Software Unit Testing: A Practical Guide
Author: The MuukTest Team
Published: January 24, 2025
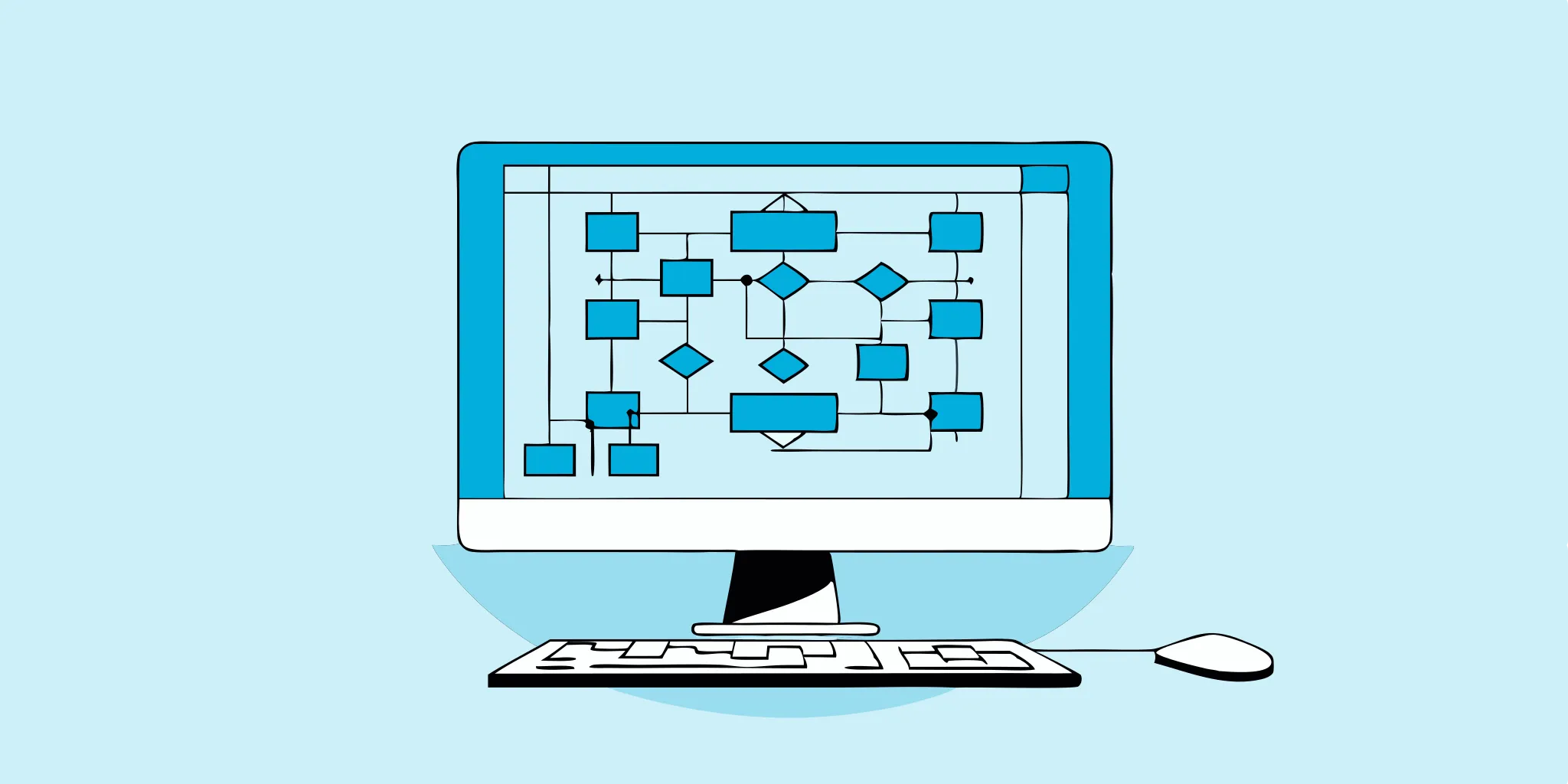
Table of Contents
Building quality software requires rigorous testing, and it all begins with software unit testing. Think of it as checking the individual parts of a machine before assembling the whole thing. This process isolates and tests the smallest components of your code—functions or modules—to ensure they work correctly on their own. By catching bugs early with software unit testing, you prevent small issues from becoming major headaches (and save time and money!). This guide will give you the practical know-how to implement effective software unit testing strategies for more robust and reliable software.
Key Takeaways
- Unit testing is essential for software quality: Catching bugs early in the development cycle through unit testing saves time and resources, leading to more robust and reliable software.
- Well-written unit tests are independent, clear, and comprehensive: Leverage unit testing frameworks, write descriptive test names, and ensure your tests cover both typical scenarios and edge cases. Integrate unit testing into your workflow with practices like test-driven development (TDD) and continuous integration (CI).
- Stay ahead of the curve: AI, machine learning, and the shift-left approach are transforming software testing. Keep up with these trends to optimize your testing strategy and maintain a competitive edge.
What is Software Unit Testing?
Unit testing is a software testing method where individual units or components of a software program are tested in isolation. Think of it like testing individual Lego bricks before building a castle. Each brick needs to be strong and fit perfectly to ensure the entire structure is stable. Similarly, each unit of your software must function correctly for the entire application to work as expected. This process helps identify bugs early in the development, ensuring each piece of your software works as intended before integrating it with other parts of the application.
Purpose of Software Unit Testing
Unit tests are typically written by the developers as they write the code. This allows them to catch errors immediately and correct them before they become larger problems. While you can run these tests manually, automating them is much more efficient. Automated tests can be run quickly and repeatedly, providing consistent and reliable results. This helps identify regressions, which are bugs that reappear after they've been fixed. Automating your unit tests also frees up developers to focus on writing new code. The purpose of unit testing is to isolate each part of the program and verify that individual parts are correct. This is a critical step in development because, if done correctly, it can help detect early flaws in code that may be more difficult to find later.
Key Components of Unit Tests
A unit test is essentially a small piece of code designed to check another small piece of code. It provides specific input to the code being tested and verifies that the output is what you expect. Effective unit tests check several aspects of a unit's behavior: its core logic, how it handles boundary conditions (like maximum and minimum values), its error handling, and how it interacts with objects. Unit tests should be independent of each other and not rely on external systems or databases. To achieve this independence, developers often use "test doubles" like stubs or mock objects. These stand-ins mimic the behavior of real components, allowing you to isolate the unit under test and ensure its functionality is correct regardless of external factors.
A Brief History of Unit Testing
Unit testing, also known as component or module testing, focuses on verifying the smallest testable parts of a software application in isolation. Think of it as quality control at the most granular level. While the concept of testing individual components has been around for a while, its formal practice gained significant traction in the early 2000s alongside the rise of agile software development. This shift towards iterative development and rapid feedback cycles emphasized the need for automated testing to ensure code quality throughout the process.
Historically, software testing often happened towards the end of the development lifecycle. This approach meant that bugs could remain hidden until late in the game, making them more complex and costly to fix. The evolution of unit testing demonstrates a move away from this model, promoting a more proactive approach where testing is integrated from the very beginning. This shift aligns with the core principles of agile development, enabling teams to identify and address issues early on, leading to more reliable and maintainable code. As a result, the overall history of software testing has been greatly influenced by the need for more efficient and effective methods like unit testing.
The development of unit testing frameworks further accelerated its adoption. These frameworks provide tools and structures that make it easier to write, run, and manage unit tests, automating the process and making it an integral part of the software development workflow. This evolution has significantly impacted software quality, allowing developers to catch bugs early and prevent them from becoming larger problems. For companies looking to improve their testing efficiency and coverage, services like those offered by MuukTest can provide comprehensive and scalable solutions tailored to their needs. As software development continues to evolve, unit testing remains a cornerstone of building robust and reliable applications.
Why Unit Test Your Software?
Unit testing might seem like an extra step, but it’s essential for building robust and reliable software. Think of it as a safety net, catching potential issues before they snowball into major problems. This proactive approach improves the quality of your software and saves you time and money down the line.
Find Bugs Early and Improve Code
Unit testing involves checking the smallest parts of your code—individual functions or modules—to ensure they work correctly in isolation. This helps find bugs early in the development cycle, when they're much easier and cheaper to fix. Imagine trying to find a single misspelled word in a 1000-page book after it's been published—debugging large, untested codebases presents a similar challenge. Unit tests act like a spell checker, identifying errors before they become larger issues. Plus, writing unit tests often encourages developers to write cleaner, more organized code, improving overall software design.
Save Time and Money with Unit Testing
While writing unit tests requires an initial time investment, it ultimately saves you significant time and resources. Early bug detection through unit testing streamlines development, preventing costly fixes later. Fixing a bug during development is far less expensive than addressing it after release. Automated unit tests, which run automatically whenever you change your code, provide instant feedback, catching regressions and integration problems immediately. This rapid feedback loop helps maintain code quality and prevents small errors from derailing your project. By catching issues early, you avoid the time-consuming and expensive process of debugging complex problems in a fully integrated system.
Improve Software Design and Maintainability
Beyond catching bugs, unit testing significantly improves your software's design and maintainability. When you know you need to test something, you design it differently. You consider how each piece will work independently *and* as part of the whole. This leads to more modular and well-organized code, which is easier to understand, modify, and maintain. Unit testing encourages this kind of thoughtful design from the start.
Writing unit tests often forces you to think about how your code will be used and how different parts interact. This process naturally leads to cleaner, more reliable code because you're considering various scenarios and edge cases upfront. You're more confident that your code works as expected, not just in isolation but also within the larger system. This connects with the idea of unit testing as a way to improve code quality.
Because unit tests check individual units of code in isolation, they encourage developers to create components that are less dependent on each other. This isolation is key for maintainability. When components are loosely coupled, you can modify one without a ripple effect of errors throughout the system. This makes refactoring—improving the internal structure of your code—much safer and easier. Testing individual units builds a more robust and adaptable codebase.
Unit tests also act as a safety net during refactoring. They quickly verify that changes haven't introduced unintended side effects. This safety net allows developers to confidently improve the codebase without fear of breaking existing functionality. Think of unit tests as guardrails, preventing you from accidentally veering off course during development.
Finally, unit tests serve as living documentation. They clearly demonstrate how each unit of code should work. This is incredibly valuable for new developers joining a project or for anyone revisiting code after some time. Well-written unit tests can be more informative than traditional documentation because they show, rather than just tell, how the code behaves. They provide practical examples of how to use and interact with different parts of the system.
Benefits of Software Unit Testing
Unit testing offers significant advantages for software development teams. From catching bugs early to improving overall design, unit tests are crucial for delivering high-quality software. Let's explore some key benefits:
Easier Code Maintenance with Unit Tests
Well-written unit tests act as a safety net when you’re making changes to your codebase. They help identify unintended consequences early, making it much easier to maintain and update your software. As your project grows, this becomes increasingly important. Refactoring—improving your code's internal structure—becomes less risky and more manageable with a solid set of unit tests. They verify that your changes haven't introduced new bugs or broken existing functionality. This early bug detection significantly reduces the cost and effort of fixing issues later.
Better Documentation, More Confident Developers
Unit tests serve as living documentation for your code. They clearly demonstrate how individual components should be used. New team members can quickly grasp the codebase by reviewing the tests. This shared understanding fosters collaboration and reduces onboarding time. Plus, having comprehensive unit tests gives developers the confidence to make changes without fear of breaking things. This confidence promotes a more proactive and efficient development process.
Simplify Debugging and Refactoring
Debugging becomes much simpler with unit tests. When a test fails, it pinpoints the specific code causing the issue. This targeted approach eliminates long, drawn-out debugging sessions. Automated unit tests, which run whenever you change your code, provide immediate feedback. This rapid feedback loop helps you catch and address problems quickly, streamlining development. Refactoring also becomes much safer and easier with unit tests in place.
Improve Your Software Design
Writing unit tests often encourages more careful thought about your code's design. Effective unit tests require modular, well-structured code. This naturally leads to better software design principles, such as loose coupling and high cohesion. Unit testing is a cornerstone of modern software development methods like Agile and Extreme Programming (XP). By encouraging better design, unit testing contributes to more robust, maintainable, and scalable software. This results in higher quality, faster development, and increased productivity.
Limitations of Unit Testing
While unit testing is a powerful tool, it's not a complete solution. Understanding its limitations is crucial for a balanced and effective testing strategy. Like any approach, unit testing has blind spots, and relying solely on it can create a false sense of security. Let's explore some of these limitations to help you build a more robust testing approach.
Dealing with Complex Dependencies
Unit testing excels when dealing with isolated units of code. However, real-world software often involves intricate interactions between different components, external services, and databases. Think of a system that connects to a third-party payment gateway or a database. Isolating these units for testing can be tricky. As noted in Wikipedia's article on unit testing, some code, like multi-threaded or non-deterministic code, is hard to test effectively. Mocking these dependencies is helpful, but it also risks creating tests that don't accurately reflect real-world scenarios. This is where integration testing comes in, bridging the gap between isolated unit tests and the complex interactions of a complete system. Services like MuukTest can help manage these complexities and ensure comprehensive test coverage.
Testing in Isolation vs. Real-World Interactions
Unit tests excel at verifying individual components' behavior in isolation. However, this focus can also be a weakness. By concentrating on isolated units, you might miss issues arising from the interplay of these components in a fully integrated system. Wikipedia's unit testing page points out that unit tests can't find every bug, especially problems arising when different program parts work together. For example, a unit test might confirm that a shopping cart correctly calculates the total price. But it won't necessarily catch a checkout process failing to communicate with the payment gateway. This is why combining unit testing with other methods like integration and system testing is essential for a holistic view of your software's functionality. Using "test doubles" like mocks and stubs, while necessary for isolation, can sometimes create a disconnect between the test environment and real-world usage, as also highlighted on Wikipedia. This further emphasizes the need for a multi-layered testing approach.
Writing Effective Unit Tests
Solid unit tests are the foundation of a reliable codebase. They act like tiny watchdogs, constantly making sure individual parts of your software behave as expected. But how do you write unit tests that are both effective and maintainable? Here's a breakdown:
Frameworks and Test Organization
Leverage the power of unit testing frameworks. Frameworks like pytest or unittest in Python offer tools and structure for organizing and running your tests. They provide a standardized way to define test cases, manage test suites, and generate reports, which streamlines your entire testing process. Think of them as your project management system, but for tests. Using a framework makes it easier to group related tests, set up common fixtures, and handle test dependencies, ultimately leading to a more organized and efficient workflow.
Independent Tests and Edge Cases
Each unit test should be self-contained and independent of other tests. This isolation ensures that you can pinpoint the exact location of a bug when a test fails. Avoid dependencies between tests, as they can lead to cascading failures and make debugging a nightmare. In addition to testing the "happy path," make sure your tests cover edge cases and boundary conditions. Think about unusual inputs, extreme values, and error conditions. Thorough testing of these scenarios helps uncover hidden bugs and strengthens the robustness of your code.
Clear Test Names for Better Understanding
Treat your test names like mini-documentation. A well-chosen name clearly explains the purpose of the test, making your code easier to understand and maintain. Imagine coming back to your code months later—a descriptive test name can instantly remind you of the specific functionality being tested. For example, instead of a generic name like test_function1
, use a more specific name like test_function1_handles_invalid_input
. This clarity not only benefits you but also anyone else who works with your codebase.
Best Practices for Writing Unit Tests
Well-written unit tests are essential for catching bugs early and ensuring the quality of your code. They should be easy to understand, run quickly, and provide reliable results. Let's explore some best practices to guide you:
The FIRST Principle
The FIRST principle provides a helpful acronym to remember key characteristics of effective unit tests. Your tests should be Fast, running quickly to provide rapid feedback. They should be Independent, meaning one test shouldn't rely on the outcome of another. This isolation helps pinpoint issues when a test fails. Tests should also be Repeatable, producing consistent results every time they're run, regardless of the environment. Self-validating tests automatically check their own results, eliminating the need for manual verification. Finally, tests should be Timely, written alongside the code they're testing—ideally before or during development, not as an afterthought. Adhering to these principles ensures your tests are efficient, reliable, and easy to maintain. For a deeper dive into the world of unit testing, check out this helpful Wikipedia article.
Keep Tests Concise and Focused
Each unit test should have a single, well-defined purpose. Focus on testing one specific aspect of a unit's behavior at a time. This clarity makes it easier to understand what each test is verifying and simplifies debugging when a test fails. Avoid creating overly complex tests that try to cover too much ground. This guide on unit testing emphasizes the importance of keeping tests concise and focused, making them easier to understand, maintain, and debug, ultimately contributing to a more robust and reliable codebase.
Test-Driven Development (TDD)
Test-Driven Development (TDD) takes unit testing a step further by writing the tests before writing the code. This approach might seem counterintuitive, but it encourages better design and ensures your code meets its requirements from the outset. The TDD cycle involves writing a failing test, then writing the minimum amount of code necessary to pass the test, and finally, refactoring the code to improve its design. This iterative process leads to cleaner, more modular code and comprehensive test coverage. Learn more about Test-Driven Development (TDD) and how it can be a powerful tool for building high-quality software.
Integrating Unit Testing into Your Workflow
Integrating unit tests effectively into your development process is key to realizing their full benefits. This involves not only understanding how to write unit tests but also how they fit into broader software development methodologies.
Test-Driven Development (TDD) in Practice
A popular approach is test-driven development (TDD), where you write tests before you write the code. This might seem counterintuitive, but it forces you to think clearly about the desired behavior upfront. TDD helps ensure your code meets the requirements and keeps your tests focused on functionality. It also encourages simpler, more modular code, as you're essentially designing your code around its testable units.
Continuous Integration (CI) for Unit Tests
Unit tests are ideal for automated testing environments like continuous integration and continuous delivery (CI/CD) pipelines. Because unit tests should be independent and self-contained, they can run quickly and automatically whenever code changes are pushed. This helps catch regressions early, before they make it into production. To maintain this independence, use data stubs–essentially, fake data–when your unit relies on external systems. This ensures your tests remain fast and reliable, even if those external systems are unavailable. Services like MuukTest can seamlessly integrate with your CI/CD workflow, making automated unit testing a breeze.
Balancing Unit Tests with Other Testing Methods
While unit tests are foundational, remember they are just one piece of the testing puzzle. They excel at verifying individual components, but they don't catch everything. You still need other types of testing, like integration tests (which check how different parts of your code work together), functional tests (which verify the software meets requirements), and performance tests (which assess speed and resource usage). Think of unit tests as your first line of defense, catching the majority of bugs early and cheaply. Then, layer on other testing methods to ensure comprehensive coverage and a high-quality product. MuukTest can help you develop a balanced testing strategy that incorporates unit tests alongside other essential testing methods.
The Role of QA in Unit Testing
QA testers play a crucial role in ensuring software quality. While their primary focus is testing the software as a whole, they can also contribute significantly to unit testing, a process typically handled by developers. This often raises the question: should QA be involved in unit testing? In many cases, the answer is yes.
Developers write the unit tests, but QA testers can review them, identify gaps in testing, and suggest improvements (source). This collaboration enhances test coverage, leading to earlier bug detection and better code quality (source). QA's involvement isn't about replacing developers but complementing their efforts. It's about leveraging different skill sets to create higher-quality software (source). QA brings a unique perspective, often considering edge cases and user scenarios that developers might miss. This collaborative approach results in more robust and reliable software. Everyone learns from each other, fostering a more comprehensive testing strategy.
Common Unit Testing Challenges
Unit testing, while beneficial, isn't without its challenges. Understanding common techniques and how to address potential hurdles will make your unit testing efforts more effective.
State-Based vs. Behavior-Based Testing
One fundamental aspect of unit testing is understanding the difference between state-based and behavior-based testing. State-based testing focuses on verifying the state of the system after a series of operations. Think of it like checking the final balance of a bank account after a deposit and withdrawal. Does the account reflect the expected amount? In contrast, behavior-based testing looks at the system's behavior in response to specific inputs. Using the same bank account example, a behavior-based test might check that the correct interest is calculated when a certain balance threshold is met. Choosing the right approach—state-based or behavior-based—depends on the specific functionality you're testing and can significantly influence your test design. Often, a combination of both provides the most comprehensive coverage.
Mock Objects and Stubs
Isolating the unit of work is crucial for effective unit testing. This is where mock objects and stubs come into play. Stubs provide canned responses to calls made during the test, allowing you to control the dependencies of the unit under test. Imagine testing a weather app. Instead of making a live call to a weather API, a stub would return predefined weather data. Mocks, on the other hand, verify interactions between the unit and its dependencies. A mock might confirm that the weather app made the expected call to the weather API with the correct parameters. Using mock objects and stubs effectively helps create more reliable and easier-to-understand unit tests. For more insights into effective testing strategies, explore MuukTest's services on test automation.
Handling Legacy Code and Complex Dependencies
Testing legacy code presents unique challenges. Often, legacy codebases have tightly coupled dependencies, making it difficult to isolate individual units for testing. Refactoring the code to introduce interfaces or using dependency injection can help manage these complexities. Think of it like untangling a knot—carefully separating the strands makes it easier to work with. While refactoring can require an initial time investment, it simplifies testing and improves the overall maintainability and quality of the codebase. Working with existing clients, MuukTest has proven its ability to achieve comprehensive test coverage within 90 days, as showcased on their Customers page.
Overcoming Unit Testing Hurdles
Common pitfalls in unit testing include writing tests that are too complex or neglecting to maintain them as the code evolves. Keeping tests simple and focused ensures they are easy to understand, maintain, and adapt to changes. Just as concise code is easier to debug, concise tests are easier to troubleshoot and update. Prioritizing test maintainability is essential for building a robust and long-lasting testing framework. Remember, tests are just as important as the code they test, and they deserve the same level of care and attention. For those looking to get started quickly, MuukTest offers a QuickStart guide with practical guidance and support. You can also explore their pricing plans to find the best fit for your needs.
When is Unit Testing Not Suitable?
While unit testing offers numerous benefits, it's not a one-size-fits-all solution. There are situations where the costs of implementing and maintaining unit tests might outweigh the advantages. Recognizing these scenarios can help you make informed decisions about your testing strategy.
Tight Deadlines and Small Projects
When you're working against a tight deadline or on a small project, the time investment required to write comprehensive unit tests might not be feasible. If speed is paramount, focusing on delivering core functionality might take precedence over extensive unit testing. In such cases, a more streamlined approach to testing might be necessary, prioritizing the most critical parts of the application. As AWS points out, writing tests takes time, and sometimes, that time is better spent elsewhere.
Design-Centric Projects
If your project is primarily focused on visual design, like the layout and aesthetics of a website, unit testing might not provide the most value. GeeksforGeeks highlights how unit testing excels at verifying the functional logic of code. While some aspects of the user interface *can* be unit tested, the visual elements are often better suited for manual review or other forms of testing like visual regression testing.
Working with Legacy Code
Integrating unit tests into a large, pre-existing legacy codebase can be challenging. Adding tests to legacy code can be difficult and time-consuming, especially if the code wasn't designed with testability in mind. The effort required to refactor the code for testability might outweigh the immediate benefits of unit testing, particularly if the system is stable and not undergoing significant changes. Wikipedia notes these challenges when dealing with legacy systems.
Rapidly Changing Requirements
In projects with frequently changing requirements, maintaining unit tests can become a burden. If the project's goals are constantly shifting, tests might need frequent updates to stay relevant. This constant rework can slow down development and create extra overhead. AWS also mentions this, suggesting that in dynamic environments, constantly updating unit tests might not be the best use of resources. A more agile and adaptable testing approach might be more suitable.
Highly Complex Systems
Certain types of code, such as multi-threaded applications or systems with non-deterministic behavior, can be inherently difficult to unit test effectively. These complex dependencies make it hard to isolate individual units and predict their behavior reliably. While not impossible, the complexity involved in creating and maintaining these tests can be substantial. In such cases, other testing methods, like integration or system testing, might be more appropriate for ensuring the overall functionality and stability of the system. Wikipedia discusses these complexities in more detail.
Unit Testing Tools and Frameworks
Unit testing is more effective and manageable with the right tools and frameworks. They streamline the process, helping you write, organize, and run tests efficiently. This section explores popular frameworks, the advantages of automated tools, and how to choose the right tools for your needs.
Popular Unit Testing Frameworks
Frameworks provide a structured foundation for writing and running unit tests. They offer helpful features like test discovery, setup and teardown routines, and assertions for checking expected outcomes. Using a framework simplifies many aspects of unit testing, making it easier to integrate into your development workflow. Popular unit testing frameworks include JUnit for Java, NUnit for .NET, pytest and unittest for Python, and Jest for JavaScript. These frameworks are often used in conjunction with development methodologies like Agile and Extreme Programming (XP), though unit testing is now a standard practice across many development approaches. A common approach to unit testing is to provide specific input to the program, run the test, and then verify whether the output matches expectations.
Automated Tools and Code Coverage
Automated tools are essential for efficient unit testing. They let you run tests quickly and repeatedly, often integrated with your build process. This helps catch problems early in the development cycle. Automated tests also support continuous integration and continuous delivery (CI/CD) pipelines, ensuring that code changes don't introduce regressions. Another valuable feature of automated tools is code coverage analysis. Code coverage measures how much of your code is actually executed during your unit tests, giving you insights into potential gaps in your testing strategy. High code coverage, while not a guarantee of perfect testing, can increase confidence in your test suite's thoroughness. Unit tests are typically automated, running automatically whenever you modify your code.
Choosing the Right Unit Testing Tools
Selecting the right tools depends on your project's specific needs and the programming language you're using. Consider factors like the size and complexity of your project, your team's familiarity with different tools, and integration with your existing development environment. For instance, if you're working on a Python project, using a framework like pytest or unittest can simplify test creation and management. Remember that automated testing is generally more efficient than manual testing, as automated tests can be executed quickly and repeatedly without manual intervention. Explore different options and choose tools that align with your project's requirements and your team's preferences. MuukTest offers AI-powered test automation services that can further enhance your unit testing efforts, providing comprehensive test coverage and integrating seamlessly with your CI/CD workflows. Learn more about how MuukTest can help you achieve complete test coverage within 90 days by exploring our test automation services.
Unit Testing with MuukTest
MuukTest's AI-powered test automation services can significantly enhance your unit testing efforts. We provide comprehensive test coverage, ensuring that even the smallest parts of your code are thoroughly vetted. This helps catch bugs early in the development cycle, saving you time and resources. Plus, MuukTest integrates seamlessly with your existing CI/CD workflows, automating your unit testing process and providing rapid feedback.
One of the key advantages of using MuukTest is our ability to achieve complete test coverage within 90 days. This rapid turnaround ensures your software is thoroughly tested and ready for release. We work with you to understand your specific needs and tailor our services accordingly. Whether you're working on a small project or a large-scale application, MuukTest can help you achieve your testing goals. Check out our customer success stories to see how we've helped other businesses improve their software quality.
Ready to get started with MuukTest? Our QuickStart guide provides a step-by-step process for integrating MuukTest into your workflow. We also offer a variety of pricing plans to fit your budget.
Measuring and Improving Your Unit Testing
How can you tell if your unit tests are effective? And how can you improve them over time? Here’s how to measure and improve your unit testing efforts:
Code Coverage and Test Quality Metrics
Code coverage measures how much of your codebase your tests actually execute. High code coverage is a good starting point, but it doesn’t guarantee quality tests. Your tests should cover a range of scenarios, including logic checks, boundary checks, error handling, and object checks. For example, if a function expects a positive integer, your tests should check what happens when it receives zero, a negative number, or a very large number. Independent unit tests are more reliable. If your tests rely on external systems or databases, use data stubs—fake data that stands in for the real thing—to keep your tests isolated and fast.
Performance and Efficiency in Unit Testing
Well-written unit tests help ensure correct low-level code and shorten the software development cycle. They also improve developer productivity and lead to more robust software. Automated tests, which run quickly and repeatedly, are key for finding problems early. A common approach is to provide input to the program, run the test, and check if the output matches what’s expected. This helps catch regressions and ensures your code behaves as intended.
Building a Unit Testing Culture
A strong unit testing culture is essential for long-term success. Test-driven development (TDD), where you write tests before the code, is a valuable practice. These tests act as a guide when you write the code, and you run them immediately afterward to verify everything works correctly. Writing unit tests often encourages programmers to write clearer, more organized code. Include your entire team in the process. QA testers should actively participate in reviewing and improving unit tests, which helps ensure a comprehensive and effective testing strategy. This collaborative approach leads to higher quality code and a more robust product.
The Future of Unit Testing
Unit testing constantly evolves alongside software development. Keeping up with the latest trends ensures your testing strategy remains effective and efficient. Let's look at some key advancements shaping the future of unit testing.
AI and Machine Learning in Test Automation
Artificial intelligence (AI) and machine learning (ML) are rapidly transforming software testing. These technologies offer exciting possibilities for automating test case generation, improving test coverage, and predicting potential bugs. AI-powered tools can analyze codebases to identify areas needing more testing and even generate tests automatically. This speeds up the testing process and helps teams achieve more comprehensive test coverage. Expect to see more sophisticated AI and ML integration in testing tools, leading to smarter and more autonomous testing processes. Multimodal AI, which combines different types of AI, is a particularly promising area. As AI and ML mature, they will play an increasingly crucial role in enhancing the efficiency and effectiveness of testing processes.
DevSecOps and Shift-Left Testing
DevSecOps and shift-left testing represent a significant shift in the software development lifecycle. Shift-left emphasizes testing earlier in the development process, ideally starting during code writing. This approach encourages developers to adopt a testing mindset from the beginning, writing unit tests and performing code reviews with a focus on quality. Integrating testing throughout the development pipeline allows teams to catch bugs earlier, reducing the cost and effort of fixing them later. DevSecOps builds upon this by integrating security practices into the DevOps workflow. This means security is no longer an afterthought but an integral part of development. The combination of shift-left testing and DevSecOps leads to faster development cycles, improved software quality, and enhanced security. Cloud services support continuous testing and real-time feedback, essential for Agile and DevOps methodologies. This continuous testing approach ensures new code can be released to production frequently and reliably.
Frequently Asked Questions
What’s the difference between unit testing, integration testing, and functional testing?
Unit testing focuses on verifying the smallest parts of your code (individual functions or modules) in isolation. Integration testing checks how these different units work together. Functional testing verifies that the entire application meets the specified requirements, testing the system as a whole. Think of it like building a house: unit tests check individual bricks, integration tests check how the walls are built, and functional tests check if the entire house is livable.
How much time should I spend on writing unit tests?
While writing unit tests requires an initial time investment, it saves you time and effort in the long run. Think of it as an upfront investment that pays off later. The exact amount of time depends on the complexity of your project, but aim for a good balance between test coverage and development speed. Start with the most critical parts of your code and gradually increase your test coverage over time.
How do I handle unit testing for legacy code?
Legacy code can be challenging to unit test, often due to tightly coupled dependencies. Start by identifying the most critical parts of the legacy code and focus your testing efforts there. Refactoring, while sometimes time-consuming, can make legacy code more testable by introducing interfaces and reducing dependencies. Consider using tools that can help analyze your legacy code and suggest potential refactoring opportunities.
What are some common mistakes to avoid in unit testing?
Common pitfalls include writing tests that are too complex, neglecting to maintain tests as the code evolves, and focusing solely on code coverage without considering test quality. Keep your tests simple, focused, and maintainable. Ensure your tests cover a range of scenarios, including edge cases and error conditions, not just the "happy path".
What's the best way to integrate unit testing into my workflow?
Adopt a test-driven development (TDD) approach, where you write tests before you write the code. This helps you think clearly about the desired behavior and ensures your code meets the requirements. Integrate your unit tests into your continuous integration/continuous delivery (CI/CD) pipeline so they run automatically whenever code changes are pushed. This helps catch regressions early and maintain code quality.
Related Articles
Related Posts:
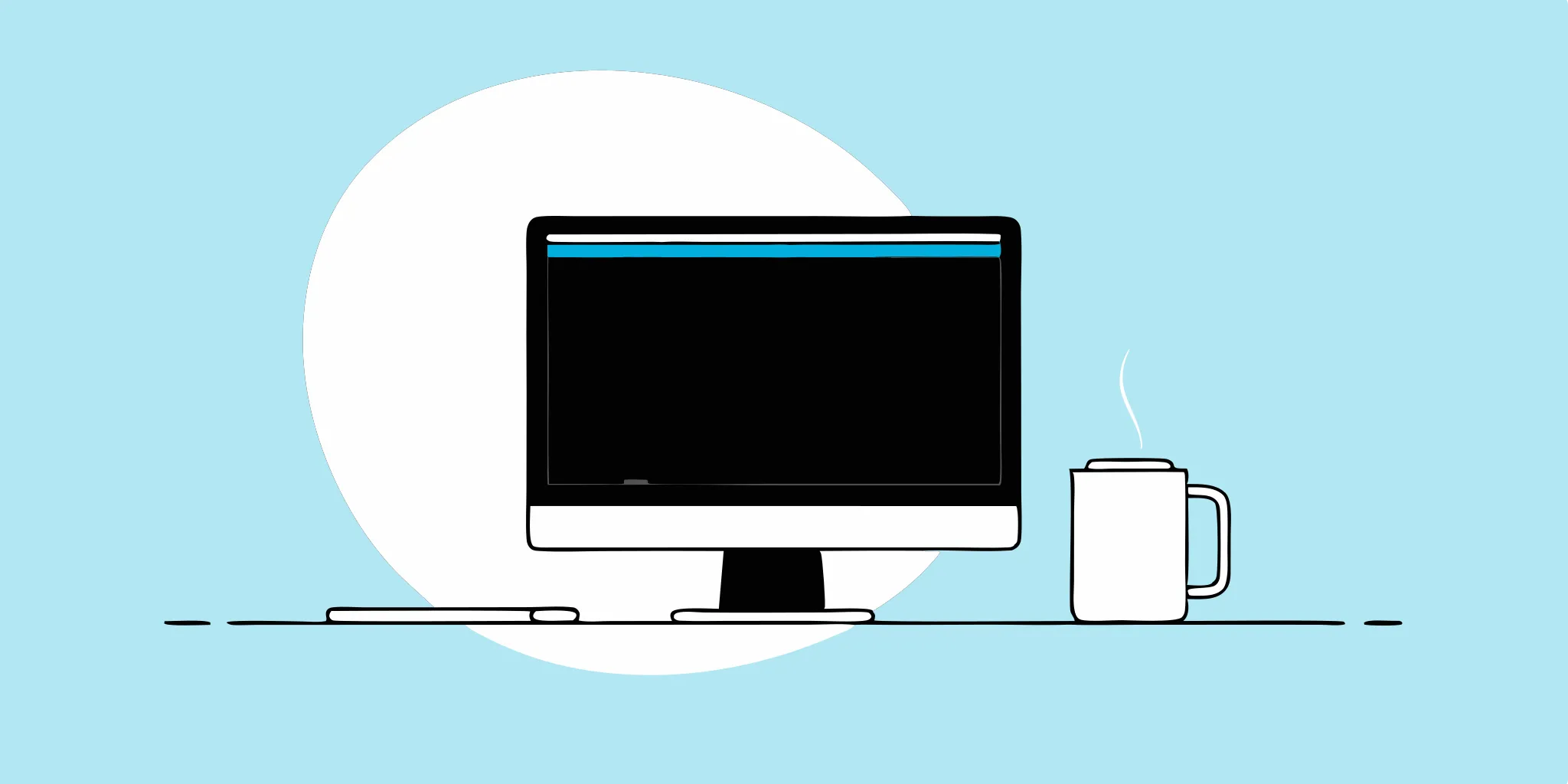
What is Unit Testing? A Practical Guide for Developers
Let's be honest, debugging is nobody's favorite activity. It's time-consuming, frustrating, and can often feel like searching for a needle in a haystack. What if you could prevent many of those bugs...
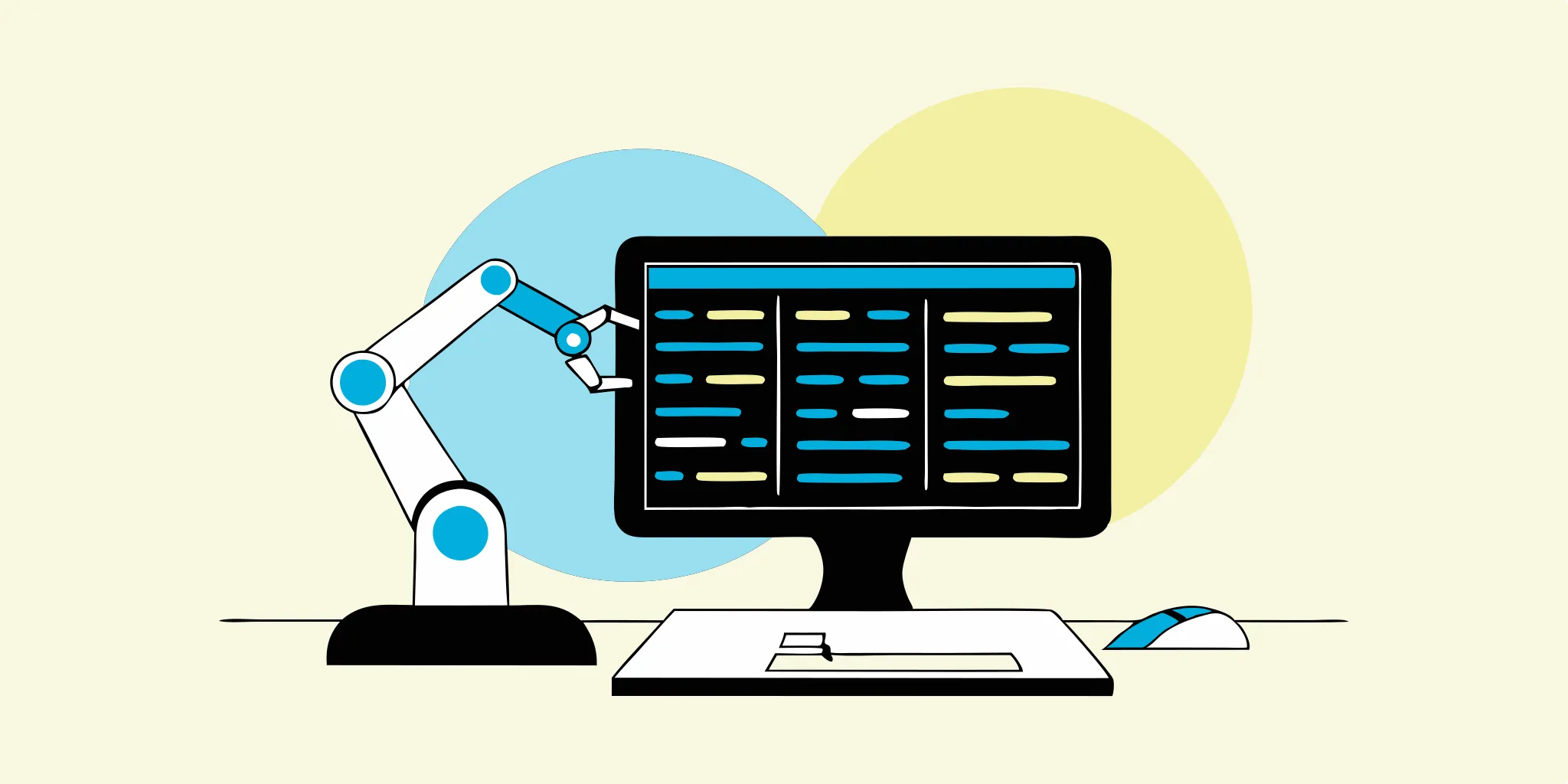
Unit Testing Automation: A Practical Guide
Master unit testing automation with this practical guide, offering insights on best practices, tools, and strategies to enhance your software development process.
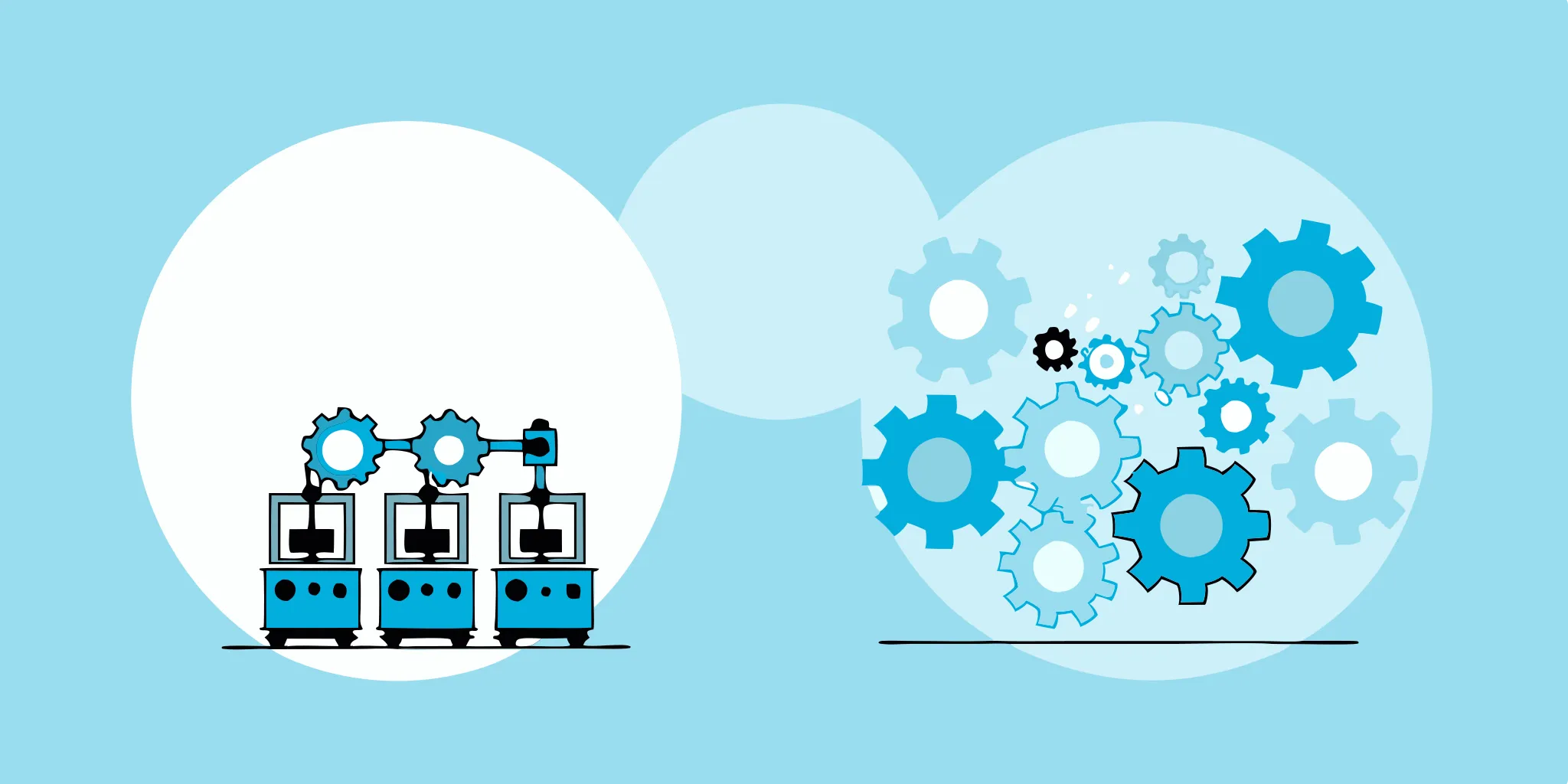
Verify Code Functions Correctly: Unit & Integration Testing
Learn how to verify that the units or pieces of code function correctly when integrated. Explore practical tips for effective unit and integration testing.