying the root cause of a problem and ensure your test suite remains reliable.
Automating Your Tests
In today's fast-paced development environment, automating your tests is essential. Integrate your tests into your CI/CD pipeline so they run automatically whenever code changes are made. This automation provides rapid feedback, allowing you to catch and address issues early in the development process. Automating both unit and integration tests helps ensure your codebase remains stable and that new changes don't introduce regressions. Learn more about automating tests within your CI/CD pipeline to streamline your testing process and improve development efficiency.
Tools and Frameworks for Testing
Knowing the right tools for unit and integration testing can streamline your testing process. This section explores some popular options.
Go-To Unit Testing Tools
For unit testing, several robust frameworks are available, depending on your programming language. JUnit is a widely-used framework for testing Java applications, providing a simple way to write and run repeatable tests. If you're working with .NET, NUnit offers similar capabilities for creating unit tests. Other popular unit testing tools include JMockit, Emma, and Quilt HTTP. A more comprehensive list includes options like HtmlUnit, Embunit, SimpleTest, ABAP Unit, Typemock, LDRA, the Microsoft unit testing framework, Unity Test Tools, Cantata, Karma, Jasmine, Mocha, Parasoft, TestNG, and JTest. Choosing the right tool often depends on your project's specific needs and your team's preferences.
JUnit
JUnit is a widely-used, open-source framework designed for testing Java applications. It provides a simple and structured way to write and run repeatable tests, making it a favorite among Java developers. JUnit helps you create and organize test suites, write individual test cases to verify specific units of code, and execute these tests for rapid feedback. Learn more about JUnit and its features on the official JUnit website.
NUnit
If you're working with .NET, NUnit offers similar capabilities to JUnit for creating unit tests. It provides a structured and user-friendly approach within the .NET ecosystem, offering a rich set of features for writing various tests, from basic assertions to more complex parameterized tests. The NUnit website has comprehensive documentation and resources to get you started.
pytest
For Python developers, pytest is a powerful and versatile testing framework known for its simplicity and scalability. It makes writing concise and effective test cases easy. Its plugin-based architecture allows for extensive customization and integration with other tools. Explore the official pytest documentation for a deeper understanding of its capabilities.
JMockit
JMockit is a popular mocking framework for Java, simplifying the creation of mock objects for unit testing. Mocking isolates the code under test by simulating the behavior of its dependencies. JMockit streamlines creating and managing mock objects, leading to more focused and reliable unit tests. The JMockit website provides detailed information and examples.
Emma
Emma focuses on code coverage, a metric showing how much of your code is exercised by your tests. This helps identify gaps in your testing strategy, ensuring thorough testing of critical code sections. Emma provides detailed reports and visualizations of code coverage, highlighting areas needing additional tests. While Emma is no longer actively maintained, its principles remain relevant. You can find information about Emma and other coverage tools through resources like Headspin's guide on unit testing.
Other Unit Testing Tools
The unit testing tools landscape is vast and constantly evolving. Many other frameworks and libraries cater to different programming languages and specific testing needs. Some notable examples include HtmlUnit for testing web applications, Typemock for .NET mocking, and JavaScript testing frameworks like Jasmine, Mocha, and Karma. Exploring these options can help you find the perfect tools for your unit testing practices. Headspin's comprehensive guide provides a broader overview, listing tools like Embunit, SimpleTest, ABAP Unit, LDRA, the Microsoft unit testing framework, Unity Test Tools, Cantata, Parasoft, TestNG, and JTest.
Effective Integration Testing Frameworks
Selenium is a powerful open-source tool for automating integration tests in web applications. Its flexibility makes it a popular choice. For Python-based projects, pytest is a valuable framework for creating and running tests. The purpose of these tools is to verify how different modules of your software work together and catch any integration defects early on. This helps ensure that individual units function correctly as a combined system.
Postman
Postman is a widely used tool for API testing, allowing developers to create and run tests for RESTful APIs. It provides a user-friendly interface for sending requests and validating responses, making it essential for integration testing. Its popularity means you’ll find many online resources and tutorials.
SoapUI
SoapUI is designed for testing SOAP and REST APIs. It offers comprehensive features for functional testing, performance testing, and security testing, making it valuable when your integration testing involves web services. Its focus on API testing makes it a strong choice for projects reliant on service integrations.
Selenium
Selenium is a powerful open-source tool for automating integration tests, especially in web applications. Its flexibility and broad browser support make it popular among testers. Selenium simulates user interactions within a browser, ideal for testing the end-to-end flow of your web application, ensuring all components work together seamlessly.
Quilt HTTP
Quilt HTTP is designed for testing HTTP-based services. Developers use it to create tests that validate the behavior of web services, ensuring they interact correctly with other components. If your project involves extensive HTTP interactions, Quilt HTTP can be a valuable addition to your testing toolkit.
HtmlUnit
HtmlUnit provides a GUI-less browser for Java programs, allowing you to test web applications by simulating a browser environment. This is useful for integration testing without a visual browser, particularly helpful for running tests in a headless environment, such as a continuous integration server.
Other Integration Testing Tools
These tools are just a small selection of available integration testing tools. Other options include JMockit and Emma, along with various frameworks for specific programming languages. The right tool depends on your project’s needs, the technologies used, and your team’s preferences. Explore different options, considering ease of use, community support, and integration with your existing workflow.
Common Testing Misconceptions
Let's clear up some common misconceptions about unit and integration testing. These misunderstandings can lead to ineffective testing strategies and, ultimately, software bugs.
Myth: Unit Tests Are Enough
While unit tests are essential for verifying individual components work correctly in isolation, they don't test how these components interact. Think of it like testing individual parts of a car—the engine, the brakes, the steering wheel. Each part might work perfectly on its own, but that doesn't guarantee they'll function seamlessly together when assembled. You still need a road test (integration testing) to ensure the entire car operates as expected. Overlooking integration tests can lead to undetected issues when different parts of your system communicate, potentially causing problems down the line.
Myth: Integration Tests Are Just Bigger Unit Tests
This misconception minimizes the distinct purpose of integration tests. Integration tests aren't simply scaled-up unit tests; they evaluate the combined functionality of multiple units as a system.
Myth: All Tests Are Black-Box
Another misconception is that all tests are "black-box," meaning the tester doesn't know the internal workings of the code. While this can be true for some integration tests, unit tests are often "white-box" tests. In white-box testing, developers use their knowledge of the code's internal structure to design targeted tests. Understanding this distinction helps choose the right testing approach for different scenarios. Integration tests can be either white-box or black-box, depending on the specific goals and the level of access to the internal code. By understanding the nuances of each testing type, you can build a more robust and effective testing strategy.
Overcoming Testing Challenges
Testing is crucial for software quality, but it's not without its challenges. Let's discuss some common roadblocks and how to address them.
Managing Complex Dependencies
One of the trickiest aspects of testing is managing dependencies between different parts of your code. Mocks can be helpful for isolating components during unit tests, but they can add complexity to integration tests. Finding the right balance is key. Consider using dependency injection frameworks or tools that help you manage and visualize dependencies to simplify your integration tests. This will allow you to isolate units effectively while still ensuring that your integrated system functions correctly.
Maintaining Test Suites
Creating tests takes time, and keeping them current as your codebase grows requires ongoing effort. It's easy to let tests fall behind, especially when deadlines are tight. Prioritize writing maintainable tests from the outset. Techniques like clear naming conventions, concise tests, and focusing on testing behavior rather than implementation details can make a big difference. Also, explore tools that can automatically update tests when code changes, saving you time and effort. This way, your test suite remains a valuable asset rather than a burden.
Handling Test Data and External Services
Testing interactions with external services and managing test data can be a real pain. You need realistic test data that reflects real-world scenarios, but generating and managing this data can be time-consuming. External services can also introduce unpredictable behavior into your tests. Consider using techniques like service virtualization to simulate external dependencies and create a more controlled testing environment. For managing test data, look into tools that can help you generate realistic synthetic data or manage test databases efficiently. Thoughtful planning and the right tools are essential for reliable integration testing.
Building a Comprehensive Testing Strategy
A well-rounded testing strategy is the backbone of robust software. It's not about choosing between unit and integration testing—it's about strategically using both. This section outlines how to combine these methods, integrate them into your development workflow, and measure their impact.
Combining Unit and Integration Testing for Better Code
Think of unit tests as your first line of defense, verifying individual components work as expected in isolation. They catch errors early in the development process, making them quicker and cheaper to fix. Unit testing is like checking the individual parts of a car—the engine, brakes, and transmission—to ensure they function correctly on their own.
Integration tests, on the other hand, examine how these components interact. They ensure that when you combine the engine, brakes, and transmission, the entire car operates smoothly. Both unit and integration testing are essential for building reliable software. While the classic testing pyramid suggests a 70/20/10 split between unit, integration, and end-to-end tests, a more flexible approach is often necessary, adapting to the specific needs of your project. Understanding the strengths and challenges of each testing approach helps you tailor your testing strategy effectively.
Continuous Integration and Testing: Streamlining Your Workflow
Integrating testing into your CI/CD pipeline is crucial for maintaining code quality and accelerating development. Every code change should automatically trigger both unit and integration tests. This automation helps catch integration issues early, even before code merges, ensuring a smoother development process. Automating your tests not only saves time but also enforces consistent testing practices across your team. This early detection of issues prevents them from snowballing into larger, more complex problems down the line.
MuukTest's Role in CI/CD
MuukTest excels at automating the crucial integration testing phase within your CI/CD pipeline. Remember how integration testing verifies that different parts of your software work together seamlessly? MuukTest automates these tests, ensuring they run automatically with every code change. This rapid feedback loop allows you to catch and address integration issues early, preventing costly problems later. By integrating MuukTest into your CI/CD workflow, you gain efficiency and ensure consistent and comprehensive test coverage, a key factor in delivering high-quality software.
Think of it this way: you've unit tested individual components, confirming they function correctly in isolation. Now, with MuukTest, you can confidently assemble these components, knowing that automated integration tests will rigorously examine their interactions. This automated approach saves developer time and enforces consistent testing practices across your team, contributing to a more robust and reliable software development lifecycle. For a deeper dive into balancing unit and integration tests, check out this article. Ready to experience the benefits? Explore MuukTest's QuickStart guide.
Measuring Test Coverage and Effectiveness
Tracking test coverage—the percentage of your code covered by tests—is a good starting point. However, high test coverage doesn't always equate to effective testing. Focus on testing critical paths and potential failure points in your application. Regularly review and refine your tests to ensure they remain relevant and catch the most important bugs. Remember, the cost of fixing bugs increases dramatically the later they are found. Prioritizing thorough testing early in the development cycle, starting with unit tests, ultimately saves time and resources. Consider exploring MuukTest's services to see how AI-powered test automation can further enhance your testing strategy and achieve comprehensive test coverage efficiently.
Frequently Asked Questions
What's the main difference between unit and integration testing?
Unit testing verifies the smallest parts of your code in isolation, like individual functions or methods. Integration testing, however, checks how these different parts work together as a system, focusing on their interactions and data flow. Think of unit testing as testing individual LEGO bricks, while integration testing ensures those bricks connect correctly to build a larger structure.
Why isn't unit testing sufficient on its own?
Unit tests are great for catching bugs early in individual components, but they don't tell you if those components work together correctly. Integration testing is essential for uncovering issues that arise from the combined behavior of multiple units, such as communication problems or data inconsistencies.
How do I decide when to use each type of test?
Use unit tests early and often during development to verify the logic of individual code units. Implement integration tests after unit testing to ensure different modules or services interact correctly. The balance between the two depends on your project's complexity and specific needs.
What are some common tools for unit and integration testing?
JUnit and NUnit are popular for unit testing Java and .NET applications, respectively. For integration testing, Selenium is a powerful tool for web applications, while pytest is a valuable framework for Python projects. Many other tools exist, so choose those that best suit your project and team.
How can I make my testing process more efficient?
Automate your tests by integrating them into your CI/CD pipeline. This ensures tests run automatically with every code change, providing rapid feedback and catching issues early. Also, focus on writing maintainable tests by keeping them concise, well-named, and focused on behavior rather than implementation details. Consider using tools to manage dependencies and test data effectively.
Related Articles
- Unit vs Integration Testing: Key Differences & When to Use Each
- Integration Testing vs Unit Testing: Key Differences & When to Use Each
- Integration Testing Software: Best Practices and Top Tools
- Software Unit Testing: The Ultimate Guide (2024)
- Automated Software Testing: Benefits, Tools, and Best Practices
Related Posts:
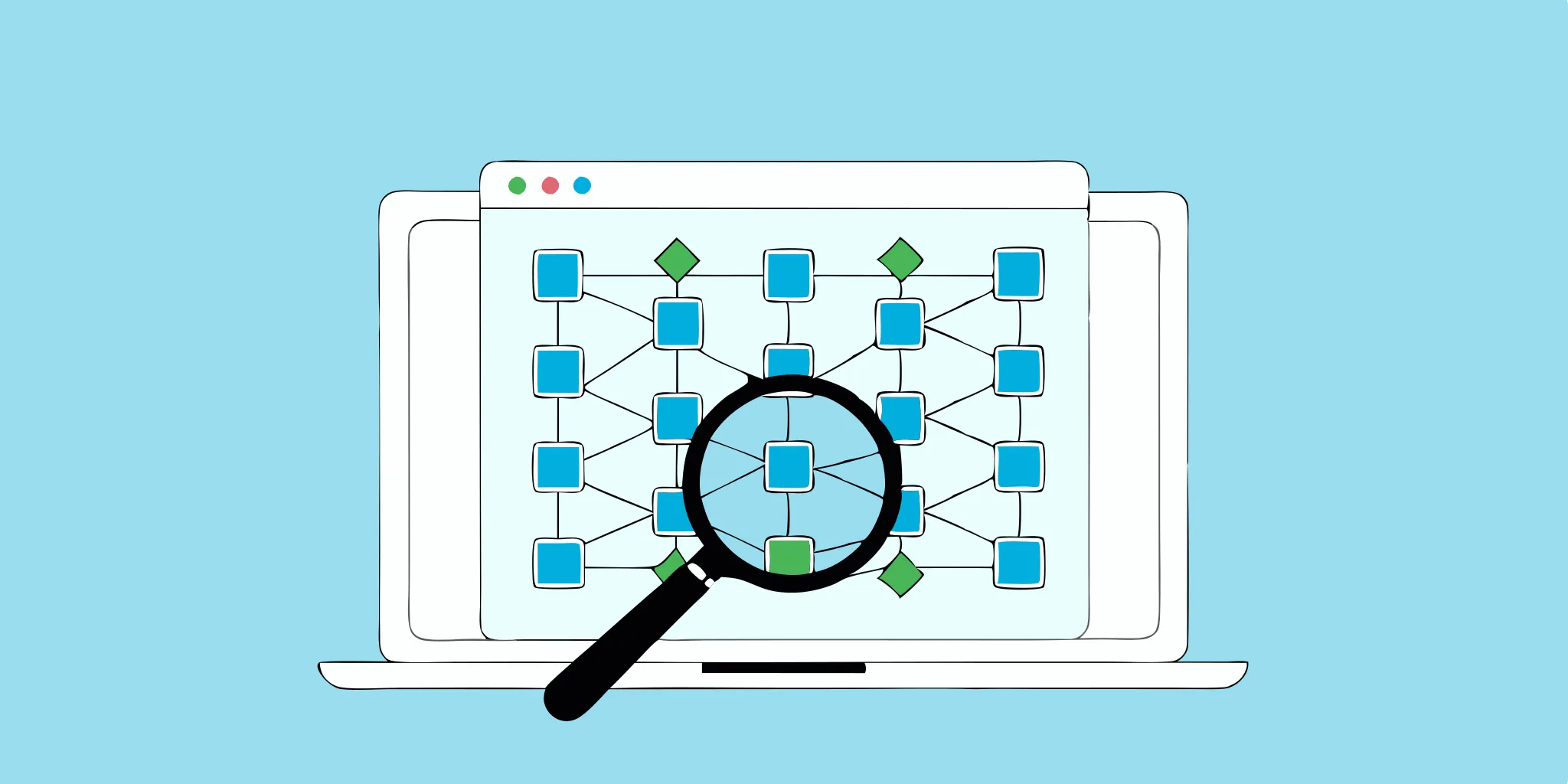
Types of Software Testing: Your Complete Guide
In the ever-evolving world of software development, quality isn't just a desirable trait—it's a necessity. And that's where software testing comes in. It's the crucial process that ensures your...
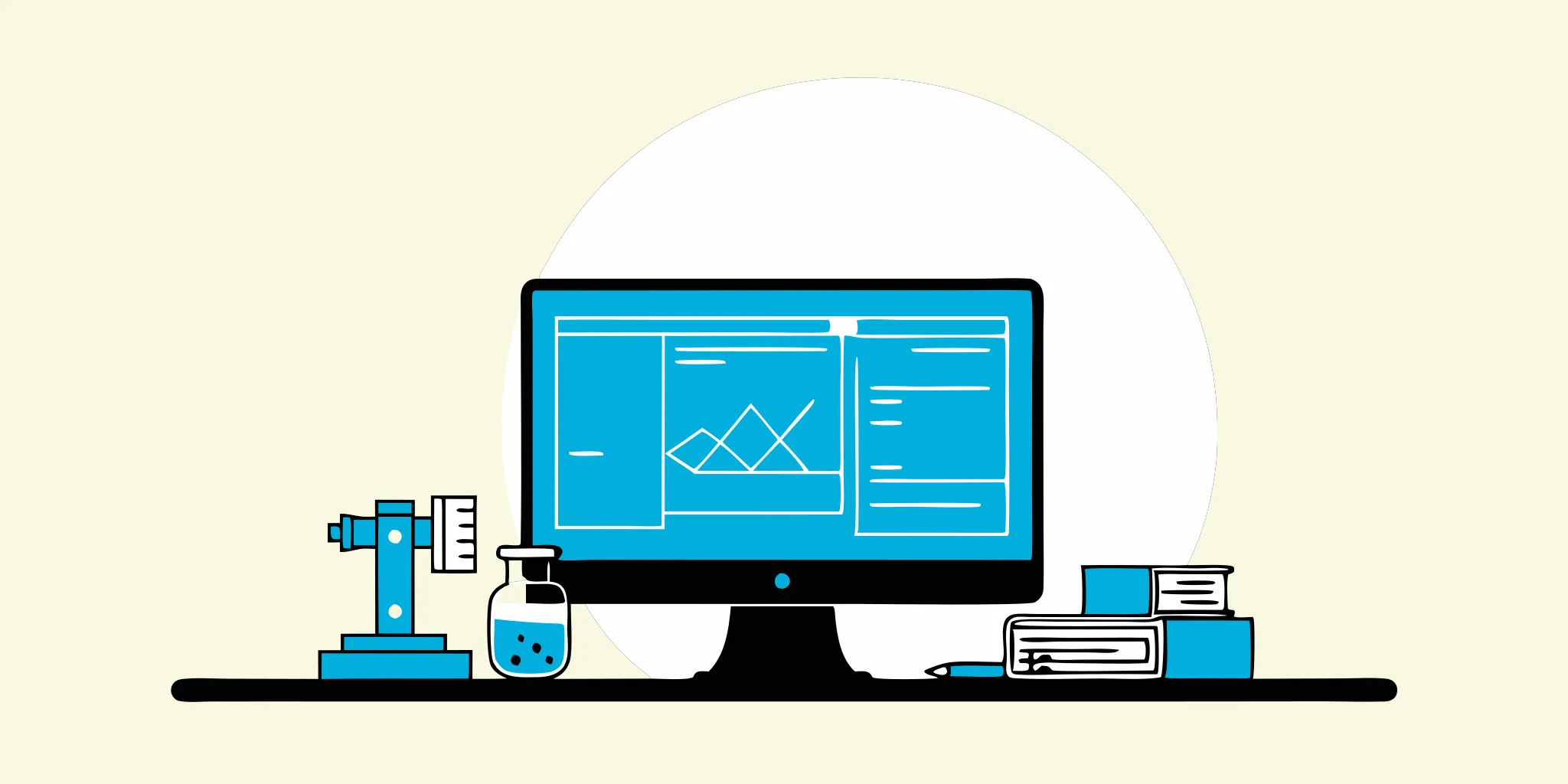
Software Testing Techniques: The Ultimate Guide
Master software testing techniques with this comprehensive guide, covering essential methods and strategies to enhance your software's quality and reliability.
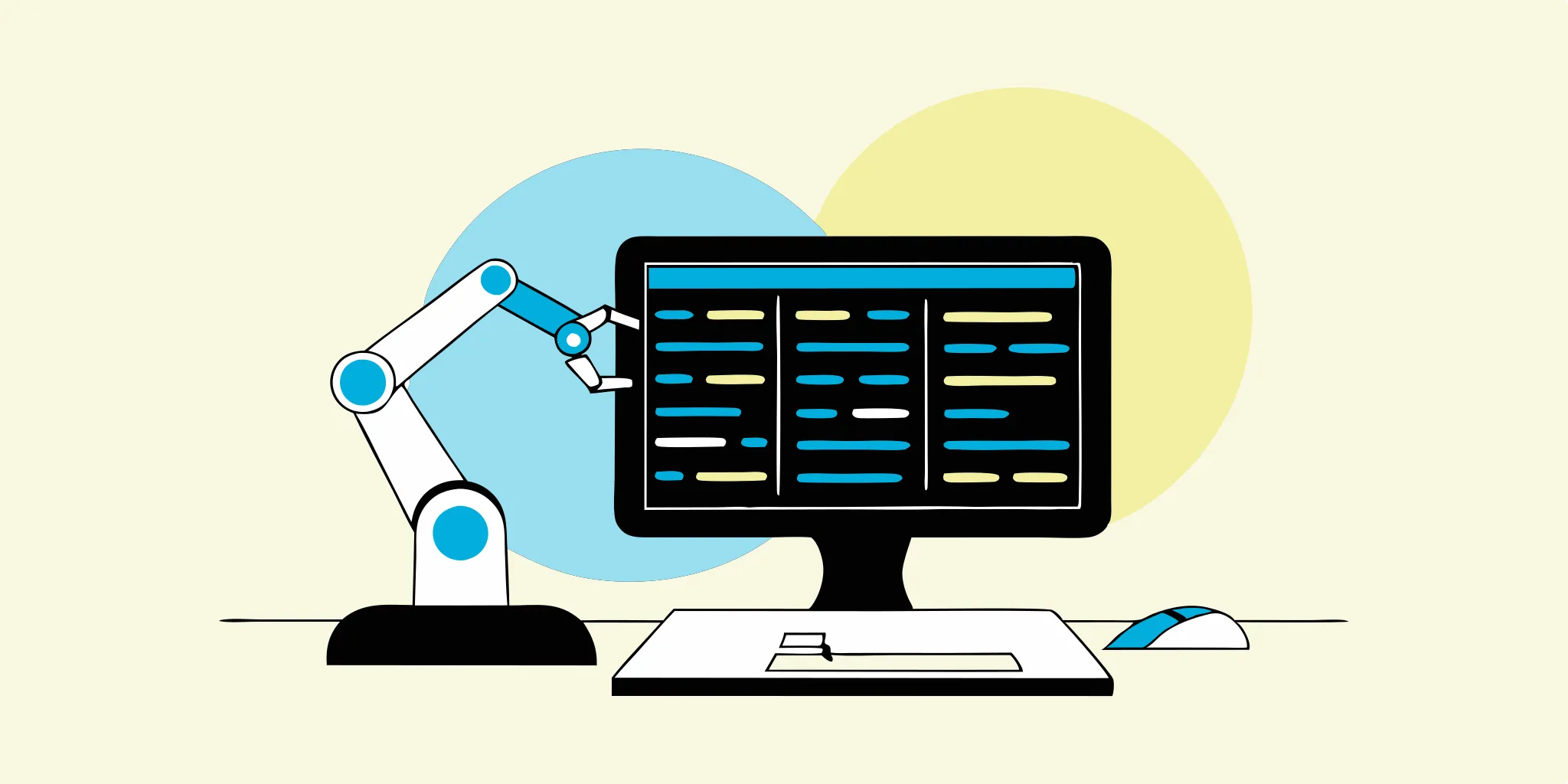
Unit Testing Automation: A Practical Guide
Master unit testing automation with this practical guide, offering insights on best practices, tools, and strategies to enhance your software development process.